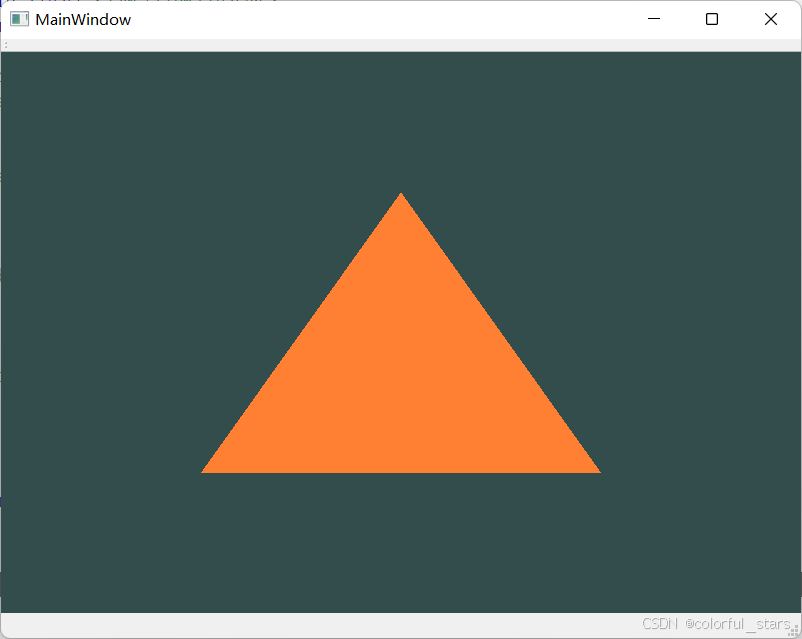
OpenGLEntity.h
cpp
#ifndef OPENGLENTITY_H
#define OPENGLENTITY_H
//#include <QWidget>
#include <QOpenGLWidget>
#include <QOpenGLFunctions_3_3_Core>
#include <QOpenGLShaderProgram>
#include <QOpenGLBuffer>
#include <QOpenGLVertexArrayObject>
class OpenGLEntity : public QOpenGLWidget, protected QOpenGLFunctions_3_3_Core
{
Q_OBJECT
public:
explicit OpenGLEntity(QWidget *parent = 0);
~OpenGLEntity();
signals:
public slots:
protected:
void initializeGL() override;
void resizeGL(int w, int h) override;
void paintGL() override;
private:
QOpenGLShaderProgram program;
QOpenGLBuffer vertexBuffer;
QOpenGLVertexArrayObject vao;
};
#endif // OPENGLENTITY_H
OpenGLEntity.cpp
cpp
#include "OpenGLEntity.h"
OpenGLEntity::OpenGLEntity(QWidget *parent) : QOpenGLWidget(parent)
{
}
OpenGLEntity::~OpenGLEntity()
{
makeCurrent();
vertexBuffer.destroy();
vao.destroy();
doneCurrent();
}
void OpenGLEntity::initializeGL()
{
initializeOpenGLFunctions();
// 编译顶点着色器
const char *vertexShaderSource = R"(
#version 330 core
layout (location = 0) in vec3 aPos;
void main()
{
gl_Position = vec4(aPos, 1.0);
}
)";
program.addShaderFromSourceCode(QOpenGLShader::Vertex, vertexShaderSource);
// 编译片段着色器
const char *fragmentShaderSource = R"(
#version 330 core
out vec4 FragColor;
void main()
{
FragColor = vec4(1.0, 0.5, 0.2, 1.0); // 橙色
}
)";
program.addShaderFromSourceCode(QOpenGLShader::Fragment, fragmentShaderSource);
// 链接程序
program.link();
// 检查链接是否成功
if (!program.isLinked()) {
qDebug() << "Failed to link program:" << program.log();
return;
}
// 设置顶点数据(一个三角形)
float vertices[] = {
-0.5f, -0.5f, 0.0f,
0.5f, -0.5f, 0.0f,
0.0f, 0.5f, 0.0f
};
// 创建并绑定 VBO
vertexBuffer.create();
vertexBuffer.bind();
vertexBuffer.allocate(vertices, sizeof(vertices));
// 创建并绑定 VAO
vao.create();
vao.bind();
// 设置顶点属性指针
glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE, 3 * sizeof(float), (void*)0);
glEnableVertexAttribArray(0);
// 解绑 VAO 和 VBO
vao.release();
vertexBuffer.release();
}
void OpenGLEntity::resizeGL(int w, int h)
{
glViewport(0, 0, w, h);
}
void OpenGLEntity::paintGL()
{
glClearColor(0.2f, 0.3f, 0.3f, 1.0f); // 设置背景颜色为深灰色
glClear(GL_COLOR_BUFFER_BIT);
// 使用我们的着色器程序
program.bind();
// 绘制三角形
vao.bind();
glDrawArrays(GL_TRIANGLES, 0, 3);
vao.release();
}
MainWindow.cpp
cpp
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include"OpenGLEntity.h"
namespace Ui {
class MainWindow;
}
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = 0);
~MainWindow();
private:
Ui::MainWindow *ui;
OpenGLEntity *openglentity;
};
#endif // MAINWINDOW_H
MainWindow.cpp
cpp
#include "MainWindow.h"
#include "ui_MainWindow.h"
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
resize(800,600);
openglentity = new OpenGLEntity(this);
setCentralWidget(openglentity);
}
MainWindow::~MainWindow()
{
delete ui;
}