简介
EllipseGeometry用于绘制一个椭圆的形状。它通常与其他图形元素结合使用,比如 Path
或者作为剪切区域来定义其他元素的外形。
- 定义椭圆 :
EllipseGeometry
用来定义一个椭圆或者圆的几何形状。 - 参与绘制 :可以被用作
Path
元素的数据,以实现绘制椭圆的效果。 - 作为剪辑路径:可以指定为某个 UI 元素的剪辑区域,使得该元素只显示在椭圆范围内的内容。
属性
- Center :表示椭圆中心点的位置,是一个
Point
类型的值,默认是(0,0)
。 - RadiusX 和 RadiusY:分别表示椭圆沿 X 轴和 Y 轴方向上的半径长度。这两个值决定了椭圆的大小和形状;如果它们相等,则创建的是一个圆形。
- Transform :允许你对椭圆应用变换,如旋转、缩放和平移等。
- TranslateTransform:用于移动(平移)对象。
- ScaleTransform:用于缩放对象。
- RotateTransform:用于旋转对象。
- SkewTransform:用于倾斜对象。
- MatrixTransform:使用矩阵来定义更复杂的变换。
- **TransformGroup:**组合变换。
示例
绘制一个中心位于 (400, 200),X 半径为 200,Y 半径为 50 的椭圆轮廓。
代码:
cs
<Window x:Class="WPFDemo.Line.Views.EllipseGeometryWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WPFDemo.Line.Views"
mc:Ignorable="d"
Title="EllipseGeometryWindow" Height="450" Width="800">
<Grid>
<Path Stroke="Red" StrokeThickness="2">
<Path.Data>
<EllipseGeometry Center="400,200" RadiusX="200" RadiusY="50" />
</Path.Data>
</Path>
</Grid>
</Window>
效果:
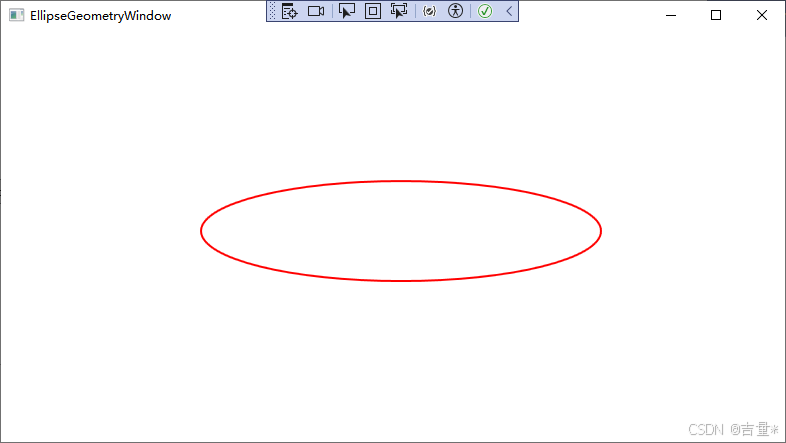
平移变换(TranslateTransform)
代码:
cs
<Window x:Class="WPFDemo.Line.Views.EllipseGeometryWindow1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WPFDemo.Line.Views"
mc:Ignorable="d"
Title="EllipseGeometryWindow1" Height="450" Width="800">
<Grid>
<Path Stroke="Red" StrokeThickness="2">
<Path.Data>
<EllipseGeometry Center="400,200" RadiusX="200" RadiusY="50" />
</Path.Data>
</Path>
<!--平移变换-->
<Path Stroke="Yellow" StrokeThickness="2">
<Path.Data>
<EllipseGeometry Center="400,200" RadiusX="200" RadiusY="50" >
<EllipseGeometry.Transform>
<TranslateTransform X="100" Y="0" />
</EllipseGeometry.Transform>
</EllipseGeometry>
</Path.Data>
</Path>
</Grid>
</Window>
效果:
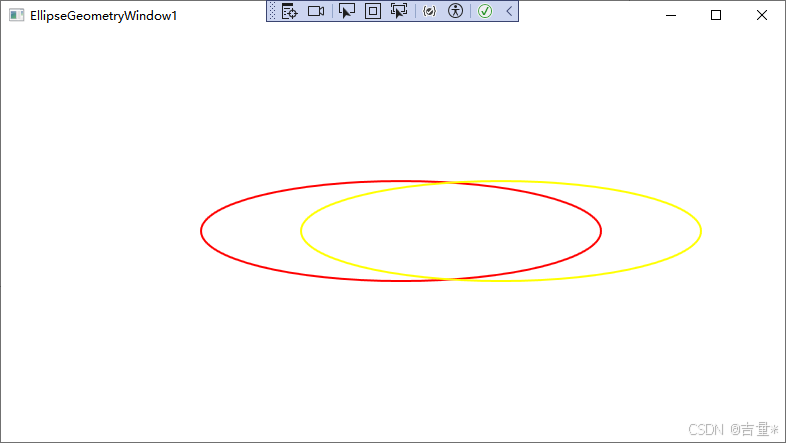
缩放变换(ScaleTransform)
代码:
cs
<Window x:Class="WPFDemo.Line.Views.EllipseGeometryWindow2"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WPFDemo.Line.Views"
mc:Ignorable="d"
Title="EllipseGeometryWindow2" Height="450" Width="800">
<Grid>
<Grid>
<Path Stroke="Red" StrokeThickness="2">
<Path.Data>
<EllipseGeometry Center="400,200" RadiusX="200" RadiusY="50" />
</Path.Data>
</Path>
<!--缩放变换-->
<Path Stroke="Yellow" StrokeThickness="2">
<Path.Data>
<EllipseGeometry Center="400,200" RadiusX="200" RadiusY="50" >
<EllipseGeometry.Transform>
<ScaleTransform ScaleX="1" ScaleY="0.7"/>
</EllipseGeometry.Transform>
</EllipseGeometry>
</Path.Data>
</Path>
</Grid>
</Grid>
</Window>
效果:
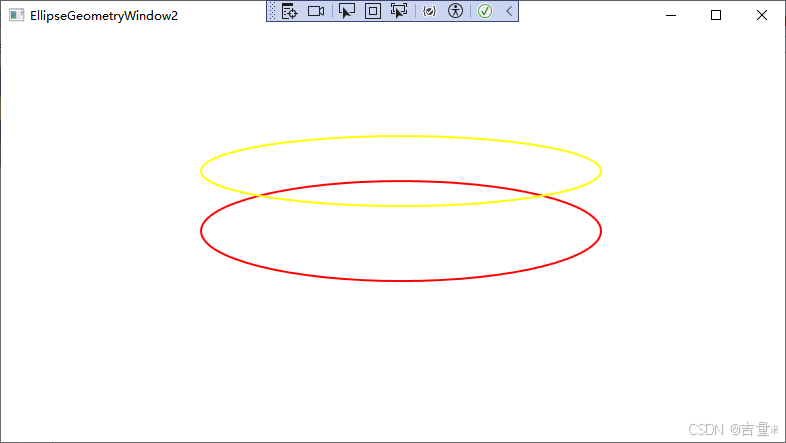
旋转变换(RotateTransform)
代码:
cs
<Window x:Class="WPFDemo.Line.Views.EllipseGeometryWindow3"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WPFDemo.Line.Views"
mc:Ignorable="d"
Title="EllipseGeometryWindow3" Height="450" Width="800">
<Grid>
<Grid>
<Path Stroke="Red" StrokeThickness="2">
<Path.Data>
<EllipseGeometry Center="400,200" RadiusX="200" RadiusY="50" />
</Path.Data>
</Path>
<!--旋转变换-->
<Path Stroke="Yellow" StrokeThickness="2">
<Path.Data>
<EllipseGeometry Center="400,200" RadiusX="200" RadiusY="50" >
<EllipseGeometry.Transform>
<RotateTransform CenterX="400" CenterY="200" Angle="90" />
</EllipseGeometry.Transform>
</EllipseGeometry>
</Path.Data>
</Path>
</Grid>
</Grid>
</Window>
效果:
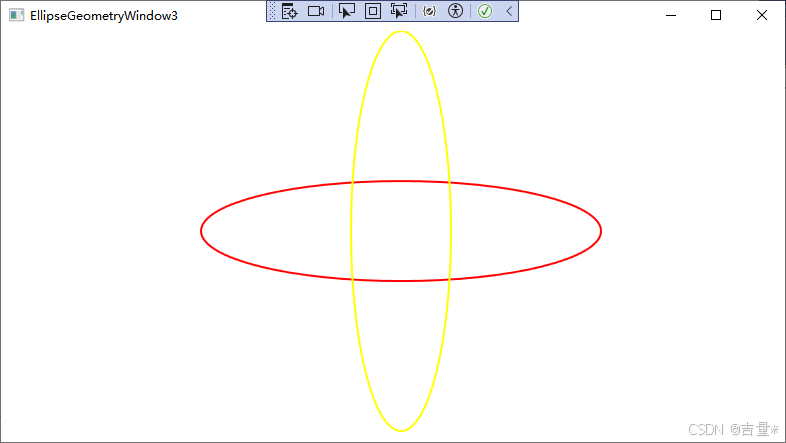
组合变换(TransformGroup)
代码:
cs
<Window x:Class="WPFDemo.Line.Views.EllipseGeometryWindow4"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WPFDemo.Line.Views"
mc:Ignorable="d"
Title="EllipseGeometryWindow4" Height="450" Width="800">
<Grid>
<Grid>
<Path Stroke="Red" StrokeThickness="2">
<Path.Data>
<EllipseGeometry Center="400,200" RadiusX="200" RadiusY="50" />
</Path.Data>
</Path>
<!--组合变换-->
<Path Stroke="Yellow" StrokeThickness="2">
<Path.Data>
<EllipseGeometry Center="400,200" RadiusX="200" RadiusY="50" >
<EllipseGeometry.Transform>
<TransformGroup>
<TranslateTransform X="100" Y="0" />
<ScaleTransform ScaleX="1" ScaleY="0.7"/>
<RotateTransform CenterX="400" CenterY="200" Angle="90" />
</TransformGroup>
</EllipseGeometry.Transform>
</EllipseGeometry>
</Path.Data>
</Path>
</Grid>
</Grid>
</Window>
效果:
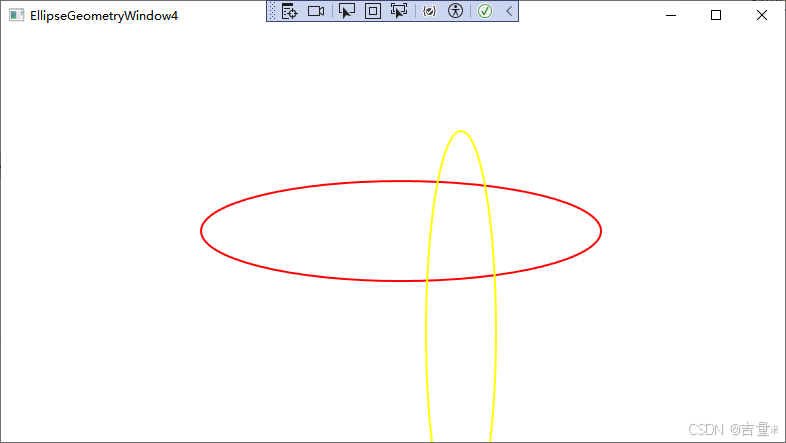