作业:
整理思维导图
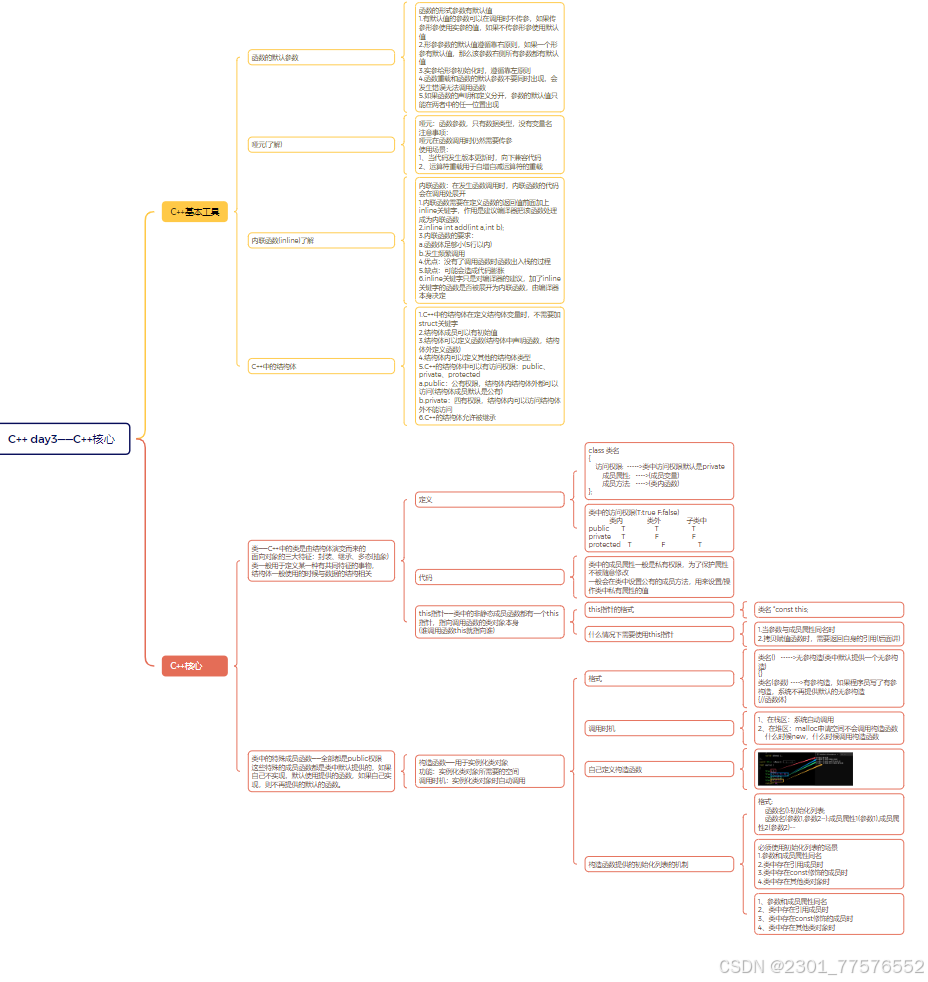
2、整理课上代码
3、把课上类的三个练习题的构造函数写出来
1、定义一个矩形类Rec
cpp
#include <iostream>
using namespace std;
class Rec
{
const int length;
int width;
public:
Rec(int length,int width):length(length),width(width)
{
cout<<"长度为:"<<length<<endl;
cout<<"宽度为:"<<width<<endl;
}
void show();
};
void Rec::show()
{
cout << "周长为:" << 2*(length+width) << endl;
cout << "面积是:" << length*width << endl;
}
int main()
{
int a,b;
cout<<"请输入长度:"<<endl;
cin>>a;
cout<<"请输入宽度:"<<endl;
cin>>b;
Rec s1(a,b);
s1.show();
return 0;
}
2、定义一个圆类
cpp
#include <iostream>
using namespace std;
class Cir
{
int &r;
public:
Cir (int &r):r(r)
{cout<<"有参构造"<<endl;}
void show(double PI=3.14);
};
void Cir::show(double PI)
{
cout << "周长:" << 2*r*PI << endl;
cout << "面积:" << r*r*PI << endl;
}
int main()
{
int r;
cout<<"请输入半径:"<<endl;
cin>>r;
Cir p1(r);
p1.show();
return 0;
}
3、定义一个Car类
cpp
#include <iostream>
using namespace std;
class Car
{
string color;
string brand;
int speed;
public:
Car (string color,string brand,int speed):color(color),brand(brand),speed(speed)
{
cout<<"有参构造"<<endl;
cout << "color: " << color << endl;
cout << "brand: " << brand << endl;
cout << "speed: " << speed << endl;
}
void acc(int a);
};
//给车加速
void Car::acc(int a)
{
speed+=a;
cout << "speed: " << speed << endl;
}
int main()
{
string c,b;
int s;
cout << "color: " << endl;
cin>>c;
cout << "brand: " << endl;
cin>>b;
cout << "speed: " << endl;
cin>>s;
Car c1(c,b,s);
int a;
cout<<"车的加速度为"<<endl;
cin>>a;
c1.acc(a);
return 0;
}