实现一个简单的效果:
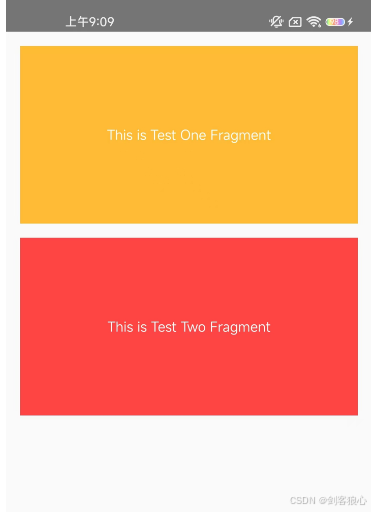
创建 TestOneFragment
java
public class TestOneFragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
// 使用一个简单的布局文件
return inflater.inflate(R.layout.fragment_test_one, container, false);
}
}
对应的 XML 布局文件:
XML
<!-- res/layout/fragment_test_one.xml -->
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/textViewOne"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="This is Test One Fragment" />
</LinearLayout>
测试显示,布局文件的layout_height为match_parent时可以正常显示
创建 TestTwoFragment
java
public class TestTwoFragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
return inflater.inflate(R.layout.fragment_test_two, container, false);
}
}
对应的XML 布局文件:
XML
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:background="@android:color/holo_red_light"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="This is Test Two Fragment"
android:textSize="18sp"
android:textColor="@android:color/white"/>
</LinearLayout>
在主界面添加 Fragment
然后,在你的活动中(比如 MainActivity
)添加这两个 Fragment
。我们可以通过 FragmentTransaction
将两个 Fragment
添加到同一个容器布局中。
java
public class MainActivity6 extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main6);
Log.d("FragmentTest", "Adding fragments...");
// 获取 FragmentManager 和 FragmentTransaction
FragmentManager fragmentManager = getSupportFragmentManager();
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
// 创建两个 Fragment 实例
TestOneFragment testOneFragment = new TestOneFragment();
TestTwoFragment testTwoFragment = new TestTwoFragment();
// 将 Fragment 添加到容器中
fragmentTransaction.add(R.id.fragmentContainerOne, testOneFragment);
fragmentTransaction.add(R.id.fragmentContainerTwo, testTwoFragment);
// 提交事务
fragmentTransaction.commit();
Log.d("FragmentTest", "Fragments added.");
}
}
在 MainActivity
的布局文件中,定义两个 FrameLayout
容器,用来显示两个 Fragment
。
XML
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp">
<!-- 第一个 Fragment 容器 -->
<FrameLayout
android:id="@+id/fragmentContainerOne"
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="@android:color/holo_blue_light"
android:layout_marginBottom="16dp" />
<!-- 第二个 Fragment 容器 -->
<FrameLayout
android:id="@+id/fragmentContainerTwo"
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="@android:color/holo_green_light"/>
</LinearLayout>