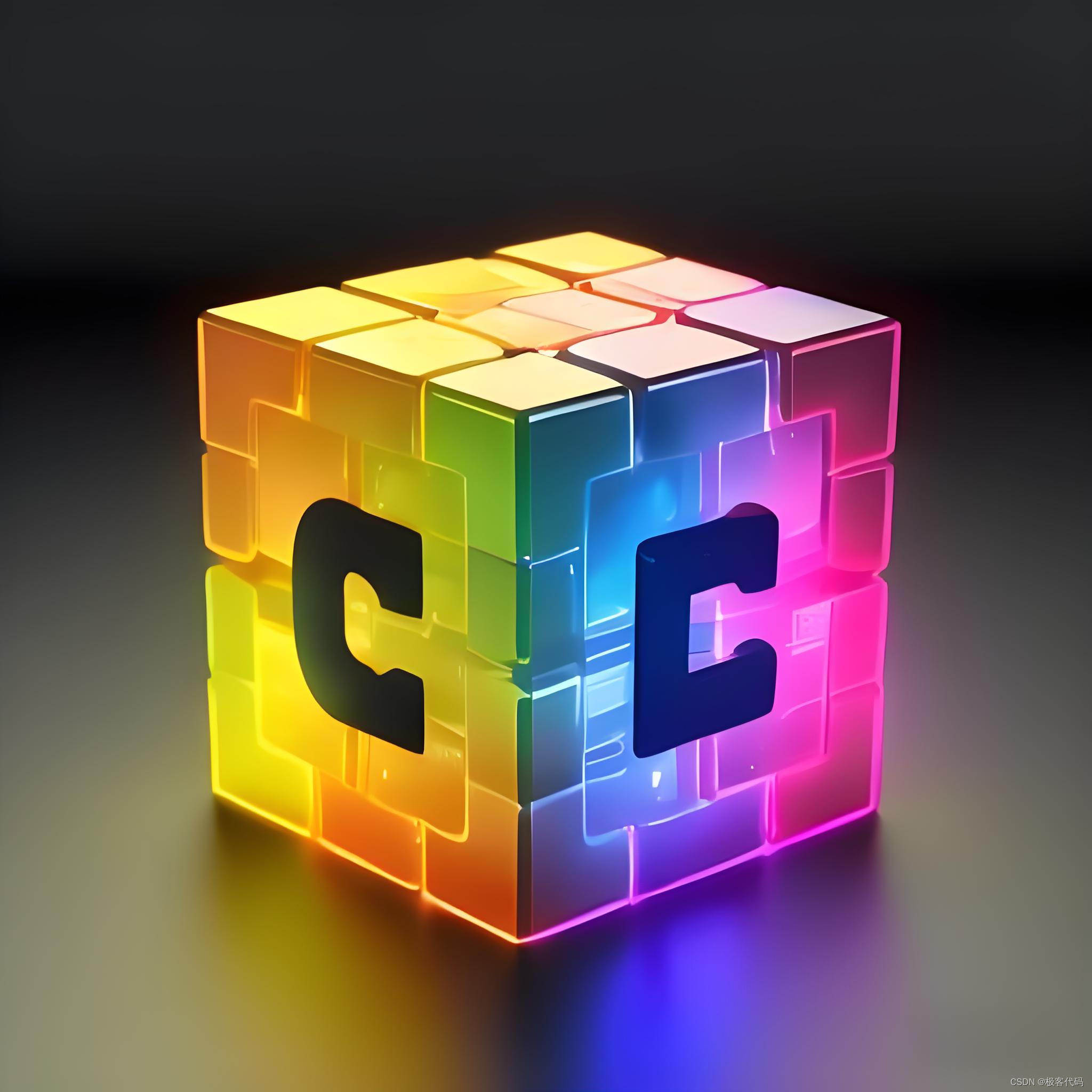
链表是一种常用的数据结构,在许多应用程序中都有广泛的应用。它由一系列节点组成,每个节点包含数据和指向下一个节点的指针。本篇文章将详细介绍如何在C语言中实现链表的封装,并提供一些基本的操作函数,如添加元素、删除元素、遍历列表等。
链表的基本概念
链表是一种动态数据结构,它不像数组那样要求连续的内存空间。链表中的每个元素(称为节点)都包含了实际的数据和一个指向下一个节点的指针。这种结构使得在链表中插入和删除元素变得非常容易。
链表节点的定义
首先,我们需要定义链表节点的结构体。一个典型的链表节点结构如下:
typedef struct ListNode {
int data; // 存储的数据
struct ListNode *next; // 指向下一个节点的指针
} ListNode;
这里定义了一个名为 `ListNode` 的结构体类型,其中包含了一个整型数据 `data` 和一个指向相同类型的指针 `next`。
链表的基本操作
接下来我们将定义几个常用的链表操作函数,这些函数将帮助我们完成对链表的增删改查等基本操作。
创建空链表
// 创建一个空链表
ListNode *createList() {
return NULL;
}
添加元素到链表尾部
// 在链表尾部添加一个新元素
void appendToList(ListNode **list, int value) {
ListNode *newNode = (ListNode *)malloc(sizeof(ListNode));
newNode->data = value;
newNode->next = NULL;
if (*list == NULL) {
*list = newNode;
} else {
ListNode *current = *list;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
从链表中删除元素
// 从链表中删除指定值的节点
void deleteFromList(ListNode **list, int value) {
ListNode *current = *list;
ListNode *previous = NULL;
while (current != NULL && current->data != value) {
previous = current;
current = current->next;
}
if (current == NULL) {
return; // 没有找到要删除的元素
}
if (previous == NULL) {
*list = current->next;
} else {
previous->next = current->next;
}
free(current); // 释放节点
}
遍历链表并打印所有元素
// 遍历链表并打印所有元素
void printList(ListNode *list) {
ListNode *current = list;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
清空链表
// 清空整个链表
void clearList(ListNode **list) {
ListNode *current = *list;
ListNode *next;
while (current != NULL) {
next = current->next;
free(current);
current = next;
}
*list = NULL;
}
完整示例代码
现在让我们将上述函数整合到一个完整的示例中,以便展示它们是如何工作的。
#include <stdio.h>
#include <stdlib.h>
typedef struct ListNode {
int data;
struct ListNode *next;
} ListNode;
// 创建一个空链表
ListNode *createList() {
return NULL;
}
// 在链表尾部添加一个新元素
void appendToList(ListNode **list, int value) {
ListNode *newNode = (ListNode *)malloc(sizeof(ListNode));
newNode->data = value;
newNode->next = NULL;
if (*list == NULL) {
*list = newNode;
} else {
ListNode *current = *list;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 从链表中删除指定值的节点
void deleteFromList(ListNode **list, int value) {
ListNode *current = *list;
ListNode *previous = NULL;
while (current != NULL && current->data != value) {
previous = current;
current = current->next;
}
if (current == NULL) {
return; // 没有找到要删除的元素
}
if (previous == NULL) {
*list = current->next;
} else {
previous->next = current->next;
}
free(current); // 释放节点
}
// 遍历链表并打印所有元素
void printList(ListNode *list) {
ListNode *current = list;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
// 清空整个链表
void clearList(ListNode **list) {
ListNode *current = *list;
ListNode *next;
while (current != NULL) {
next = current->next;
free(current);
current = next;
}
*list = NULL;
}
int main() {
ListNode *list = createList();
appendToList(&list, 10);
appendToList(&list, 20);
appendToList(&list, 30);
printf("Original List:\n");
printList(list);
deleteFromList(&list, 20);
printf("After Deleting 20:\n");
printList(list);
clearList(&list);
printf("After Clearing the List:\n");
printList(list);
return 0;
}
总结
以上就是关于链表封装的介绍。通过本篇文章,你已经学会了如何在C语言中定义链表节点、实现链表的基本操作函数,并且通过一个完整的示例了解了这些函数的使用方法。链表是一种非常灵活的数据结构,掌握了这些基本操作之后,你可以根据需要扩展更多的功能。