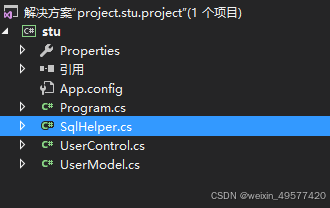
1#数据库操作类 SqlHelper.cs
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Data.SqlClient;
using System.Data;
namespace stu
{
class SqlHelper
{
private static string DbConfig = "Server=localhost;database=data_student;Trusted_Connection=True;";
/// <summary>
/// 对数据库进行 添加,修改,删除操作
/// </summary>
/// <param name="sql"></param>
/// <returns></returns>
public static int EditSql(string sql)
{
SqlConnection conn = new SqlConnection();
conn.ConnectionString = DbConfig;
conn.Open();
SqlCommand command = new SqlCommand(sql,conn);
int count = 0;
try
{
count = command.ExecuteNonQuery();
}
catch (Exception)
{
count = -1;
Console.WriteLine("数据库写入失败!");
}
command.Dispose();
conn.Close();
return count;
}
/// <summary>
/// 查询数据库,返回DataTable
/// </summary>
/// <param name="sql"></param>
/// <returns></returns>
public static DataTable LookUp(string sql)
{
SqlConnection conn = new SqlConnection();
conn.ConnectionString = DbConfig;
conn.Open();
SqlCommand command = new SqlCommand(sql, conn);
SqlDataAdapter apter = new SqlDataAdapter();
apter.SelectCommand = command;
DataSet ds = new DataSet();
apter.Fill(ds);
DataTable table = ds.Tables[0];
conn.Close();
return table;
}
}
}
2# 实体类 UserModel.cs
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace stu
{
public class UserModel
{
public string UserName { get; set; }
public string PassWord { get; set; }
public string NickName { get; set; }
public string Gender { get; set; }
public override string ToString()
{
return string.Format("UserName:{0} PassWord:{1} NickName:{2} Gender:{3}",UserName,PassWord,NickName,Gender);
}
}
}
3# 数据交换层 UserControl.cs
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Data;
namespace stu
{
public class UserControl
{
public static UserModel DataRowToUserModel(DataRow currentRow)
{
UserModel user = new UserModel();
user.UserName = currentRow["UserName"].ToString().Trim();
user.PassWord = currentRow["PassWord"].ToString().Trim();
user.NickName = currentRow["NickName"].ToString().Trim();
user.Gender = currentRow["Gender"].ToString().Trim();
return user;
}
/// <summary>
/// 登录,如果返回null 说明不存在
/// </summary>
/// <param name="username"></param>
/// <param name="pwd"></param>
/// <returns></returns>
public static UserModel Login(string username,string pwd)
{
string sql = $"select * from TableUser where UserName='{username}' and PassWord='{pwd}'; ";
DataTable table= SqlHelper.LookUp(sql);
if (table.Rows.Count<=0) // 这里要用小于等于0
{
return null;
}
UserModel user = DataRowToUserModel(table.Rows[0]);
return user;
}
/// <summary>
/// 返回所有数据
/// </summary>
/// <returns></returns>
public static List<UserModel> ShowAllUsers()
{
List<UserModel> list = new List<stu.UserModel>();
string sql = $"select * from TableUser";
DataTable table= SqlHelper.LookUp(sql);
if (table.Rows.Count<=0)
{
return null;
}
for (int i = 0; i < table.Rows.Count; i++)
{
UserModel model = DataRowToUserModel(table.Rows[i]);
list.Add(model);
}
return list;
}
/// <summary>
/// 修改用户信息
/// </summary>
/// <param name="user"></param>
public static bool EditUserInfo(UserModel user)
{
string sql = $"update TableUser SET password='{user.PassWord}',nickname='{user.NickName}',gender='{user.Gender}'where username='{user.UserName}'; ";
int count = SqlHelper.EditSql(sql);
if (count>=0)
{
return true;
}else
{
return false;
}
}
}
}
界面层
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Data;
namespace stu
{
class Program
{
static void Main(string[] args)
{
while (true)
{
Console.WriteLine("欢迎进入登录系统....");
Console.Write("输入用户名:");
string name = Console.ReadLine();
Console.Write("输入用户密码:");
string pwd = Console.ReadLine();
UserModel user = UserControl.Login(name, pwd);
if (user==null)
{
Console.WriteLine("不存在");
continue;
}else
{
Console.WriteLine("登录成功");
break;
}
}
List<UserModel> users = UserControl.ShowAllUsers();
foreach (UserModel item in users)
{
Console.WriteLine(item);
}
// 修改数据
Console.WriteLine("------------------------------------------------------------------------" );
UserModel u = new UserModel() { UserName = "李阿猫", PassWord = "123456", NickName = "guoguo"};
bool flag = UserControl.EditUserInfo(u);
if (flag)
{
Console.WriteLine("修改成功");
}
Console.ReadKey();
}
}
}