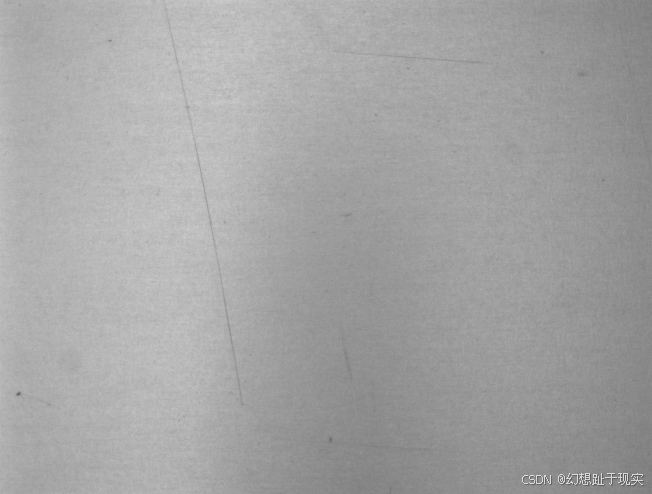
划痕检测,我这里用到的是Sobel算子和blob斑点匹配以及blob里面的形态学调整
Sobel 是一种在数字图像处理和计算机视觉领域广泛应用的算法,主要用于边缘检测
脚本展示
cs
#region namespace imports
using System;
using System.Collections;
using System.Drawing;
using System.IO;
using System.Windows.Forms;
using Cognex.VisionPro;
using Cognex.VisionPro.ToolBlock;
using Cognex.VisionPro3D;
using Cognex.VisionPro.ImageProcessing;
using Cognex.VisionPro.Blob;
#endregion
public class CogToolBlockAdvancedScript : CogToolBlockAdvancedScriptBase
{
#region Private Member Variables
private Cognex.VisionPro.ToolBlock.CogToolBlock mToolBlock;
#endregion
CogGraphicCollection dt = new CogGraphicCollection();
/// <summary>
/// Called when the parent tool is run.
/// Add code here to customize or replace the normal run behavior.
/// </summary>
/// <param name="message">Sets the Message in the tool's RunStatus.</param>
/// <param name="result">Sets the Result in the tool's RunStatus</param>
/// <returns>True if the tool should run normally,
/// False if GroupRun customizes run behavior</returns>
public override bool GroupRun(ref string message, ref CogToolResultConstants result)
{
// To let the execution stop in this script when a debugger is attached, uncomment the following lines.
// #if DEBUG
// if (System.Diagnostics.Debugger.IsAttached) System.Diagnostics.Debugger.Break();
// #endif
CogBlobTool blob = mToolBlock.Tools["CogBlobTool1"]as CogBlobTool;
// Run each tool using the RunTool function
foreach(ICogTool tool in mToolBlock.Tools)
mToolBlock.RunTool(tool, ref message, ref result);
for(int i = 0;i < blob.Results.GetBlobs().Count;i++)
{
double x = blob.Results.GetBlobs()[i].CenterOfMassX;
double y = blob.Results.GetBlobs()[i].CenterOfMassY;
dt.Add(Create(x, y));
}
return false;
}
private CogRectangleAffine Create(double x, double y)
{
CogRectangleAffine tt = new CogRectangleAffine();
tt.SideXLength = 8;
tt.SideYLength = 14;
tt.CenterX = x;
tt.CenterY = y;
tt.Color = CogColorConstants.Red;
tt.LineWidthInScreenPixels = 4;
return tt;
}
#region When the Current Run Record is Created
/// <summary>
/// Called when the current record may have changed and is being reconstructed
/// </summary>
/// <param name="currentRecord">
/// The new currentRecord is available to be initialized or customized.</param>
public override void ModifyCurrentRunRecord(Cognex.VisionPro.ICogRecord currentRecord)
{
}
#endregion
#region When the Last Run Record is Created
/// <summary>
/// Called when the last run record may have changed and is being reconstructed
/// </summary>
/// <param name="lastRecord">
/// The new last run record is available to be initialized or customized.</param>
public override void ModifyLastRunRecord(Cognex.VisionPro.ICogRecord lastRecord)
{
foreach(ICogGraphic s in dt)
{
mToolBlock.AddGraphicToRunRecord(s, lastRecord, "CogSobelEdgeTool1.InputImage", "script");
}
}
#endregion
#region When the Script is Initialized
/// <summary>
/// Perform any initialization required by your script here
/// </summary>
/// <param name="host">The host tool</param>
public override void Initialize(Cognex.VisionPro.ToolGroup.CogToolGroup host)
{
// DO NOT REMOVE - Call the base class implementation first - DO NOT REMOVE
base.Initialize(host);
// Store a local copy of the script host
this.mToolBlock = ((Cognex.VisionPro.ToolBlock.CogToolBlock)(host));
}
#endregion
}
效果
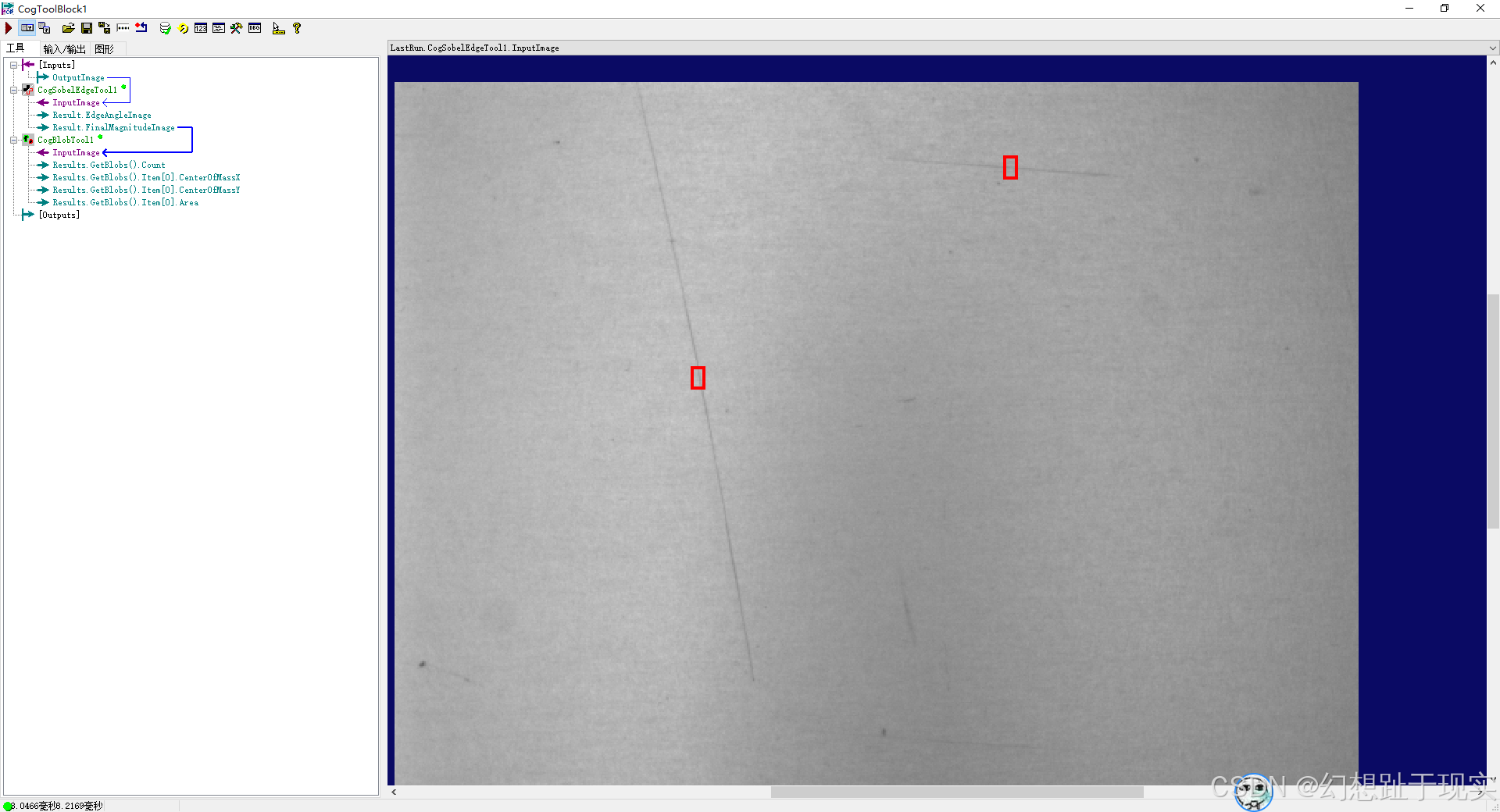
下面的我们先用Pixel 通过直方图调节来突出边缘和表面特征
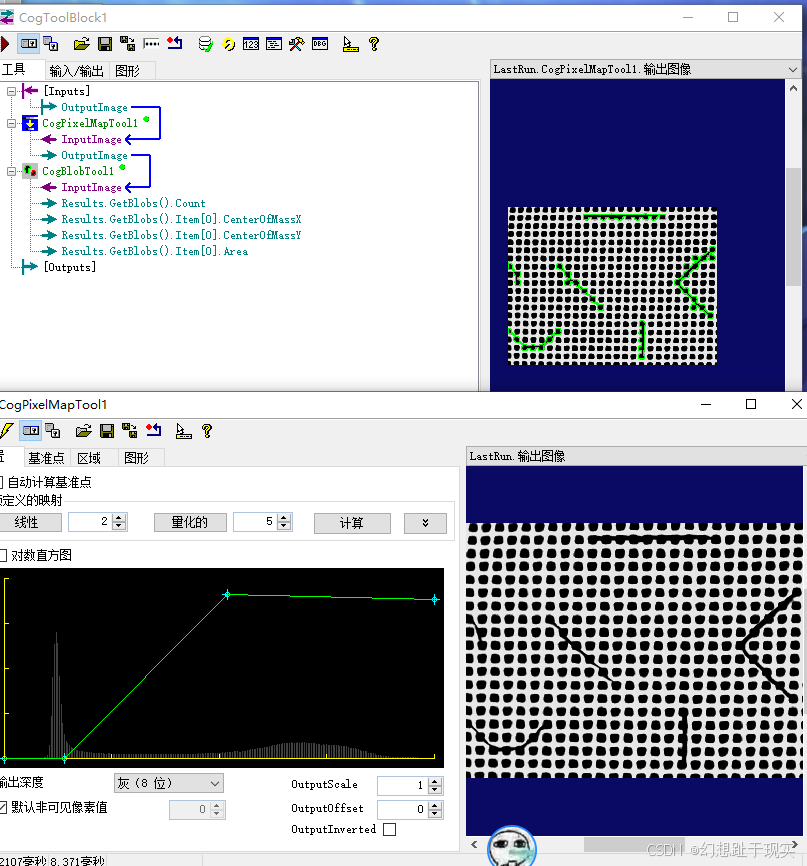
在通过调节二值化阈值等来突出
脚本
cs
#region namespace imports
using System;
using System.Collections;
using System.Drawing;
using System.IO;
using System.Windows.Forms;
using Cognex.VisionPro;
using Cognex.VisionPro.ToolBlock;
using Cognex.VisionPro3D;
using Cognex.VisionPro.PixelMap;
using Cognex.VisionPro.Blob;
#endregion
public class CogToolBlockAdvancedScript : CogToolBlockAdvancedScriptBase
{
#region Private Member Variables
private Cognex.VisionPro.ToolBlock.CogToolBlock mToolBlock;
#endregion
CogGraphicCollection dt = new CogGraphicCollection();
CogPolygon polygon;
/// <summary>
/// Called when the parent tool is run.
/// Add code here to customize or replace the normal run behavior.
/// </summary>
/// <param name="message">Sets the Message in the tool's RunStatus.</param>
/// <param name="result">Sets the Result in the tool's RunStatus</param>
/// <returns>True if the tool should run normally,
/// False if GroupRun customizes run behavior</returns>
public override bool GroupRun(ref string message, ref CogToolResultConstants result)
{
// To let the execution stop in this script when a debugger is attached, uncomment the following lines.
// #if DEBUG
// if (System.Diagnostics.Debugger.IsAttached) System.Diagnostics.Debugger.Break();
// #endif
dt.Clear();
CogBlobTool blob = mToolBlock.Tools["CogBlobTool1"]as CogBlobTool;
// Run each tool using the RunTool function
foreach(ICogTool tool in mToolBlock.Tools)
mToolBlock.RunTool(tool, ref message, ref result);
for(int i = 0;i < blob.Results.GetBlobs().Count;i++)
{
polygon = new CogPolygon();
polygon = blob.Results.GetBlobs()[i].GetBoundary();
polygon.Color = CogColorConstants.Red;
dt.Add(polygon);
}
return false;
}
#region When the Current Run Record is Created
/// <summary>
/// Called when the current record may have changed and is being reconstructed
/// </summary>
/// <param name="currentRecord">
/// The new currentRecord is available to be initialized or customized.</param>
public override void ModifyCurrentRunRecord(Cognex.VisionPro.ICogRecord currentRecord)
{
}
#endregion
#region When the Last Run Record is Created
/// <summary>
/// Called when the last run record may have changed and is being reconstructed
/// </summary>
/// <param name="lastRecord">
/// The new last run record is available to be initialized or customized.</param>
public override void ModifyLastRunRecord(Cognex.VisionPro.ICogRecord lastRecord)
{
foreach(ICogGraphic s in dt)
{
mToolBlock.AddGraphicToRunRecord(s, lastRecord, "CogPixelMapTool1.InputImage", "script");
}
}
#endregion
#region When the Script is Initialized
/// <summary>
/// Perform any initialization required by your script here
/// </summary>
/// <param name="host">The host tool</param>
public override void Initialize(Cognex.VisionPro.ToolGroup.CogToolGroup host)
{
// DO NOT REMOVE - Call the base class implementation first - DO NOT REMOVE
base.Initialize(host);
// Store a local copy of the script host
this.mToolBlock = ((Cognex.VisionPro.ToolBlock.CogToolBlock)(host));
}
#endregion
}
效果
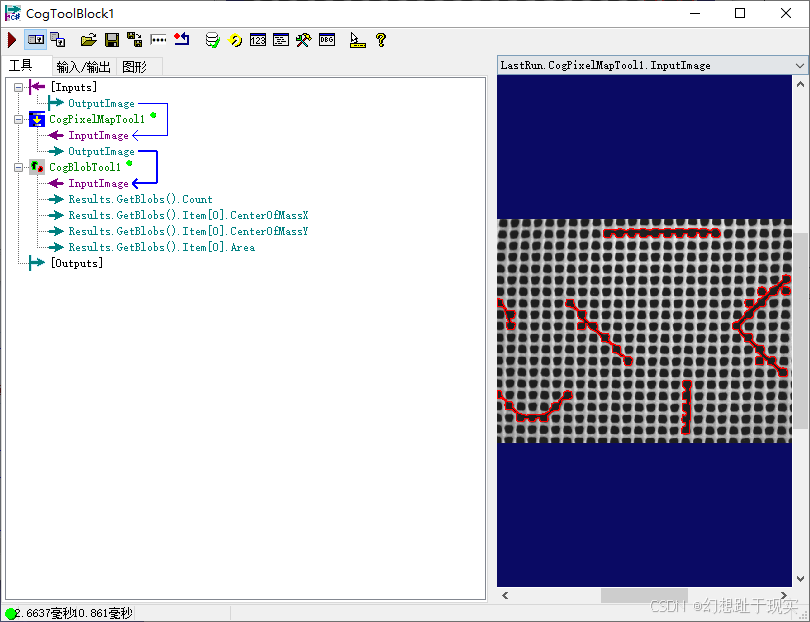
cs
#region namespace imports
using System;
using System.Collections;
using System.Drawing;
using System.IO;
using System.Windows.Forms;
using Cognex.VisionPro;
using Cognex.VisionPro.ToolBlock;
using Cognex.VisionPro3D;
using Cognex.VisionPro.ImageProcessing;
using Cognex.VisionPro.PMAlign;
using Cognex.VisionPro.CalibFix;
using Cognex.VisionPro.PixelMap;
using Cognex.VisionPro.Blob;
#endregion
public class CogToolBlockAdvancedScript : CogToolBlockAdvancedScriptBase
{
#region Private Member Variables
private Cognex.VisionPro.ToolBlock.CogToolBlock mToolBlock;
#endregion
CogGraphicCollection dt = new CogGraphicCollection();
CogGraphicLabel label = new CogGraphicLabel();
/// <summary>
/// Called when the parent tool is run.
/// Add code here to customize or replace the normal run behavior.
/// </summary>
/// <param name="message">Sets the Message in the tool's RunStatus.</param>
/// <param name="result">Sets the Result in the tool's RunStatus</param>
/// <returns>True if the tool should run normally,
/// False if GroupRun customizes run behavior</returns>
public override bool GroupRun(ref string message, ref CogToolResultConstants result)
{
// To let the execution stop in this script when a debugger is attached, uncomment the following lines.
// #if DEBUG
// if (System.Diagnostics.Debugger.IsAttached) System.Diagnostics.Debugger.Break();
// #endif
dt.Clear();
CogBlobTool blob = mToolBlock.Tools["CogBlobTool1"]as CogBlobTool;
CogPolarUnwrapTool polar = mToolBlock.Tools["CogPolarUnwrapTool1"]as CogPolarUnwrapTool;
// Run each tool using the RunTool function
foreach(ICogTool tool in mToolBlock.Tools)
mToolBlock.RunTool(tool, ref message, ref result);
for(int i = 0;i < blob.Results.GetBlobs().Count;i++)
{
double x = blob.Results.GetBlobs()[i].CenterOfMassX;
double y = blob.Results.GetBlobs()[i].CenterOfMassY;
double lastx,lasty;
polar.RunParams.GetInputPointFromOutputPoint(polar.InputImage, polar.Region, x, y, out lastx, out lasty);
dt.Add(CreateCircle(lastx, lasty));
}
if(blob.Results.GetBlobs().Count > 0)
{
label.SetXYText(100, 100, "NG");
label.Alignment = CogGraphicLabelAlignmentConstants.TopLeft;
label.Font = new Font("楷体", 20);
label.Color = CogColorConstants.Red;
dt.Add(label);
}else
{
label.SetXYText(100, 100, "Pass");
label.Alignment = CogGraphicLabelAlignmentConstants.TopLeft;
label.Font = new Font("楷体", 20);
label.Color = CogColorConstants.Green;
dt.Add(label);
}
return false;
}
private CogCircle CreateCircle(double x, double y)
{
CogCircle co = new CogCircle();
co.CenterX = x;
co.CenterY = y;
co.Radius = 30;
co.Color = CogColorConstants.Red;
co.LineWidthInScreenPixels = 6;
return co;
}
#region When the Current Run Record is Created
/// <summary>
/// Called when the current record may have changed and is being reconstructed
/// </summary>
/// <param name="currentRecord">
/// The new currentRecord is available to be initialized or customized.</param>
public override void ModifyCurrentRunRecord(Cognex.VisionPro.ICogRecord currentRecord)
{
}
#endregion
#region When the Last Run Record is Created
/// <summary>
/// Called when the last run record may have changed and is being reconstructed
/// </summary>
/// <param name="lastRecord">
/// The new last run record is available to be initialized or customized.</param>
public override void ModifyLastRunRecord(Cognex.VisionPro.ICogRecord lastRecord)
{
foreach(ICogGraphic s in dt)
{
mToolBlock.AddGraphicToRunRecord(s, lastRecord, "CogImageConvertTool1.InputImage", "");
}
}
#endregion
#region When the Script is Initialized
/// <summary>
/// Perform any initialization required by your script here
/// </summary>
/// <param name="host">The host tool</param>
public override void Initialize(Cognex.VisionPro.ToolGroup.CogToolGroup host)
{
// DO NOT REMOVE - Call the base class implementation first - DO NOT REMOVE
base.Initialize(host);
// Store a local copy of the script host
this.mToolBlock = ((Cognex.VisionPro.ToolBlock.CogToolBlock)(host));
}
#endregion
}