文章目录
-
- 一、转换方法总结
- 二、详细步骤
-
- [1. Unicode 项目(`CStringW` → `std::string`)](#1. Unicode 项目(
CStringW
→std::string
)) - [2. 多字节项目(`CStringA` → `std::string`)](#2. 多字节项目(
CStringA
→std::string
))
- [1. Unicode 项目(`CStringW` → `std::string`)](#1. Unicode 项目(
- 三、注意事项
- 四、总结
- 更多信息(知识点存在重复,可跳过)
以下是对 MFC 中 CString
转 std::string
方法 的总结,涵盖不同字符集配置下的解决方案及注意事项:
一、转换方法总结
项目字符集 | 转换方法 | 代码示例 |
---|---|---|
Unicode 字符集 | 使用 CT2A 宏或 WideCharToMultiByte 函数进行宽字符到多字节的转换 |
CStringW cstr = L"Unicode文本"; std::string str = CT2A(cstr).m_psz; |
多字节字符集 | 直接通过 CStringA 或 GetString() 获取 char* 赋值给 std::string |
CStringA cstr = "多字节文本"; std::string str(cstr.GetString()); |
二、详细步骤
1. Unicode 项目(CStringW
→ std::string
)
-
方法 1:使用
CT2A
宏(推荐)cpp#include <atlconv.h> // 必须包含头文件 CString cstr = _T("Hello, 你好!"); std::string str = CT2A(cstr.GetString()); // 自动转换(默认编码为 ANSI)
- 指定编码 :如需 UTF-8,修改为
CT2A(cstr, CP_UTF8)
。
- 指定编码 :如需 UTF-8,修改为
-
方法 2:手动调用
WideCharToMultiByte
cppCStringW cstr = L"Hello, 你好!"; int size = WideCharToMultiByte(CP_UTF8, 0, cstr.GetString(), -1, nullptr, 0, nullptr, nullptr); std::string str(size, '\0'); WideCharToMultiByte(CP_UTF8, 0, cstr.GetString(), -1, &str[0], size, nullptr, nullptr); str.pop_back(); // 去除末尾的 '\0'
2. 多字节项目(CStringA
→ std::string
)
-
直接赋值(无需复杂转换):
cppCStringA cstr = "Hello, World!"; std::string str(cstr.GetString());
三、注意事项
-
编码一致性
- Unicode 项目中,转换时需明确目标编码(如 UTF-8、GBK)。
- 中文文本建议统一使用 UTF-8 避免乱码。
-
CT2A
宏的潜在问题- 依赖
<atlconv.h>
头文件。 - 临时转换时,注意其内部使用栈内存,可能引发缓冲区溢出(大文本慎用)。
- 依赖
-
跨项目适配
-
使用
_T()
宏定义字符串,配合条件编译:cppCString cstr = _T("Hello, 你好!"); #ifdef _UNICODE std::string str = CT2A(cstr.GetString(), CP_UTF8); #else std::string str = cstr.GetString(); #endif
-
-
处理空字符
- 使用
WideCharToMultiByte
转换后,std::string
末尾可能包含\0
,需手动调用pop_back()
清理。
- 使用
四、总结
场景 | 推荐方法 | 优点 | 缺点 |
---|---|---|---|
Unicode → UTF-8 | WideCharToMultiByte |
精确控制编码 | 代码较冗长 |
Unicode → ANSI | CT2A 宏 |
简洁高效 | 依赖 ATL 库 |
多字节 → ANSI | 直接赋值 | 无需转换,性能最优 | 仅限多字节项目 |
通过上述方法,可灵活应对 MFC 中 CString
到 std::string
的转换需求,确保代码兼容性与编码安全。
在 MFC 中将 CString
转换为 std::string
需要根据项目的字符集配置(Unicode 或多字节)选择合适的方法。以下是两种常见场景的转换方法:
更多信息(知识点存在重复,可跳过)
方法 1:项目使用 Unicode 字符集(默认)
若项目是 Unicode 编码(CString
实际为 CStringW
,存储 wchar_t
),需将宽字符转换为多字节字符:
使用 WideCharToMultiByte
函数
cpp
#include <string>
CStringW cstr = L"你好,世界!";
// 计算所需缓冲区大小
int size = WideCharToMultiByte(CP_UTF8, 0, cstr.GetString(), -1, nullptr, 0, nullptr, nullptr);
std::string str(size, '\0');
WideCharToMultiByte(CP_UTF8, 0, cstr.GetString(), -1, &str[0], size, nullptr, nullptr);
// 去除末尾的 null 终止符
if (!str.empty() && str.back() == '\0') {
str.pop_back();
}
使用 CT2A
宏(自动适配编码)
cpp
#include <string>
#include <atlconv.h> // 需包含此头文件
CString cstr = _T("Hello, World!");
std::string str = CT2A(cstr.GetString()); // 自动转换
方法 2:项目使用 多字节字符集
若项目是多字节编码(CString
为 CStringA
,存储 char
),可直接转换:
cpp
CStringA cstr = "Hello, World!";
std::string str(cstr.GetString()); // 直接赋值
注意事项
-
字符编码兼容性
使用
WideCharToMultiByte
时,通过CP_UTF8
、CP_ACP
等参数指定编码。若处理中文,建议统一使用 UTF-8 避免乱码。 -
简化转换宏
CT2A
宏(T2A
)会自动处理 Unicode 到多字节的转换,但需确保项目包含<atlconv.h>
头文件。 -
跨项目适配
若代码需在 Unicode/多字节配置下通用,可使用
_T()
宏定义字符串,并通过条件编译处理:cppCString cstr = _T("Hello, 你好!"); #ifdef _UNICODE std::string str = CT2A(cstr.GetString()); #else std::string str = cstr.GetString(); #endif
完整示例代码
cpp
#include <string>
#include <atlconv.h>
void ConvertCStringToStdString() {
// Unicode 项目示例
CStringW unicodeStr = L"Unicode: 你好!";
std::string utf8Str = CT2A(unicodeStr, CP_UTF8); // 显式指定 UTF-8
// 多字节项目示例
CStringA mbStr = "Multi-byte: 你好!";
std::string ansiStr = mbStr.GetString();
}
选择方法时,优先考虑编码一致性及项目配置。若需深度控制编码细节,推荐手动使用 WideCharToMultiByte
;若追求简洁,CT2A
是更快捷的选择。
总结
在 MFC 开发中,CString
与 std::string
的转换需关注项目的字符编码配置:
- Unicode 项目 优先使用
CT2A
宏或WideCharToMultiByte
函数,确保宽字符到多字节的安全转换; - 多字节项目可直接通过构造函数赋值,简洁高效。
无论选择哪种方法,务必统一字符编码(如 UTF-8),避免因编码差异导致乱码或兼容性问题。若需跨配置适配,可通过 _T()
宏和条件编译提升代码灵活性。
希望本文能为您提供清晰的解决方案,若有更多疑问,欢迎探索微软官方文档或社区资源,祝编程愉快! 🚀
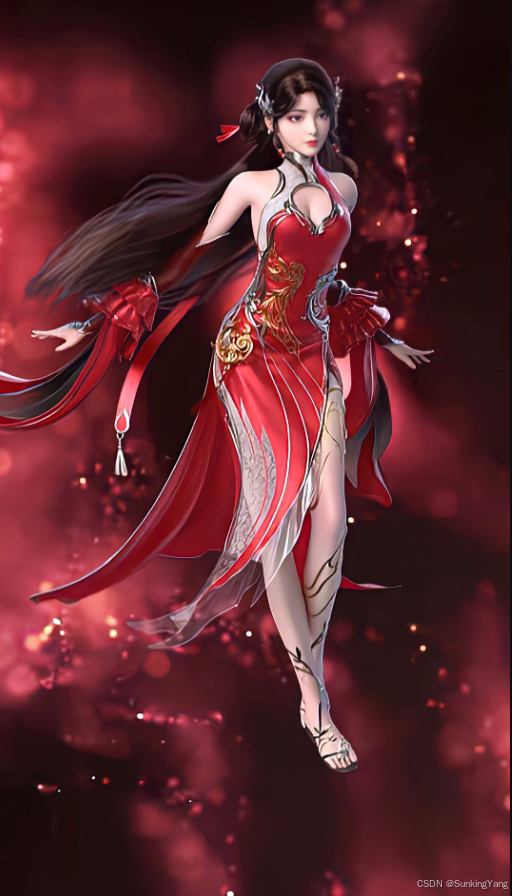