直接上图上代码吧
javascript
// login/login.js
const app = getApp()
Page({
/**
* 页面的初始数据
*/
data: {
},
/**
* 生命周期函数--监听页面加载
*/
onLoad(options) {
},
/**
* 生命周期函数--监听页面初次渲染完成
*/
onReady() {
},
/**
* 生命周期函数--监听页面显示
*/
onShow() {
},
/**
* 生命周期函数--监听页面隐藏
*/
onHide() {
},
/**
* 生命周期函数--监听页面卸载
*/
onUnload() {
},
/**
* 页面相关事件处理函数--监听用户下拉动作
*/
onPullDownRefresh() {
},
/**
* 页面上拉触底事件的处理函数
*/
onReachBottom() {
},
/**
* 用户点击右上角分享
*/
onShareAppMessage() {
},
handleClick({currentTarget}){
let userType = currentTarget.dataset.type
// debugger
app.globalData.userType = currentTarget.dataset.type
const targetPath = userType === 'a' ? '/pages/person/home/home' : '/pages/company/gift/gift';
wx.switchTab({ url: targetPath }); // 关键:跳转到Tab页
}
})
html
<!--login/login.wxml-->
<text>login/login.wxml</text>
<view><button data-type="a" bind:tap="handleClick">登陆个人</button></view>
<view><button data-type="b" bind:tap="handleClick">登陆企业</button></view>
1,这是我的目录解构
开启自定义tabbar开关custom:true,
然后list需要把所有tabar页面都注册进去
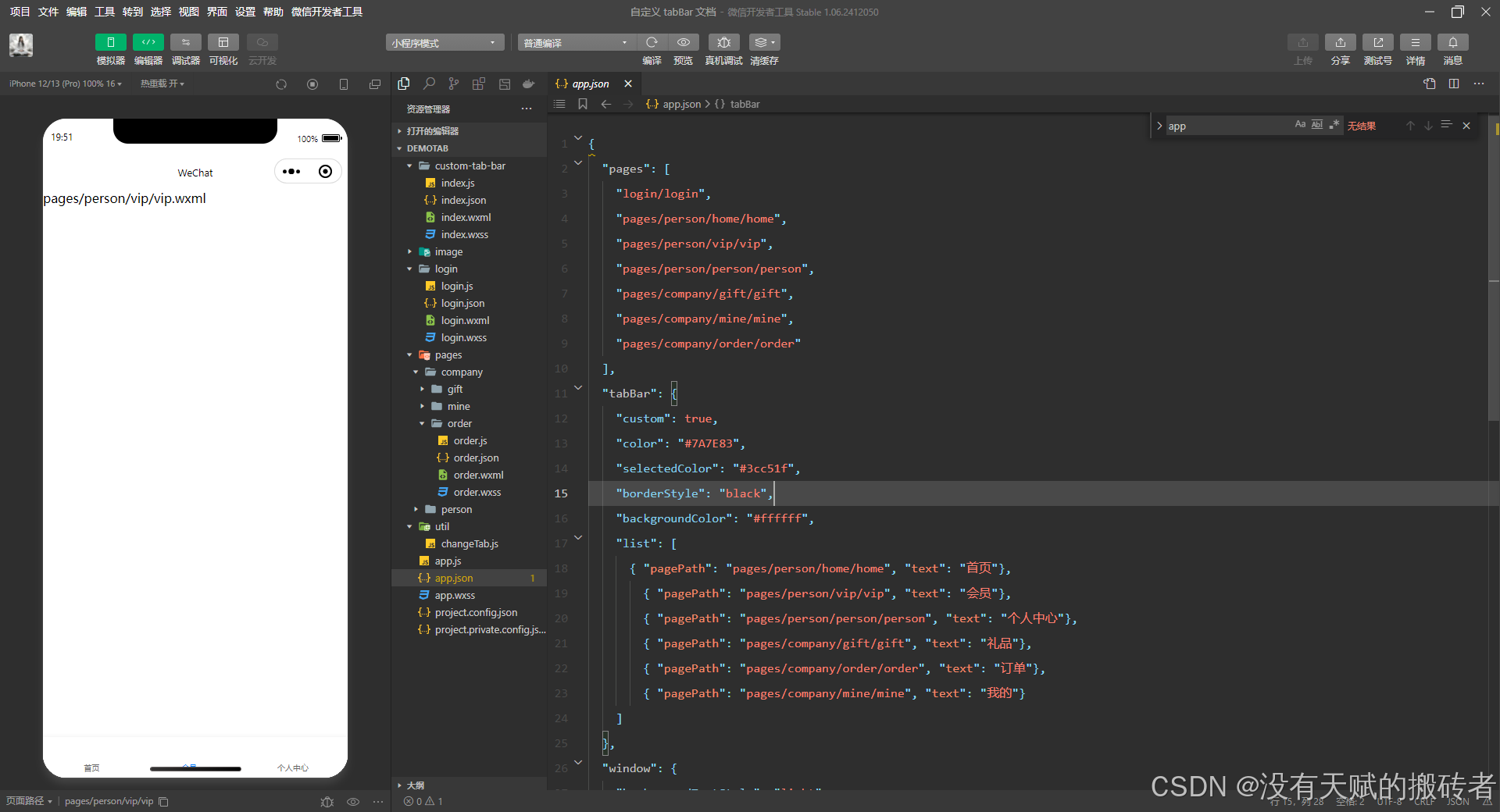
javascript
// app.json
{
"pages": [
"login/login",
"pages/person/home/home",
"pages/person/vip/vip",
"pages/person/person/person",
"pages/company/gift/gift",
"pages/company/mine/mine",
"pages/company/order/order"
],
"tabBar": {
"custom": true,
"color": "#7A7E83",
"selectedColor": "#3cc51f",
"borderStyle": "black",
"backgroundColor": "#ffffff",
"list": [
{ "pagePath": "pages/person/home/home", "text": "首页"},
{ "pagePath": "pages/person/vip/vip", "text": "会员"},
{ "pagePath": "pages/person/person/person", "text": "个人中心"},
{ "pagePath": "pages/company/gift/gift", "text": "礼品"},
{ "pagePath": "pages/company/order/order", "text": "订单"},
{ "pagePath": "pages/company/mine/mine", "text": "我的"}
]
},
"window": {
"backgroundTextStyle": "light",
"navigationBarBackgroundColor": "#fff",
"navigationBarTitleText": "WeChat",
"navigationBarTextStyle": "black"
},
"usingComponents": {}
}
2.自定义tabbar文件夹 custom-tab-bar
javascript
// custom-tab-bar/index.js
Component({
data: {
tabList: [], // 根据用户类型动态设置
activeIndex: 0 // 当前选中Tab
},
methods: {
switchTab(e) {
// const path = e.currentTarget.dataset.path;
// const index = e.currentTarget.dataset.index;
// wx.switchTab({ url: path });
// this.setData({ activeIndex: index });
const { path, index } = e.currentTarget.dataset;
console.log(path)
wx.switchTab({
url: path,
success: () => {
console.log('跳转成功');
this.setData({ activeIndex: index });
},
fail: (err) => {
console.error('跳转失败:', err);
}
});
},
// 根据用户类型更新Tab列表
updateTabList(userType) {
const tabs = {
a: [
{ pagePath: "/pages/person/home/home", text: "首页"},
{ pagePath: "/pages/person/vip/vip", text: "会员"},
{ pagePath: "/pages/person/person/person", text: "个人中心"}
],
b: [
{ pagePath: "/pages/company/gift/gift", text: "礼品"},
{ pagePath: "/pages/company/order/order", text: "订单"},
{ pagePath: "/pages/company/mine/mine", text: "我的"}
]
};
this.setData({ tabList: tabs[userType] });
}
},
});
javascript
//index.json
{
"component": true
}
html
<!-- custom-tab-bar/index.wxml -->
<!-- <view class="tab-bar">
<block wx:for="{{tabList}}" wx:key="index">
<view
class="tab-item {{activeIndex === index ? 'active' : ''}}"
bindtap="switchTab"
data-path="{{item.pagePath}}"
data-index="{{index}}"
>
<image src="{{activeIndex === index ? item.selectedIconPath : item.iconPath}}"></image>
<text>{{item.text}}</text>
</view>
</block>
</view> -->
<!-- custom-tab-bar/index.wxml -->
<cover-view class="tab-bar">
<block wx:for="{{tabList}}" wx:key="index">
<cover-view
class="tab-item {{activeIndex === index ? 'active' : ''}}"
bindtap="switchTab"
data-path="{{item.pagePath}}"
data-index="{{index}}"
>
<cover-image class="tab-icon" src="{{activeIndex === index ? item.selectedIconPath : item.iconPath}}" />
<cover-view class="tab-text">{{item.text}}</cover-view>
</cover-view>
</block>
</cover-view>
css
/* custom-tab-bar/index.wxss */
.tab-bar {
position: fixed;
bottom: 0;
left: 0;
right: 0;
height: 100rpx; /* 高度与原生TabBar一致 */
background: #ffffff;
display: flex;
align-items: center;
justify-content: space-around;
box-shadow: 0 -2rpx 10rpx rgba(0,0,0,0.05); /* 顶部阴影 */
z-index: 9999; /* 确保层级最高 */
}
.tab-item {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100%;
padding: 0 20rpx;
}
.tab-icon {
width: 48rpx;
height: 48rpx;
margin-bottom: 4rpx;
}
.tab-text {
font-size: 20rpx;
color: #666666;
}
/* 选中状态样式 */
.tab-item.active .tab-text {
color: #007AFF; /* 主题色 */
}
.tab-item.active .tab-icon {
filter: brightness(0.8); /* 图标选中效果 */
}
3.自定义util
javascript
// util/changetab.js
const app = getApp()
module.exports = Behavior({
methods: {
updateTabBarIndex(index) {
const userType = app.globalData.userType;
const tabBar = this.getTabBar();
if (tabBar) {
tabBar.updateTabList(userType);
tabBar.setData({ activeIndex: index })
};
}
}
});
z最后在各个tabbar对于的页面的onshow中引入util方法
javascript
// pages/person/home/home.js
const changeTab = require('../../../util/changeTab')
Page({
behaviors:[changeTab],
/**
* 页面的初始数据
*/
data: {
},
/**
* 生命周期函数--监听页面显示
*/
onShow() {
this.updateTabBarIndex(0);
},
/**
* 生命周期函数--监听页面隐藏
*/
onHide() {
},
/**
* 生命周期函数--监听页面卸载
*/
onUnload() {
},
/**
* 页面相关事件处理函数--监听用户下拉动作
*/
onPullDownRefresh() {
},
/**
* 页面上拉触底事件的处理函数
*/
onReachBottom() {
},
/**
* 用户点击右上角分享
*/
onShareAppMessage() {
}
})
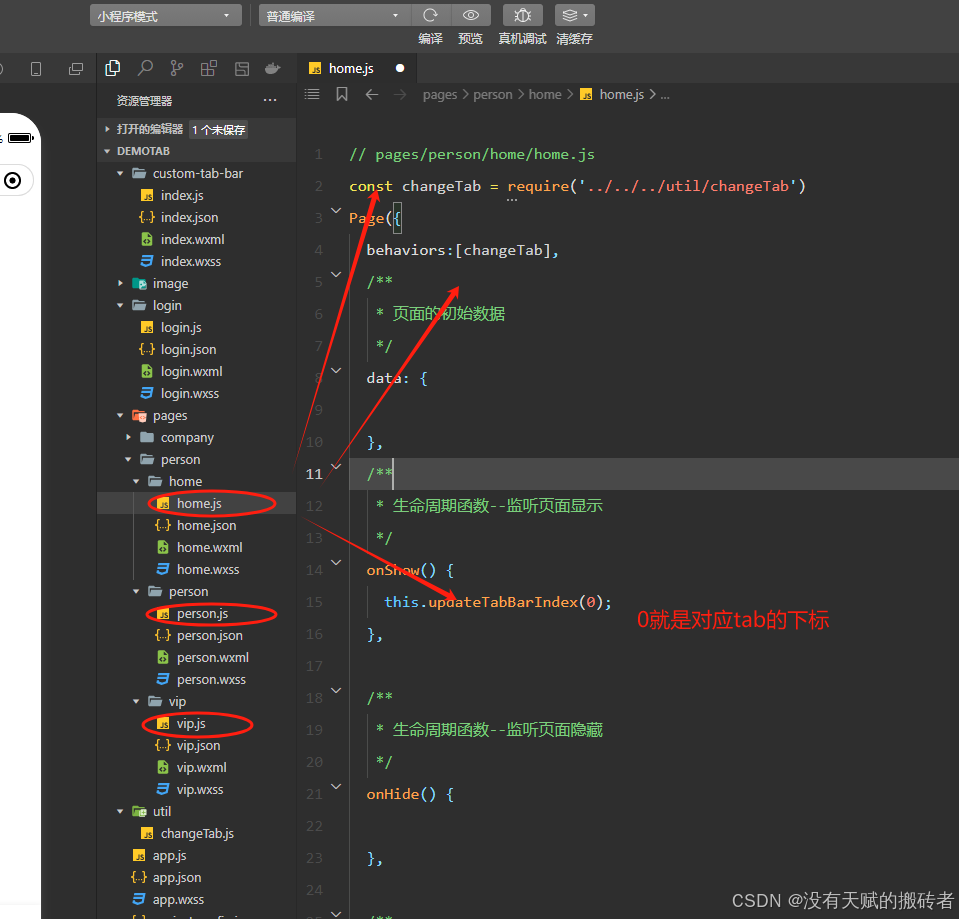