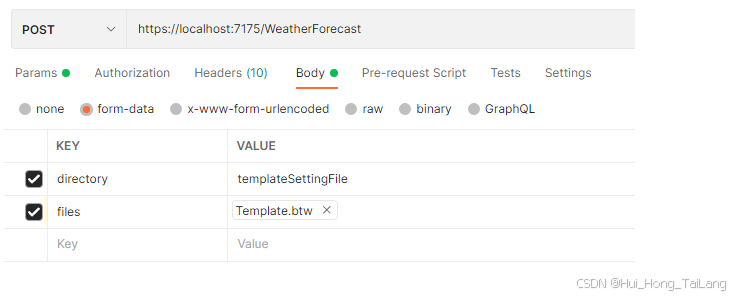
请求发起
.NET Framework 3.5 版
csharp
/// <summary>
/// 使用multipart/form-data方式上传文件及其他数据
/// </summary>
/// <param name="headers">请求头参数</param>
/// <param name="nameValueCollection">键值对参数</param>
/// <param name="fileCollection">文件参数:参数名,文件路径</param>
/// <returns>接口返回结果</returns>
public static string PostMultipartFormData(string url, Dictionary<string, string> headers, NameValueCollection nameValueCollection, NameValueCollection fileCollection)
{
using (var client = new HttpClient())
{
foreach (var item in headers)
{
client.DefaultRequestHeaders.Add(item.Key, item.Value);
}
using (var content = new MultipartFormDataContent())
{
// 键值对参数
string[] allKeys = nameValueCollection.AllKeys;
foreach (string key in allKeys)
{
var dataContent = new ByteArrayContent(Encoding.UTF8.GetBytes(nameValueCollection[key]));
dataContent.Headers.ContentDisposition = new ContentDispositionHeaderValue("form-data")
{
Name = key
};
content.Add(dataContent);
}
//处理文件内容
string[] fileKeys = fileCollection.AllKeys;
foreach (string key in fileKeys)
{
byte[] bmpBytes = File.ReadAllBytes(fileCollection[key]);
var fileContent = new ByteArrayContent(bmpBytes);//填充文件字节
fileContent.Headers.ContentDisposition = new ContentDispositionHeaderValue("form-data")
{
Name = key,
FileName = Path.GetFileName(fileCollection[key])
};
content.Add(fileContent);
}
var result = client.PostAsync(url, content).Result;//post请求
string data = result.Content.ReadAsStringAsync().Result;
return data;//返回操作结果
}
}
}
.NET Framework 4.+ 版
csharp
/// <summary>
/// 使用multipart/form-data方式上传文件及其他数据
/// </summary>
/// <param name="headers">请求头参数</param>
/// <param name="nameValueCollection">键值对参数</param>
/// <param name="fileCollection">文件参数:参数名,文件路径</param>
/// <returns>接口返回结果</returns>
public static string PostMultipartFormData(string url, Dictionary<string, string> headers, NameValueCollection nameValueCollection, NameValueCollection fileCollection)
{
using (var client = new HttpClient())
{
foreach (var item in headers)
{
client.DefaultRequestHeaders.Add(item.Key, item.Value);
}
using (var content = new MultipartFormDataContent())
{
// 键值对参数
string[] allKeys = nameValueCollection.AllKeys;
foreach (string key in allKeys)
{
var dataContent = new ByteArrayContent(Encoding.UTF8.GetBytes(nameValueCollection[key]));
dataContent.Headers.ContentDisposition = new ContentDispositionHeaderValue("form-data")
{
Name = key
};
content.Add(dataContent);
}
//处理文件内容
string[] fileKeys = fileCollection.AllKeys;
foreach (string key in fileKeys)
{
byte[] bmpBytes = File.ReadAllBytes(fileCollection[key]);
var fileContent = new ByteArrayContent(bmpBytes);//填充文件字节
fileContent.Headers.ContentDisposition = new ContentDispositionHeaderValue("form-data")
{
Name = key,
FileName = Path.GetFileName(fileCollection[key])
};
content.Add(fileContent);
}
var result = client.PostAsync(url, content).Result;//post请求
string data = result.Content.ReadAsStringAsync().Result;
return data;//返回操作结果
}
}
}
Web API 接收接口
ASP .NET Core Web API 接口接收 multipart/form-data 文件、数据
csharp
[HttpPost]
//public string Post([FromForm] string directory, [FromForm] IList<IFormFile> files )
public string Post([FromForm] string directory, [FromForm] IFormFile files)
{
return "";
}
[HttpPut]
public string Put([FromForm] IFormFile templateFile, [FromForm] string id, [FromForm] string objectVersionNumber)
{
return "";
}
ASP.NET 4.x 版
csharp
[HttpPost(Name = "PostWeatherForecast")]
//public string Post([FromForm] string directory, [FromForm] IList<IFormFile> files )
public string Post([FromForm] string directory, [FromForm] IFormFile files)
{
// Check if the request contains multipart/form-data.
if (!Request.ContentType.IsMimeMultipartContent())
{
throw new HttpResponseException(HttpStatusCode.UnsupportedMediaType);
}
string root = Request.HttpContext.Current.Server.MapPath("~/App_Data");
var provider = new MultipartFormDataStreamProvider(root);
try
{
// Read the form data.
await Request.Content.ReadAsMultipartAsync(provider);
// This illustrates how to get the file names.
//foreach (MultipartFileData file in provider.FileData)
//{
// Trace.WriteLine(file.Headers.ContentDisposition.FileName);
// Trace.WriteLine("Server file path: " + file.LocalFileName);
//}
// return Request.CreateResponse(HttpStatusCode.OK);
}
catch (System.Exception e)
{
// return Request.CreateErrorResponse(HttpStatusCode.InternalServerError, e);
}
return "";
}