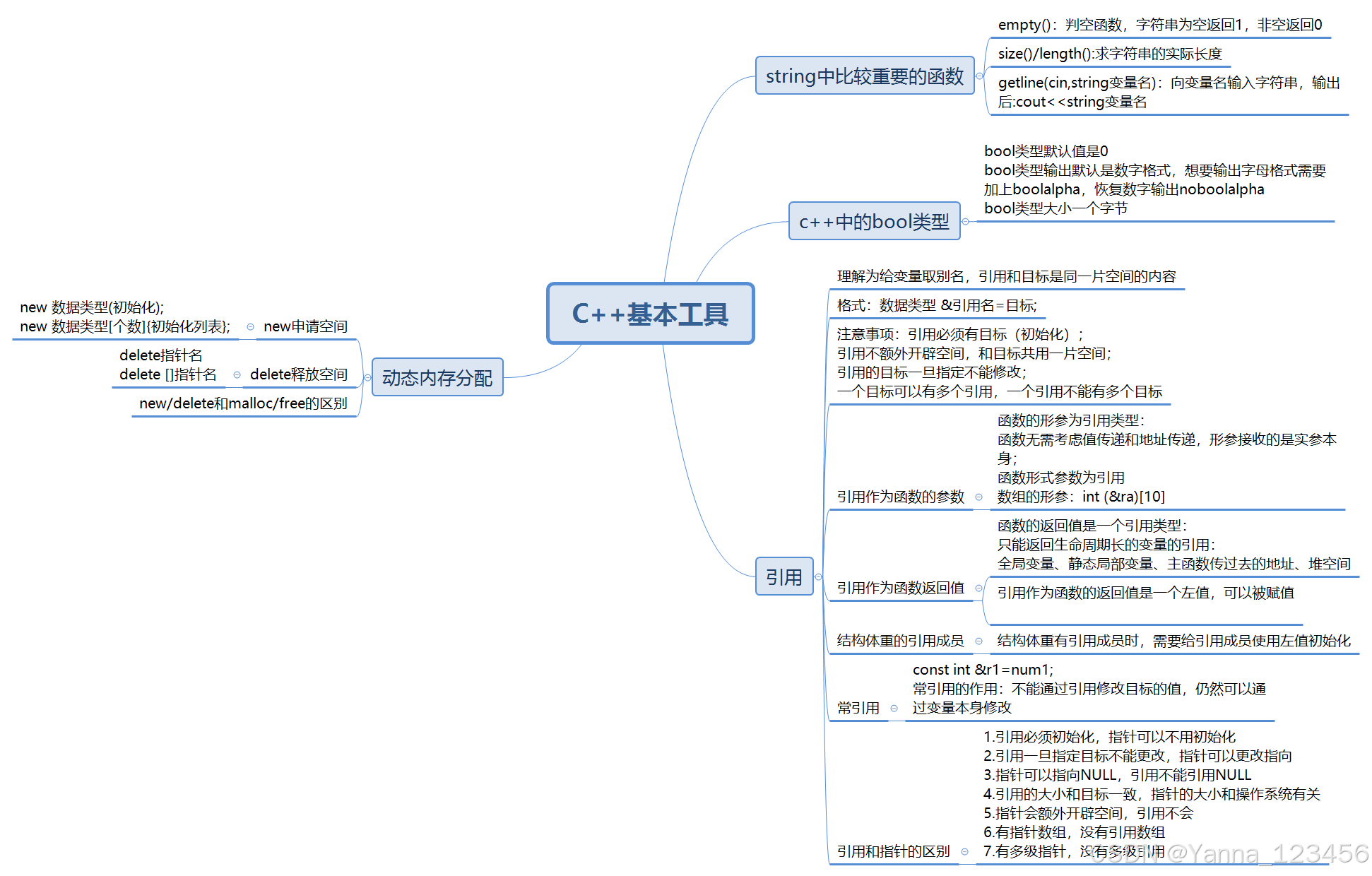
利用函数重载实现冒泡排序:
cpp
#include <iostream>
using namespace std;
void sort(int a[],int len)
{
int i,j,t;
for(i=1;i<len;i++)
{
for(j=0;j<len-i;j++)
{
if(a[j]>a[j+1])
{
t=a[j];
a[j]=a[j+1];
a[j+1]=t;
}
}
}
cout<<"整数数组:";
for(i=0;i<len;i++)
{
cout<<a[i]<<"\t";
}
cout<<endl;
}
void sort(float a[],int len)
{
int i,j;
float t;
for(i=1;i<len;i++)
{
for(j=0;j<len-i;j++)
{
if(a[j]>a[j+1])
{
t=a[j];
a[j]=a[j+1];
a[j+1]=t;
}
}
}
cout<<"浮点数数组:";
for(i=0;i<len;i++)
{
cout<<a[i]<<"\t";
}
cout<<endl;
}
int main()
{
int a[5]={7,9,1,3,6};
int len1=sizeof(a)/sizeof(int);
float b[5]={3.5,4.1,1,6.6,0};
int len2=sizeof(b)/sizeof(float);
sort(a,len1);
sort(b,len2);
cout << "Hello World!" << endl;
return 0;
}
在堆区申请一个数组的空间,并完成对该数组中数据的输入和输出,程序结束释放堆区空间
cpp
#include <iostream>
using namespace std;
int main()
{
int *p=new int[5];
int i;
for(i=0;i<5;i++)
{
cin>>p[i];
}
for(i=0;i<5;i++)
{
cout<<p[i]<<"\t";
}
cout<<endl;
delete []p;
cout << "Hello World!" << endl;
return 0;
}
已知一个数组table,用宏定义,求出数据的元素个数
c
#include <myhead.h>
#define ARR_SIZE(arr) sizeof(arr)/sizeof(arr[0])
int main(int argc, const char *argv[])
{
int table[]={9,7,0,2,1,88};
int size=ARR_SIZE(table);
printf("%d\n",size);
return 0;
}
给定一个整型变量a,写两段代码,第一个设置a的bit3,第二个清除a的bit3
c
#include <myhead.h>
int main(int argc, const char *argv[])
{
int a=0;
int mask=1<<3;
a=a|mask;
printf("a=%d\n",a);
a=31;
mask=1<<3;
mask=~mask;
a=a&mask;
printf("a=%d\n",a);
return 0;
}