gsoap实现webservice服务
在实现Web服务时,使用gSOAP是一个很好的选择,因为它提供了强大的工具和库来创建SOAP和RESTful服务。gSOAP是一个C和C++语言开发的库,它支持SOAP协议的各种版本,包括SOAP 1.1和SOAP 1.2。下面是如何使用gSOAP来实现一个简单的Web服务的步骤:
1、下载gSOAP
仅离线安装【二进制安装】需要下载
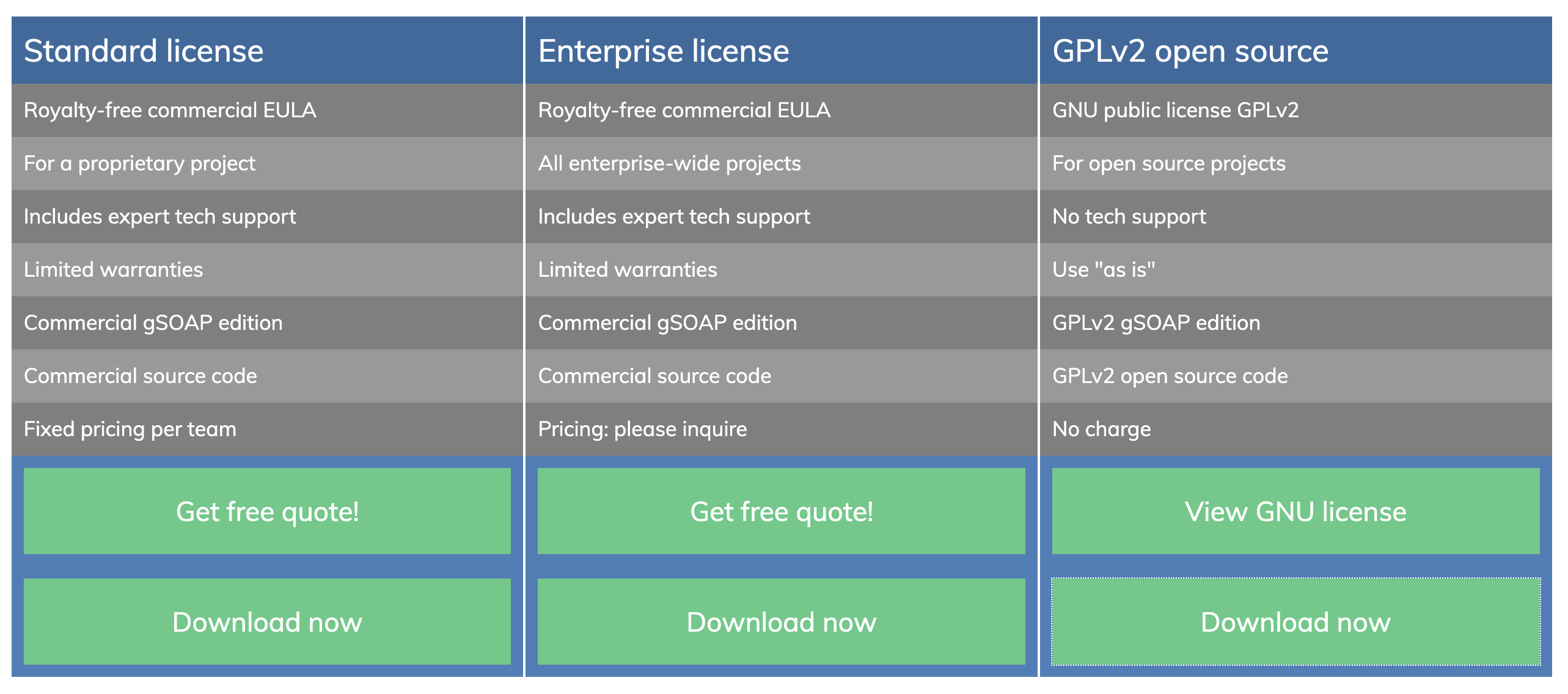
2、安装gSOAP
首先,你需要在你的系统上安装gSOAP。你可以从gSOAP的官方网站下载源代码或者使用包管理器安装。
-》在Ubuntu上安装
sudo apt-get install gsoap
-》在CentOS上安装
二进制安装【离线安装】
bash
# 安装依赖项
sudo yum install g++ libtool autoconf automake make
# 解压并编译(使用下载的包)
tar -zxvf gsoap-2.8x.tar.gz # 替换为你的gSOAP版本号
cd gsoap-2.8x # 替换为你的gSOAP版本号
./configure
make
sudo make install
yum安装
bash
sudo yum install gsoap
-》在Windows上安装
下载gSOAP的Windows版本,解压并设置环境变量,确保soapcpp2.exe
等工具的路径被添加到你的系统路径中。
3、定义你的服务接口
-》手写方式
创建一个名为helloWorldService.h
的文件
cpp
// helloWorldService.h
// gSOAP header file for the calculator service
// gSOAP SOAP Header file
// gSOAP service definition:
// gSOAP service definition generated from helloWorldService.h
// gSOAP XML binding for service: HelloWorldServiceBinding
// gSOAP service namespace: urn:HelloWorldNamespace
int sayHello(char *name, char **response); // 服务接口函数
-》工具使用helloWorld.wsd自动生成
如果先手工编写了wsdl(使用gSOAP的SOAP/XML schema语言定义你的Web服务接口)可以通过wsdl自动生成服务端代码(不包含实现逻辑)
bash
soapcpp2 -j helloWorld.wsdl
这将生成一系列文件,包括soapC.cpp
, soapClient.cpp
, soapH.h
等。
-》wsdl实例
XML
<definitions xmlns="http://schemas.xmlsoap.org/wsdl/"
xmlns:tns="http://example.com/service"
xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/"
targetNamespace="http://example.com/service">
<types>
<xsd:schema xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<xsd:element name="SayHelloRequest" type="xsd:string"/>
<xsd:element name="SayHelloResponse" type="xsd:string"/>
</xsd:schema>
</types>
<message name="SayHelloRequest">
<part name="parameters" element="tns:SayHelloRequest"/>
</message>
<message name="SayHelloResponse">
<part name="parameters" element="tns:SayHelloResponse"/>
</message>
<portType name="GreetingPortType">
<operation name="SayHello">
<input message="tns:SayHelloRequest"/>
<output message="tns:SayHelloResponse"/>
</operation>
</portType>
<binding name="GreetingBinding" type="tns:GreetingPortType">
<soap:binding style="rpc" transport="http://schemas.xmlsoap.org/soap/http"/>
<operation name="SayHello">
<soap:operation soapAction="http://example.com/SayHello"/>
<input>
<soap:body use="encoded" namespace="http://example.com/service" encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"/>
</input>
<output>
<soap:body use="encoded" namespace="http://example.com/service" encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"/>
</output>
</operation>
</binding>
<service name="GreetingService">
<port name="GreetingPort" binding="tns:GreetingBinding">
<soap:address location="http://www.example.com/greeting"/>
</port>
</service>
</definitions>
4. 生成源代码
使用soapcpp2
工具从你的.h
文件生成源代码(不包含实现逻辑)。这包括C++服务器端代码、客户端存根和类型映射代码。
cpp
soapcpp2 -j helloWorldService.h
5. 实现服务逻辑
在生成的.cpp
文件中实现你的服务逻辑。例如,在soapServer.cpp
中:
cpp
#include "soapHelloWorldService.h"
#include "helloWorldService.nsmap"
int main() {
struct soap soap; // 创建soap对象实例
helloWorldServiceBinding binding(&soap); // 绑定到SOAP服务实现
if (!soap_valid_socket(soap_bind(&soap, NULL, 8080, 100))) { // 绑定到端口8080
soap_print_fault(&soap, stderr); // 打印错误信息
return -1;
}
if (soap_serve(&soap)) { // 处理请求
soap_print_fault(&soap, stderr); // 打印错误信息
}
soap_destroy(&soap); // 清理内存资源
soap_end(&soap); // 关闭连接和释放资源
return 0;
}
编译和运行你的服务
编译你的服务代码,并运行它。确保链接了gSOAP库。例如:
bash
g++ -o helloWorldServer soapServer.cpp soapC.cpp soapHelloWorldService.cpp stdsoap2.cpp -lgsoap++ -lpthread -lssl -lcrypto -lssl++ -lcrypto++ -lnsl -lsocket -lresolv -ldl -lm -lrt -lpthread -ldl -lgmp -lgmpxx -lgsl -lgslcblas -lgomp -lstdc++ -lstdc++fs -lboost_system -lboost_filesystem -lboost_thread -lboost_date_time -lboost_chrono -lboost_atomic -lboost_regex -lboost_random -lboost_program_options -lboost_iostreams -lboost_locale -lboost_context -lboost_stacktrace_noop -lboost_stacktrace_addr2line -lboost_timer -lboost_test_exec_monitor -lboost_prg_exec_monitor -lboost_unit_test_framework -lboost_log_setup -lboost_log -lboost_program_options -lboost_system -lboost_filesystem -lpthread -ldl -lm -lrt -lpthread -ldl -lgmp -lgmpxx -lgsl -lgslcblas -lgomp -lstdc++ -lstdc++fs
./helloWorldServer
测试你的服务
你可以使用gSOAP提供的客户端工具或者编写一个简单的客户端来测试你的SOAP服务。例如,创建一个客户端来调用sayHello
方法:
1、获取wsdl
-》方式一:使用服务端提供的
当你运行服务器时,gSOAP 会自动在运行时提供一个 WSDL URL
通常是 http://localhost:8080/?wsdl
如果没有服务端就不是很正规了
-》方式二:用头文件手动生成
你也可以手动生成 WSDL 文件:使用 wsdl2h
和 soapcpp2
来从你的头文件生成 WSDL 文件。例如:
bash
# 注意这里的参数和用法可能需要根据实际gSOAP版本调整,具体请参考gSOAP文档。
# 通常wsdl2h是用来生成WSDL的,但直接从源代码生成WSDL可能不完全准确,因为它依赖于运行时信息。
# 通常建议在服务器运行时访问WSDL URL来获取最新信息
wsdl2h -ceg -o helloWorld.wsdl -t helloWorld.xsd helloWorld.h
2、编写客户端代码
-》第一步:使用wsdl2h工具生成头文件
bash
wsdl2h -o sayHello.h sayHello.wsdl
-》第二步:使用soapcpp2工具生成源代码
bash
soapcpp2 -j helloWorld.h
-》第三步:编写C代码实现SOAP客户端
在你的项目中,创建一个新的C++源文件,例如helloWorld.cpp
,并编写客户端代码来调用SOAP服务。
cpp
#include "helloWorld.h" // 包含由soapcpp2生成的头文件
#include "helloWorld.nsmap" // 可能由soapcpp2生成,包含命名空间映射
#include <iostream>
int main() {
struct soap soap; // 创建soap环境
soap_init(&soap); // 初始化soap环境
soap.send_timeout = 10; // 设置发送超时时间(秒)
soap.recv_timeout = 10; // 设置接收超时时间(秒)
soap.connect_timeout = 10; // 设置连接超时时间(秒)
// 实例化服务端点URL和命名空间
const char *endpoint = "http://example.com/helloWorld"; // SOAP服务的URL
const char *action = ""; // SOAP action(如果有)
struct ns__yourServiceFunctionName soap_response; // 根据WSDL定义的函数名和参数类型创建结构体实例
// 调用SOAP服务函数(替换yourServiceFunctionName为实际的函数名)
if (soap_call_ns__yourServiceFunctionName(&soap, endpoint, NULL, action, /* 传递参数 */, &soap_response) == SOAP_OK) {
// 处理响应数据
std::cout << "Response received: " << soap_response._yourResponseField << std::endl; // 输出响应数据(替换yourResponseField为实际的响应字段名)
} else {
std::cerr << "Error in soap_call_ns__yourServiceFunctionName" << std::endl;
soap_print_fault(&soap, stderr); // 打印错误信息
}
soap_end(&soap); // 清理soap环境
soap_done(&soap); // 完全销毁soap环境
return 0;
}
2、编译和运行客户端
使用gSOAP提供的soapcpp2
生成的.cpp
文件和你的client.cpp
文件一起编译。例如:
cpp
g++ -o client client.cpp soapC.cpp soapClient.cpp -lgsoap++ # 根据你的系统和gSOAP版本,可能需要调整编译命令和链接库选项
然后运行生成的客户端程序:
bash
./client
注意
-
确保替换代码中的
yourServiceFunctionName
、yourResponseField
、服务URL以及任何特定的参数和命名空间以匹配你的实际SOAP服务。 -
检查WSDL文件以确保所有细节正确无误,包括命名空间、方法名、参数类型等。
-
根据需要调整超时设置以适应网络条件。
通过上述步骤,你应该能够创建一个简单的C++ SOAP服务端、客户端来,并测试你的SOAP服务。
说明
1、当运行服务器时,gSOAP 会自动在运行时提供一个 WSDL URL
通常是 http://localhost:8080/?wsdl
如果没有实现这一功能,那么服务端就不正规