1.题目解析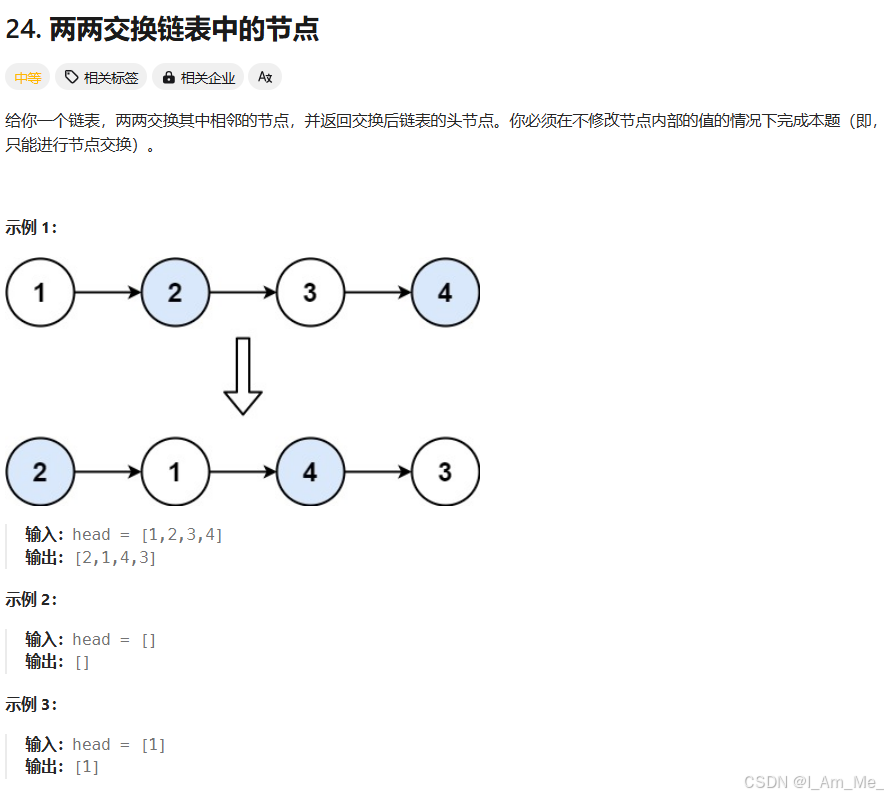
2.讲解算法原理
- 让前两个节点后面的链表先逆置,并且把头结点返回
- 把当前两个结点进行交换,并且找到新的头结点
- 把后面链表直接添加到当前已经逆置的两个节点后面
3.编写代码
java
复制代码
/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode() {}
* ListNode(int val) { this.val = val; }
* ListNode(int val, ListNode next) { this.val = val; this.next = next; }
* }
*/
class Solution {
public ListNode swapPairs(ListNode head) {
ListNode newHead=dfs(head);
return newHead;
}
public ListNode dfs(ListNode head){
if(head==null||head.next==null){
return head;
}
ListNode newHead=dfs(head.next.next);
ListNode ret=head.next;
ret.next=head;
head.next=newHead;
return ret;
}
}