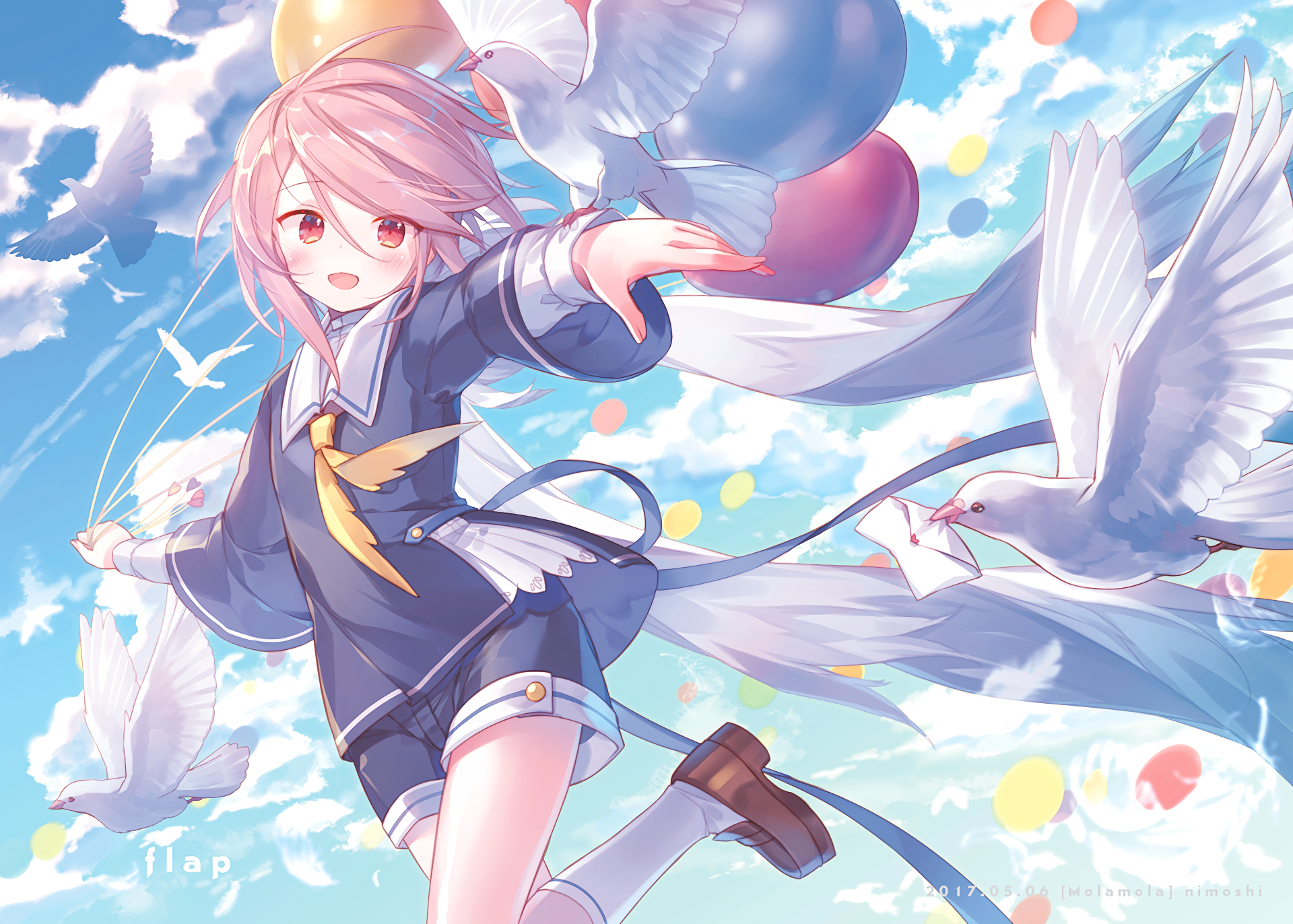
镇楼图↑
在前一篇博客中,我们学习了Python的基础知识。本篇博客将进一步探索Python的进阶特性,包括数据结构、面向对象编程、文件操作和简单的异常处理。通过这些内容,你将能够解决更复杂的问题。
一、数据结构
1. 列表(List)
列表是一个可变的、有序的数据集合,可以存储不同类型的元素。
示例代码:
python
# 创建列表
fruits = ["apple", "banana", "cherry"]
# 添加元素
fruits.append("orange")
# 删除元素
fruits.remove("banana")
# 遍历列表
for fruit in fruits:
print(fruit)
分析:
append()
:在列表末尾添加元素。remove()
:删除指定的元素。
运行结果:
apple
cherry
orange
2. 字典(Dictionary)
字典用于存储键值对,类似于现实中的词典。
示例代码:
python
# 创建字典
student = {"name": "Alice", "age": 20, "grade": "A"}
# 访问键值
print("Name:", student["name"])
# 修改值
student["age"] = 21
# 遍历字典
for key, value in student.items():
print(f"{key}: {value}")
分析:
student["name"]
:通过键访问值。.items()
:返回所有的键值对。
运行结果:
Name: Alice
name: Alice
age: 21
grade: A
3. 集合(Set)
集合是一个无序且不重复的元素集合。
示例代码:
python
# 创建集合
numbers = {1, 2, 3, 4}
# 添加元素
numbers.add(5)
# 移除元素
numbers.remove(3)
# 集合运算
other_numbers = {3, 4, 5, 6}
print("交集:", numbers & other_numbers)
print("并集:", numbers | other_numbers)
print("差集:", numbers - other_numbers)
分析:
- 集合运算 :使用
&
(交集)、|
(并集)、-
(差集)操作。
运行结果:
交集: {4, 5}
并集: {1, 2, 4, 5, 6}
差集: {1, 2}
二、面向对象编程(OOP)
1. 创建类和对象
Python是支持面向对象编程的语言,类和对象是其核心概念。
示例代码:
python
class Student:
# 初始化方法
def __init__(self, name, age):
self.name = name
self.age = age
# 方法
def introduce(self):
print(f"My name is {self.name} and I am {self.age} years old.")
# 创建对象
student1 = Student("Alice", 20)
student2 = Student("Bob", 22)
# 调用方法
student1.introduce()
student2.introduce()
分析:
__init__
方法:在创建对象时自动调用,用于初始化属性。self
:表示类的实例对象,所有方法的第一个参数必须是self
。
运行结果:
My name is Alice and I am 20 years old.
My name is Bob and I am 22 years old.
2. 继承
通过继承可以创建新的类,同时复用已有类的功能。
示例代码:
python
# 父类
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
print(f"{self.name} makes a sound.")
# 子类
class Dog(Animal):
def speak(self):
print(f"{self.name} barks.")
# 创建对象
dog = Dog("Buddy")
dog.speak()
分析:
- 子类
Dog
继承了父类Animal
,并重写了speak()
方法。
运行结果:
Buddy barks.
三、文件操作
Python可以轻松地读写文件。
示例代码:
python
# 写入文件
with open("example.txt", "w") as file:
file.write("Hello, Python!\nThis is a sample file.")
# 读取文件
with open("example.txt", "r") as file:
content = file.read()
print("File content:")
print(content)
分析:
open()
:打开文件,模式"w"
表示写入,"r"
表示读取。with
语句:保证文件操作完成后自动关闭文件。
运行结果:
File content:
Hello, Python!
This is a sample file.
四、异常处理
当程序出现错误时,Python提供了捕获异常的机制。
示例代码:
python
try:
num = int(input("Enter a number: "))
result = 10 / num
print("Result:", result)
except ValueError:
print("Invalid input! Please enter a number.")
except ZeroDivisionError:
print("Division by zero is not allowed.")
finally:
print("Program ended.")
分析:
try
:尝试执行可能出错的代码。except
:捕获并处理特定类型的异常。finally
:无论是否发生异常,都会执行的代码块。
运行示例:
Enter a number: 0
Division by zero is not allowed.
Program ended.
五、综合实例:学生成绩管理系统
示例代码:
python
class Student:
def __init__(self, name, grades):
self.name = name
self.grades = grades
def average(self):
return sum(self.grades) / len(self.grades)
students = [
Student("Alice", [85, 90, 78]),
Student("Bob", [92, 88, 84]),
Student("Charlie", [70, 65, 80])
]
# 输出学生成绩
for student in students:
print(f"{student.name}'s average grade: {student.average():.2f}")
分析:
- **
sum()
**和len()
:分别计算列表的总和和长度。 - 列表存储对象 :每个学生是一个
Student
对象。
运行结果:
Alice's average grade: 84.33
Bob's average grade: 88.00
Charlie's average grade: 71.67
六、总结
通过本篇博客,你学习了Python的一些进阶知识:
- 常用的数据结构及操作(列表、字典、集合)。
- 面向对象编程的基础(类与对象、继承)。
- 文件的读写操作。
- 异常处理机制。
这些知识将帮助你在编写复杂程序时更加游刃有余。继续实践和探索吧,Python的世界充满了可能性!