1. 系统概述
此订货系统主要面向有中英文双语需求的企业,旨在方便国内外客户进行商品订购。系统涵盖商品展示、订单管理、用户管理等核心功能,支持中英文界面自由切换,为用户提供良好的交互体验。
2. 功能模块设计
用户管理模块
- 用户注册与登录:用户可使用邮箱或手机号注册账号,并通过账号密码登录系统。
- 用户信息管理:用户能够修改个人信息,如联系方式、收货地址等。
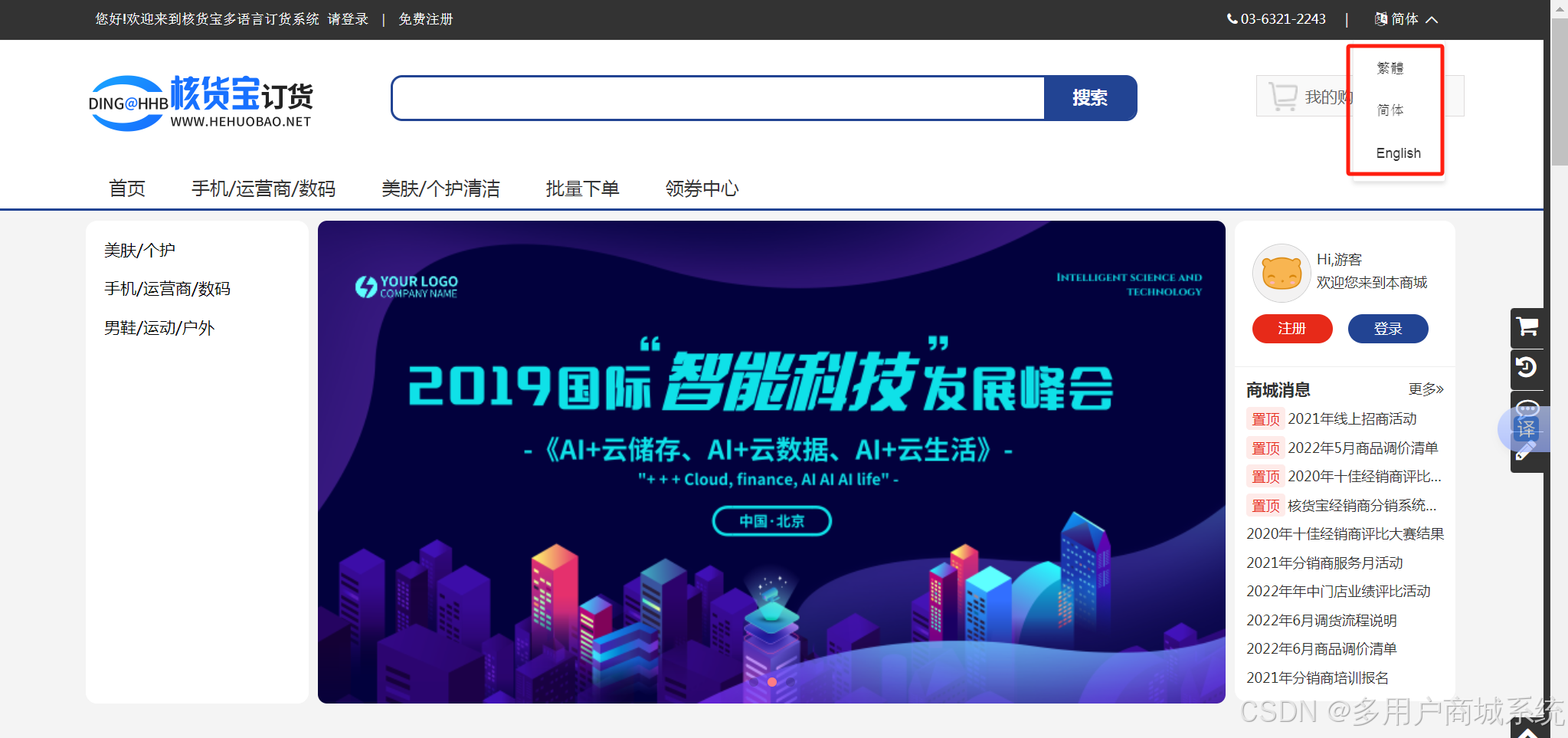
商品管理模块
- 商品添加与编辑:管理员可添加新商品,包括商品名称、价格、库存、描述等信息,也能对已有商品信息进行修改。
- 商品分类管理:对商品进行分类,方便用户查找,如按品类、用途等分类。
订货模块
- 商品浏览与选择:用户可以浏览商品列表,查看商品详情,选择要订购的商品及数量。
- 订单提交与确认:用户填写收货信息后提交订单,确认订单信息无误。
订单管理模块
- 订单查看与处理:管理员可查看所有订单信息,包括订单状态(待付款、已付款、已发货等),并进行相应处理。
- 订单搜索与筛选:支持按订单号、用户姓名、订单状态等条件搜索和筛选订单。
语言切换模块
- 界面语言切换:用户可随时在中英文界面之间进行切换,系统自动更新所有文本信息。
总体实现功能:
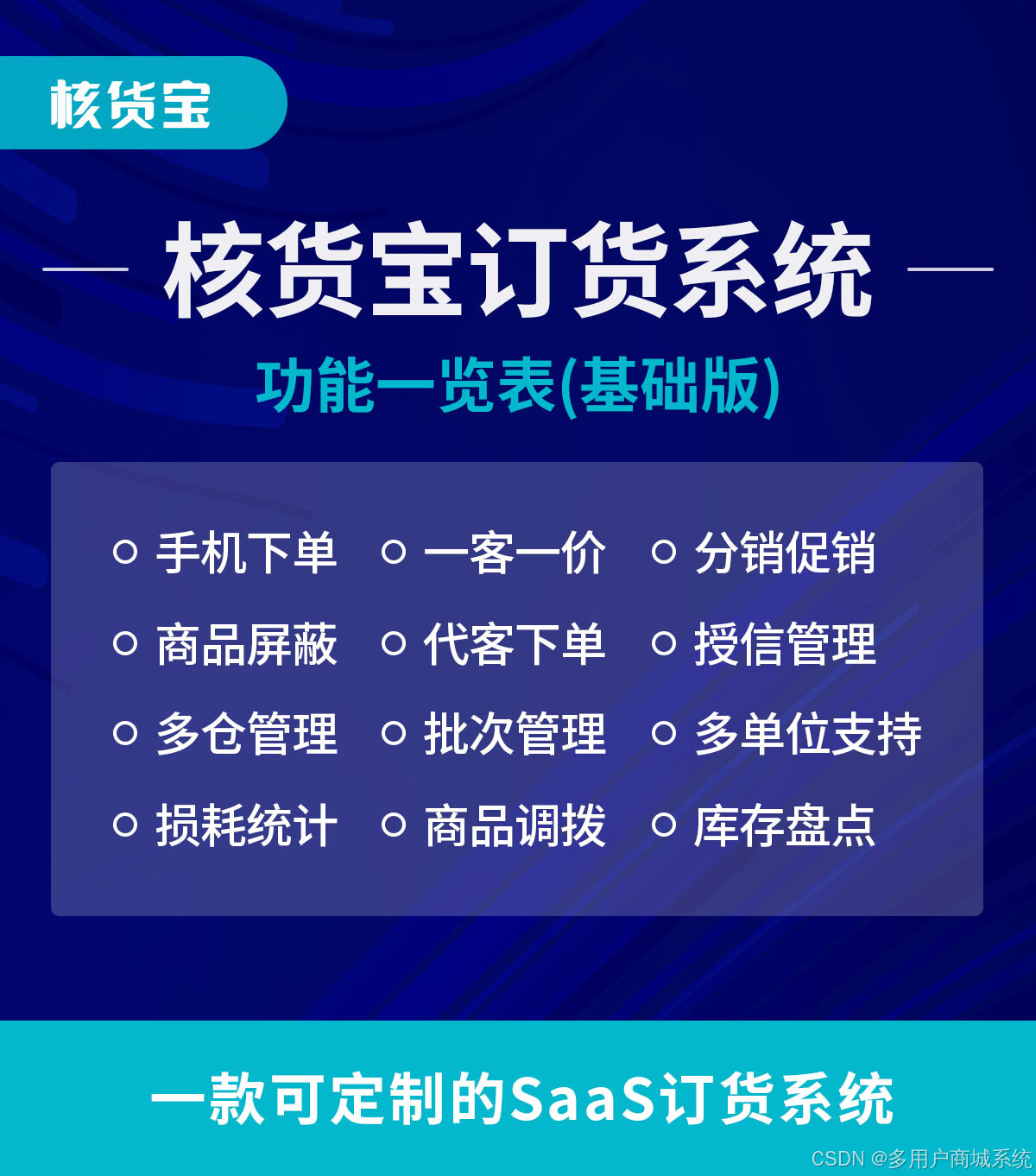
3. 数据库设计
用户表(users)
字段名 | 类型 | 描述 |
---|---|---|
id | int(11) | 用户 ID,主键 |
username | varchar(50) | 用户名 |
password | varchar(255) | 用户密码 |
varchar(100) | 用户邮箱 | |
phone | varchar(20) | 用户手机号 |
address | text | 用户收货地址 |
language | varchar(10) | 用户选择的语言 |
商品表(products)
字段名 | 类型 | 描述 |
---|---|---|
id | int(11) | 商品 ID,主键 |
name_en | varchar(255) | 商品英文名称 |
name_zh | varchar(255) | 商品中文名称 |
price | decimal(10, 2) | 商品价格 |
stock | int(11) | 商品库存 |
description_en | text | 商品英文描述 |
description_zh | text | 商品中文描述 |
category_id | int(11) | 商品分类 ID |
订单表(orders)
字段名 | 类型 | 描述 |
---|---|---|
id | int(11) | 订单 ID,主键 |
user_id | int(11) | 用户 ID,外键 |
product_id | int(11) | 商品 ID,外键 |
quantity | int(11) | 商品数量 |
status | varchar(20) | 订单状态 |
create_time | datetime | 订单创建时间 |
商品分类表(categories)
字段名 | 类型 | 描述 |
---|---|---|
id | int(11) | 分类 ID,主键 |
name_en | varchar(255) | 分类英文名称 |
name_zh | varchar(255) | 分类中文名称 |
源码分享
数据库连接文件:
<?php
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$dbname = "your_database";
// 创建连接
$conn = new mysqli($servername, $username, $password, $dbname);
// 检查连接
if ($conn->connect_error) {
die("Connection failed: ". $conn->connect_error);
}
?>
语言切换文件
<?php
session_start();
if (isset($_GET['lang'])) {
$_SESSION['lang'] = $_GET['lang'];
}
if (!isset($_SESSION['lang'])) {
$_SESSION['lang'] = 'en';
}
$lang = $_SESSION['lang'];
if ($lang == 'en') {
$text = [
'title' => 'Ordering System',
'login' => 'Login',
'register' => 'Register',
'product_name' => 'Product Name',
'price' => 'Price',
'quantity' => 'Quantity',
'order' => 'Order',
'my_orders' => 'My Orders'
];
} else {
$text = [
'title' => '订货系统',
'login' => '登录',
'register' => '注册',
'product_name' => '商品名称',
'price' => '价格',
'quantity' => '数量',
'order' => '订购',
'my_orders' => '我的订单'
];
}
?>
商品列表页面
<?php
include 'config.php';
include 'lang.php';
?>
<!DOCTYPE html>
<html lang="<?php echo $lang; ?>">
<head>
<meta charset="UTF-8">
<title><?php echo $text['title']; ?></title>
</head>
<body>
<h1><?php echo $text['title']; ?></h1>
<a href="?lang=<?php echo ($lang == 'en') ? 'zh' : 'en'; ?>"><?php echo ($lang == 'en') ? '中文' : 'English'; ?></a>
<table>
<thead>
<tr>
<th><?php echo $text['product_name']; ?></th>
<th><?php echo $text['price']; ?></th>
<th><?php echo $text['quantity']; ?></th>
<th><?php echo $text['order']; ?></th>
</tr>
</thead>
<tbody>
<?php
$sql = "SELECT id, name_{$lang}, price FROM products";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while ($row = $result->fetch_assoc()) {
echo "<tr>";
echo "<td>". $row["name_{$lang}"]. "</td>";
echo "<td>". $row["price"]. "</td>";
echo "<td><input type='number' value='1'></td>";
echo "<td><a href='order.php?product_id=". $row["id"]. "'>". $text['order']. "</a></td>";
echo "</tr>";
}
}
?>
</tbody>
</table>
</body>
</html>
订单页面
<?php
include 'config.php';
include 'lang.php';
if (isset($_GET['product_id'])) {
$product_id = $_GET['product_id'];
$sql = "SELECT id, name_{$lang}, price FROM products WHERE id = $product_id";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
$row = $result->fetch_assoc();
echo "<h1>". $text['order']. " - ". $row["name_{$lang}"]. "</h1>";
echo "<p>". $text['price']. ": ". $row["price"]. "</p>";
// 处理订单逻辑
}
}
?>