(1)以下是一个网上的使用 pipe 编程的范例:
c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/types.h>
#include <sys/wait.h>
int main() {
int pipefd[2];
pid_t pid;
char writeMsg[] = "Hello from parent process!";
char readMsg[100];
// 创建管道
if (pipe(pipefd) == -1) {
perror("pipe");
exit(EXIT_FAILURE);
}
// 创建子进程
pid = fork();
if (pid == -1) {
perror("fork");
exit(EXIT_FAILURE);
}
if (pid > 0) { // 父进程
close(pipefd[0]); // 关闭读取端
// 向管道写入消息
write(pipefd[1], writeMsg, strlen(writeMsg) + 1); // +1 是为了包含终止符 '\0'
close(pipefd[1]); // 写入完成后关闭写入端
wait(NULL); // 等待子进程结束
}
else { // 子进程
close(pipefd[1]); // 关闭写入端
// 从管道读取消息
ssize_t numBytes = read(pipefd[0], readMsg, sizeof(readMsg) - 1);
if (numBytes == -1) {
perror("read");
exit(EXIT_FAILURE);
}
readMsg[numBytes] = '\0'; // 添加字符串终止符
// 打印读取的消息
printf("Received message: %s\n", readMsg);
close(pipefd[0]); // 读取完成后关闭读取端
}
return 0;
}
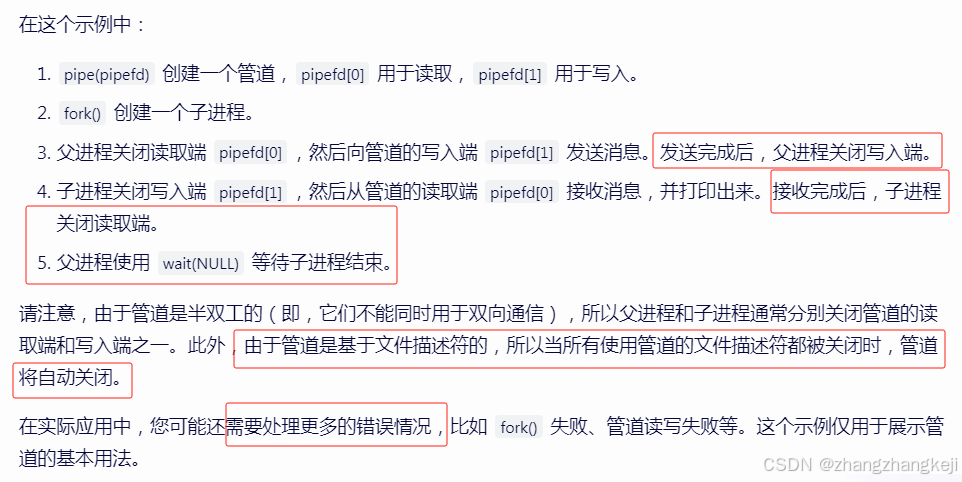
(2)上面引用了 wait() 函数,给出其定义:
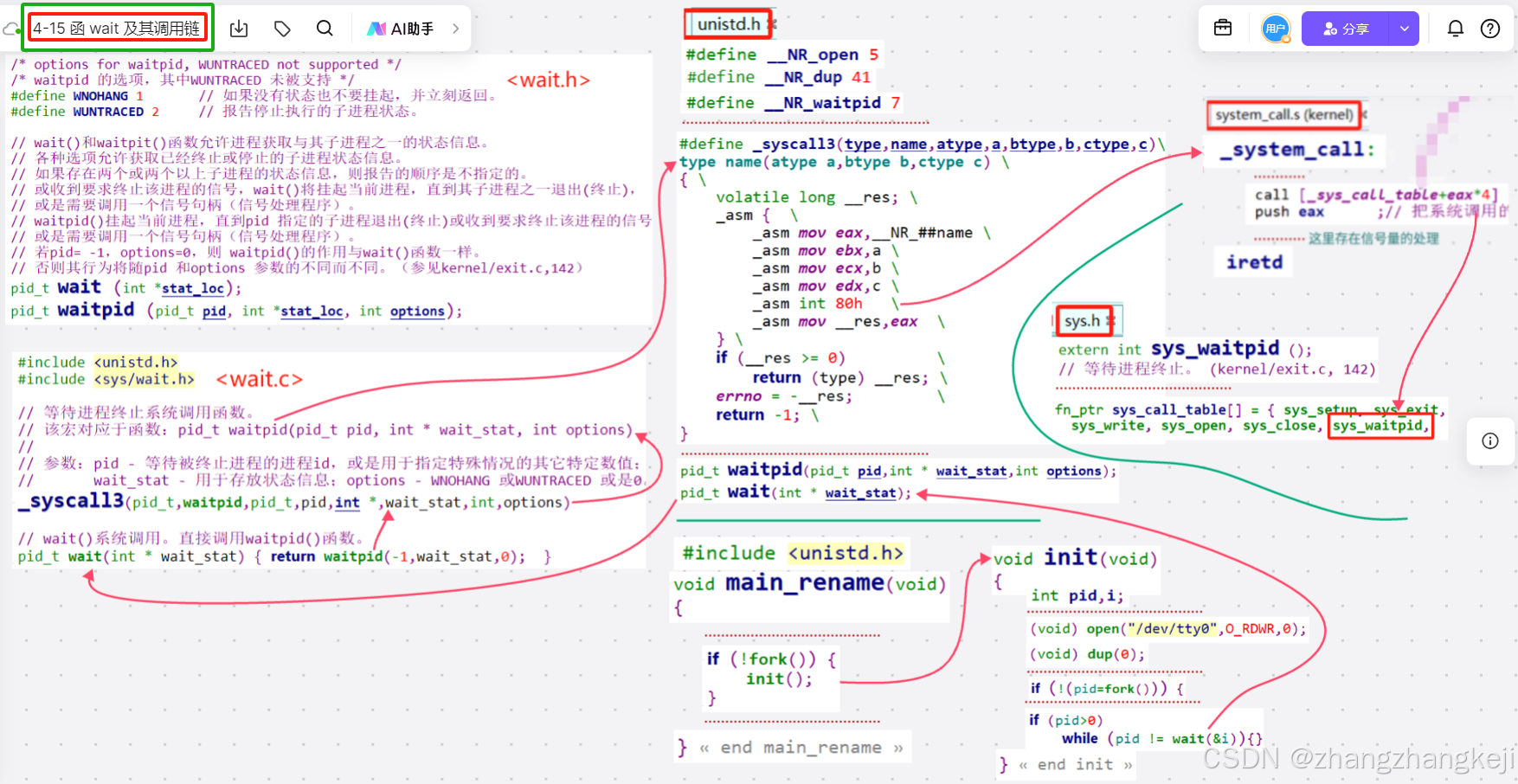
(3)再给出 linux 0.11 里很重要的管道文件的创建源码:
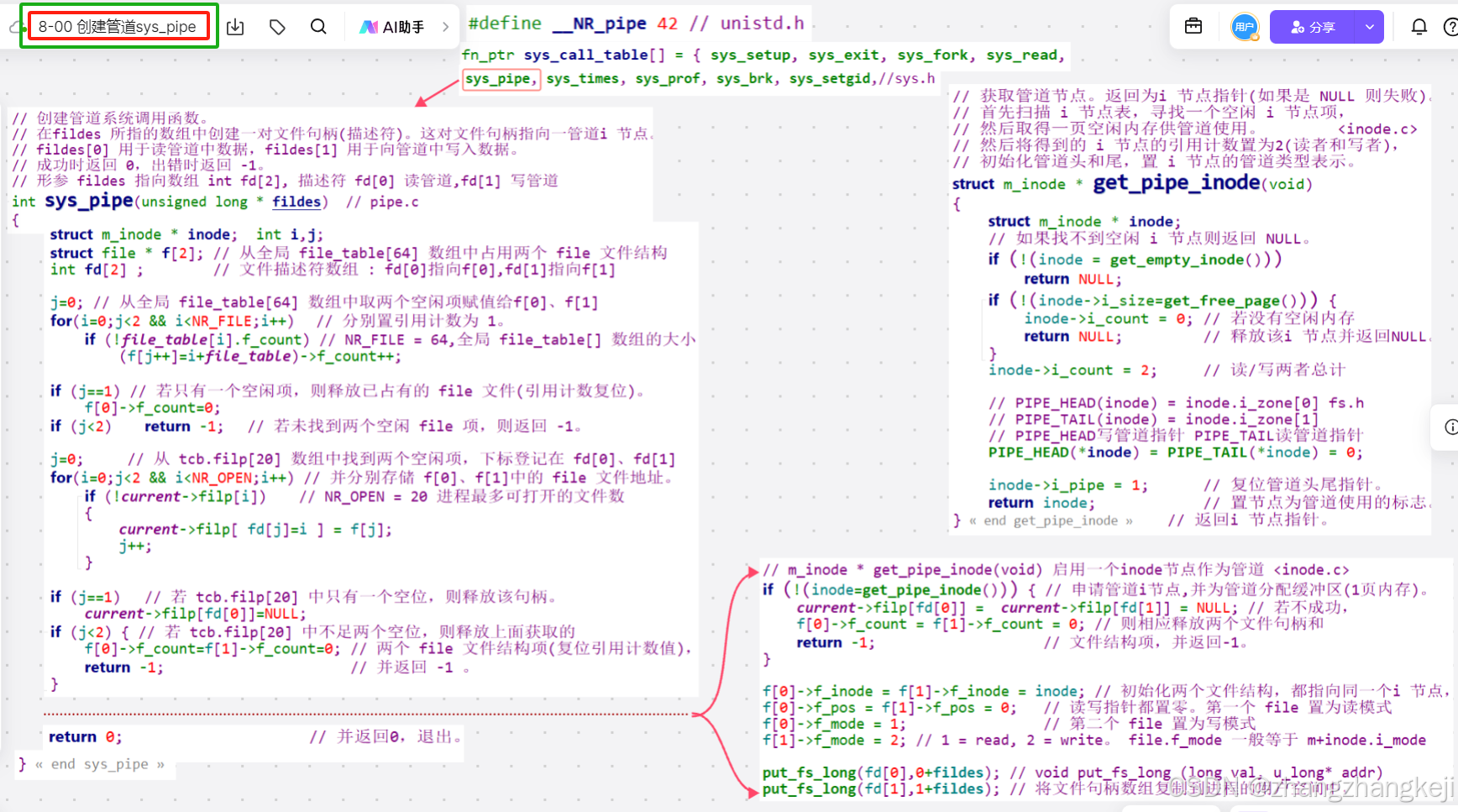
(4)文件都对应 inode 节点,接着给出文件描述符 ,file 文件结构 ,inode 节点结构的定义与关联关系:
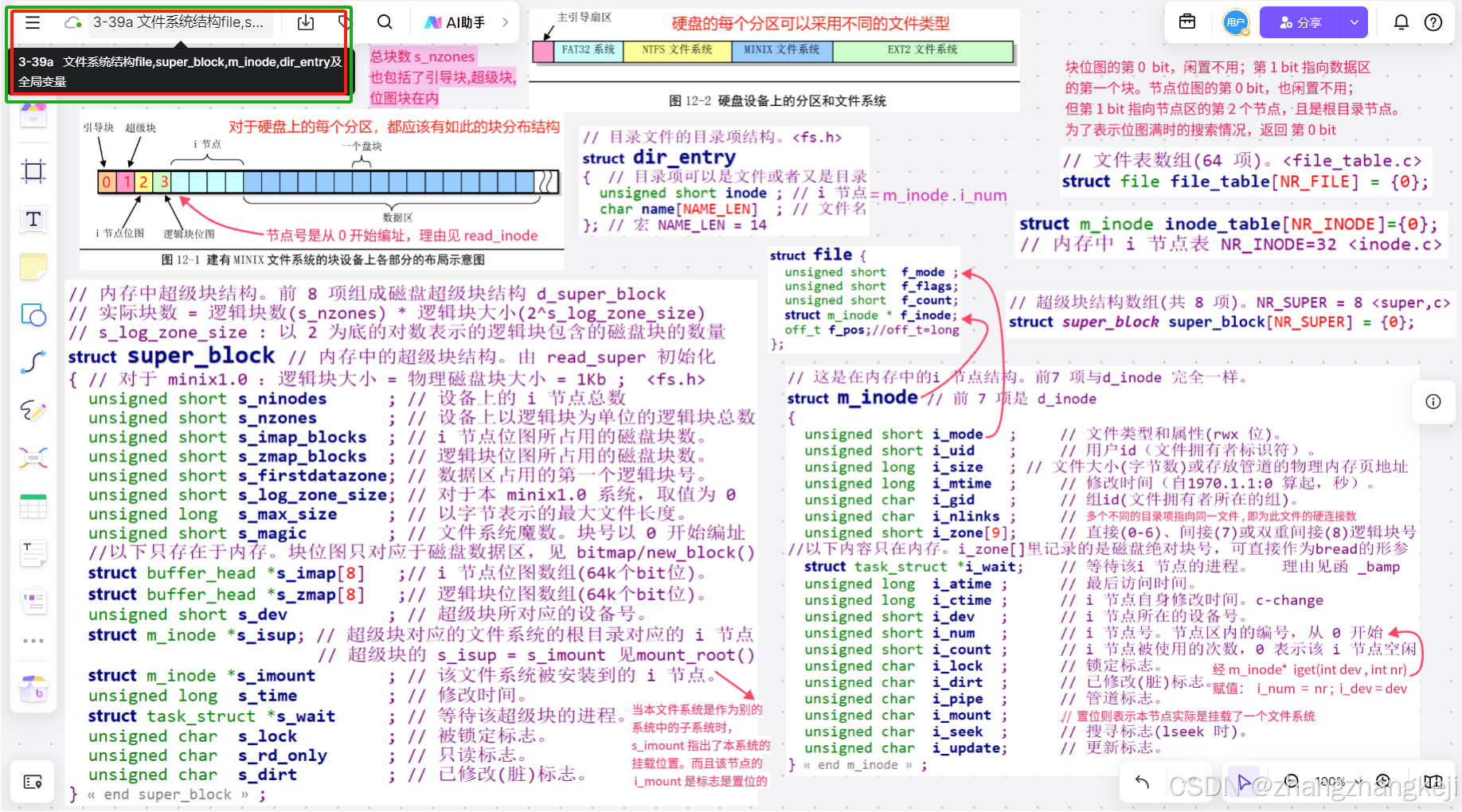
(5)读写管道,主要依赖于 linux 0.11 系统的这俩系统函数:
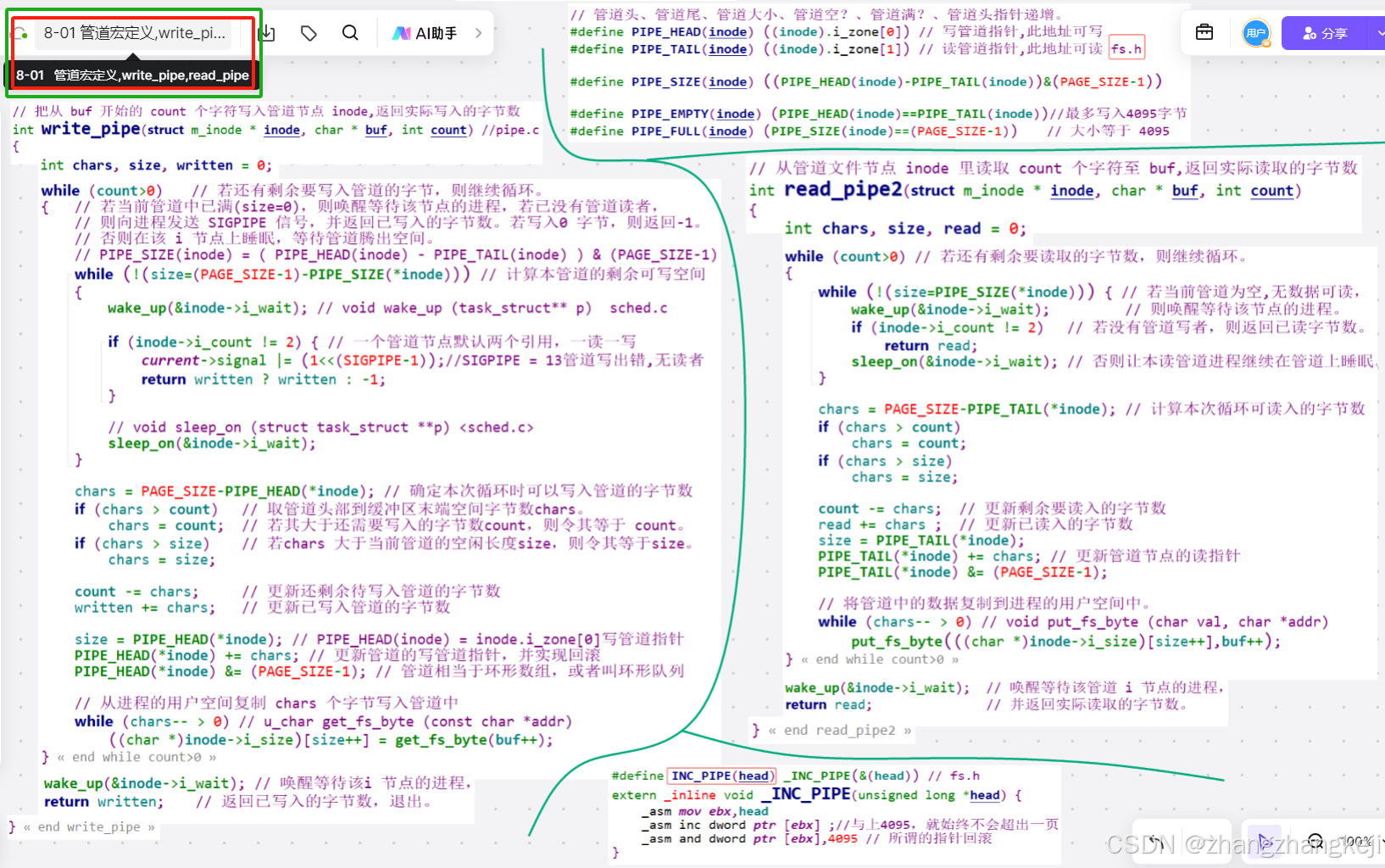
(6)给出我自己的简单版的 实验结果:
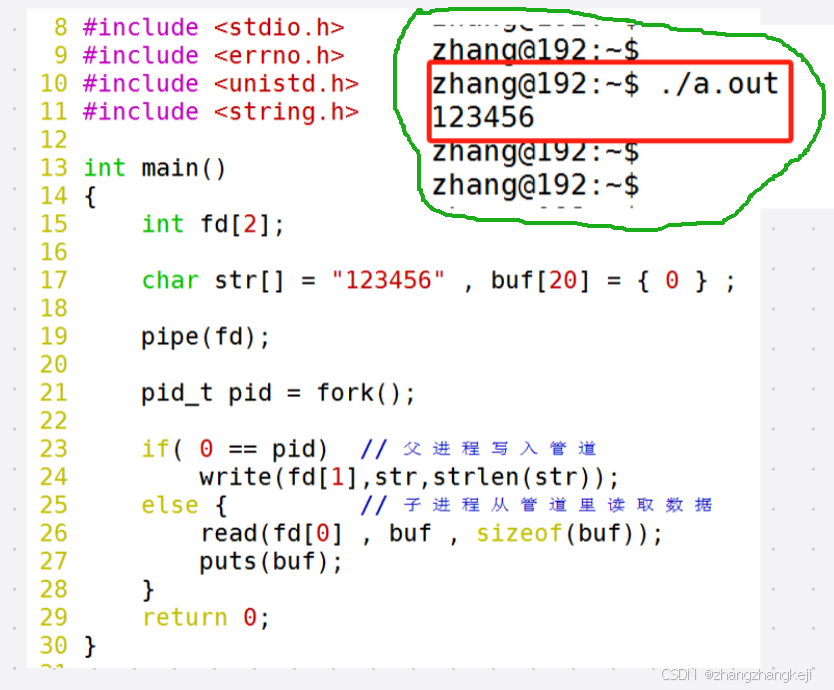
(7)
谢谢