页面跳转基础
引言
在微信小程序开发中,页面跳转和参数传递是常见的需求。本文将通过一个实际案例------"我的咖啡店"小程序,详细介绍如何在微信小程序中实现页面跳转和参数传递。
页面跳转基础
在微信小程序中,页面跳转主要通过wx.navigateTo
和wx.redirectTo
两个API实现。wx.navigateTo
用于跳转到新页面,并且可以返回;而wx.redirectTo
则是关闭当前页面,跳转到应用内的某个页面。
1. 跳转到普通页面
在mine.js
中,我们定义了goIndex
函数,用于跳转到index
页面:
javascript
// pages/mine/mine.js
Page({
goIndex() {
wx.navigateTo({
url: '../index/index',
});
},
// 其他函数...
});
2. 跳转到tabBar页面
对于tabBar页面的跳转,我们使用wx.switchTab
:
javascript
// pages/mine/mine.js
Page({
goHome() {
wx.switchTab({
url: '../home/home',
});
},
// 其他函数...
});
带参数跳转
在某些情况下,我们需要在跳转页面时传递参数。这可以通过在URL后面添加查询字符串来实现。
3. 跳转页面并带参数
在mine.js
中,我们定义了goAA
函数,用于跳转到AA
页面并传递参数:
javascript
// pages/mine/mine.js
Page({
goAA(event) {
let a = event.currentTarget.dataset.a;
console.log("event", a);
wx.navigateTo({
url: '../AA/AA?aa=' + a,
});
},
// 其他函数...
});
获取传递的参数
在目标页面,我们可以通过onLoad
函数的options
参数来获取传递的参数。
4. 在跳转的页面中获取参数
在AA.js
中,我们定义了onLoad
函数,用于获取传递的参数:
javascript
// pages/AA/AA.js
Page({
onLoad(options) {
console.log("options", options.aa);
this.getxxx(options.aa);
},
getxxx(aa) {
console.log("aa===", aa);
}
});
结语
通过上述步骤,我们成功在"我的咖啡店"小程序中实现了页面跳转和参数传递功能。用户可以在不同页面间跳转,并且可以传递必要的参数。如果你在实现过程中遇到问题,可能是由于路径错误或者参数传递不正确。请检查路径的合法性,并确保参数正确传递。希望这篇文章能帮助你在开发自己的微信小程序时,更好地实现页面跳转和参数传递功能。
完整代码
app.json
javascript
{
"pages": [
"pages/home/home",
"pages/index/index",
"pages/logs/logs",
"pages/order/order",
"pages/ordering/ordering",
"pages/mine/mine",
"pages/search/search",
"pages/AA/AA"
],
"window": {
"navigationBarTextStyle": "black",
"navigationBarTitleText": "我的咖啡店",
"navigationBarBackgroundColor": "#ffffff"
},
"style": "v2",
"componentFramework": "glass-easel",
"sitemapLocation": "sitemap.json",
"lazyCodeLoading": "requiredComponents",
"tabBar": {
"color": "#bfbfbf",
"selectedColor": "#8B7355",
"backgroundColor": "#ffffff",
"list": [
{
"pagePath": "pages/home/home",
"text": "首页",
"iconPath": "./image/home.png",
"selectedIconPath": "./image/home-active.png"
},
{
"pagePath": "pages/order/order",
"text": "点餐",
"iconPath": "./image/order.png",
"selectedIconPath": "./image/order-active.png"
},
{
"pagePath": "pages/ordering/ordering",
"text": "订单",
"iconPath": "./image/ordering.png",
"selectedIconPath": "./image/ordering-active.png"
},
{
"pagePath": "pages/mine/mine",
"text": "我的",
"iconPath": "./image/mine.png",
"selectedIconPath": "./image/mine-active.png"
}
]
},
"usingComponents": {
"van-search": "@vant/weapp/search/index",
"van-cell": "@vant/weapp/cell/index",
"van-cell-group": "@vant/weapp/cell-group/index"
}
}
mine.wxml
XML
<!-- pages/mine/mine.wxml -->
<view class="mine">
<van-cell title="去Index" bind:click="goIndex" is-link />
<van-cell title="去Home" bind:click="goHome" is-link />
<van-cell title="去AA" bind:click="goAA" is-link />
</view>
mine.js
javascript
// pages/mine/mine.js
Page({
// 1. 跳转到普通页面
goIndex() {
wx.navigateTo({
url: '../index/index',
});
},
// 2. 跳转到tabBar页面
goHome() {
wx.switchTab({
url: '../home/home',
});
},
// 3. 跳转页面 带参数
goAA(event) {
// 获取自定义属性a的值
let a = event.currentTarget.dataset.a;
console.log("event", a);
wx.navigateTo({
// 带参数的url怎么写?
// 路径?参数名=值
url: '../AA/AA?aa=' + a,
});
}
});
AA.js
javascript
// pages/AA/AA.js
Page({
// 生命周期函数--监听页面加载
onLoad(options) {
console.log("options", options.aa);
// 调用
this.getxxx(options.aa);
},
// 获取参数
getxxx(aa) {
console.log("aa==>", aa);
}
});
展示
我的页面
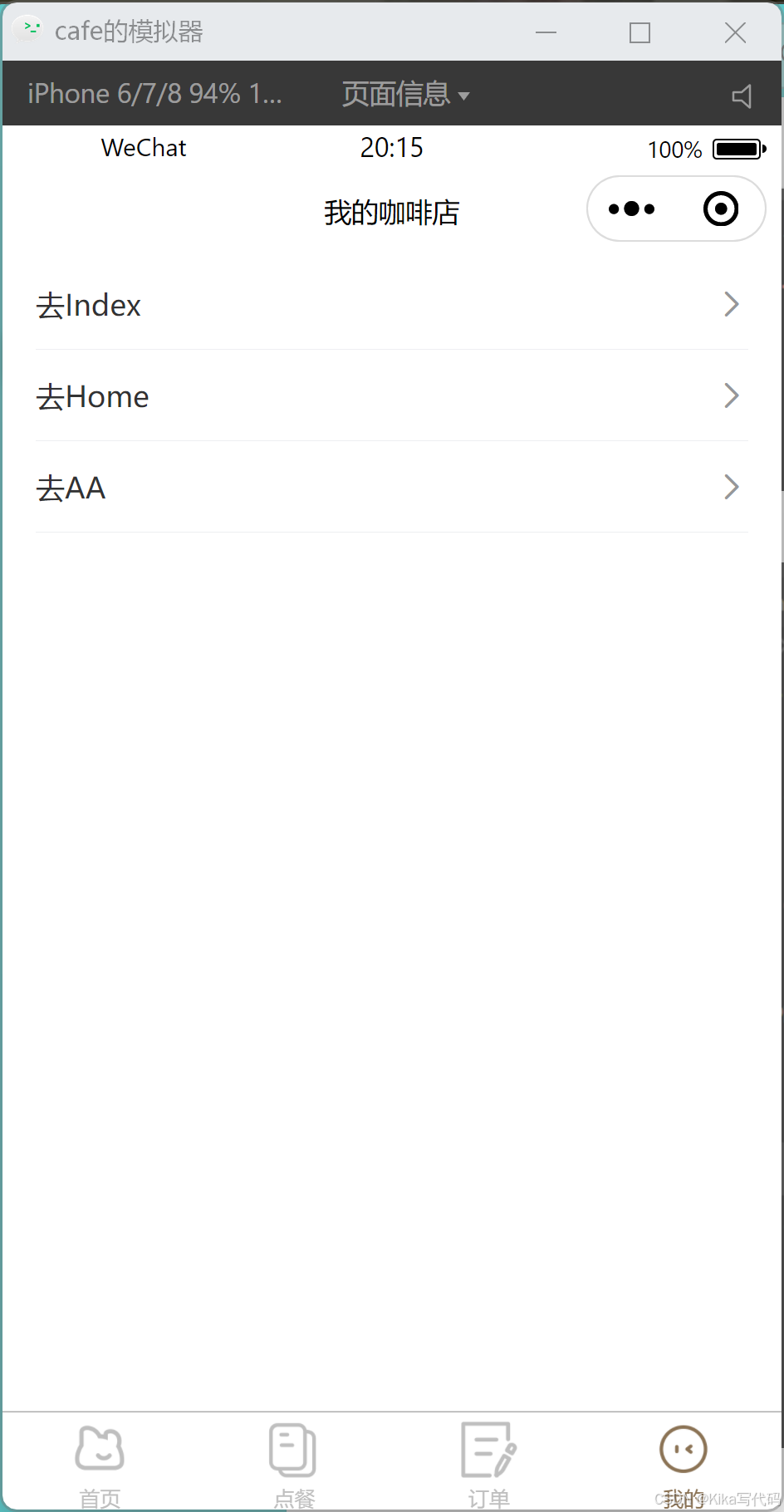
去Index页面-普通页面
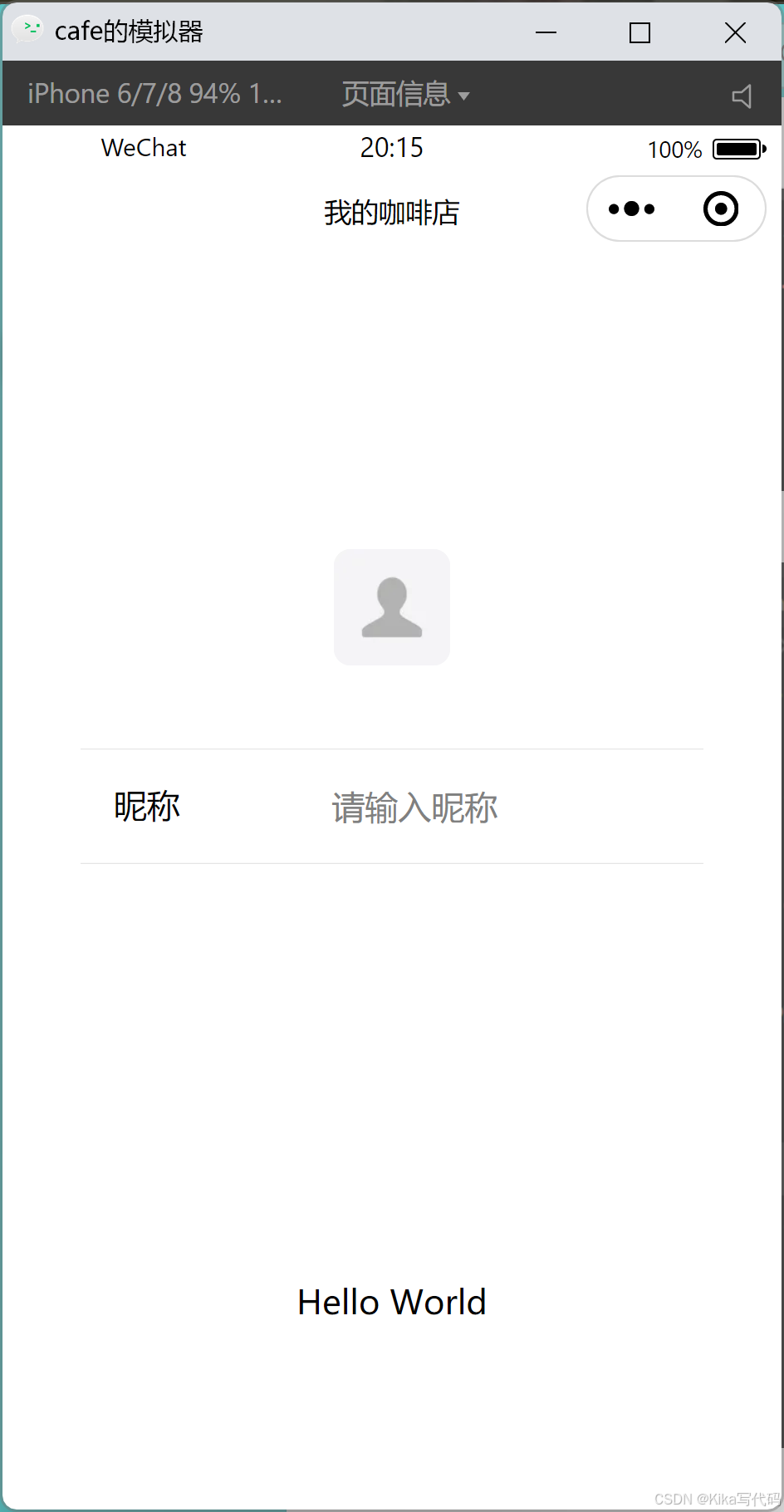
去Home页面-tabBar页面
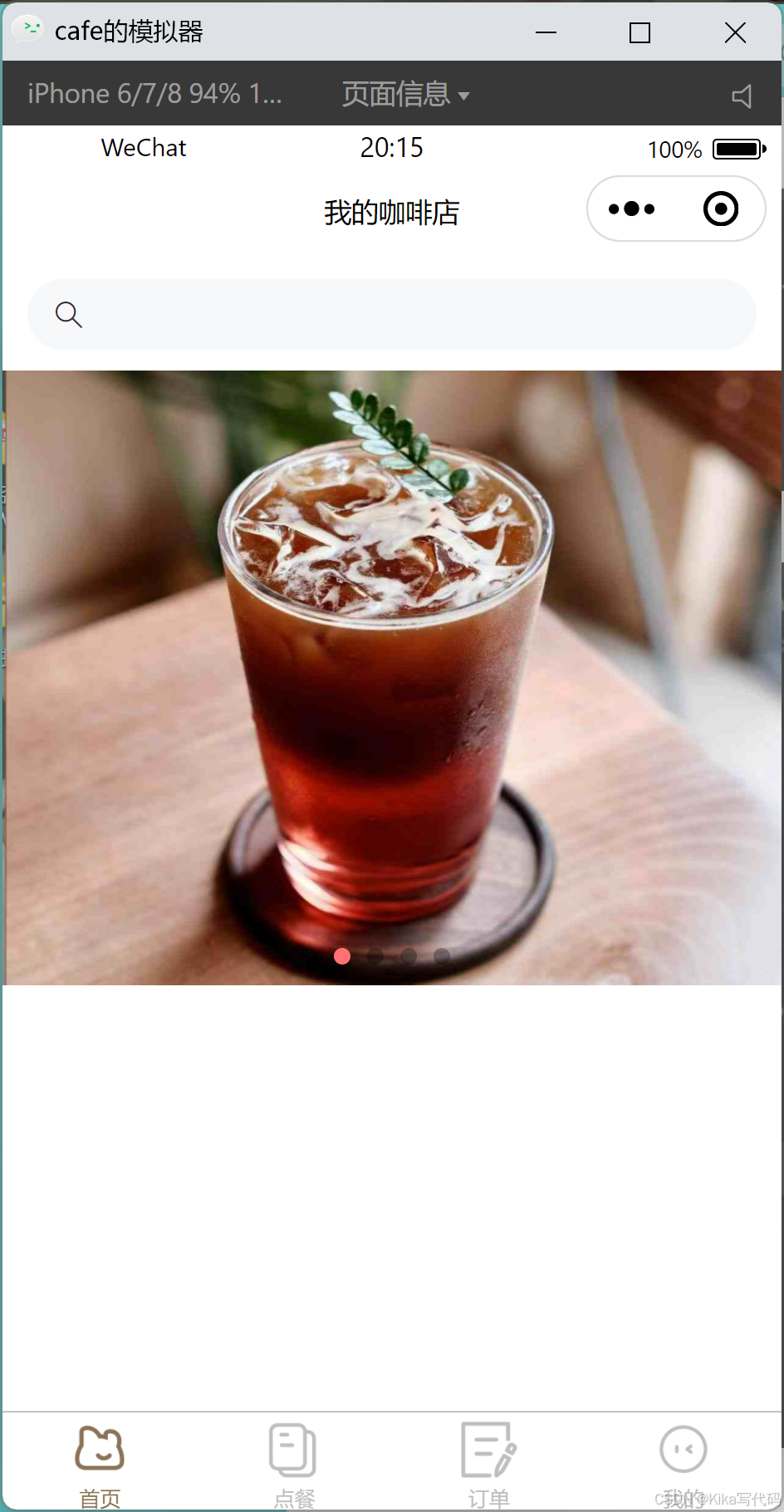
去AA页面-在跳转的页面中获取参数
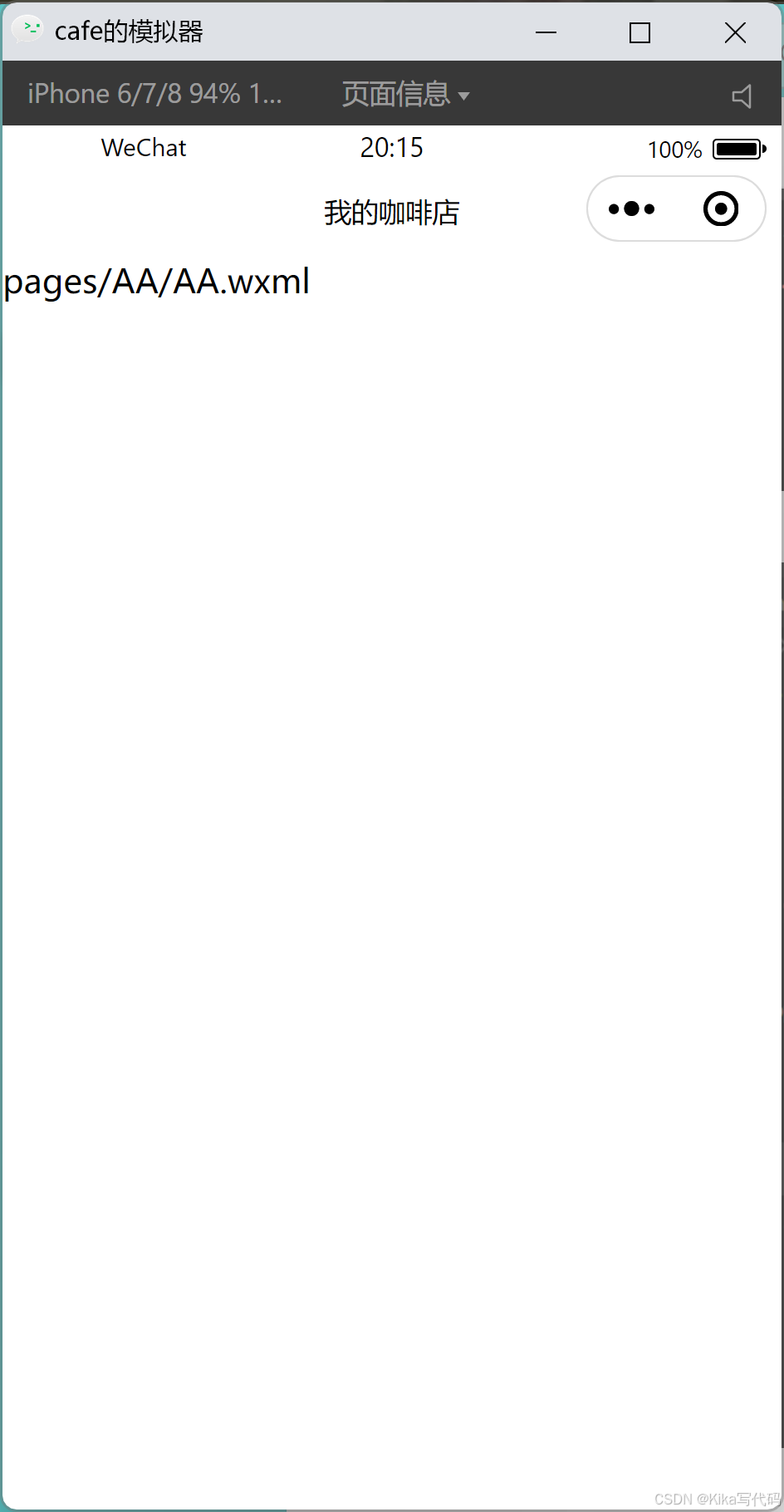
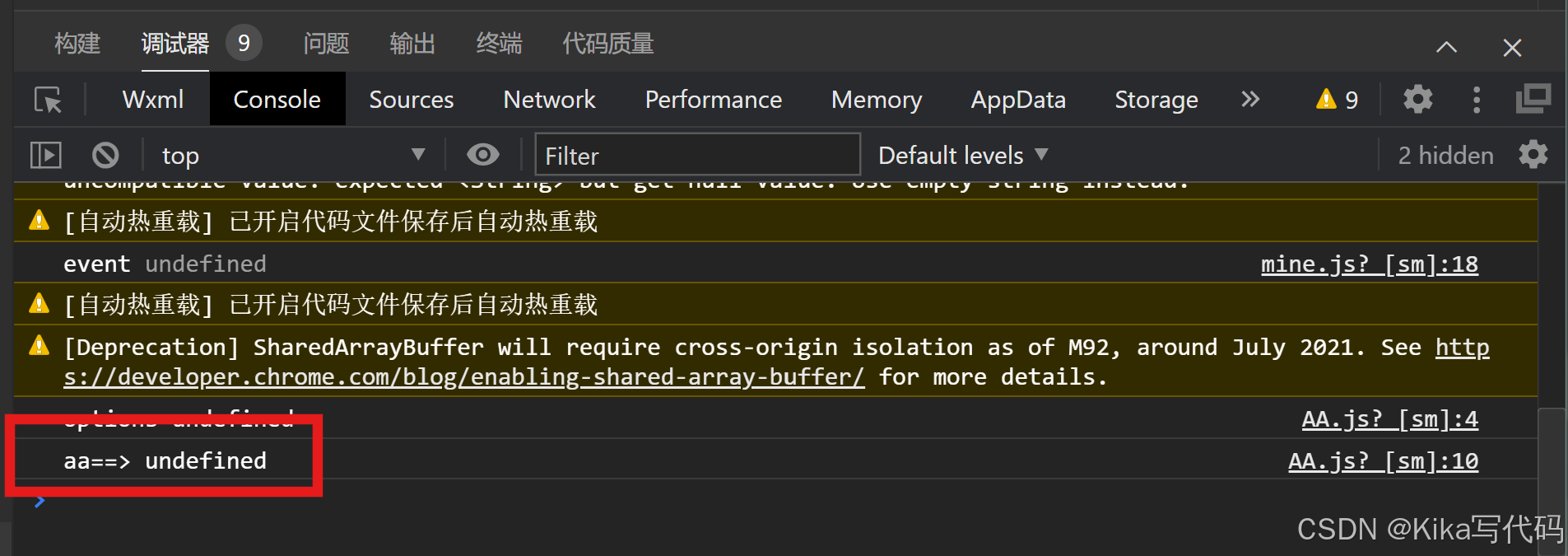