cout的格式输出
printf 函数在输出数据的时候,可以指定格式来输出,⽐如:指定宽度,⽐如指定⼩数点后的位
数,对⻬⽅式等等。cout 结合<iomanip>
(IO manipulators)头⽂件中的操纵符,可以灵活控制输出格式,从⽽满⾜各种格式化需求。
控制宽度和填充
- setw :设置字段宽度(只对紧接着的输出项有效)。
- setfill :设置填充字符。
c++
#include <iostream>
using namespace std;
#include <iomanip>
int main()
{
int a = 123;
cout << "默认宽度: " << a << endl;
cout << "宽度设置为10: " << setw(10) << a << endl;
cout << "宽度为10,不够时填充*: " << setw(10) << setfill('*') << a << endl;
return 0;
}

控制数值格式
- fixed :以固定⼩数点表⽰浮点数,设置后就不会以科学计数法展⽰了。
- scientific :以科学计数法表⽰浮点数。
- setprecision :设置浮点数的精度,以控制⼩数点后的数字位数,⼀般先固定⼩数点,再设置精度。
c++
#include <iostream>
using namespace std;
#include <iomanip>
int main()
{
double pi = 3.141592653589793;
cout << "默认: " << pi << endl;
cout << "固定⼩时点⽅式: " << fixed << pi << endl;
cout << "科学计数法⽅式: " << scientific << pi << endl;
cout << "固定⼩数点,⼩数点后2位有效数字: " << fixed << setprecision(2) << pi << endl;
return 0;
}
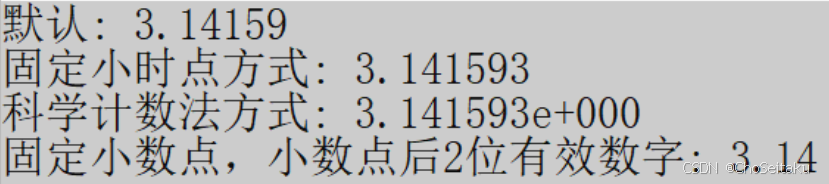
控制整数格式
- dec :以⼗进制格式显⽰整数(默认)。
- hex :以⼗六进制格式显⽰整数。
- oct :以⼋进制格式显⽰整数。
c++
#include <iostream>
using namespace std;
#include <iomanip>
int main()
{
int n = 255;
cout << "⼗进制 : " << dec << n << endl;
cout << "⼗六进制: " << hex << n << endl;
cout << "⼋进制 : " << oct << n << endl;
return 0;
}
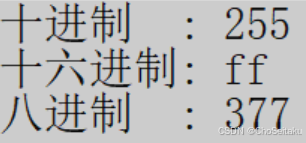
控制对⻬⽅式
- left :左对⻬。
- right :右对⻬(默认)。
c++
#include <iostream>
using namespace std;
#include <iomanip>
int main()
{
int n = 123;
cout << "右对⻬: " << setw(10) << right << n << endl;
cout << "左对⻬: " << setw(10) << left << n << endl;
return 0;
}
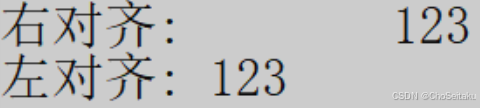
题目
P5705 【深基2.例7】数字反转
c++
#include <iostream>
using namespace std;
int main()
{
char a, b, c, d, e;
cin >> a >> b >> c >> d >> e;
cout << e << d << c << b << a << endl;
return 0;
}
c++
#include <cstdio>
int main()
{
char a, b, c, d;
scanf("%c%c%c.%c", &a, &b, &c, &d);
printf("%c.%c%c%c\n", d, c, b, a);
return 0;
}
从题⽬的中可以看出,想要输⼊⼩数,然后反转输出,如果真按照这样的思路往下⾛,也能解决问题,但是可能就复杂了。其实在不管什么类型的数据都是以字符流的形式输⼊和输出的,那我们就可以把输⼊的这个⼩数和⼩数点,都当做字符依次读取,然后再按照想要的顺序输出就⾏。
如果使⽤ scanf 和 printf 函数要指定好占位符,如果使⽤ cin/cout 要指定好变量的类型。
P5708 【深基2.习2】三角形面积
c++
#include <iostream>
#include <cmath>
#include <cstdio>
using namespace std;
double a, b, c;
int main()
{
cin >> a >> b >> c;
double p = (a + b + c) / 2;
double s = sqrt(p * (p - a) * (p - b) * (p - c));
printf("%.1f\n", s);
return 0;
}