题目
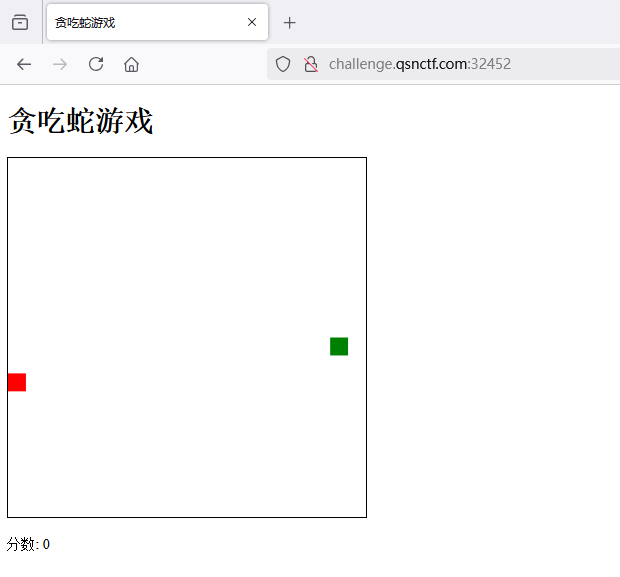
Ctrl+U快捷键查看页面源代码
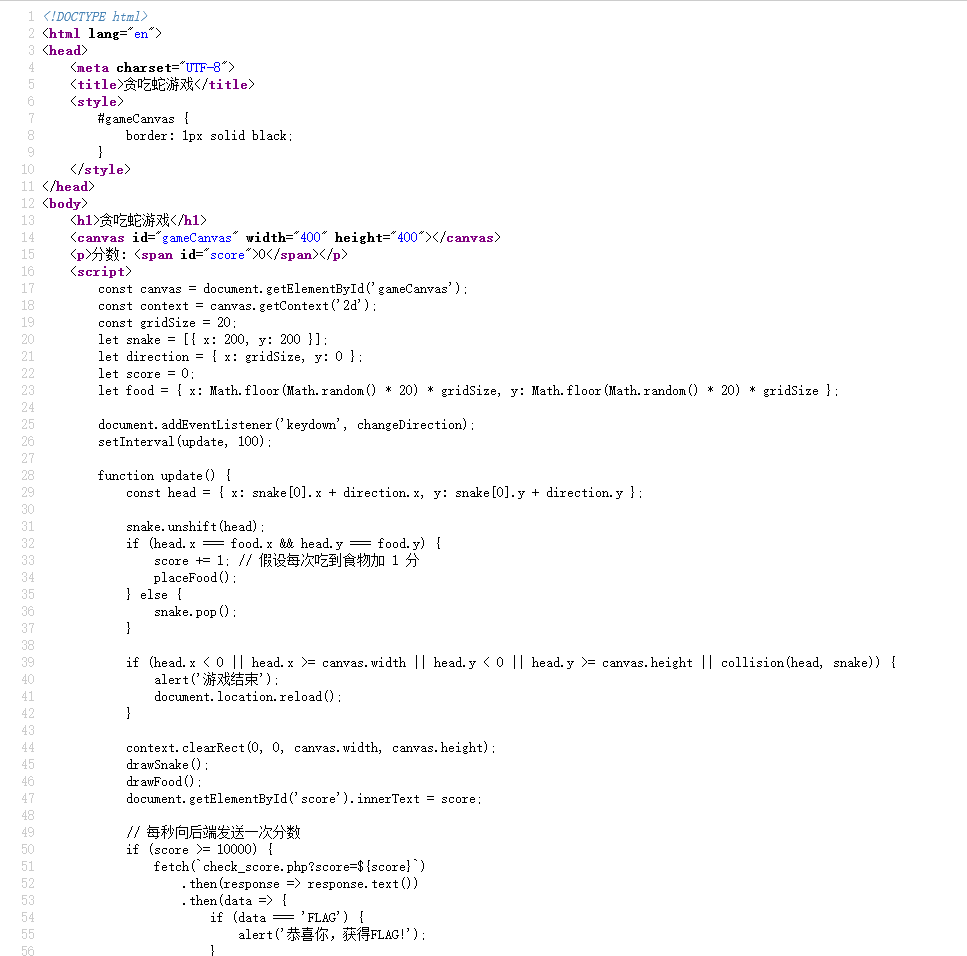
源码
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>贪吃蛇游戏</title>
<style>
#gameCanvas {
border: 1px solid black;
}
</style>
</head>
<body>
<h1>贪吃蛇游戏</h1>
<canvas id="gameCanvas" width="400" height="400"></canvas>
<p>分数: <span id="score">0</span></p>
<script>
const canvas = document.getElementById('gameCanvas');
const context = canvas.getContext('2d');
const gridSize = 20;
let snake = [{ x: 200, y: 200 }];
let direction = { x: gridSize, y: 0 };
let score = 0;
let food = { x: Math.floor(Math.random() * 20) * gridSize, y: Math.floor(Math.random() * 20) * gridSize };
document.addEventListener('keydown', changeDirection);
setInterval(update, 100);
function update() {
const head = { x: snake[0].x + direction.x, y: snake[0].y + direction.y };
snake.unshift(head);
if (head.x === food.x && head.y === food.y) {
score += 1; // 假设每次吃到食物加 1 分
placeFood();
} else {
snake.pop();
}
if (head.x < 0 || head.x >= canvas.width || head.y < 0 || head.y >= canvas.height || collision(head, snake)) {
alert('游戏结束');
document.location.reload();
}
context.clearRect(0, 0, canvas.width, canvas.height);
drawSnake();
drawFood();
document.getElementById('score').innerText = score;
// 每秒向后端发送一次分数
if (score >= 10000) {
fetch(`check_score.php?score=${score}`)
.then(response => response.text())
.then(data => {
if (data === 'FLAG') {
alert('恭喜你,获得FLAG!');
}
});
}
}
function changeDirection(event) {
switch (event.keyCode) {
case 37:
if (direction.x === 0) direction = { x: -gridSize, y: 0 };
break;
case 38:
if (direction.y === 0) direction = { x: 0, y: -gridSize };
break;
case 39:
if (direction.x === 0) direction = { x: gridSize, y: 0 };
break;
case 40:
if (direction.y === 0) direction = { x: 0, y: gridSize };
break;
}
}
function drawSnake() {
context.fillStyle = 'green';
snake.forEach(segment => {
context.fillRect(segment.x, segment.y, gridSize, gridSize);
});
}
function drawFood() {
context.fillStyle = 'red';
context.fillRect(food.x, food.y, gridSize, gridSize);
}
function placeFood() {
food = {
x: Math.floor(Math.random() * 20) * gridSize,
y: Math.floor(Math.random() * 20) * gridSize,
};
}
function collision(head, snake) {
for (let i = 1; i < snake.length; i++) {
if (head.x === snake[i].x && head.y === snake[i].y) {
return true;
}
}
return false;
}
</script>
</body>
</html>
代码分析
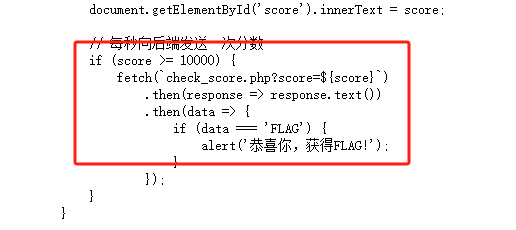
check_score.php接口传参score当score >= 10000获取flag
payload
html
/check_score.php?score=10000
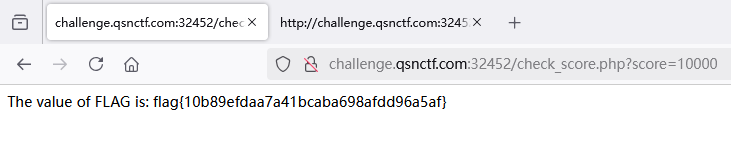
成功获取flag
flag{10b89efdaa7a41bcaba698afdd96a5af}