1.反转链表
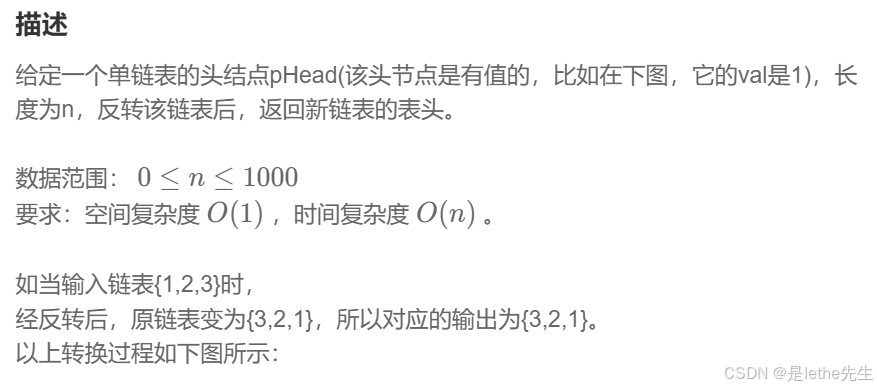
【1】代码思想:
1、设置三个结点,分别为pre、cur、temp。其中cur指向头节点处(cur=head),pre是cur的前面,temp是cur的后面。
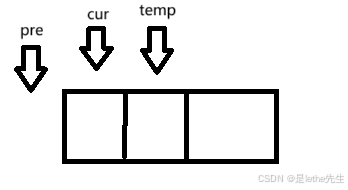
2、先初始化(把pre和temp置空),然后使用一个循环,把pre和temp放到上面说的位置,然后让cur和pre换位置(cur->next=pre;pre=cur;)
3.还在循环内,将pre、cur和temp均往后移动,直到循环结束即可~
【2】代码
//结构体定义
typedef struct node{
int val;
struct node *next;
}ListNode;
//单链表反转
ListNode* ReverseList(struct ListNode* head) {
ListNode *pre=NULL,*cur=head,*temp=NULL;
while(cur!=NULL){
temp=cur->next;//保存当前结点的下一个结点
cur->next=pre;//交换
pre=cur;//交换
cur=temp;//结点后移
}
return pre;
}
2.判断回文字符串:回文是正着反着都相等
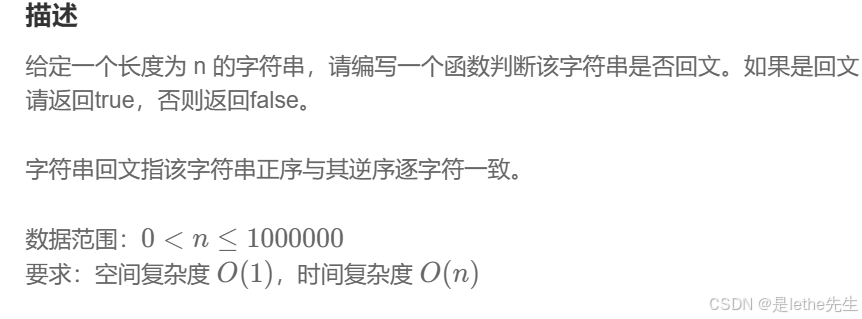
bool judge(char* str ) {
int len=strlen(str);
int i=0;
int j=len-1;
while(i<=j){
if(str[i]!=str[j]){
return false;
}
i++;
j--;
}
return ture;
}
3.反转字符串
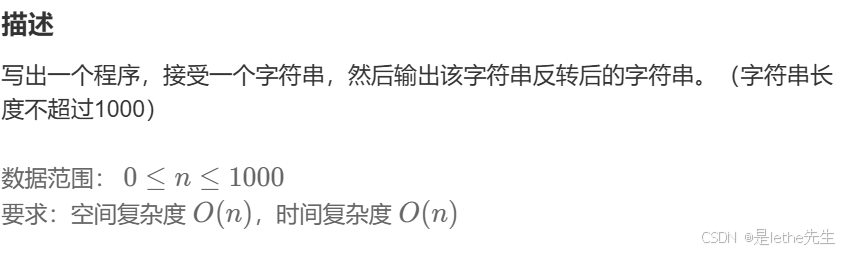
char* solve(char* str ) {
int len=strlen(str);
int i=0;
int j=len-1;
while(i<=j){
char temp=str[i];
str[i]=str[j];
str[j]=temp;
i++;
j--;
}
return str;
}
4.斐波那契数列:前两项为1,第三项开始,该项等于前两项的和
int Fibonacci(int n ) {
if(n==0||n==1){
return 1;
}
int a[41];//n小于等于40
a[1]=a[2]=1;
for(int i=3;i<=n;i++){
a[i]=a[i-1]+a[i-2];
}
return a[n];
}