基于Springboot的dubb调用
1.zookeeper的使用
-
下载window版本的zookeeper
shell官网下载地址:https://archive.apache.org/dist/zookeeper/zookeeper-3.6.4/apache-zookeeper-3.6.4-bin.tar.gz
-
下载后,解压到非中文目录下,然后,创建data目录
-
将zoo_sample.cfg拷贝一份,并命名zoo.cfg
-
更改zoo.cfg内容,并保存
shell# The number of milliseconds of each tick tickTime=2000 # The number of ticks that the initial # synchronization phase can take initLimit=10 # The number of ticks that can pass between # sending a request and getting an acknowledgement syncLimit=5 # the directory where the snapshot is stored. # do not use /tmp for storage, /tmp here is just # example sakes. dataDir=D:\\JavaDev\\zookeeper-3.6.4\\data # 存放的数据目录 # the port at which the clients will connect clientPort=2181 # zookeeper客户端连接端口
-
双击进行启动
2.创建父工程进行依赖管理
创建一个maven项目进行管理,项目结构、代码如下:
pom.xml
xml
<groupId>com.demo</groupId>
<artifactId>dubbo-demo</artifactId>
<packaging>pom</packaging>
<version>1.0-SNAPSHOT</version>
<modules>
<module>dubbo-api</module>
<module>dubbo-provider</module>
<module>dubbo-consumer</module>
</modules>
<properties>
<dubbo.version>2.7.3</dubbo.version>
<boot.version>2.7.2</boot.version>
</properties>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>${boot.version}</version>
</dependency>
<dependency>
<groupId>org.apache.dubbo</groupId>
<artifactId>dubbo-spring-boot-starter</artifactId>
<version>${dubbo.version}</version>
</dependency>
<!--注意类型必须为pom-->
<dependency>
<groupId>org.apache.dubbo</groupId>
<artifactId>dubbo-dependencies-zookeeper</artifactId>
<version>${dubbo.version}</version>
<type>pom</type>
</dependency>
</dependencies>
</dependencyManagement>
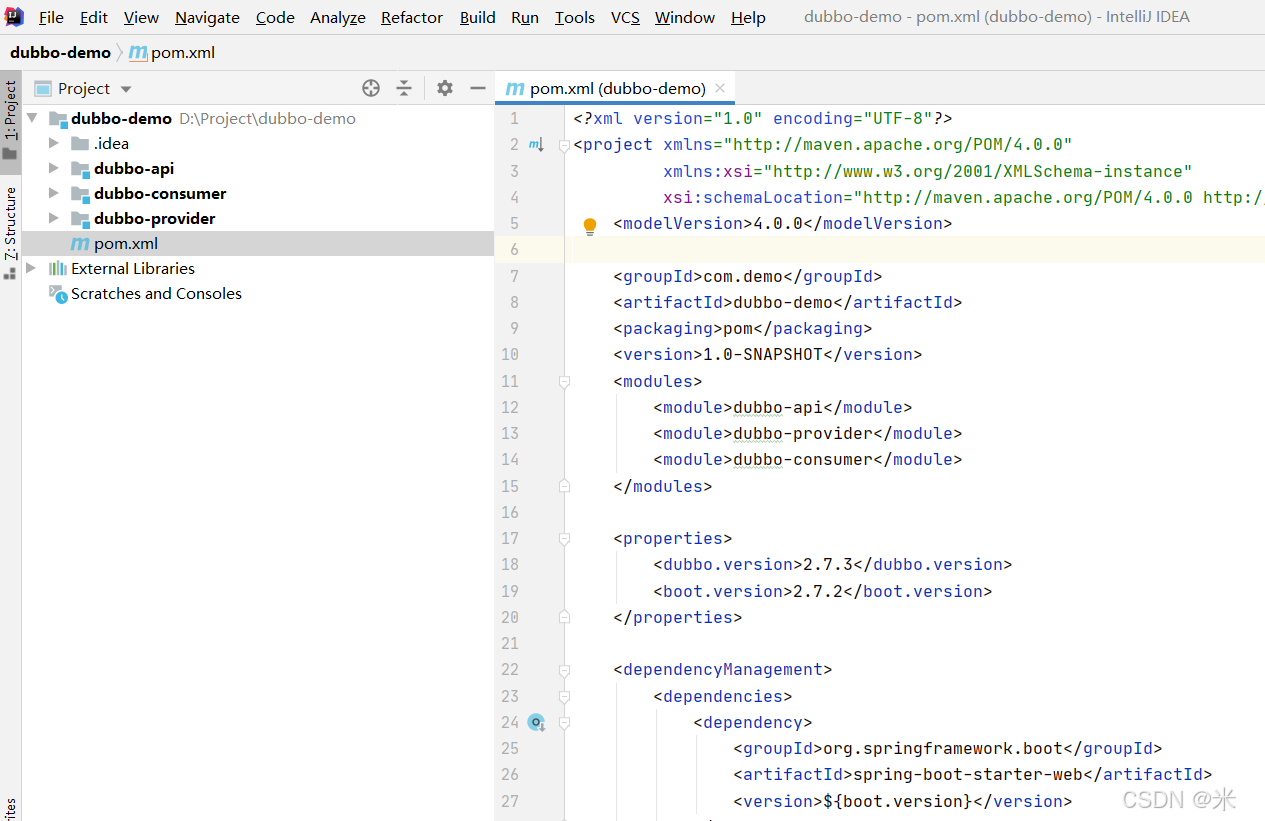
3.创建dubbo-api公共模块
项目结构如下图,主要是创建公共的用户接口和用户实体类:
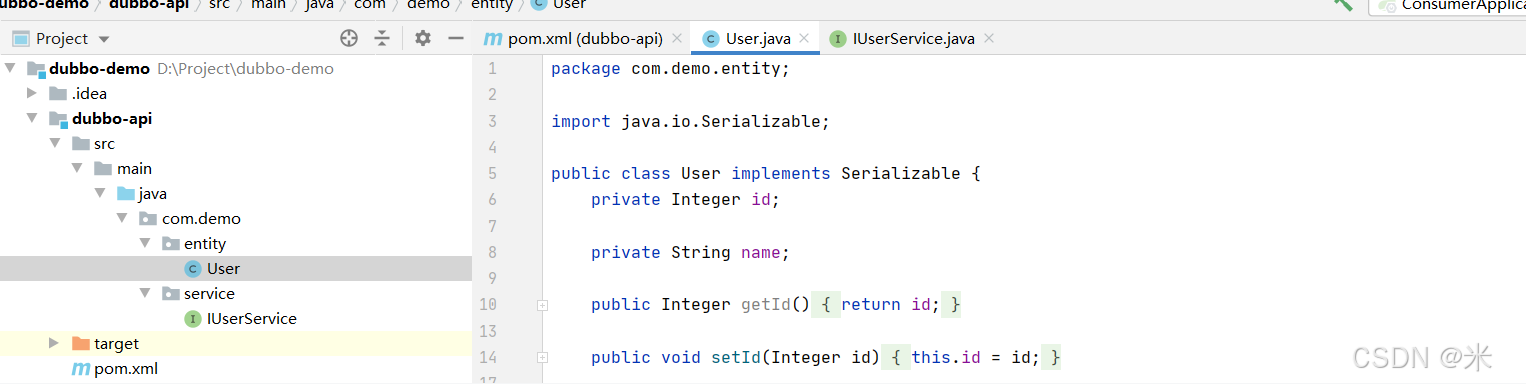
用户接口:
java
package com.demo.service;
import com.demo.entity.User;
public interface IUserService {
public User selectUserById(Integer id);
}
项目的pom文件依赖:
xml
<dependencies>
<dependency>
<groupId>org.apache.dubbo</groupId>
<artifactId>dubbo-spring-boot-starter</artifactId>
</dependency>
<!--注意类型必须为pom-->
<dependency>
<groupId>org.apache.dubbo</groupId>
<artifactId>dubbo-dependencies-zookeeper</artifactId>
<type>pom</type>
<!--防止依赖冲突,这个必须排除-->
<exclusions>
<exclusion>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-log4j12</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
4.dubbo服务注册
创建dubbo-provider模块进行服务注册,使用之前需要引入dubbo-api模块。
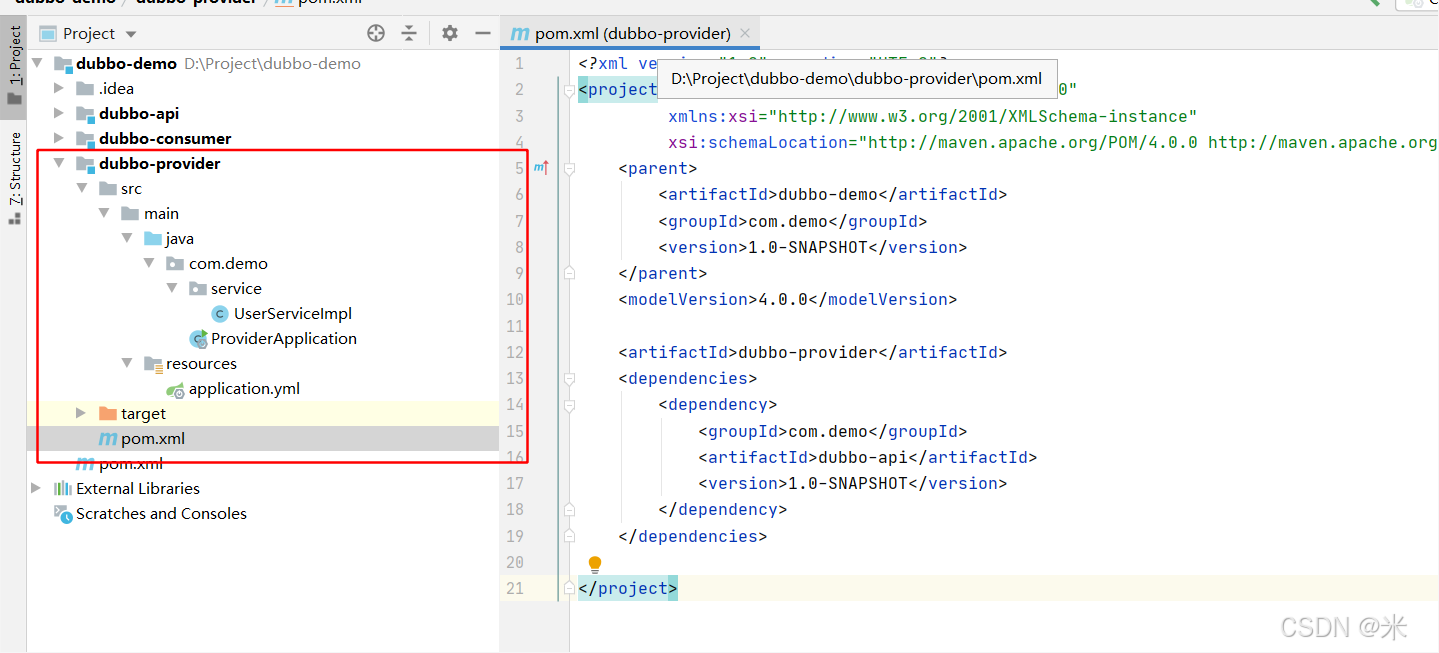
用户类服务实现:
@service
的注解位于org.apache.dubbo下,不是spring的
java
package com.demo.service;
import com.demo.entity.User;
import org.apache.dubbo.config.annotation.Service;
@Service
public class UserServiceImpl implements IUserService{
@Override
public User selectUserById(Integer id) {
User user = new User();
user.setId(id);
user.setName("hhh");
return user;
}
}
在application.yml配置服务注册:
yaml
server:
port: 8081
dubbo:
registry:
address: zookeeper://localhost:2181
timeout: 6000
protocol:
name: dubbo #协议名称
port: 10888 # 通信端口
application:
name: provider
scan:
base-packages: com.demo.service # 扫描成服务的包名
5.dubbo服务发现
创建dubbo-consumer模块进行服务消费,使用之前需要引入dubbo-api模块。
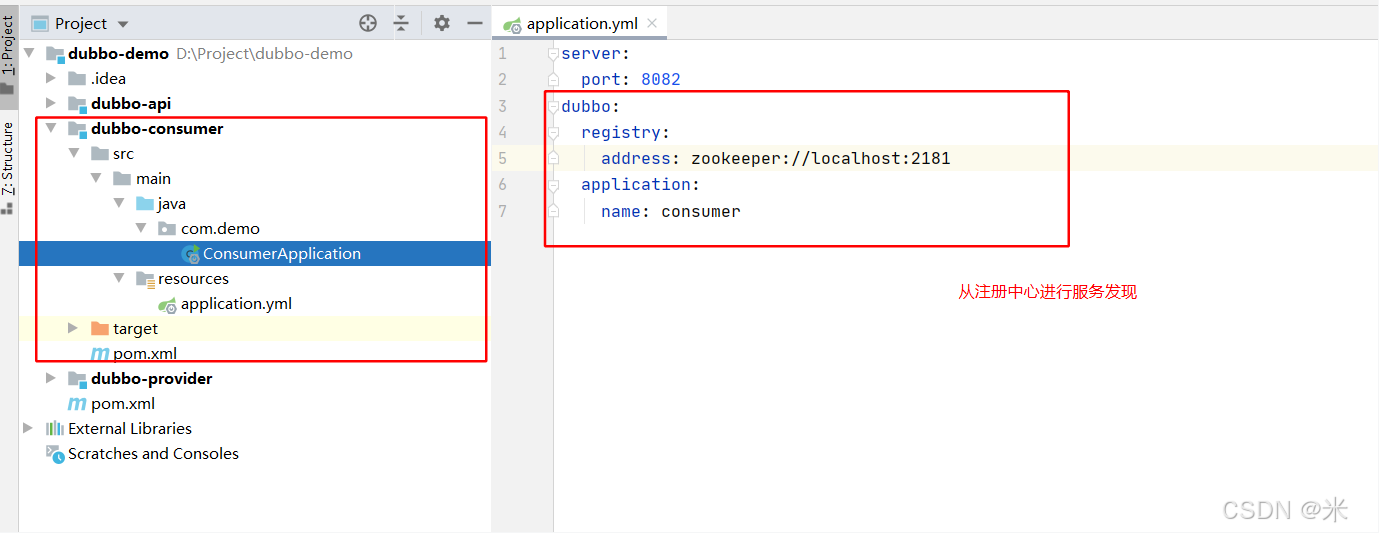
消费对方暴露的服务:
@Reference
表示从注册中心拉取对方的服务,并实例化
java
package com.demo;
import com.demo.entity.User;
import com.demo.service.IUserService;
import org.apache.dubbo.config.annotation.Reference;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class ConsumerApplication implements CommandLineRunner {
public static void main(String[] args) {
SpringApplication.run(ConsumerApplication.class, args);
}
@Reference
private IUserService userService;
@Override
public void run(String... args) throws Exception {
User user = userService.selectUserById(888);
System.out.println(user);
}
}
6.测试
启动dubbo-provider程序:
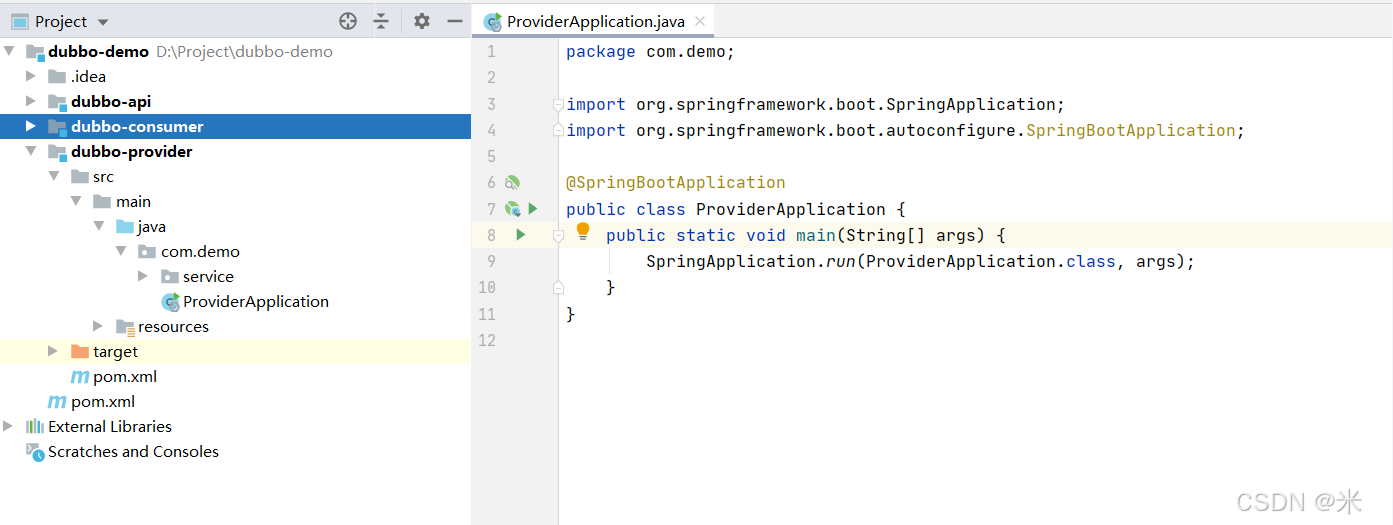
启动dubbo-consumer程序:
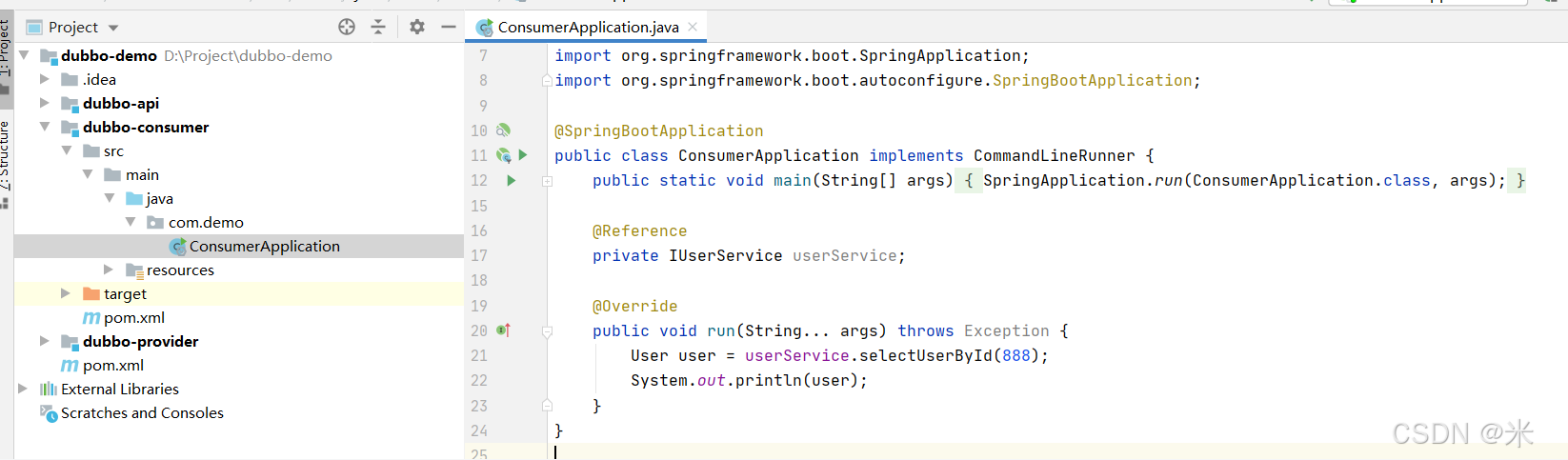
结果如下:
