大佬的博客获取一页数据没有问题。多页请求就会触发猫眼的验证中心,没有数据,所以优化加强了一下,一起进步学习。
ip代理网站:https://www.zdaye.com/free/4/ip(用了selenium这一步骤ip代理可以忽略)
cmd命令 pip install selenium
还需要安装:ChromeDriver安装包到python安装位置
Selenium 需要安装 ChromeDriver:确保你已经安装了与 Chrome 浏览器版本匹配的 ChromeDriver。
地址下载:https://registry.npmmirror.com/binary.html?path=chrome-for-testing
找到对应的谷歌版本
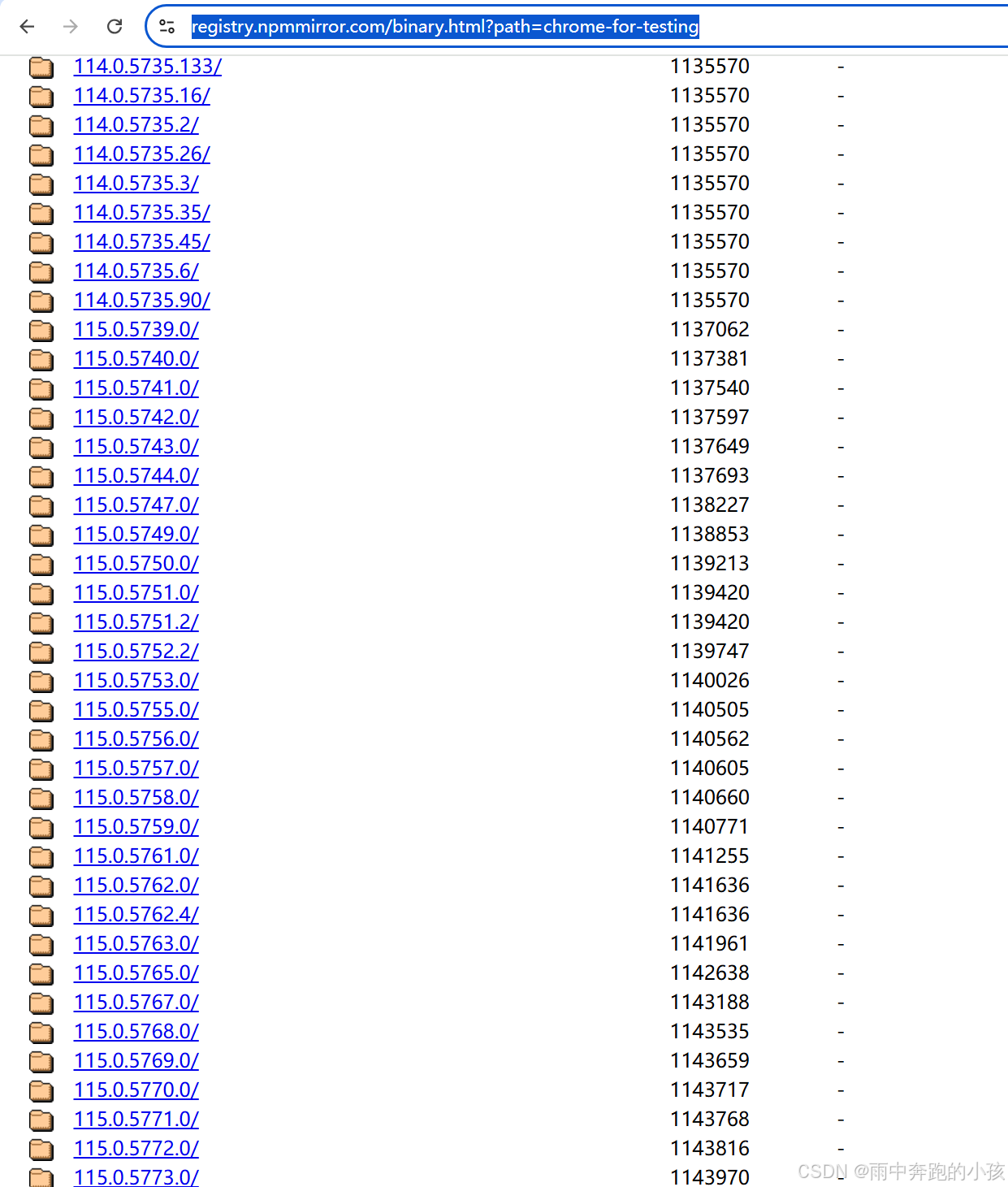
将下载的包解压,把内容全部复制到python的目录下
测试:
python
import time
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("http://www.bing.com")
time.sleep(3)
driver.quit()
运行代码,自动打开浏览器
开始爬取猫眼电影数据
pip安装库
powershell
pip install requests beautifulsoup4 pandas matplotlib seaborn
python
# 导入selenium 库
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
# 导入解析html库BeautifulSoup
from bs4 import BeautifulSoup
# 导入数据分析
import pandas as pd
import matplotlib.pyplot as plt
from matplotlib import rcParams
import seaborn as sns
import re
import time
import random
# 设置Matplotlib使用的字体为SimHei(黑体),以支持中文显示
rcParams['font.sans-serif'] = ['SimHei'] # 使用黑体
rcParams['axes.unicode_minus'] = False # 解决负号 '-' 显示为方块的问题
# 设置目标URL基础部分
base_url = 'https://www.maoyan.com/board/4?offset={}'
# 设置Chrome选项
chrome_options = Options()
chrome_options.add_argument("--headless") # 无头模式,不打开浏览器窗口
chrome_options.add_argument("--disable-gpu")
chrome_options.add_argument("--no-sandbox")
# 设置ChromeDriver路径
service = Service('D:\\env\\python3\\chromedriver.exe')
# 初始化WebDriver
driver = webdriver.Chrome(service=service, options=chrome_options)
# 存储所有电影信息的列表
movies = []
page = 0
# 爬取10页数据,每页偏移量为0, 10, 20, ..., 90
for offset in range(0, 91, 10):
url = base_url.format(offset) # 构造每一页的URL
print(url)
driver.get(url)
time.sleep(random.uniform(5, 10)) # 等待页面加载
# 获取页面源代码
html = driver.page_source
# 提取电影列表
# 使用BeautifulSoup解析HTML
soup = BeautifulSoup(html, 'html.parser')
# print(soup)
print(soup.find_all('dd'))
# 提取电影列表
page += 1
for item in soup.find_all('dd'):
movie = {}
print("正在爬取第{}页数据".format(page))
# 获取电影名称,从a标签的title属性中提取
movie['name'] = item.find('a')['title'].strip() if item.find('a') else 'N/A'
# 获取评分,确保评分字段存在
score_tag = item.find('p', class_='score')
movie['score'] = score_tag.text.strip() if score_tag else 'N/A'
# 获取上映时间,确保上映时间字段存在
release_time_tag = item.find('p', class_='releasetime')
release_time = release_time_tag.text.strip() if release_time_tag else 'N/A'
# 使用正则表达式清洗数据,提取年份部分
movie['release_time'] = re.findall(r'\d{4}', release_time) # 匹配年份
if movie['release_time']:
movie['release_time'] = movie['release_time'][0] # 只取第一个年份
else:
movie['release_time'] = 'N/A' # 如果没有找到年份,设置为'N/A'
# 将电影信息添加到列表中
movies.append(movie)
# 关闭浏览器
driver.quit()
# 将数据存储到pandas DataFrame
df = pd.DataFrame(movies)
# 输出前5行数据
# print("爬取的数据:")
# print(df.head())
# 数据清洗:去除空值并处理评分数据
df.dropna(subset=['score', 'release_time'], inplace=True) # 删除评分和上映时间为空的行
# 将评分转换为数值类型,无法转换的设置为NaN
df['score'] = pd.to_numeric(df['score'], errors='coerce')
# 删除评分为空的行
df.dropna(subset=['score'], inplace=True)
# 将release_time列转换为数值类型的年份
df['release_year'] = pd.to_numeric(df['release_time'], errors='coerce')
# 输出清洗后的数据
# print("清洗后的数据:")
# print(df.head())
# 保存数据为CSV文件
df.to_csv('maoyan_top100.csv', index=False)
# 数据分析:电影评分分布
plt.figure(figsize=(8, 6))
sns.histplot(df['score'], bins=20, kde=True)
plt.title('电影评分分布')
plt.xlabel('评分')
plt.ylabel('数量')
plt.show()
# 数据分析:电影上映年份分布
plt.figure(figsize=(10, 6))
sns.countplot(x='release_year', data=df)
plt.title('电影上映年份分布')
plt.xticks(rotation=45)
plt.xlabel('年份')
plt.ylabel('电影数量')
plt.show()
# 结束
print("爬取和分析完成!数据已保存至 maoyan_top100.csv")