目录
练习1:标题统计
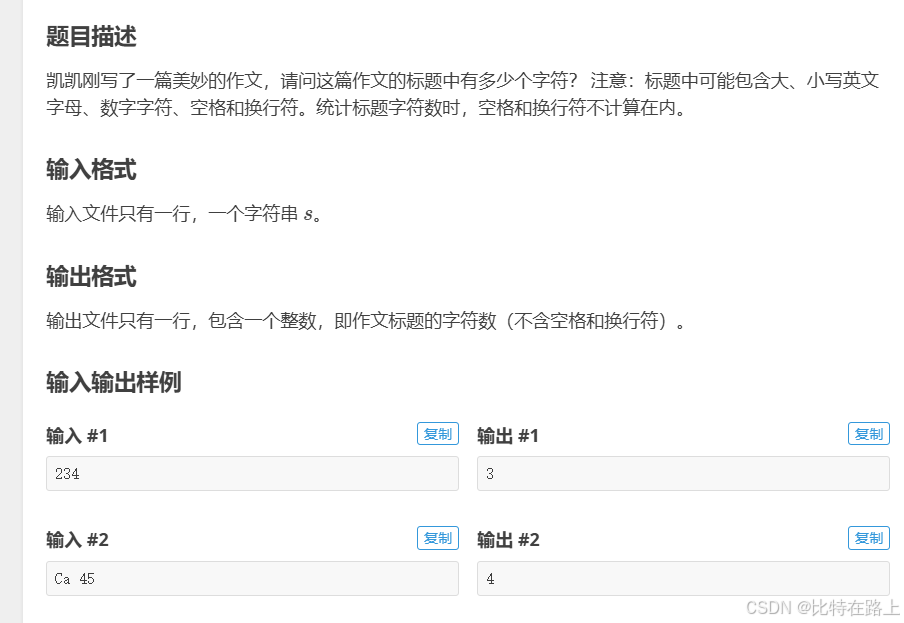
方法1:一次性读取整行字符,然后统计
cpp
#include <iostream>
using namespace std;
#include <string>
#include <cctype>
int main()
{
string s;
getline(cin , s);
int cint = 0;
for(auto e: s)
{
if(isspace(e))
{
continue;
}
else
{
cint++;
}
}
cout << cint << endl;
return 0;
}
注:isspace()函数是专门来判断一个字符是否为空白字符(空格、换行符都为空白字符);需要包含头文件<cctype>;
方法2:按照单词读取
cpp
测试点信息源代码
源代码 复制
#include <iostream>
using namespace std;
#include <string>
int main()
{
string s;
int count = 0;
while(cin >> s)
{
count += s.size();
}
cout << count << endl;
return 0;
}
小提示:
有时候处理一个字符串的时候,也不一定要一次性读取完整个字符串,如果字符串中有空格的话,其实可以当做多个单词,一次读取。
cin >>S会返回一个流对象的引l用,即cin本身。在 C++中,流对象(如cin)可以被用作布尔值来检查流的状态。如果流的状态良好(即没有发生错误),流对像的布尔值为true。如果发生错误(如遇到输入结束符或类型不匹配),布尔值为false。
在while(cin>>s)语句中,循环的条件部分检查cin流的状态。如果流成功读取到一个值,cin >〉s返回的流对象cin 将被转换为true,循环将继续。如果读取失败(例如遇到输入结束符或无法读取到一个值),cin>〉s返回的流对象cin 将被转换为false,循环将停止。
练习2:石头剪子布
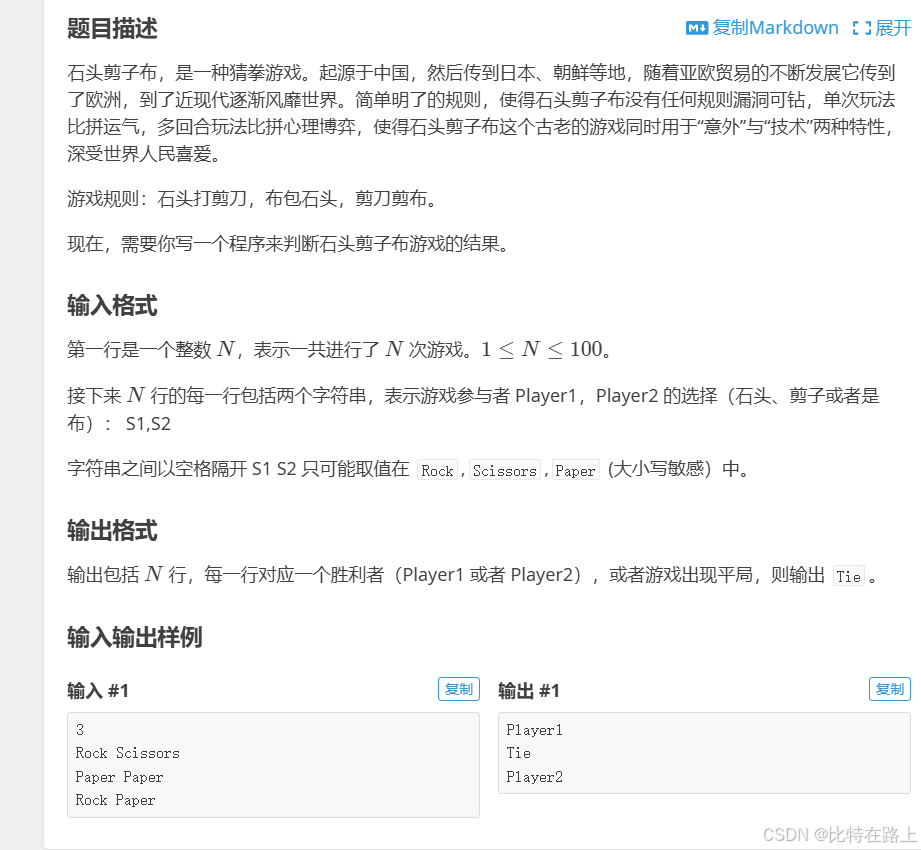
cpp
#include <iostream>
#include <string>
using namespace std;
int main()
{
int n = 0;
cin >> n;
int i = 0;
string s1;
string s2;
while(n--)
{
cin >> s1;
cin >> s2;
if (s1 == s2)
cout << "Tie" << endl;
else if (s1 == "Rock" && s2 == "Scissors")
cout << "Player1" << endl;
else if (s1 == "Scissors" && s2 == "Paper")
cout << "Player1" << endl;
else if (s1 == "Paper" && s2 == "Rock")
cout << "Player1" << endl;
else
cout << "Player2" << endl;
}
return 0;
}
练习3:密码翻译
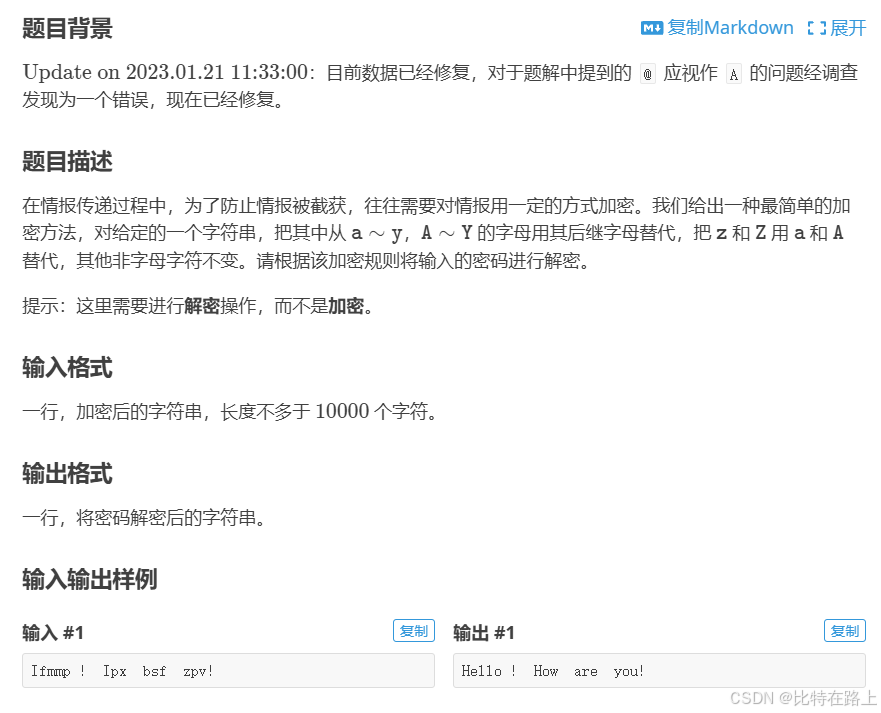
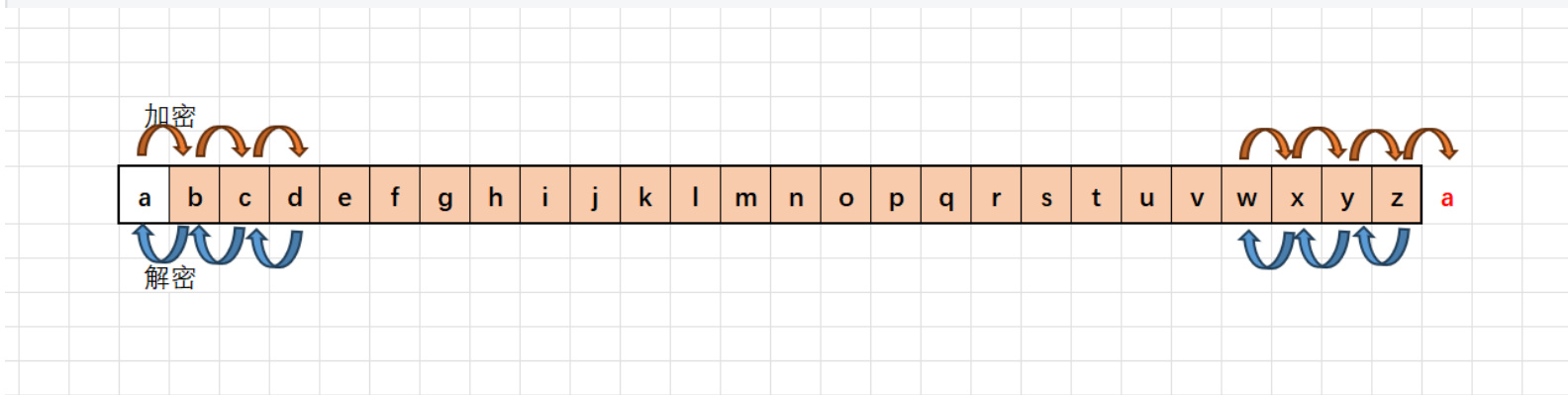
cpp
#include <iostream>
using namespace std;
#include <string>
int main()
{
string s;
getline(cin, s);
for(int i = 0; i < s.size(); i++)
{
if(s[i] <= 'z' && s[i] > 'a' || s[i] > 'A' && s[i] <= 'Z')
{
s[i]--;
}
else if(s[i] == 'a')
{
s[i] = 'z';
}
else if(s[i] == 'A')
{
s[i] = 'Z';
}
else
continue;
}
cout << s << endl;
return 0;
}
练习4:文字处理软件
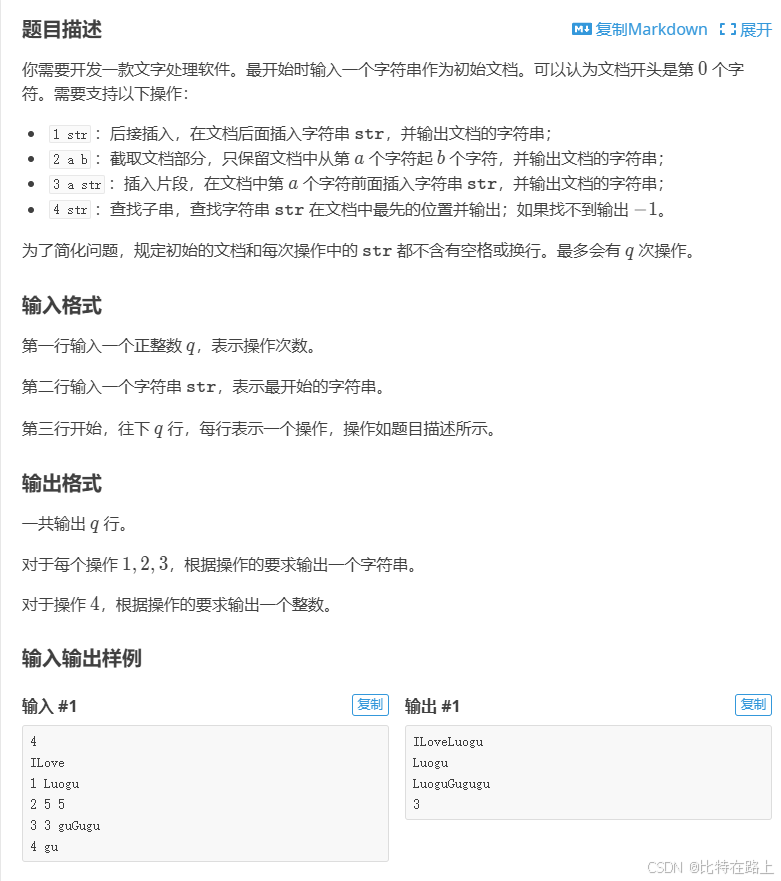
cpp
#include <iostream>
using namespace std;
#include <string>
int main()
{
int q; //循环几次
int m;
string s;//原始字符串
string str; //需要处理的数据
int a, b;
cin >> q >> s;
while (q--)
{
cin >> m;
switch (m)
{
case 1:
cin >> str;
s += str;
cout << s << endl;
break;
case 2:
cin >> a >> b;
s = s.substr(a, b);
cout << s << endl;
break;
case 3:
{
cin >> a >> str;
string s1 = s.insert(a, str);
cout << s1 << endl;
break;
}
case 4:
cin >> str;
size_t n = s.find(str);
if (n == string::npos)
cout << -1 << endl;
else
cout << n << endl;
break;
}
}
return 0;
}
练习5:单词的长度
cpp
#include <iostream>
using namespace std;
#include <string>
int main()
{
string s;
cin >> s;
cout << s.size();
while(cin >> s)
{
cout << ',' << s.size();
}
return 0;
}
cpp
//还可以这样处理:
int main()
{
string s;
bool flag = true;
while(cin >> s)
{
if(flag == true)
{
cout << s.size();
flag = false;
}
else
{
size_t n = s.size();
cout << ',' << n;
}
}
return 0;
}
练习6:单词翻转
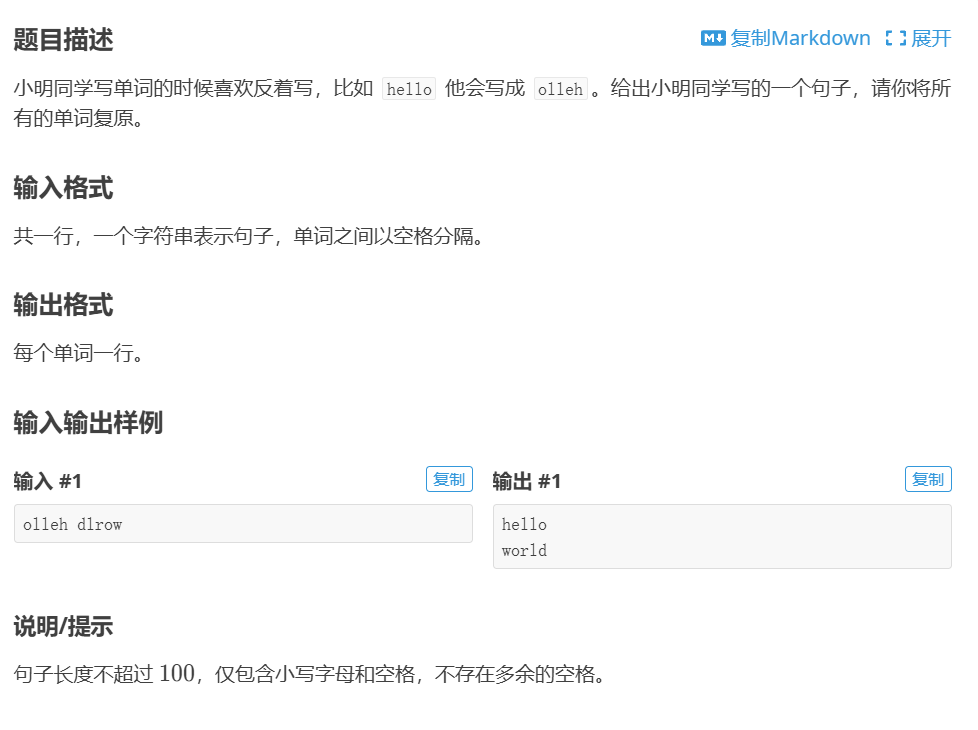
cpp
int main()
{
string str;
while (cin >> str)
{
int left = 0;
int right = str.size() - 1;
//⼿动逆序
while (left < right)
{
char tmp = str[left];
str[left] = str[right];
str[right] = tmp;
left++;
right--;
}
cout << str << endl;
}
return 0;
}
其实在C++ 的STL中,包含一个算法叫reverse,可以完成字符串的逆序(反转)。需要的头文件是<algorithm>
reverse 会逆序范围[first,last)内的元素。
cpp
string s = "abcdef";
reverse(s.begin(), s.end())
这⾥做个测试:
cpp
#include <iostream>
#include <algorithm>
using namespace std;
int main()
{
//反转字符串
string s("hello world");
reverse(s.begin(), s.end());
cout << s << endl;
//反转数组
int arr[] = { 2,6,3,6,5,5,3,9,3 };
int size = sizeof(arr) / sizeof(arr[0]);
//对数组中的元素进?反转
reverse(arr, arr+size);
for (auto e : arr)
{
cout << e << " ";
}
cout << endl;
return 0;
}
练习7:判断字符串是否为回文
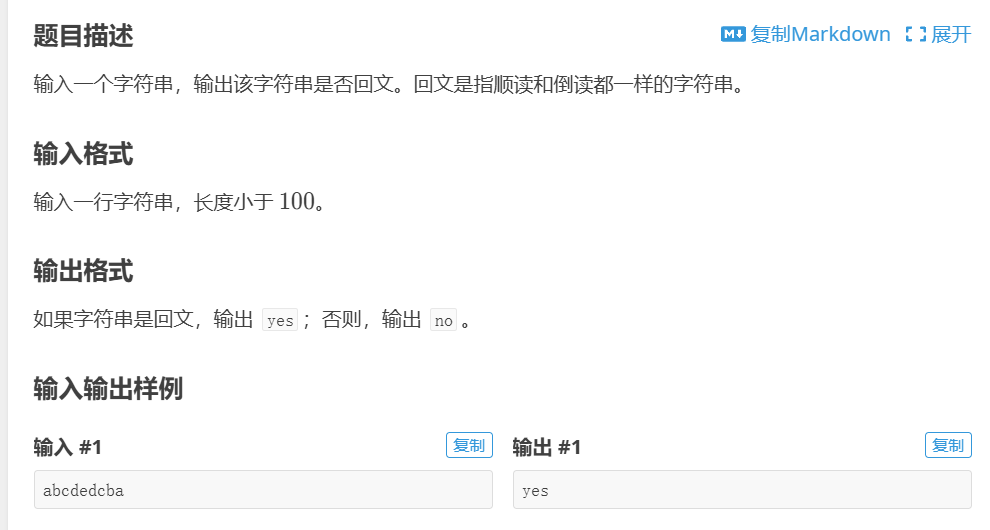
cpp
#include <iostream>
#include <algorithm>
using namespace std;
int main()
{
string s;
getline(cin, s);
int left = 0;
int right = s.size() - 1;
while(left < right)
{
if(s[left] != s[right])
{
cout << "no" << endl;
return 0;
}
else
{
left++;
right--;
}
}
cout << "yes" << endl;
return 0;
}
当然也可以将这个字符串逆序后和原字符串比较,看是否相等,如果相等就是回文字符串,否则就不是。代码也可以这样写:
cpp
#include <iostream>
#include <algorithm>
using namespace std;
#include <algorithm>
int main()
{
string s;
getline(cin, s);
string s1 = s;
reverse(s.begin(), s.end());
if(s == s1)
cout << "yes" << endl;
else
cout << "no" << endl;
return 0;
}
练习8:手机
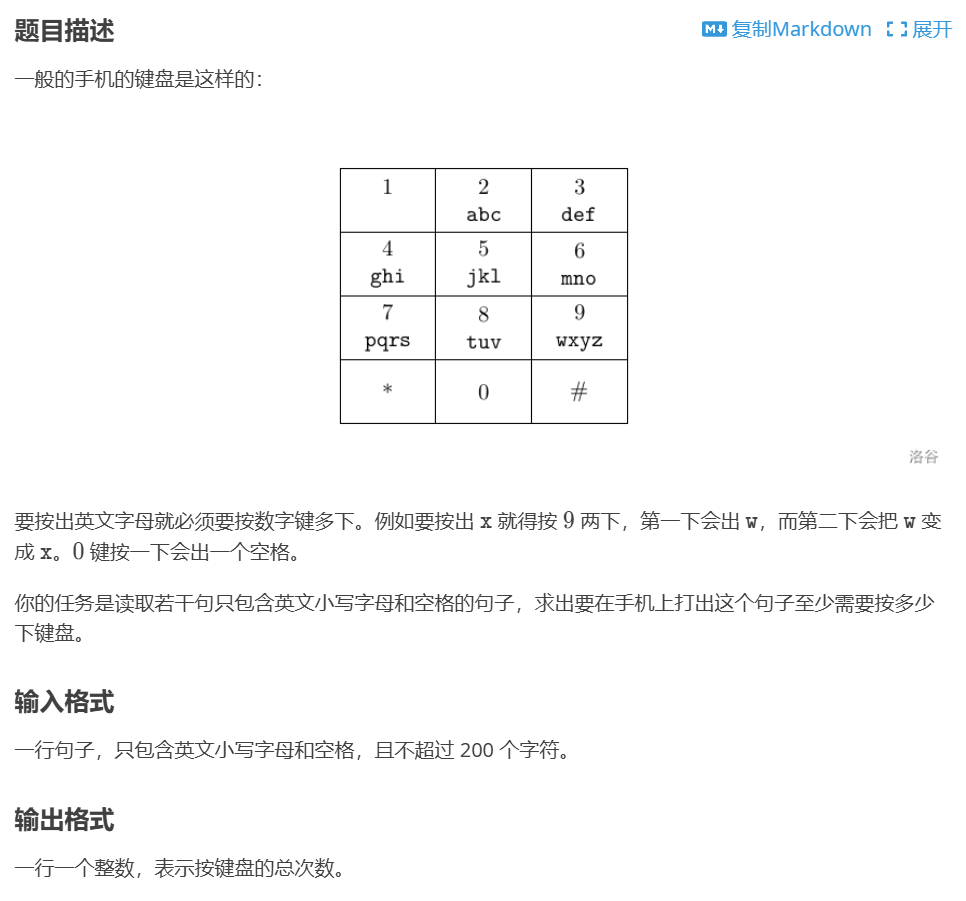
cpp
#include <iostream>
#include <string>
using namespace std;
int count[26] = {1,2,3, 1,2,3, 1,2,3, 1,2,3, 1,2,3, 1,2,3,4, 1,2,3, 1,2,3,4};
int main()
{
string s;
int sum = 0;
getline(cin , s);
for(auto c: s)
{
if(c == ' ')
sum += 1;
else
sum += count[c - 'a'];
}
cout << sum << endl;
return 0;
}
练习9:口算练习题
cpp
#include <iostream>
using namespace std;
#include <string>
int main()
{
int i = 0;
string op;
string last;//记录上一次的运算方式
cin >> i;
while(i--)
{
string ans;
int n1, n2;
int r;//结果
cin >> op;
if(op == "a" || op == "b" || op == "c") //有三个数据
{
cin >> n1 >> n2;
ans += to_string(n1);
if(op == "a")
{
r = n1 + n2;
ans += "+";
ans += to_string(n2);
ans += "=";
ans += to_string(r);
}
else if(op == "b")
{
r = n1 - n2;
ans += "-";
ans += to_string(n2);
ans += "=";
ans += to_string(r);
}
else
{
r = n1 * n2;
ans += "*";
ans += to_string(n2);
ans += "=";
ans += to_string(r);
}
last = op;
}
else //有两个数字
{
ans += op;
n1 = stoi(op);
cin >> n2;
if(last == "a")
{
r = n1 + n2;
ans += "+";
ans += to_string(n2);
ans += "=";
ans += to_string(r);
}
else if(last == "b")
{
r = n1 - n2;
ans += "-";
ans += to_string(n2);
ans += "=";
ans += to_string(r);
}
else
{
r = n1 * n2;
ans += "*";
ans += to_string(n2);
ans += "=";
ans += to_string(r);
}
}
cout << ans << endl;
cout << ans.size() << endl;
}
return 0;
}