背景
设备是EPICS流式细胞仪,这个设备的控制系统是在DOS系统上的。数据存储在硬盘上,不带串口通讯功能。我们遇到了这个设备后,就开发了一个DOS下的执行程序通过串口,将最新的数据自动上传到服务器上。
编译工具 Turbo C++
数据内容
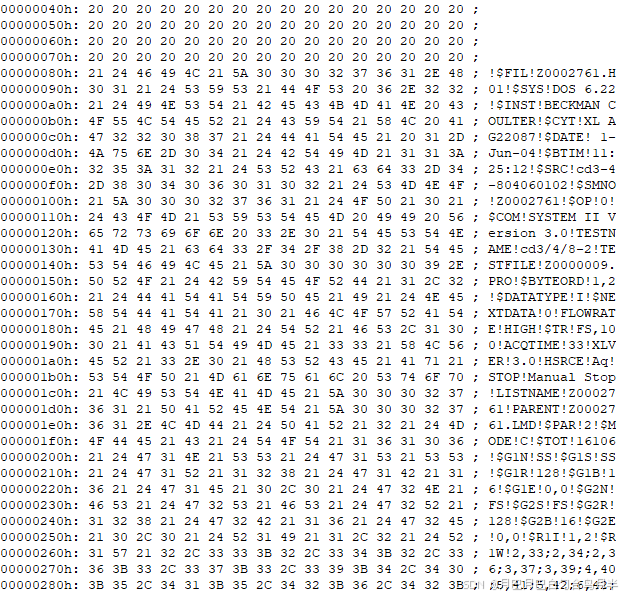
代码
cpp
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <dir.h>
#include <dos.h>
#include <ctype.h>
#include <string.h>
#include <GSerial.h>
#include <fcntl.h>
#include <io.h>
#define sendbuf_size 1024
char searchp[255];
int jkport = COM1;
long jkspeed = 19200L;
int jkparity = LCR_NO_PARITY;
int jkbits = 8;
int jkstopbits = 1;
serial jkcom (jkport, jkspeed, jkparity, jkbits, jkstopbits); ;
char sendbuf[sendbuf_size+1];
char sendbuf_2[sendbuf_size*3+3];
int send(char *str,int size )
{
int i;
for (i=0;i<size;i++)
{
jkcom<<(str[i]);
}
delay(10);
return(0);
}
int send2(char *str,int size )
{
int i;
int i2;
int k;
int recount;
i2=0;
i=0;
do
{
if( !str[i])
{
recount=0;
for (k=i;k<size;k++)
{
if (str[k] == str[i])
{
recount=recount+1;
}
else
break;
}
if (recount>255)
{
recount=255;
}
sendbuf_2[i2]=char(0);
i2=i2+1;
sendbuf_2[i2]=char(recount);
i2=i2+1;
sendbuf_2[i2]=str[i];
i2=i2+1;
i=i+recount;
}
else
{
recount=0;
for (k=i;k<size;k++)
{
if (str[k] == str[i])
{
recount=recount+1;
}
else
break;
}
if (recount>255)
{
recount=255;
}
if( recount>2)
{
sendbuf_2[i2]=char(0);
i2=i2+1;
sendbuf_2[i2]=char(recount);
i2=i2+1;
sendbuf_2[i2]=str[i];
i2=i2+1;
i=i+recount;
}
else
{
for (k = 1 ;k<=recount;k++)
{
sendbuf_2[i2]=str[i];
i2=i2+1;
};
i=i+recount;
}
}
}
while(i<size);
for (i=0;i<i2;i++)
{
jkcom<<(sendbuf_2[i]);
}
return(0);
}
int rece()
{
char receivebuf;
int i;
int k;
k=-1;
i=0;
do
{
delay(10);
jkcom>>receivebuf;
i=i+1;
k= receivebuf-char(0);
if ( (k>=4) && (k<=7) )
{
break;
}
}
while (i<300);
return(k);
}
int filesend(char *str)
{
char fn[255];
long fl;
int handle;
stpcpy(fn,searchp);
strcat(fn,str);
handle=open(fn,O_RDONLY);
fl=filelength(handle);
close(handle);
gotoxy(1, 9);
printf(" file name: %s ", fn);
gotoxy(1, 11);
printf(" file size: %8ld ", fl);
FILE *fp;
int nowread;
long allread;
allread=0;
gotoxy(1,13);
printf(" sending: %8ld", allread );
char tmp[20];
jkcom<<(char(02));
jkcom<<("headfromdos;");
jkcom<<(str);
jkcom<<(";");
ltoa(fl,tmp,10);
jkcom<<(tmp);
jkcom<<(char(03));
gotoxy(1,15);
printf(" send head: %s;%s", str,tmp );
int receivebuf;
receivebuf=rece();
gotoxy(1,17);
printf("head receive: #%-4d ",receivebuf);
nowread=0;
allread=0;
if( receivebuf ==4)
{
fp=fopen(fn,"rb");
nowread=0;
gotoxy(1, 9);
printf(" file name: %s ", fn);
while(!feof(fp))
{
sendbuf[nowread]=fgetc(fp);
nowread=nowread+1;
if( nowread>=sendbuf_size)
{
allread=allread+nowread;
gotoxy(1,13);
printf(" sending: %8ld", allread );
send2(sendbuf,nowread);
nowread=0;
}
}
if (nowread>1)
{
allread=allread+nowread-1;
gotoxy(1,13);
printf(" sending: %8ld", allread );
send2(sendbuf,nowread-1);
}
fclose(fp);
receivebuf=rece();
gotoxy(1,19);
printf("data receive: #%-4d ",receivebuf);
if( receivebuf ==6)
{
return(1);
}
else
return(0);
}
else
return(0);
}
void filesearch(char *str)
{
struct ffblk ffblk;
int done;
int filecount;
int failcount;
struct date d;
failcount=0;
filecount=0;
char fn[255];
char backp[MAXPATH+8];
char tmpdir[255];
char tmp[255];
char doscmd[255];
int mord;
getcwd(backp,MAXPATH);
getdate(&d);
itoa(d.da_year,tmp,10);
stpcpy(tmpdir,tmp);
mord=d.da_mon-char(0);
itoa(mord,tmp,10);
if (mord<10)
strcat(tmpdir,"0");
strcat(tmpdir,tmp);
mord=d.da_day-char(0);
itoa(mord,tmp,10);
if (mord<10)
strcat(tmpdir,"0");
strcat(tmpdir,tmp);
mkdir(tmpdir);
strcat(backp,"\\");
strcat(backp,tmpdir);
strcat(backp,"\\");
gotoxy(1,21);
printf(" back path: %s ",backp);
stpcpy(fn,str);
strcat(fn,"*.*");
gotoxy(1,1);
printf(" search file: %s ",fn );
done = findfirst(fn,&ffblk,0);
while (!done)
{
filecount=filecount+1;
done =findnext(&ffblk);
}
gotoxy(1,3);
printf(" all count: %d ",filecount);
filecount=0;
gotoxy(1,5);
printf(" sended count: %d ",filecount);
gotoxy(1,7);
printf(" failed count: %d ",failcount);
done = findfirst(fn,&ffblk,0);
int acce;
while (!done)
{
acce=filesend(ffblk.ff_name);
if (acce==1 )
{
gotoxy(1,5);
filecount=filecount+1;
printf(" sended count: %d ",filecount);
stpcpy(doscmd,"copy ");
strcat(doscmd,str);
strcat(doscmd,ffblk.ff_name);
strcat(doscmd," ");
strcat(doscmd,backp);
strcat(doscmd,ffblk.ff_name);
gotoxy(1,22);
system(doscmd);
gotoxy(1,22);
printf("%40s","");
stpcpy(doscmd,"del ");
strcat(doscmd,str);
strcat(doscmd,ffblk.ff_name);
gotoxy(1,23);
system(doscmd);
//delay(10);
}
else
{
gotoxy(1,7);
failcount=failcount+1;
printf(" failed count: %d ",failcount);
delay(2500);
};
done =findnext(&ffblk);
}
}
main()
{
clrscr();
stpcpy(searchp,"c:\\data\\");
filesearch(searchp);
delay(1500);
gotoxy(1,15);
printf("\n" );
return(0);
}