本文讲述:WPF 进度条(ProgressBar)简单的样式修改和使用。
进度显示界面:使用UserControl把ProgressBar和进度值以及要显示的内容全部组装在UserControl界面中,方便其他界面直接进行使用。
XML
<UserControl x:Class="DefProcessBarDemo.DefProcessBar"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:local="clr-namespace:DefProcessBarDemo"
mc:Ignorable="d"
x:Name="MyWatingViewControl">
<UserControl.Background>
<VisualBrush>
<VisualBrush.Visual>
<Border x:Name="ControlBackground"
Background="Black"
Opacity="0.45" />
</VisualBrush.Visual>
</VisualBrush>
</UserControl.Background>
<Viewbox x:Name="myViewBox"
Stretch="UniformToFill"
StretchDirection="DownOnly"
UseLayoutRounding="True">
<Grid Margin="0 0 0 0"
HorizontalAlignment="Center"
VerticalAlignment="Center"
MouseDown="Image_MouseDown">
<Border CornerRadius="5"
SnapsToDevicePixels="True">
<Border.Effect>
<DropShadowEffect Color="#000000"
BlurRadius="10"
ShadowDepth="3"
Opacity="0.35"
Direction="270" />
</Border.Effect>
<Border Background="#4a4a4a"
CornerRadius="5"
Margin="5"
BorderBrush="#9196a0"
BorderThickness="1"
SnapsToDevicePixels="True">
<Grid Width="500"
Height="150">
<Grid.RowDefinitions>
<RowDefinition Height="auto" />
<RowDefinition Height="35" />
<RowDefinition Height="*" />
<RowDefinition Height="30" />
</Grid.RowDefinitions>
<Image Name="CloseIco"
Width="25"
Height="25"
Margin="0,0,0,0"
MouseDown="Image_MouseDown"
HorizontalAlignment="Right"
VerticalAlignment="Top" />
<StackPanel Grid.Row="1"
Orientation="Horizontal"
HorizontalAlignment="Center">
<TextBlock Text="{Binding Message,ElementName=MyWatingViewControl}"
FontSize="18"
Foreground="Yellow"
TextWrapping="WrapWithOverflow"
TextTrimming="CharacterEllipsis"
MaxWidth="450"
VerticalAlignment="Bottom" />
<TextBlock Text="("
FontSize="18"
Foreground="Yellow"
VerticalAlignment="Bottom" />
<TextBlock Text="{Binding ElementName=progressBar, Path=Value, StringFormat={}{0:0}%}"
FontSize="18"
Foreground="Yellow"
FontFamily="楷体"
VerticalAlignment="Bottom" />
<TextBlock Text=")"
FontSize="18"
Foreground="Yellow"
VerticalAlignment="Bottom" />
</StackPanel>
<Grid Grid.Row="2"
HorizontalAlignment="Center"
VerticalAlignment="Top"
Margin="0 10">
<ProgressBar x:Name="progressBar"
Maximum="100"
Height="25"
Width="420"
Foreground="Green"
Background="LightGray"
HorizontalContentAlignment="Center"
VerticalContentAlignment="Center"
Value="{Binding ProcessBarValue,ElementName=MyWatingViewControl}" />
</Grid>
</Grid>
</Border>
</Border>
</Grid>
</Viewbox>
</UserControl>
进度显示界面:UserControl 后台逻辑实现,主要定义了进度值、显示的文本、以及UserControl的大小。
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace DefProcessBarDemo
{
/// <summary>
/// DefProcessBar.xaml 的交互逻辑
/// </summary>
public partial class DefProcessBar : UserControl
{
public DefProcessBar()
{
InitializeComponent();
this.Loaded += WaitingView_Loaded;
}
void WaitingView_Loaded(object sender, RoutedEventArgs e)
{
if (this.Parent != null)
{
var root = (FrameworkElement)this.Parent;
if (root != null)
{
this.Width = root.ActualWidth;
this.Height = root.ActualHeight;
ControlBackground.Width = root.ActualWidth;
ControlBackground.Height = root.ActualHeight;
}
}
}
#region Property
public string Message
{
get { return (string)GetValue(MessageProperty); }
set { SetValue(MessageProperty, value); }
}
public static readonly DependencyProperty MessageProperty = DependencyProperty.Register("Message", typeof(string), typeof(DefProcessBar),
new PropertyMetadata(""));
public double ProcessBarValue
{
get { return (double)GetValue(ProcessBarValueProperty); }
set { SetValue(ProcessBarValueProperty, value); }
}
public static readonly DependencyProperty ProcessBarValueProperty = DependencyProperty.Register("ProcessBarValue", typeof(double), typeof(DefProcessBar),
new PropertyMetadata(0.0));
#endregion
private void Image_MouseDown(object sender, MouseButtonEventArgs e)
{
this.Visibility = Visibility.Hidden;
}
}
}
在MainWindow界面中调用[进度显示界面],示例如下:
XML
<Window x:Class="DefProcessBarDemo.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:DefProcessBarDemo"
mc:Ignorable="d" Title="DefProcessBar" Width="600" Height="500"
WindowStartupLocation="CenterScreen" x:Name="mainwnd"
xmlns:pdb="clr-namespace:DefProcessBarDemo" Background="Teal"
>
<StackPanel>
<Button Height="30" Width="120" Margin="20" Content="点击" Click="Button_Click"/>
<pdb:DefProcessBar VerticalAlignment="Center"
ProcessBarValue="{Binding ExportValue, Mode=TwoWay,UpdateSourceTrigger=PropertyChanged}"
Message="{Binding ExportMessage, Mode=TwoWay, UpdateSourceTrigger=PropertyChanged}" />
</StackPanel>
</Window>
后台模拟进度变化,使用Task任务,更新进度值,代码示例如下:
cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Diagnostics;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace DefProcessBarDemo
{
/// <summary>
/// MainWindow.xaml 的交互逻辑
/// </summary>
public partial class MainWindow : Window, System.ComponentModel.INotifyPropertyChanged
{
public MainWindow()
{
InitializeComponent();
this.DataContext = this;
}
private string m_ExportMessage = "正在导出,请稍后....";
/// <summary>
///
/// <summary>
public string ExportMessage
{
get { return m_ExportMessage; }
set
{
m_ExportMessage = value;
OnPropertyChanged("ExportMessage");
}
}
private double m_ExportValue = 0.0;
/// <summary>
///
/// <summary>
public double ExportValue
{
get { return m_ExportValue; }
set
{
m_ExportValue = value;
OnPropertyChanged("ExportValue");
}
}
#region MyRegion
public event System.ComponentModel.PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged(string propertyName)
{
if (PropertyChanged != null)
{
PropertyChanged(this, new System.ComponentModel.PropertyChangedEventArgs(propertyName));
}
}
#endregion
private void Button_Click(object sender, RoutedEventArgs e)
{
Task.Run(() =>
{
for(int i = 1; i < 101; i++)
{
ExportValue++;
System.Threading.Thread.Sleep(1000);
if (ExportValue == 100)
ExportMessage = "完成";
}
});
string strRes = "";
bool bRet = GetCmdResult("netsh wlan show profiles", out strRes);
}
}
}
运行时,点击【点击】按钮,即可看到进度持续不断地更新,界面如下图所示:
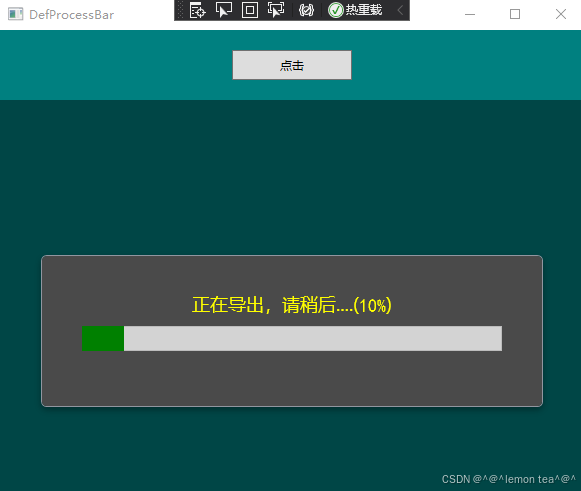