《Qt窗口动画实战:窗口渐隐渐现效果》
在桌面应用开发中,优雅的窗口动画能显著提升用户体验。本文将分享我如何使用Qt实现窗口的渐隐渐现效果,以及在此过程中解决的各种技术难题。
1、看看效果如何
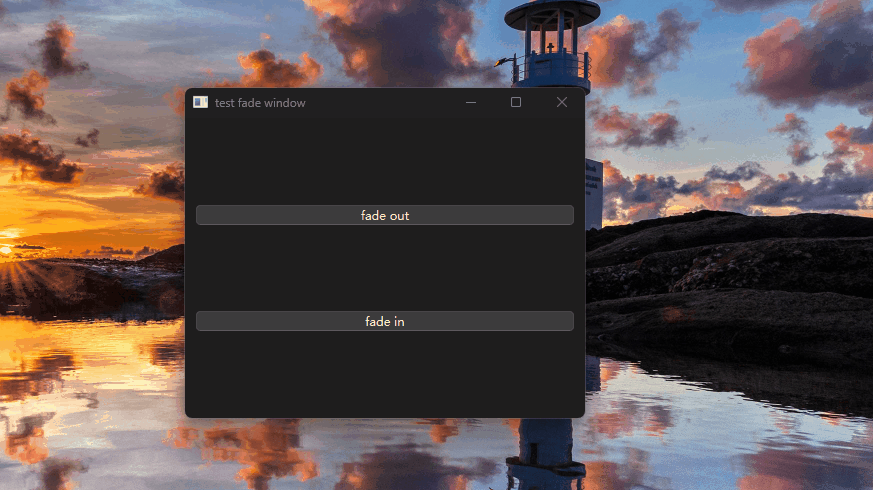
2、设计过程
1、设计思路
- 窗口显示时:从完全透明渐变到完全不透明
- 窗口隐藏时:从完全不透明渐变到完全透明
- 支持自定义动画时长
- 兼容Windows、macOS、Linux三大平台
2、设计方案
经过调研,我选择了以下技术方案:
核心机制:QPropertyAnimation
3、实现的具体过程
mainwindow.h
文件的实现
cpp
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include <QPropertyAnimation>
#include <QPushButton>
class MainWindow : public QMainWindow
{
Q_OBJECT
Q_PROPERTY(qreal windowOpacity READ windowOpacity WRITE setWindowOpacity)
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private slots:
void fadeOut();
void fadeIn();
private:
QPropertyAnimation *animation;
QPushButton *fadeOutBtn;
QPushButton *fadeInBtn;
};
#endif // MAINWINDOW_H
mainwindow.cpp
文件的实现
cpp
#include "mainwindow.h"
#include <QVBoxLayout>
#include <QWidget>
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
{
setWindowTitle("test fade window");
resize(400, 300);
QWidget *centralWidget = new QWidget(this);
QVBoxLayout *layout = new QVBoxLayout(centralWidget);
setCentralWidget(centralWidget);
fadeOutBtn = new QPushButton("fade out", this);
fadeInBtn = new QPushButton("fade in", this);
layout->addWidget(fadeOutBtn);
layout->addWidget(fadeInBtn);
animation = new QPropertyAnimation(this, "windowOpacity", this);
animation->setDuration(1000);
connect(fadeOutBtn, &QPushButton::clicked, this, &MainWindow::fadeOut);
connect(fadeInBtn, &QPushButton::clicked, this, &MainWindow::fadeIn);
}
MainWindow::~MainWindow()
{
}
void MainWindow::fadeOut()
{
animation->setStartValue(1.0);
animation->setEndValue(0.0);
animation->start();
}
void MainWindow::fadeIn()
{
animation->setStartValue(0.0);
animation->setEndValue(1.0);
animation->start();
}
main.cpp
实现:
cpp
#include "mainwindow.h"
#include <QApplication>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MainWindow w;
w.show();
return a.exec();
}