1.创建cocoa touch class
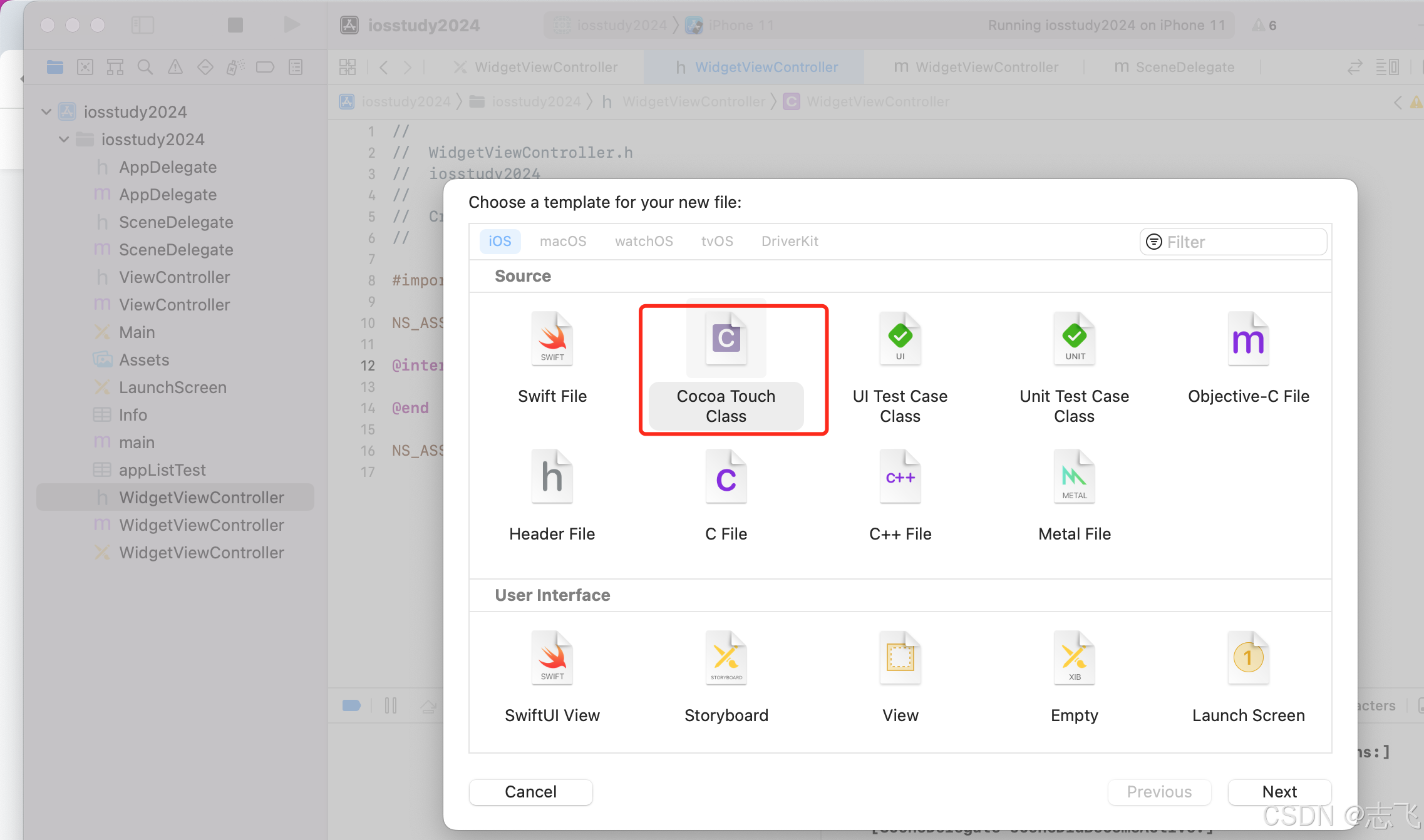
2.同时创建xib页面
3.SceneDelegate设置根视图控制器
objectivec
//
// SceneDelegate.m
// iosstudy2024
//
// Created by figo on 2024/8/5.
//
#import "SceneDelegate.h"
#import "WidgetViewController.h"
@interface SceneDelegate ()
@end
@implementation SceneDelegate
- (void)scene:(UIScene *)scene willConnectToSession:(UISceneSession *)session options:(UISceneConnectionOptions *)connectionOptions {
// Use this method to optionally configure and attach the UIWindow `window` to the provided UIWindowScene `scene`.
// If using a storyboard, the `window` property will automatically be initialized and attached to the scene.
// This delegate does not imply the connecting scene or session are new (see `application:configurationForConnectingSceneSession` instead).
//默认是使用ViewController,这里改成WidgetViewController为根视图
WidgetViewController * wvc = [[WidgetViewController alloc]init];
//[self.window setRootViewController:wvc];
self.window.rootViewController=wvc;
}
- (void)sceneDidDisconnect:(UIScene *)scene {
// Called as the scene is being released by the system.
// This occurs shortly after the scene enters the background, or when its session is discarded.
// Release any resources associated with this scene that can be re-created the next time the scene connects.
// The scene may re-connect later, as its session was not necessarily discarded (see `application:didDiscardSceneSessions` instead).
}
- (void)sceneDidBecomeActive:(UIScene *)scene {
// Called when the scene has moved from an inactive state to an active state.
// Use this method to restart any tasks that were paused (or not yet started) when the scene was inactive.
NSLog(@"%s",__func__);
}
- (void)sceneWillResignActive:(UIScene *)scene {
// Called when the scene will move from an active state to an inactive state.
// This may occur due to temporary interruptions (ex. an incoming phone call).
NSLog(@"%s",__func__);
}
- (void)sceneWillEnterForeground:(UIScene *)scene {
// Called as the scene transitions from the background to the foreground.
// Use this method to undo the changes made on entering the background.
NSLog(@"%s",__func__);
}
- (void)sceneDidEnterBackground:(UIScene *)scene {
// Called as the scene transitions from the foreground to the background.
// Use this method to save data, release shared resources, and store enough scene-specific state information
// to restore the scene back to its current state.
NSLog(@"%s",__func__);
}
@end
4.设计xib页面
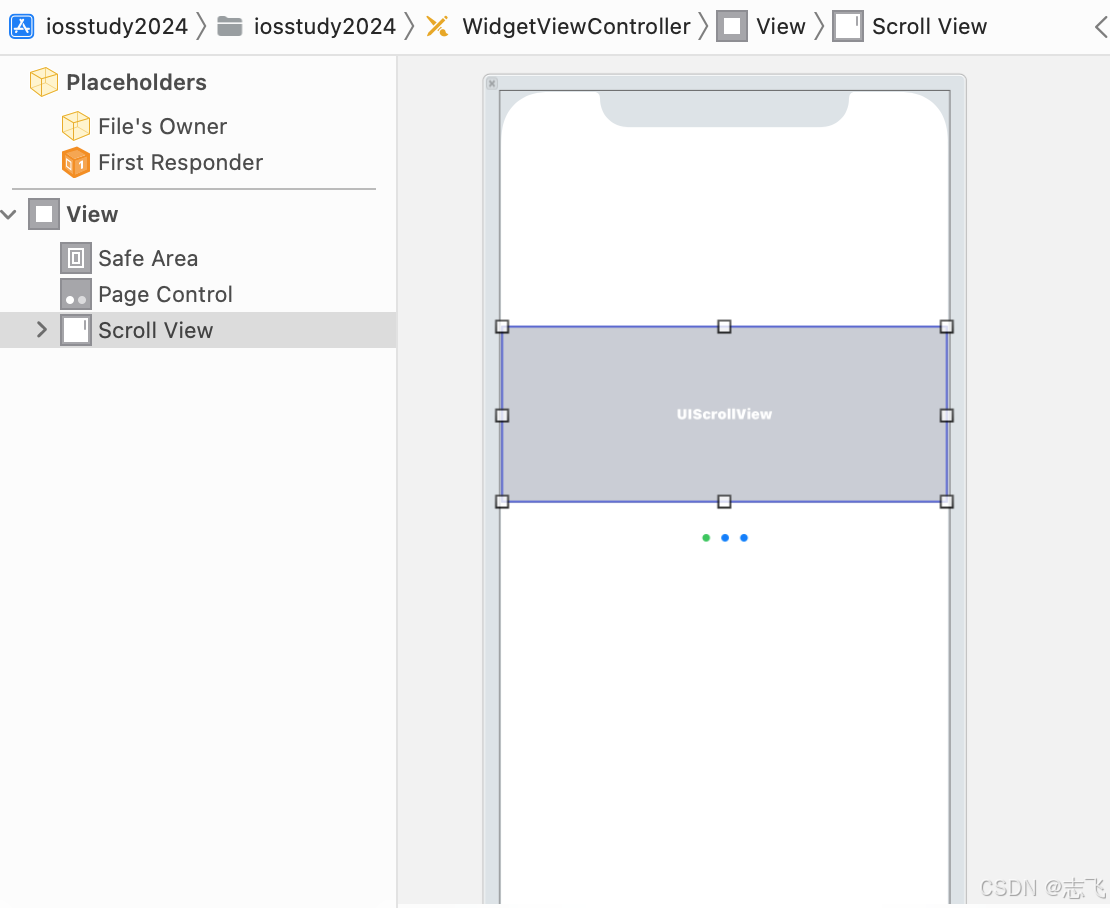
5.编写WidgetViewController
objectivec
//
// WidgetViewController.m
// iosstudy2024
//
// Created by figo on 2024/12/5.
//
#import "WidgetViewController.h"
@interface WidgetViewController ()<UIScrollViewDelegate>
@property (weak, nonatomic) IBOutlet UIScrollView *scrollViewTest;
@property (weak, nonatomic) IBOutlet UIPageControl *pageControll;
@end
@implementation WidgetViewController
- (void)viewDidLoad {
[super viewDidLoad];
//1.实现了UIScrollViewDelegate,代理设置成自己
self.scrollViewTest.delegate=self;
//2.获取屏幕宽度
float width=[UIScreen mainScreen].bounds.size.width;
//3.设置scrollView宽和高
[self.scrollViewTest setFrame:CGRectMake(0, 200, width, 200)];
self.scrollViewTest.contentSize=CGSizeMake(3*width, 200);
//4.添加3张imgView,imgView相对scrollView来说,那么它的左上角坐标是0,0,不要设置错,否则会不显示
self.pageControll.numberOfPages=3;//这里设置页数,同图片数量,这里简单的添加3张
UIImageView *imgView=[[UIImageView alloc]initWithFrame:CGRectMake(0, 0, width, 200)];
imgView.image=[UIImage imageNamed:@"star"];
[self.scrollViewTest addSubview:imgView];
UIImageView *imgView1=[[UIImageView alloc]initWithFrame:CGRectMake(0+width, 0, width, 200)];
imgView1.image=[UIImage imageNamed:@"diamond"];
[self.scrollViewTest addSubview:imgView1];
UIImageView *imgView2=[[UIImageView alloc]initWithFrame:CGRectMake(0+2*width, 0, width, 200)];
imgView2.image=[UIImage imageNamed:@"grape"];
[self.scrollViewTest addSubview:imgView2];
}
//5.通过scrollView滑动偏移量计算pageIndex
-(void)scrollViewDidEndDecelerating:(UIScrollView *)scrollView{
CGFloat offset=self.scrollViewTest.contentOffset.x;
NSInteger index=offset/[UIScreen mainScreen].bounds.size.width;
self.pageControll.currentPage=index;
}
/*
#pragma mark - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender {
// Get the new view controller using [segue destinationViewController].
// Pass the selected object to the new view controller.
}
*/
@end
6.运行效果
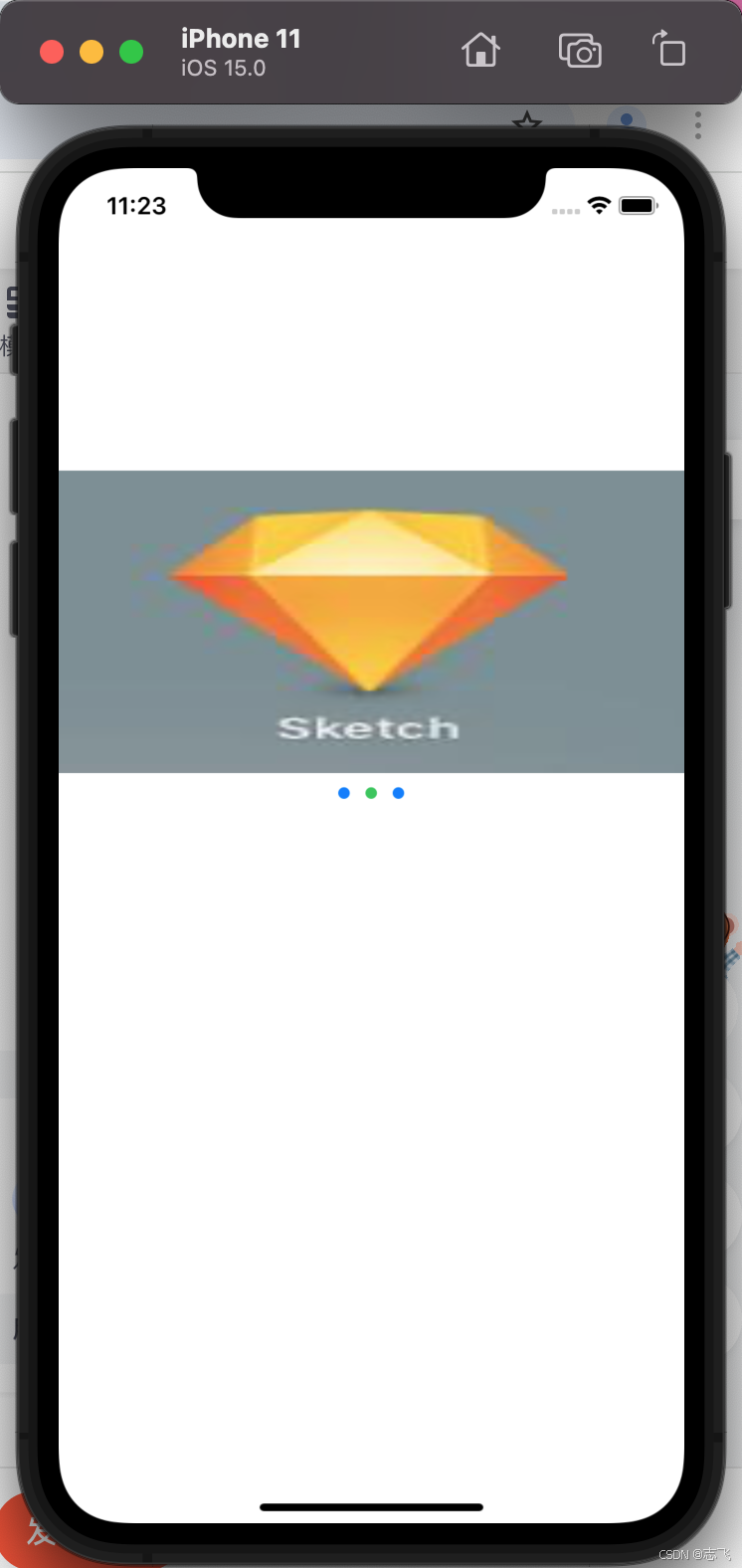