工作中需要利用padlist + Chip size + Chip Center + Pixel size + Pixel Center生成一副Chip Layout tool SVG图像
1.参数输入
cpp
QtColorPropertyManager *m_colorManager = nullptr;
QtDoublePropertyManager *m_doubleManager = nullptr;
QtBoolPropertyManager *m_boolManager = nullptr;
QtStringPropertyManager *m_stringManager = nullptr;
QtFontPropertyManager *m_fontManager = nullptr;
使用各个QT property manager去生成各个UI界面,确定输入的参数的类型,颜色,字体
2.使用QGraphicsView去画图,
所有的对象堆叠在Widget上,每个widget上会堆叠不同的group,group中包含一个个具有共同属性的Item
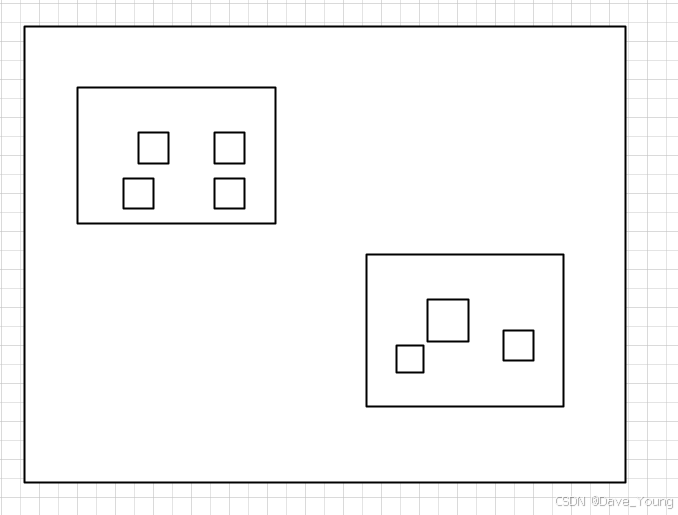
注意设定可交互性,背景大小,抗锯齿,可拖动性
cpp
ChipLayoutWidget::ChipLayoutWidget(QWidget* parent): QGraphicsView(parent)
{
m_scene = new QGraphicsScene(0, 0, 7200, 7200);
QColor transparentColor(Qt::transparent);
QBrush transparentBrush(transparentColor);
m_scene->setBackgroundBrush(transparentBrush);
this->setInteractive(true);
QMap<ChipLayoutGroup::ChipLayoutGroupType, ChipLayoutGroup *> m_groupList = {};
for(int i = 0; i < ChipLayoutGroup::GroupTypeDefaut; i++)
{
m_groupMap[ChipLayoutGroup::ChipLayoutGroupType(i)] = new ChipLayoutGroup();
m_groupMap[ChipLayoutGroup::ChipLayoutGroupType(i)]->setFlag(QGraphicsItem::ItemIsSelectable,
false);
m_scene->addItem(m_groupMap[ChipLayoutGroup::ChipLayoutGroupType(i)]);
}
this->setRenderHint(QPainter::Antialiasing);
m_scene->setSceneRect(0, 0, 7200, 7200);
this->setScene(m_scene);
m_scene->setBackgroundBrush(QColor(0x33, 0x33, 0x33));
this->setDragMode(QGraphicsView::ScrollHandDrag);
this->setCacheMode(QGraphicsView::CacheBackground);
m_scene->update();
}
3.遇到的需要注意的一些问题
- 为什么有时候我的滑动没有能够及时刷新
QGraphicsItem内的一个函数必须要override正确
cpp
QRectF boundingRect() const override;
- 不需要单独的为每一个Item设置属性,比如是否被选中,可以直接用group去做操作
- 放大缩小view的code
cpp
qreal Widget::getScale()
{
return syncScaledValue;
}
void Widget::setScale(qreal scaled)
{
if (!qFuzzyCompare(scaled , this->syncScaledValue))
{
LOGD(QString("%1").arg(scaled));
this->syncScaledValue = scaled;
update();
}
}
void Widget::setRelatedScale(qreal relatedScale)
{
if (!qFuzzyCompare(relatedScale , this->relatedScaleValue))
{
relatedScaleValue = relatedScale;
update();
}
}
void Widget::resetRelatedScale()
{
setRelatedScale(1.0);
}
void Widget::zoomIn()
{
syncScaledValue = qBound(MIN_FACTOR , pow(1.2, 1) * syncScaledValue, MAX_FACTOR);
update();
}
void Widget::zoomOut()
{
syncScaledValue = qBound(MIN_FACTOR , pow(1.2, -1) * syncScaledValue, MAX_FACTOR);
update();
}
void Widget::wheelEvent(QWheelEvent *e)
{
qreal distance = (e->angleDelta() / 15 / 8).y();
qreal val;
if (distance != 0)
{
val = pow(1.2, distance);
if (val < 2.0)
{
syncScaledValue *= val;
syncScaledValue = qBound(MIN_FACTOR , syncScaledValue , MAX_FACTOR);
// emit notifyScaledChanged(syncScaledValue, relatedScaleValue);
update();
}
}
}