【高心星出品】
文章目录
NodeContainer自定义占位节点
NodeContainer是用来占位的系统组件,主要用于自定义节点以及自定义节点树的显示,支持组件的通用属性,对通用属性的处理请参考默认左上角对齐的Stack组件。
NodeController提供了一系列生命周期回调,通过makeNode回调返回一个 FrameNode 节点树的根节点。将FrameNode节点树挂载到对应的NodeContainer下。同时提供了aboutToAppear、aboutToDisappear、aboutToResize、onTouchEvent、rebuild五个回调方法用于监听对应的NodeContainer的状态。
在这里如果使用NodeContainer可以有两种使用方式:
nodecontainer-->nodectroller-->framnode--->typenode
nodecontainer-->nodectroller-->framenode-->buildernode-->builder构建函数
案例
下面我们编写一个案例,用typenode生成布局和用builder构建函数生成布局两种方式来创建framnode,并通过nodecontroller挂在到nodecontainer中。
运行结果
运行过程中"布局文本"是build函数中的组件,在其下面有builder构建函数生成的节点也有通过typenode生成的几点,我们通过按钮点击来切换。
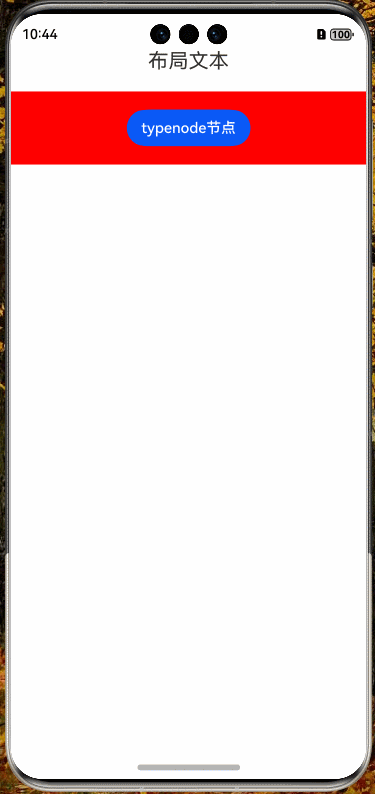
开发步骤
全局构建函数
interface param{
text1:string
text2:string
}
// 全局构建函数
@Builder
function buildcontainer(p:param){
Column({space:10}){
Text(p.text1).fontSize(22)
Text(p.text2).fontSize(22)
}.width('100%')
.padding(10)
}
typenode生成函数
//使用typenode生成framenode
function gentypenode(uicontext:UIContext){
let row=typeNode.createNode(uicontext,'Row')
row.initialize()
row.attribute.width('100%')
row.attribute.padding(20)
row.attribute.backgroundColor(Color.Red)
row.attribute.justifyContent(FlexAlign.Center)
let button=typeNode.createNode(uicontext,'Button')
button.initialize('typenode节点')
row.appendChild(button)
return row
}
nodecontroller对象加载framenode
在这里面根据type选择挂载builder构建函数还是typenode,根据isshow决定是否显示布局。
rebuild方法会引起nodectroller重新调用makeNode方法。
class mynodectl extends NodeController{
node:BuilderNode<[param]>|null=null
private isshow:boolean=true
// 根据不同的type决定是typenode或者是构建函数
private _type: string = 'builder'
public settype(value: string) {
this._type = value
}
constructor(type: string) {
super()
this._type = type
}
toshow(){
this.isshow=true
// 重新构建节点 重新调用makenode方法
this.rebuild()
}
tohide(){
this.isshow=false
// 重新构建节点 重新调用makenode方法
this.rebuild()
}
makeNode(uiContext: UIContext): FrameNode | null {
if (!this.isshow){
return null
}
if(this.node==null)
{
// 将build构建函数转化成节点
if(this._type=='builder') {
this.node = new BuilderNode(uiContext)
this.node.build(wrapBuilder<[param]>(buildcontainer), { text1: 'builder文本节点1', text2: 'builder文本节点2' })
}else{
return gentypenode(uiContext)
}
}
return this.node.getFrameNode()
}
}
页面代码
这里面通过调用tohide或者toshow来调控布局的显示,通过settype来调控显示的布局内容是来自builder还是typenode。
@Entry
@Component
struct Nodecontainerpage {
@State message: string = 'Hello World';
// 定义节点对象
nodelayout:mynodectl=new mynodectl('type')
build() {
Column({space:20}){
Text('布局文本').fontSize(22)
.onClick(()=>{
this.nodelayout.settype('builder')
this.nodelayout.toshow()
})
//nodecontainer-->nodectroller-->framnode--->typenode
//nodecontainer-->nodectroller-->framenode-->buildernode-->builder构建函数
NodeContainer(this.nodelayout)
.onClick(()=>{
this.nodelayout.tohide()
})
}
.height('100%')
.width('100%')
}
}
全部代码
import { BuilderNode, NodeController, typeNode, UIContext } from '@kit.ArkUI'
interface param{
text1:string
text2:string
}
// 全局构建函数
@Builder
function buildcontainer(p:param){
Column({space:10}){
Text(p.text1).fontSize(22)
Text(p.text2).fontSize(22)
}.width('100%')
.padding(10)
}
//使用typenode生成framenode
function gentypenode(uicontext:UIContext){
let row=typeNode.createNode(uicontext,'Row')
row.initialize()
row.attribute.width('100%')
row.attribute.padding(20)
row.attribute.backgroundColor(Color.Red)
row.attribute.justifyContent(FlexAlign.Center)
let button=typeNode.createNode(uicontext,'Button')
button.initialize('typenode节点')
row.appendChild(button)
return row
}
class mynodectl extends NodeController{
node:BuilderNode<[param]>|null=null
private isshow:boolean=true
// 根据不同的type决定是typenode或者是构建函数
private _type: string = 'builder'
public settype(value: string) {
this._type = value
}
constructor(type: string) {
super()
this._type = type
}
toshow(){
this.isshow=true
// 重新构建节点 重新调用makenode方法
this.rebuild()
}
tohide(){
this.isshow=false
// 重新构建节点 重新调用makenode方法
this.rebuild()
}
makeNode(uiContext: UIContext): FrameNode | null {
if (!this.isshow){
return null
}
if(this.node==null)
{
// 将build构建函数转化成节点
if(this._type=='builder') {
this.node = new BuilderNode(uiContext)
this.node.build(wrapBuilder<[param]>(buildcontainer), { text1: 'builder文本节点1', text2: 'builder文本节点2' })
}else{
return gentypenode(uiContext)
}
}
return this.node.getFrameNode()
}
}
@Entry
@Component
struct Nodecontainerpage {
@State message: string = 'Hello World';
// 定义节点对象
nodelayout:mynodectl=new mynodectl('type')
build() {
Column({space:20}){
Text('布局文本').fontSize(22)
.onClick(()=>{
this.nodelayout.settype('builder')
this.nodelayout.toshow()
})
//nodecontainer-->nodectroller-->framnode--->typenode
//nodecontainer-->nodectroller-->framenode-->buildernode-->builder构建函数
NodeContainer(this.nodelayout)
.onClick(()=>{
this.nodelayout.tohide()
})
}
.height('100%')
.width('100%')
}
}
de
//nodecontainer-->nodectroller-->framenode-->buildernode-->builder构建函数
NodeContainer(this.nodelayout)
.onClick(()=>{
this.nodelayout.tohide()
})
}
.height('100%')
.width('100%')
}
}