-
- S pringboot 整合springmvc
学习springmvc和springboot的自动配置我们必须对springmvc的组件足够了解,起码知道怎么用。Springmvc的组件基本都被springboot来做了自动的配置。
-
-
- S pringmvc 的自动解管理
-
中央转发器(DispatcherServlet)
控制器
视图解析器
静态资源访问
消息转换器
格式化
静态资源管理
-
-
-
- 中央 转发器
-
-
Xml无需配置
|----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| <servlet > <servlet-name >chapter2</servlet-name > <servlet-class >org.springframework.web.servlet.DispatcherServlet</servlet-class > <load-on-startup >1</load-on-startup > </servlet > <servlet-mapping > <servlet-name >chapter2</servlet-name > <url-pattern >/</url-pattern > </servlet-mapping > |
中央转发器被springboot自动接管,不再需要我们在web.xml中配置,我们现在的项目也不是web项目,也不存在web.xml,
org.springframework.boot.autoconfigure.web.servlet.DispatcherServletAutoConfiguration, \
-
-
-
- 控制器
-
-
控制器Controller在springboot的注解扫描范围内自动管理。
-
-
-
- 视图 解析器 自动 管理
-
-
Inclusion of ContentNegotiatingViewResolver and BeanNameViewResolver beans.
ContentNegotiatingViewResolver:组合所有的视图解析器的;
曾经的配置文件无需再配
|---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| <bean id ="de" class ="org.springframework.web.servlet.view.InternalResourceViewResolver" > <property name ="prefix" value ="/WEB-INF/jsp/" ></property > <property name ="suffix" value ="*.jsp" ></property > </bean > |
源码:
|---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| public ContentNegotiatingViewResolver viewResolver(BeanFactory beanFactory) { ContentNegotiatingViewResolver resolver = new ContentNegotiatingViewResolver(); resolver.setContentNegotiationManager((ContentNegotiationManager)beanFactory.getBean(ContentNegotiationManager.class )); resolver.setOrder(-2147483648); return resolver; } |
当我们做文件上传的时候我们也会发现multipartResolver 是自动被配置好的
页面
|------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| <form action= "/upload" method= "post" enctype= "multipart/form-data" > <input name= "pic" type= "file" > <input type= "submit" > </form > |
Controller
|----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| @ResponseBody @RequestMapping("/upload" ) public String upload(@RequestParam("pic" )MultipartFile file, HttpServletRequest request){ String contentType = file.getContentType(); String fileName = file.getOriginalFilename(); /*System.out.println("fileName-->" + fileName); System.out.println("getContentType-->" + contentType);*/ //String filePath = request.getSession().getServletContext().getRealPath("imgupload/"); String filePath = "D:/imgup / " ; try { this .uploadFile (file.getBytes(), filePath, fileName); } catch (Exception e) { // TODO: handle exception } return "success" ; } public static void uploadFile(byte [] file, String filePath, String fileName) throws Exception { File targetFile = new File(filePath); if (!targetFile.exists()){ targetFile.mkdirs(); } FileOutputStream out = new FileOutputStream(filePath+fileName); out.write(file); out.flush(); out.close(); } |
文件上传大小可以通过配置来修改
打开application.properties, 默认限制是10MB,我们可以任意修改
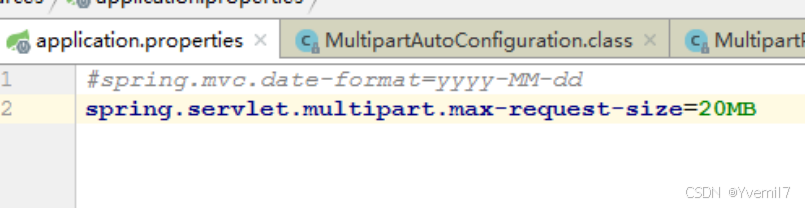
-
-
-
- 静态 资源访问
-
-
参见4.2
-
-
-
- 消息 转换和 格式化
-
-
Springboot自动配置了消息转换器
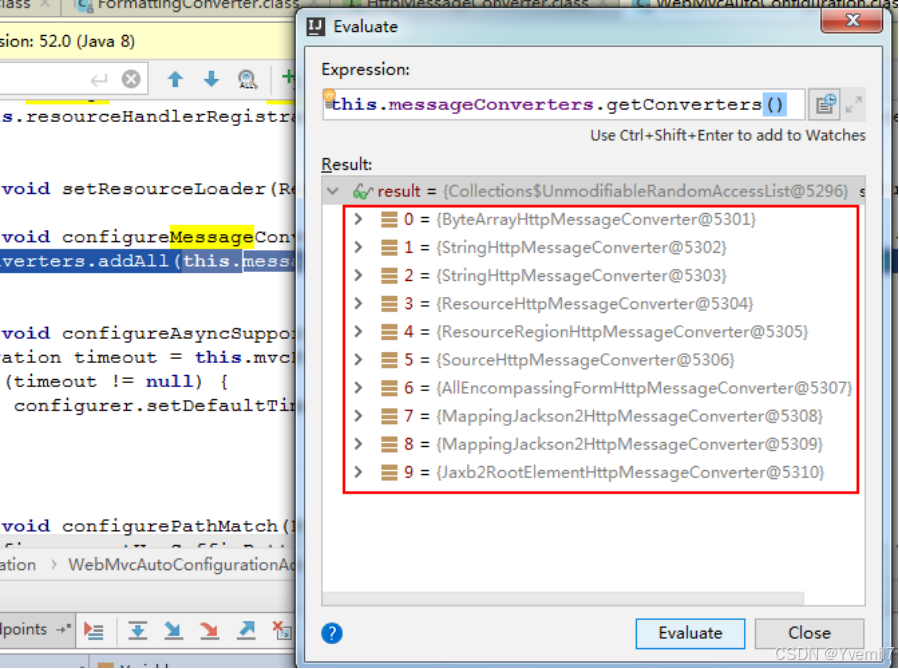
格式化转换器的自动注册
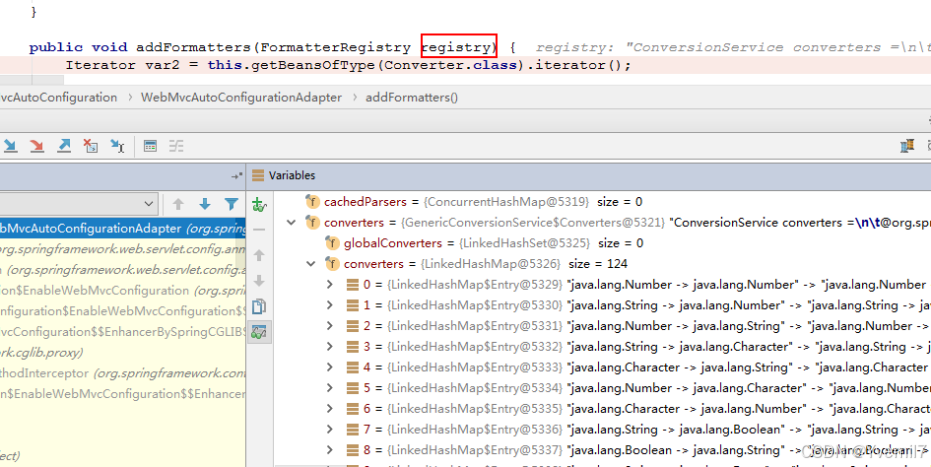
时间类型我们可以在这里修改
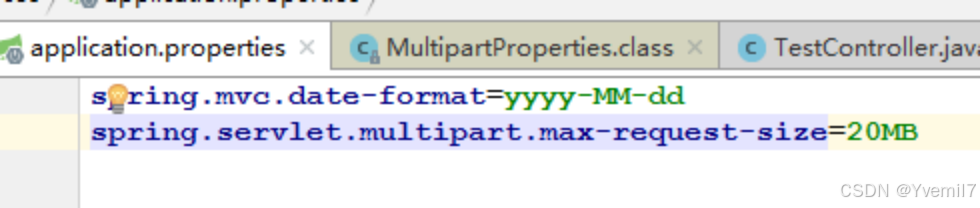
在配置文件中指定好时间的模式我们就可以输入了
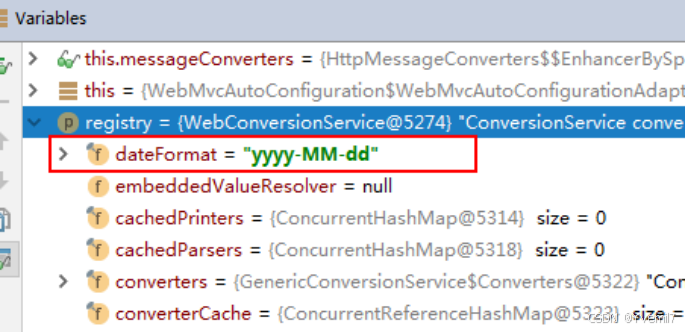
-
-
-
- 欢迎页面 的自动配置
-
-
Springboot自动指定resources下的index.html
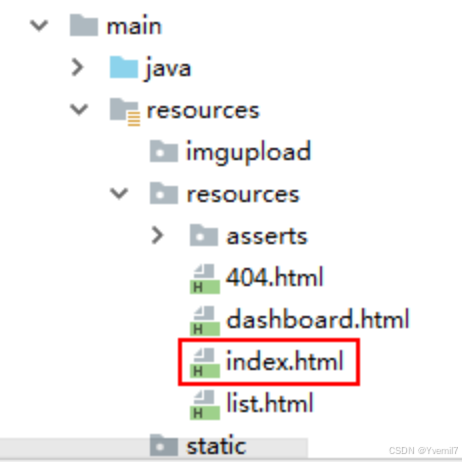
-
-
- Springboot扩展springmvc
-
在实际开发中springboot并非完全自动化,很多跟业务相关我们需要自己扩展,springboot给我提供了接口。
我们可以来通过实现WebMvcConfigurer接口来扩展
|--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| public interface WebMvcConfigurer { default void configurePathMatch(PathMatchConfigurer configurer) { } default void configureContentNegotiation(ContentNegotiationConfigurer configurer) { } default void configureAsyncSupport(AsyncSupportConfigurer configurer) { } default void configureDefaultServletHandling(DefaultServletHandlerConfigurer configurer) { } default void addFormatters(FormatterRegistry registry) { } default void addInterceptors(InterceptorRegistry registry) { } default void addResourceHandlers(ResourceHandlerRegistry registry) { } default void addCorsMappings(CorsRegistry registry) { } default void addViewControllers(ViewControllerRegistry registry) { } default void configureViewResolvers(ViewResolverRegistry registry) { } default void addArgumentResolvers(List<HandlerMethodArgumentResolver> resolvers) { } default void addReturnValueHandlers(List<HandlerMethodReturnValueHandler> handlers) { } default void configureMessageConverters(List<HttpMessageConverter<?>> converters) { } default void extendMessageConverters(List<HttpMessageConverter<?>> converters) { } default void configureHandlerExceptionResolvers(List<HandlerExceptionResolver> resolvers) { } default void extendHandlerExceptionResolvers(List<HandlerExceptionResolver> resolvers) { } @Nullable default Validator getValidator() { return null ; } @Nullable default MessageCodesResolver getMessageCodesResolver() { return null ; } } |
-
-
-
- 在 容器中 注册视图 控制器 ( 请求转发 )
-
-
创建一个MyMVCCofnig实现WebMvcConfigurer接口,实现一下addViewControllers方法,我们完成通过/tx访问,转发到success.html的工作
|--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| @Configuration public class MyMVCCofnig implements WebMvcConfigurer{ @Override public void addViewControllers(ViewControllerRegistry registry) { registry.addViewController("/tx" ).setViewName("success" ); } |
-
-
-
- 注册 格式化器
-
-
用来可以对请求过来的日期格式化的字符串来做定制化。当然通过application.properties配置也可以办到。
|-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| @Override public void addFormatters(FormatterRegistry registry) { registry.addFormatter(new Formatter<Date>() { @Override public String print(Date date, Locale locale) { return null ; } @Override public Date parse(String s, Locale locale) throws ParseException { return new SimpleDateFormat("yyyy-MM-dd" ).parse(s); } }); } |
-
-
-
- 消息 转换器扩展 fastjson
-
-
在pom.xml中引入fastjson
|---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| <dependency > <groupId >com.alibaba</groupId > <artifactId >fastjson</artifactId > <version >1.2.47</version > </dependency > |
配置消息转换器,添加fastjson
|-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| @Override public void configureMessageConverters(List<HttpMessageConverter<?>> converters) { FastJsonHttpMessageConverter fc = new FastJsonHttpMessageConverter(); FastJsonConfig fastJsonConfig = new FastJsonConfig(); fastJsonConfig.setSerializerFeatures(SerializerFeature.PrettyFormat ); fc.setFastJsonConfig(fastJsonConfig); converters.add(fc); } |
在实体类上可以继续控制
|-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| public class User { private String username ; private String password ; private int age ; private int score ; private int gender ; @JSONField(format = "yyyy-MM-dd" ) private Date date ; |
-
-
-
- 拦截器注册
-
-
- 创建拦截器
|----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| public class MyInterceptor implements HandlerInterceptor { @Override public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception { System.out .println(" 前置拦截 " ); return true ; } @Override public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView) throws Exception { System.out .println(" 后置拦截 " ); } @Override public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) throws Exception { System.out .println(" 最终拦截 " ); } } |
拦截器注册
|-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| @Override public void addInterceptors(InterceptorRegistry registry) { registry.addInterceptor(new MyInterceptor()) .addPathPatterns("/**" ) .excludePathPatterns("/hello2" ); } |
-
- 配置嵌入式 服务器
- 如何定制和修改 Servlet 容器的相关配置;
- 配置嵌入式 服务器
修改和server有关的配置(ServerProperties);
|---------------------------------------------------------------------------|
| server.port=8081 server.context‐path=/tx server.tomcat.uri‐encoding=UTF‐8 |
-
-
- 注册 Servlet 三大组件【 Servlet 、 Filter 、 Listener 】
-
由于SpringBoot默认是以jar包的方式启动嵌入式的Servlet容器来启动SpringBoot的web应用,没有web.xml文件。
1.servlet
|---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| //注册三大组件 @Bean public ServletRegistrationBean myServlet(){ ServletRegistrationBean registrationBean = new ServletRegistrationBean(new MyServlet(),"/myServlet"); return registrationBean; } |
- FilterRegistrationBean
|-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| @Bean public FilterRegistrationBean myFilter(){ FilterRegistrationBean registrationBean = new FilterRegistrationBean(); registrationBean.setFilter(new MyFilter()); registrationBean.setUrlPatterns(Arrays.asList("/hello","/myServlet")); return registrationBean; } |
- ServletListenerRegistrationBean
|-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| @Bean public ServletListenerRegistrationBean myListener(){ ServletListenerRegistrationBean<MyListener> registrationBean = new ServletListenerRegistrationBean<>(new MyListener()); return registrationBean; } |
SpringBoot帮我们自动SpringMVC的时候,自动的注册SpringMVC的前端控制器;DispatcherServlet;
DispatcherServletAutoConfiguration中:
|-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| @Bean(name = DEFAULT_DISPATCHER_SERVLET_REGISTRATION_BEAN_NAME) @ConditionalOnBean(value = DispatcherServlet.class, name = DEFAULT_DISPATCHER_SERVLET_BEAN_NAME) public ServletRegistrationBean dispatcherServletRegistration( DispatcherServlet dispatcherServlet) { ServletRegistrationBean registration = new ServletRegistrationBean( dispatcherServlet, this.serverProperties.getServletMapping()); //默认拦截: / 所有请求;包静态资源,但是不拦截jsp请求; /*会拦截jsp //可以通过server.servletPath来修改SpringMVC前端控制器默认拦截的请求路径 registration.setName(DEFAULT_DISPATCHER_SERVLET_BEAN_NAME); registration.setLoadOnStartup( this.webMvcProperties.getServlet().getLoadOnStartup()); if (this.multipartConfig != null) { registration.setMultipartConfig(this.multipartConfig); } return registration; } |