在 Android 中,RelativeLayout
是一种布局,它允许你根据其他视图的位置来相对地定位视图。
以下面的代码为例:
案例一:
android:layout_alignParentTop="true"
: 将视图的顶部对齐到父容器的顶部。
android:layout_centerHorizontal="true"
: 将视图水平居中。
文本视图中的**android:layout_below="@id/button1"
** : 将视图放置在另一个视图(在此例中是 Button
)的下方。
XML
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<!-- 按钮,顶部对齐父容器,并水平居中 -->
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 1"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true" />
<!-- 文本视图,位于按钮下方,水平居中 -->
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, World!"
android:layout_below="@id/button1"
android:layout_centerHorizontal="true"
android:layout_marginTop="20dp" />
android:layout_alignParentBottom="true"
: 将视图的底部对齐父容器的底部。
android:layout_alignParentRight="true"
: 将视图的右侧对齐父容器的右侧。
android:layout_marginBottom="50dp"
和 android:layout_marginRight="50dp"
: 为视图的底部和右侧添加间距。
XML
<!-- 一个按钮,位于图片视图的右侧,并且对齐父容器的底部 -->
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 2"
android:layout_alignParentBottom="true"
android:layout_alignParentRight="true"
android:layout_marginBottom="50dp"
android:layout_marginRight="50dp" />
android:layout_toLeftOf="@id/imageView1"
: 将视图放置在指定视图(imageView1
)的左侧。android:layout_alignTop="@id/imageView1"
: 将视图与指定视图(imageView1
)的顶部对齐。
XML
<!-- 一个文本视图,位于图片视图的左侧 -->
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Another Text"
android:layout_toLeftOf="@id/imageView1"
android:layout_alignTop="@id/imageView1"
android:layout_marginRight="10dp" />
<!-- 一个按钮,位于文本视图的下方 -->
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 3"
android:layout_below="@id/textView2"
android:layout_centerHorizontal="true"
android:layout_marginTop="15dp" />
总体效果图如下:
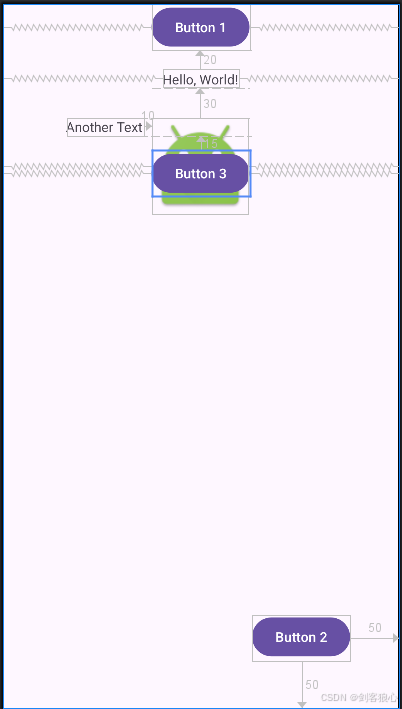
再看一个案例:
案例二:
先给出效果图
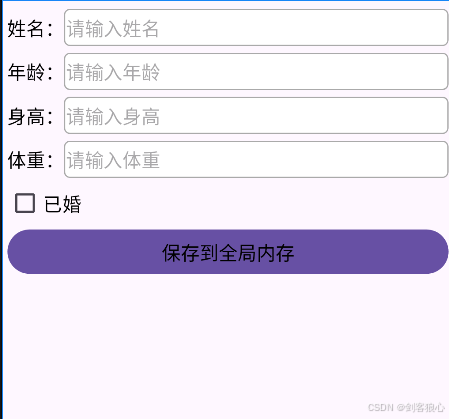
完整代码如下:
线性布局作为根,垂直方向排列,android:padding="5dp"设置了内边距,也就是视图内容上下左右各压缩了5dp的显示区域。
线性布局内是5个相对布局组件,gravity="left|center"
具体含义是:内容会水平靠左,同时垂直居中。通过5个相对布局组件,可以实现内容的自由排列。
XML
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="5dp" >
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="40dp" >
<TextView
android:id="@+id/tv_name"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:gravity="center"
android:text="姓名:"
android:textColor="@color/black"
android:textSize="17sp" />
<EditText
android:id="@+id/et_name"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginBottom="3dp"
android:layout_marginTop="3dp"
android:layout_toRightOf="@+id/tv_name"
android:background="@drawable/editext_selector"
android:gravity="left|center"
android:hint="请输入姓名"
android:inputType="text"
android:maxLength="12"
android:textColor="@color/black"
android:textSize="17sp" />
</RelativeLayout>
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="40dp" >
<TextView
android:id="@+id/tv_age"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:gravity="center"
android:text="年龄:"
android:textColor="@color/black"
android:textSize="17sp" />
<EditText
android:id="@+id/et_age"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginBottom="3dp"
android:layout_marginTop="3dp"
android:layout_toRightOf="@+id/tv_age"
android:background="@drawable/editext_selector"
android:gravity="left|center"
android:hint="请输入年龄"
android:inputType="number"
android:maxLength="2"
android:textColor="@color/black"
android:textSize="17sp" />
</RelativeLayout>
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="40dp" >
<TextView
android:id="@+id/tv_height"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:gravity="center"
android:text="身高:"
android:textColor="@color/black"
android:textSize="17sp" />
<EditText
android:id="@+id/et_height"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginBottom="3dp"
android:layout_marginTop="3dp"
android:layout_toRightOf="@+id/tv_height"
android:background="@drawable/editext_selector"
android:gravity="left|center"
android:hint="请输入身高"
android:inputType="number"
android:maxLength="3"
android:textColor="@color/black"
android:textSize="17sp" />
</RelativeLayout>
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="40dp" >
<TextView
android:id="@+id/tv_weight"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:gravity="center"
android:text="体重:"
android:textColor="@color/black"
android:textSize="17sp" />
<EditText
android:id="@+id/et_weight"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginBottom="3dp"
android:layout_marginTop="3dp"
android:layout_toRightOf="@+id/tv_weight"
android:background="@drawable/editext_selector"
android:gravity="left|center"
android:hint="请输入体重"
android:inputType="numberDecimal"
android:maxLength="5"
android:textColor="@color/black"
android:textSize="17sp" />
</RelativeLayout>
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="40dp" >
<CheckBox
android:id="@+id/ck_married"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:gravity="center"
android:checked="false"
android:text="已婚"
android:textColor="@color/black"
android:textSize="17sp" />
</RelativeLayout>
<Button
android:id="@+id/btn_save"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="保存到全局内存"
android:textColor="@color/black"
android:textSize="17sp" />
</LinearLayout>