目录
[⭕ debug_1:dynamic_pointer_cast](#⭕ debug_1:dynamic_pointer_cast)
Request
RpcRequest测试:
#include "message.hpp"
using namespace bitrpc;
int main()
{
RpcRequest::ptr rrp = MessageFactory::create<RpcRequest>();
Json::Value param;
param["num1"] = 11;
param["num2"] = 22;
rrp->setMethod("Add");
rrp->setParams(param);
std::string ret = rrp->serialize();
std::cout << ret << std::endl;
//
BaseMessage::ptr bmp = MessageFactory::create(MType::REQ_RPC);
bool res = bmp->unserialize(ret);
if(bmp->check() == false)
{
return -1;
}
//!!!!!!! dynamic_pointer_cast的使用
RpcRequest::ptr rrp2 = std::dynamic_pointer_cast<RpcRequest>(bmp);
std::cout << rrp2->method() << std::endl;
std::cout << rrp2->params()["num1"] << std::endl;
std::cout << rrp2->params()["num2"] << std::endl;
return 0;
}
运行:
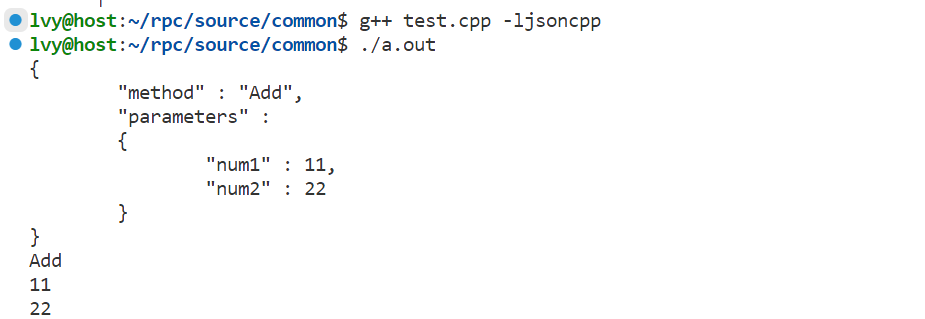
⭕ debug_1:dynamic_pointer_cast
📒dynamic_cast 和 dynamic_Pointer_cast的使用
1.指引或者引用的向上转换,向下转换
例如基类A ,派生类B.。A->B 则为向下转换。。B->A则为向上转换。。向上转换为隐士转换。向下转换需要dynamic_cast或者c的转换方式。
B * b = new B;
A * a = b;
此时b就是向上转换。无需显式转换既可以编译通过。
2.dynamic_cast
一般用于有继承关系的类之间的向下转换。
3.dynamic_pointer_cast
当指针是智能指针时候,向下转换,用dynamic_Cast 则编译不能通过,此时需要使用dynamic_pointer_cast。
TopicRequest测试
int main()
{
TopicRequest::ptr trp = MessageFactory::create<TopicRequest>();
trp->setTopicKey("news");
trp->setOptype(TopicOptype::TOPIC_PUBLISH);
trp->setTopicMsg("hello world");
std::string str = trp->serialize();
std::cout << str << std::endl;
BaseMessage::ptr bmp = MessageFactory::create(MType::REQ_TOPIC);
bmp->unserialize(str);
bool ret = bmp->check();
TopicRequest::ptr trp2 = std::dynamic_pointer_cast<TopicRequest>(bmp);
std::cout << trp2->topicKey() << std::endl;
std::cout << (int)trp2->optype() << std::endl;
std::cout << trp2->topicMsg() << std::endl;
return 0;
}
运行:
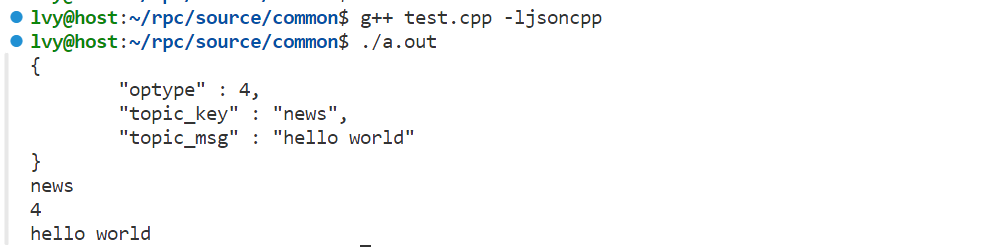
ServiceRequest测试
int main()
{
ServiceRequest::ptr trp = MessageFactory::create<ServiceRequest>();
trp->setMethod("Add");
trp->setOptype(ServiceOptype::SERVICE_REGISTRY);
//服务注册 测试
trp->setHost(Address("127.0.0.1", 8080));
std::string str = trp->serialize();
std::cout << str << std::endl;
BaseMessage::ptr bmp = MessageFactory::create(MType::REQ_SERVICE);
bmp->unserialize(str);
bool ret = bmp->check();
ServiceRequest::ptr trp2 = std::dynamic_pointer_cast<ServiceRequest>(bmp);
std::cout << trp2->method() << std::endl;
std::cout << (int)trp2->optype() << std::endl;
std::cout << trp2->host().first << std::endl;
std::cout << trp2->host().second << std::endl;
return 0;
}
⭕debug_2:gdb
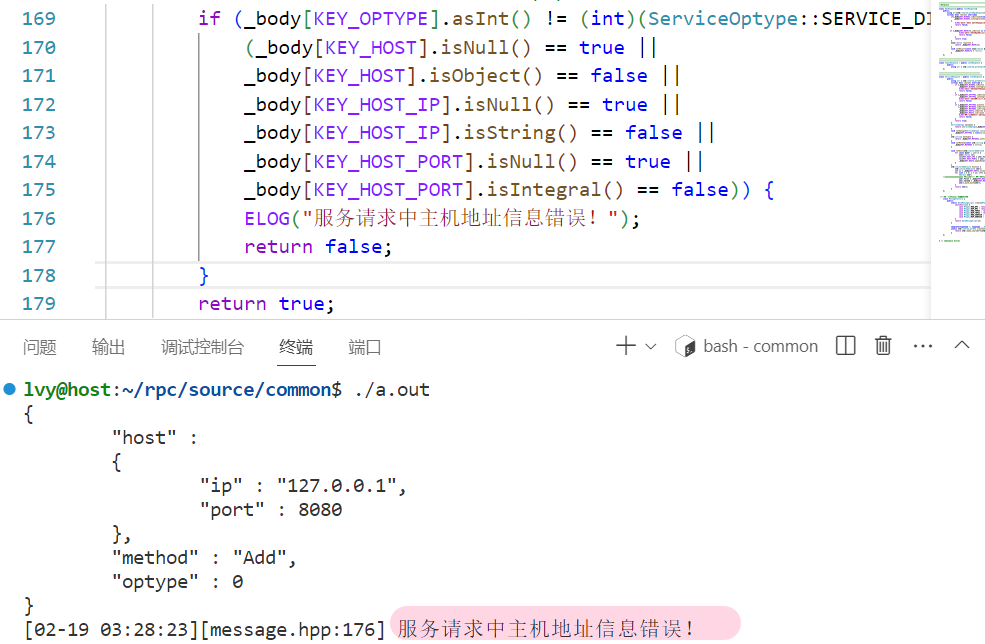
调试:
使用 gdb

(gdb) break message.hpp:169
run
gdb 调试中 不能使用宏 !!!!!!!!!!!!!!!!!!!!
后续采用 p 打印查看
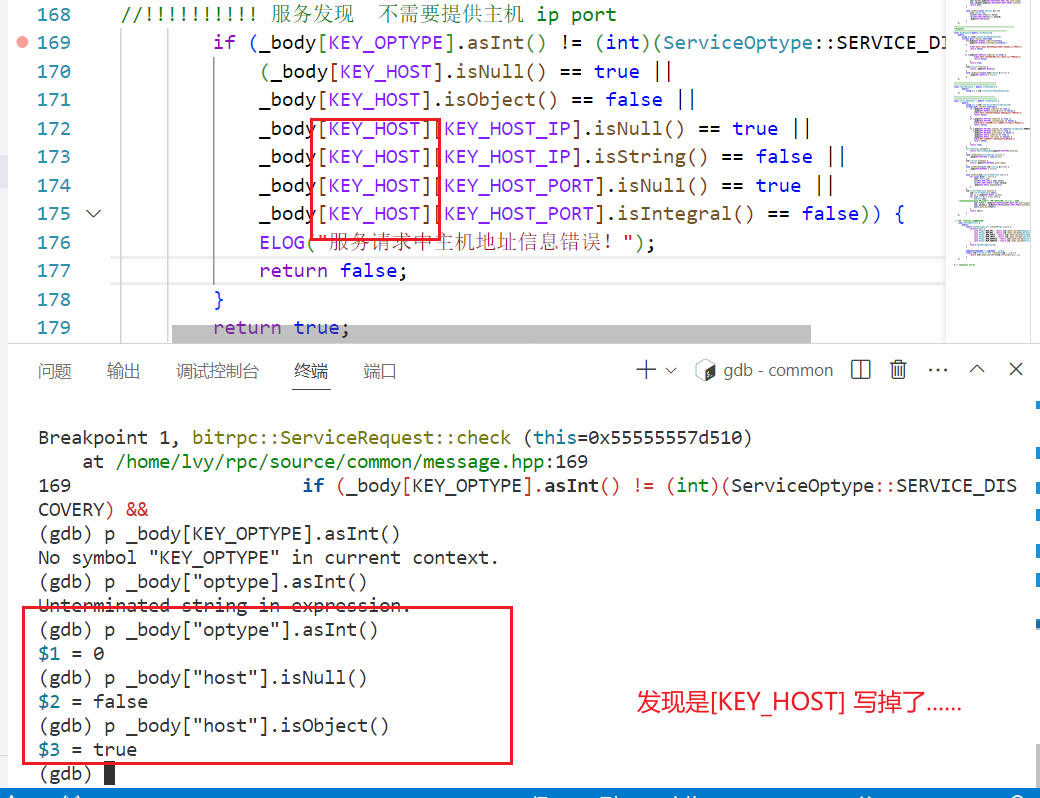
Response
RpcResponse测试
int main()
{
RpcResponse::ptr trp = MessageFactory::create<RpcResponse>();
trp->setRCode(RCode::RCODE_OK);
trp->setResult(33);
std::string str = trp->serialize();
std::cout << str << std::endl;
BaseMessage::ptr bmp = MessageFactory::create(MType::RSP_RPC);
bmp->unserialize(str);
bool ret = bmp->check();
RpcResponse::ptr trp2 = std::dynamic_pointer_cast<RpcResponse>(bmp);
std::cout << (int)trp2->rcode() << std::endl;
std::cout << trp2->result().asInt() << std::endl;
return 0;
}
运行:
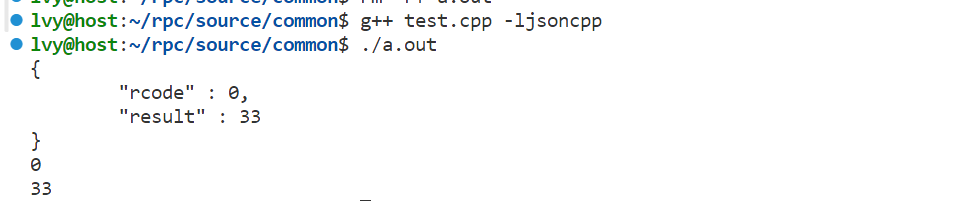
TopicResponse测试
int main()
{
TopicResponse::ptr trp = MessageFactory::create<TopicResponse>();
trp->setRCode(RCode::RCODE_OK);
std::string str = trp->serialize();
std::cout << str << std::endl;
BaseMessage::ptr bmp = MessageFactory::create(MType::RSP_TOPIC);
bmp->unserialize(str);
bool ret = bmp->check();
TopicResponse::ptr trp2 = std::dynamic_pointer_cast<TopicResponse>(bmp);
std::cout << (int)trp2->rcode() << std::endl;
return 0;
}
运行:

ServiceResponse测试
int main()
{
ServiceResponse::ptr trp = MessageFactory::create<ServiceResponse>();
trp->setRCode(RCode::RCODE_OK);
trp->setMethod("Add");
trp->setOptype(ServiceOptype::SERVICE_DISCOVERY);
std::vector<Address> addrs;
addrs.push_back(Address{"127.0.0.1", 8080});
addrs.push_back(Address{"127.0.0.2", 9090});
trp->setHost(addrs);
std::string str = trp->serialize();
std::cout << str << std::endl;
BaseMessage::ptr bmp = MessageFactory::create(MType::RSP_SERVICE);
bmp->unserialize(str);
bool ret = bmp->check();
ServiceResponse::ptr trp2 = std::dynamic_pointer_cast<ServiceResponse>(bmp);
std::cout << (int)trp2->rcode() << std::endl;
std::cout << trp2->method() << std::endl;
std::cout << (int)trp2->optype() << std::endl;
std::vector<Address> addrs1 = trp2->hosts();
for(auto& addr : addrs1)
{
std::cout << addr.first << std::endl;
std::cout << addr.second << std::endl;
}
return 0;
}
运行:
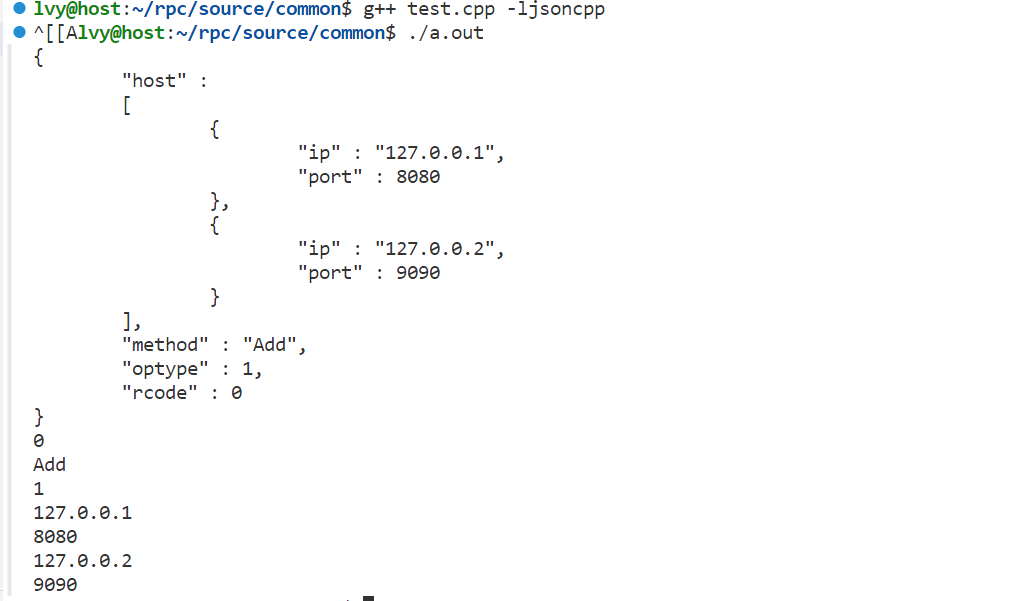