前言
C#特性学习、主要是用在数据库连接时如何动态创建对应的表,正常开发应该使用如Entity Framework等ORM框架实现自动创建生成。
代码
1、声明特性
csharp
[AttributeUsage(AttributeTargets.Property)]
public class PrimaryKeyAttribute : Attribute { }
[AttributeUsage(AttributeTargets.Property)]
public class ForeignKeyAttribute : Attribute
{
public string ReferenceTable { get; }
public string ReferenceColumn { get; }
public ForeignKeyAttribute(string referenceTable, string referenceColumn)
{
ReferenceTable = referenceTable;
ReferenceColumn = referenceColumn;
}
}
[AttributeUsage(AttributeTargets.Property)]
public class RequiredAttribute : Attribute { }
[AttributeUsage(AttributeTargets.Property)]
public class UniqueAttribute : Attribute { }
2、定义特性方法
csharp
//特性方法
public static class AttributeMethod
{
//反射+泛型打印特性名
public static void GetAttributies<T>()
{
Type objType = typeof(T);
foreach (var property in objType.GetProperties())
{
Console.WriteLine($"Property: {property.Name}");
if (property.GetCustomAttribute<PrimaryKeyAttribute>() != null)
{
Console.WriteLine(" - Primary Key");
}
if (property.GetCustomAttribute<ForeignKeyAttribute>() != null)
{
var foreignKey = property.GetCustomAttribute<ForeignKeyAttribute>();
Console.WriteLine($" - Foreign Key: References {foreignKey.ReferenceTable}.{foreignKey.ReferenceColumn}");
}
if (property.GetCustomAttribute<RequiredAttribute>() != null)
{
Console.WriteLine(" - Required");
}
if (property.GetCustomAttribute<UniqueAttribute>() != null)
{
Console.WriteLine(" - Unique");
}
}
}
}
3、创建类并指定特性
csharp
public class User
{
[PrimaryKey]
public int Id { get; set; }
[Required]
[Unique]
public string Username { get; set; }
[Required]
public string Password { get; set; }
[ForeignKey("Role", "Id")]
public int RoleId { get; set; }
}
public class Role
{
[PrimaryKey]
public int Id { get; set; }
[Required]
public string Name { get; set; }
}
调用方法获取并打印特性名
csharp
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("输出User特性:");
AttributeMethod.GetAttributies<User>();
Console.WriteLine();
Console.WriteLine("输出Role特性:");
AttributeMethod.GetAttributies<Role>();
Console.ReadKey();
}
}
结果
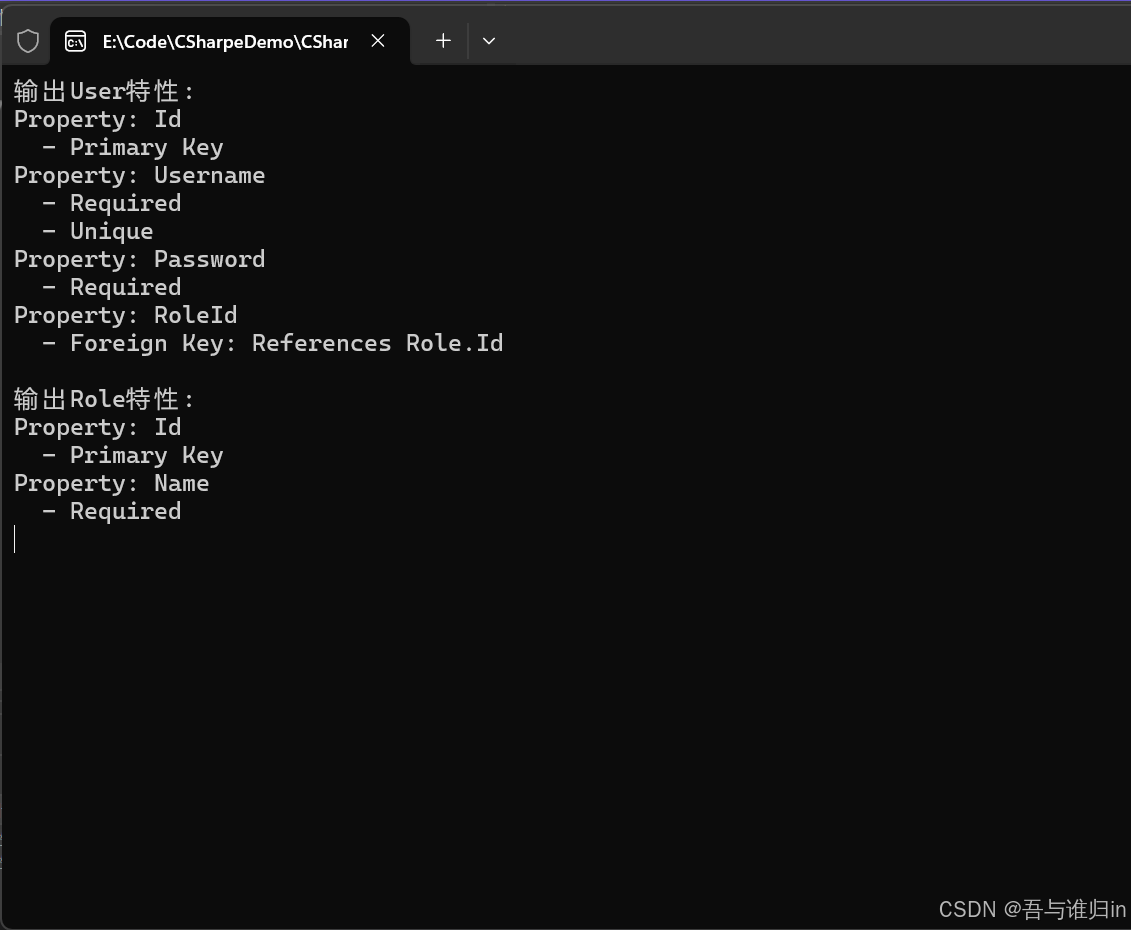