19. Three.js案例-创建一个带有纹理映射的旋转平面
实现效果
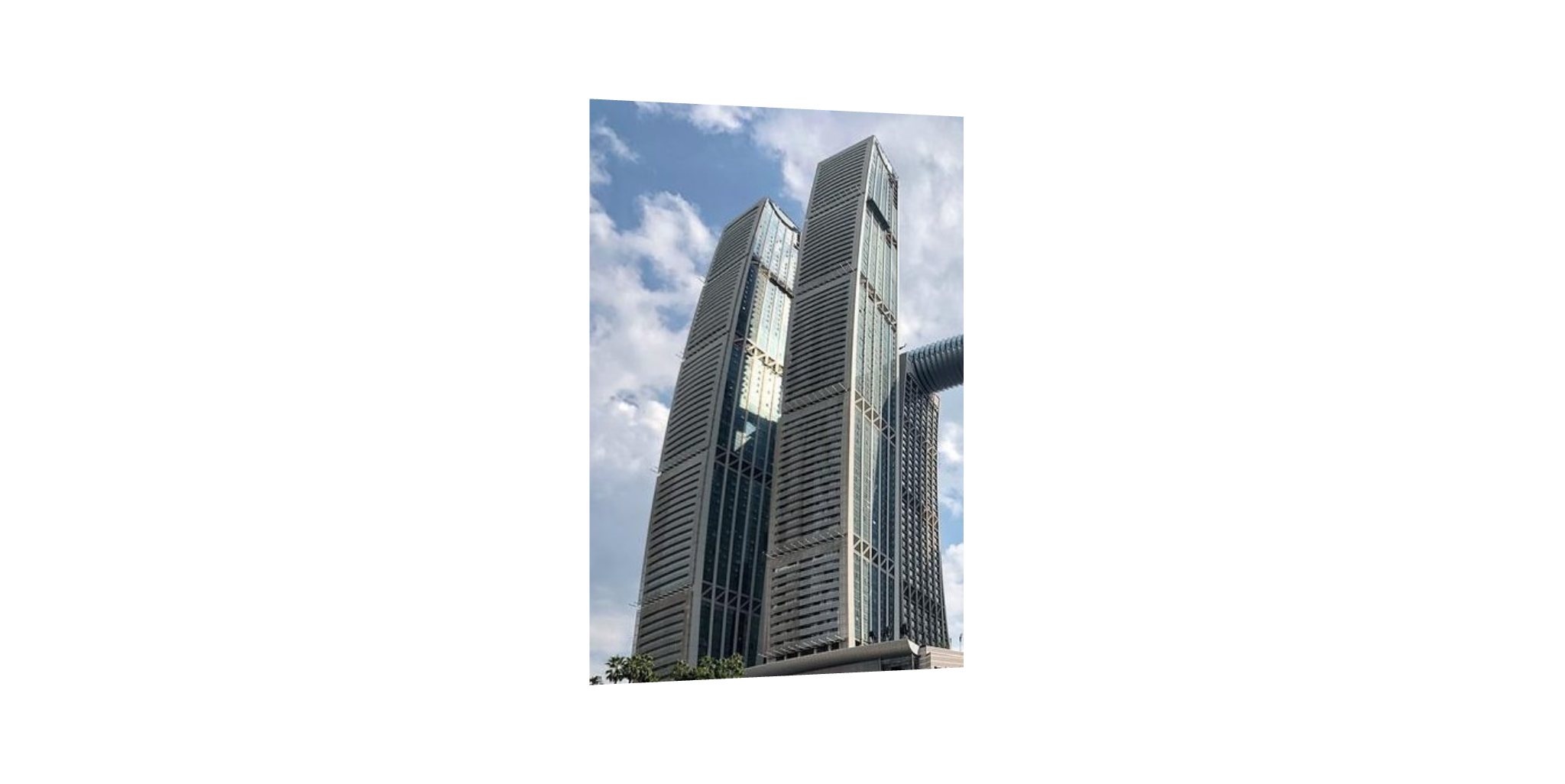
知识点
WebGLRenderer (WebGL渲染器)
WebGLRenderer
是 Three.js 中用于渲染场景的主要类。它利用 WebGL 技术在浏览器中绘制 3D 图形。
构造器
javascript
new THREE.WebGLRenderer(parameters)
参数 | 类型 | 描述 |
---|---|---|
parameters | object | 可选参数对象,用于配置渲染器的各种属性。常用参数包括: |
antialias | bool | 是否开启抗锯齿,默认为 false 。开启后可以提高图像质量,但会增加性能消耗。 |
方法
setSize(width, height)
: 设置渲染器的尺寸。render(scene, camera)
: 渲染指定的场景和相机。
Scene (场景)
Scene
是 Three.js 中用于存储和管理所有 3D 对象的容器。
构造器
javascript
new THREE.Scene()
属性
background
: 场景的背景颜色或环境贴图。
PerspectiveCamera (透视相机)
PerspectiveCamera
是 Three.js 中用于模拟人眼视角的相机类。
构造器
javascript
new THREE.PerspectiveCamera(fov, aspect, near, far)
参数 | 类型 | 描述 |
---|---|---|
fov | float | 视野角度,单位为度。 |
aspect | float | 相机的宽高比,通常设置为 window.innerWidth / window.innerHeight 。 |
near | float | 近裁剪面距离,小于该距离的对象不会被渲染。 |
far | float | 远裁剪面距离,大于该距离的对象不会被渲染。 |
方法
position.set(x, y, z)
: 设置相机的位置。lookAt(vector)
: 设置相机的朝向。
AmbientLight (环境光)
AmbientLight
是 Three.js 中用于模拟环境光的光源类。环境光会使场景中的所有物体均匀受光。
构造器
javascript
new THREE.AmbientLight(color, intensity)
参数 | 类型 | 描述 |
---|---|---|
color | color | 光源的颜色,可以是十六进制颜色值或颜色名称。 |
intensity | float | 光源的强度,默认为 1。 |
PlaneGeometry (平面几何体)
PlaneGeometry
是 Three.js 中用于创建平面几何体的类。
构造器
javascript
new THREE.PlaneGeometry(width, height, widthSegments, heightSegments)
参数 | 类型 | 描述 |
---|---|---|
width | float | 平面的宽度。 |
height | float | 平面的高度。 |
widthSegments | int | 水平方向上的分段数,默认为 1。 |
heightSegments | int | 垂直方向上的分段数,默认为 1。 |
Mesh (网格)
Mesh
是 Three.js 中用于组合几何体和材质的类。
构造器
javascript
new THREE.Mesh(geometry, material)
参数 | 类型 | 描述 |
---|---|---|
geometry | object | 几何体对象。 |
material | object | 材质对象。 |
MeshPhongMaterial (Phong材质)
MeshPhongMaterial
是 Three.js 中用于创建 Phong 照明模型的材质类。
构造器
javascript
new THREE.MeshPhongMaterial(parameters)
参数 | 类型 | 描述 |
---|---|---|
map | texture | 纹理贴图。 |
side | constant | 渲染面的显示方式,可选值为 THREE.FrontSide 、THREE.BackSide 和 THREE.DoubleSide 。 |
ImageUtils (图像工具)
ImageUtils
是 Three.js 中用于加载和处理图像的工具类。
方法
loadTexture(url)
: 加载指定 URL 的图像并返回一个Texture
对象。
动画
requestAnimationFrame
是浏览器提供的 API,用于请求浏览器在下一次重绘之前调用指定的回调函数。
方法
requestAnimationFrame(callback)
: 请求浏览器在下一次重绘之前调用指定的回调函数。
示例代码
html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<script src="ThreeJS/three.js"></script>
<script src="ThreeJS/jquery.js"></script>
</head>
<body>
<center id="myContainer"></center>
<script>
// 创建渲染器
var myRenderer = new THREE.WebGLRenderer({antialias: true});
myRenderer.setSize(window.innerWidth, window.innerHeight);
$("#myContainer").append(myRenderer.domElement);
// 创建场景
var myScene = new THREE.Scene();
myScene.background = new THREE.Color('white');
// 创建相机
var myCamera = new THREE.PerspectiveCamera(45,
window.innerWidth / window.innerHeight, 1, 1000);
myCamera.position.set(0, 0, 400);
myScene.add(myCamera);
// 添加环境光
myScene.add(new THREE.AmbientLight(0xffffff));
// 创建平面
var myPlaneGeometry = new THREE.PlaneGeometry(160, 240, 5, 5);
var myMap = THREE.ImageUtils.loadTexture("images/img137.jpg");
var myPlane = new THREE.Mesh(myPlaneGeometry,
new THREE.MeshPhongMaterial({map: myMap, side: THREE.DoubleSide}));
myScene.add(myPlane);
// 渲染平面
animate();
function animate() {
myRenderer.render(myScene, myCamera);
myPlane.rotation.y += 0.01;
requestAnimationFrame(animate);
}
</script>
</body>
</html>