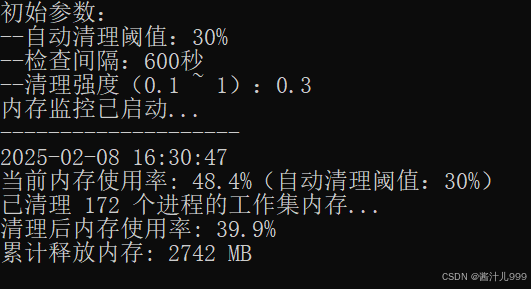
python
import ctypes
import psutil
import time
import sys
import os
from datetime import datetime
import pyautogui
# 检查管理员权限
def is_admin():
try:
return ctypes.windll.shell32.IsUserAnAdmin()
except:
return False
# 内存清理核心功能
def cleanup_memory(aggressiveness=0.5):
try:
# 获取所有进程
processes = psutil.process_iter()
# 系统关键进程白名单
system_processes = {
"System Idle Process", "System", "svchost.exe",
"wininit.exe", "csrss.exe", "lsass.exe"
}
# 初始化WinAPI
PROCESS_QUERY_INFORMATION = 0x0400
PROCESS_SET_QUOTA = 0x0100
# 加载 psapi.dll
psapi = ctypes.WinDLL('psapi', use_last_error=True)
EmptyWorkingSet = psapi.EmptyWorkingSet
EmptyWorkingSet.argtypes = [ctypes.c_void_p]
cleaned = 0
for proc in processes:
try:
# 获取进程信息
pid = proc.pid
name = proc.name()
# 跳过系统关键进程和自身
if name in system_processes or pid == os.getpid():
continue
# 尝试获取进程句柄
hProcess = ctypes.windll.kernel32.OpenProcess(
PROCESS_QUERY_INFORMATION | PROCESS_SET_QUOTA,
False,
pid
)
if hProcess:
# 尝试清空工作集
if EmptyWorkingSet(hProcess):
cleaned += 1
ctypes.windll.kernel32.CloseHandle(hProcess)
except (psutil.NoSuchProcess, psutil.AccessDenied, psutil.ZombieProcess):
continue
return cleaned
except Exception as e:
print(f"清理过程中发生错误: {str(e)}")
return 0
# 内存监控
def memory_monitor(threshold=30, interval=60, aggressiveness=0.5):
print("内存监控已启动...")
try:
while True:
mem = psutil.virtual_memory()
used_percent = mem.percent
print(
f"--------------------\n{datetime.now().strftime('%Y-%m-%d %H:%M:%S')} \n当前内存使用率: {used_percent}%(自动清理阈值:{threshold}%)")
if used_percent > threshold:
# print("开始清理内存...")
cleaned = cleanup_memory(aggressiveness)
print(f"已清理 {cleaned} 个进程的工作集内存...")
# 清理后再次检查
time.sleep(5) # 预留清理过程的时间
new_mem = psutil.virtual_memory()
print(f"清理后内存使用率: {new_mem.percent}%")
print(f"累计释放内存: {(mem.used - new_mem.used) // 1024 // 1024} MB")
time.sleep(interval)
except KeyboardInterrupt:
print("\n监控已停止")
if __name__ == "__main__":
if not is_admin():
print("需要管理员权限运行!")
ctypes.windll.shell32.ShellExecuteW(
None, "runas", sys.executable, " ".join(sys.argv), None, 1)
sys.exit()
# 配置参数
config = {
'threshold': 30, # 触发清理的内存使用率阈值(百分比)
'interval': 600, # 检查间隔(秒)
'aggressiveness': 0.3 # 清理强度(0.1 ~ 1)
}
# 内存使用率阈值(百分比)
try:
config['threshold'] = int(pyautogui.prompt('请输入触发清理的内存使用率阈值-(整数)-(默认30%)!'))
except:
config['threshold'] = 30
print(f"初始参数:\n--自动清理阈值:{config['threshold']}%")
# 检查间隔(秒)
try:
config['interval'] = int(pyautogui.prompt('请设置检查间隔(秒)-(整数)-(默认600秒)!'))
except:
config['interval'] = 600
print(f"--检查间隔:{config['interval']}秒")
# 清理强度(0.1~1.0)
try:
config['aggressiveness'] = float(pyautogui.prompt('请设置清理强度(0.1 ~ 1)-(默认0.3)!'))
except:
config['aggressiveness'] = 0.3
print(f"--清理强度(0.1 ~ 1):{config['aggressiveness']}")
# 执行内存监控
try:
memory_monitor(**config)
except Exception as e:
print(f"程序发生错误: {str(e)}")