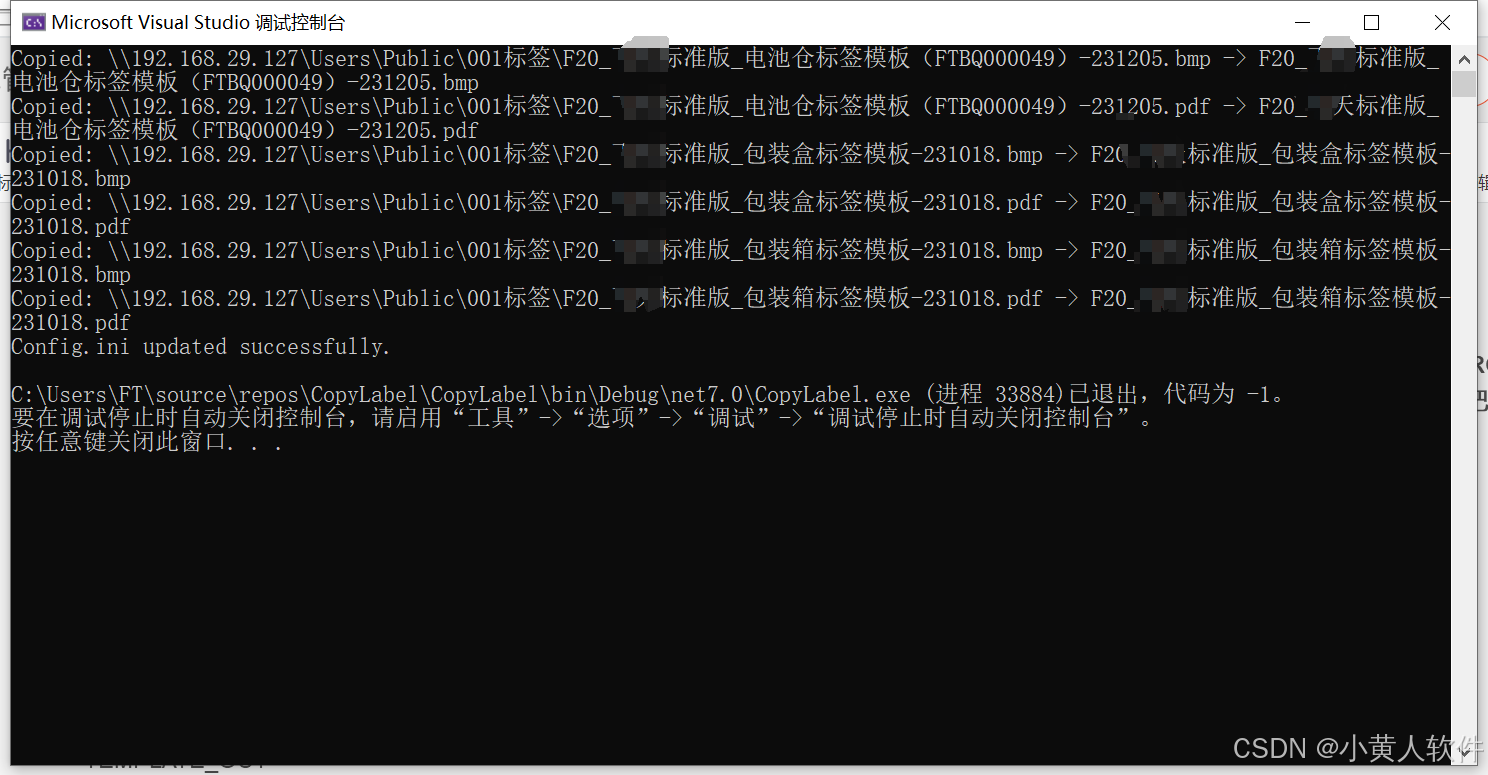
减少测试等人员重复配置或复制标签的功能:一次配置,终身使用
【开发人员】放标签到远程并手工配置好
【使用人员】只需选择型号和group,点从远程获取,所有标签与pdf自动从远程复制到本地。(比如F20标准版)
远程获取标签方案
用C#写一个程序:读取config.ini文件里的Model和TEMPLATE_GROUP,在label.ini文件中读取[Model@TEMPLATE_GROUP]下所有key-value把这个key-value写入到[PRINT]下,并且复制每个Path+value文件复制到当前目录。如果EnableCopyPdf=1把value对应的.pdf(即把文件扩展名.bmp改成.pdf)也复制到当前目录。
config.ini文件
[DEVICE]
Model=F200
[PRINT]
TEMPLATE_GROUP=0
TEMPLATE=
TEMPLATE_OUT=
TEMPLATE_BOX=
label.ini文件
[Common]
#所有开发调试好的标签存放在这里
Path=\192.168.29.127\Users\Public\001标签
EnableCopyPdf=1
[F200@0]
TEMPLATE=F20_测试标准版_电池仓标签模板(FTBQ000049)-231205.bmp
TEMPLATE_OUT=F20_测试标准版_包装盒标签模板-231018.bmp
TEMPLATE_BOX=F20_测试标准版_包装箱标签模板-231018.bmp
打包dll 没成功 因为非.net framework的dll无法打包
cd C:\Users\FT\source\repos\CopyLabel\CopyLabel\bin\Release\net7.0
ILRepack.exe /out:CopyLabelMerged.exe CopyLabel.exe CopyLabel.dll
csharp
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
class Program
{
static void Main()
{
string configPath = "config.ini";
string labelPath = "label.ini";
// 读取 config.ini 文件
var config = ReadIniFile(configPath);
string model = config["DEVICE"]["Model"];
string templateGroup = config["PRINT"]["TEMPLATE_GROUP"];
// 读取 label.ini 文件
var labelConfig = ReadIniFile(labelPath);
string sectionKey = $"{model}@{templateGroup}";
if (!labelConfig.ContainsKey(sectionKey))
{
Console.WriteLine($"Section [{sectionKey}] not found in label.ini");
return;
}
// 检查是否启用 PDF 文件复制
bool enableCopyPdf = labelConfig["Common"].ContainsKey("EnableCopyPdf") &&
labelConfig["Common"]["EnableCopyPdf"] == "1";
// 获取指定 section 的键值对
var keyValuePairs = labelConfig[sectionKey];
// 写入 [PRINT] 部分
if (!config.ContainsKey("PRINT"))
{
config["PRINT"] = new Dictionary<string, string>();
}
foreach (var kvp in keyValuePairs)
{
config["PRINT"][kvp.Key] = kvp.Value;
// 复制文件到当前目录
string path = labelConfig["Common"]["Path"];
string sourceFile = Path.Combine(path, kvp.Value);
string destFile = Path.GetFileName(kvp.Value);
try
{
File.Copy(sourceFile, destFile, overwrite: true);
Console.WriteLine($"Copied: {sourceFile} -> {destFile}");
}
catch (Exception ex)
{
Console.WriteLine($"Error copying {sourceFile}: {ex.Message}");
}
// 如果启用 PDF 文件复制,复制对应的 .pdf 文件
if (enableCopyPdf)
{
string pdfSourceFile = Path.ChangeExtension(sourceFile, ".pdf");
string pdfDestFile = Path.ChangeExtension(destFile, ".pdf");
try
{
File.Copy(pdfSourceFile, pdfDestFile, overwrite: true);
Console.WriteLine($"Copied: {pdfSourceFile} -> {pdfDestFile}");
}
catch (Exception ex)
{
Console.WriteLine($"Error copying {pdfSourceFile}: {ex.Message}");
}
}
}
// 保存更新后的 config.ini
WriteIniFile(configPath, config);
Console.WriteLine("Config.ini updated successfully.");
Console.ReadLine();
}
// 读取 ini 文件为字典结构
static Dictionary<string, Dictionary<string, string>> ReadIniFile(string filePath)
{
var data = new Dictionary<string, Dictionary<string, string>>();
string currentSection = null;
foreach (var line in File.ReadLines(filePath))
{
string trimmed = line.Trim();
if (string.IsNullOrEmpty(trimmed) || trimmed.StartsWith("#"))
continue;
if (trimmed.StartsWith("[") && trimmed.EndsWith("]"))
{
currentSection = trimmed.Trim('[', ']');
if (!data.ContainsKey(currentSection))
{
data[currentSection] = new Dictionary<string, string>();
}
}
else if (currentSection != null && trimmed.Contains("="))
{
var parts = trimmed.Split(new[] { '=' }, 2);
string key = parts[0].Trim();
string value = parts[1].Trim();
data[currentSection][key] = value;
}
}
return data;
}
// 将字典结构写入 ini 文件
static void WriteIniFile(string filePath, Dictionary<string, Dictionary<string, string>> data)
{
var sb = new StringBuilder();
foreach (var section in data)
{
sb.AppendLine($"[{section.Key}]");
foreach (var kvp in section.Value)
{
sb.AppendLine($"{kvp.Key}={kvp.Value}");
}
sb.AppendLine();
}
File.WriteAllText(filePath, sb.ToString());
}
}