前言
欢迎来到我的博客
个人主页:北岭敲键盘的荒漠猫-CSDN博客
本文主要为学过多门语言玩家快速入门C++
没有基础的就放弃吧。
全部都是精华,看完能直接上手改别人的项目。
输出内容
std::代表了这里的cout使用的标准库,避免不同库中的相同命名导致混乱
std::cout <<输出内容右侧可以输出代码执行的结果,字符串,数字
可以多段<<进行拼接
std::endl等价于\n,表示结束语句换行
cpp
#include <iostream>
int main()
{
std::cout << 123+456<<"\n";
std::cout << "我喜欢"<< 1+1<<"\n";
std::cout << "Hello World!\n"<<"我还有一行哦"<< std::endl;
std::cout << "你好你好" << std::endl;
}
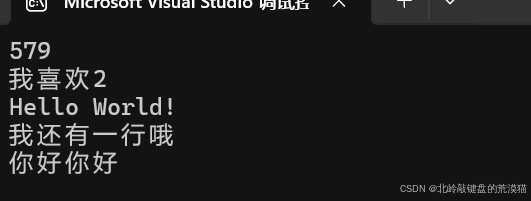
变量定义
cpp
#include <iostream>
#include <string>
int main()
{
int a = 5; //整形变量
std::string b = "Hello World"; //字符串变量
float c = 3.14; //浮点型变量
std::cout << a << "\n" << b << "\n" << c;
}
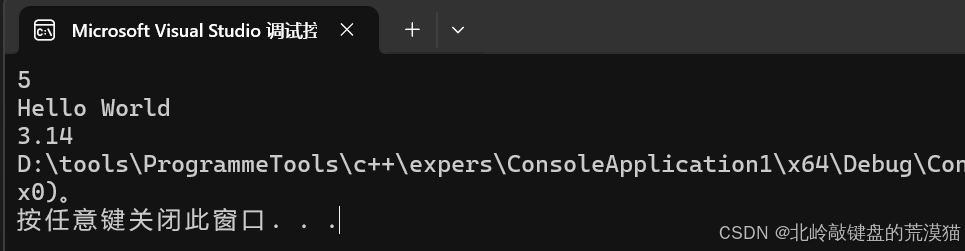
运算符
cpp
#include <iostream>
#include <string>
int main()
{
int a = 5; //整形变量
std::string b = "Hello World"; //字符串变量
float c = 3.14; //浮点型变量
std::cout << a + c << '\n'; //输出a+c的值
std::cout << a - c << '\n'; //输出a-c的值
std::cout << a * c << '\n'; //输出a*c的值
std::cout << a / c << '\n'; //输出a/c的值
std::cout << a % 2 << '\n'; //输出a对2取模的值
std::cout << a++ << '\n'; //输出a的值并将a加1
std::cout << a-- << '\n'; //输出a的值并将a减1
std::cout << ++a << '\n'; //输出a的值并将a加2
std::cout << --a << '\n'; //输出a的值并将a减2
}
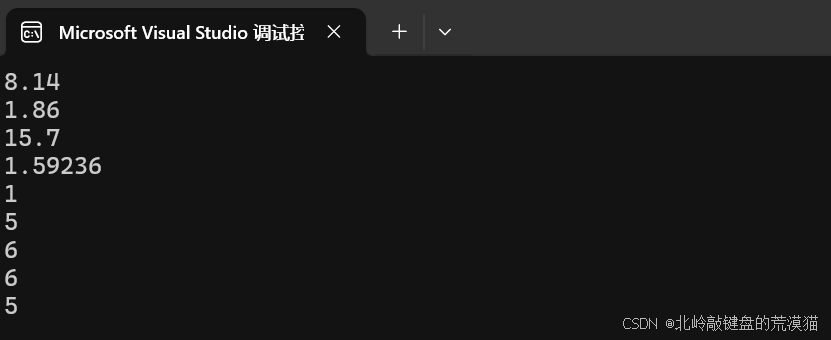
条件语句
if-else语句
cpp
#include <iostream>
int main()
{
int a = 5; //整形变量
if (a > 10) //判断a是否大于10
{
std::cout << "a大于10" << std::endl; //输出a大于10
}
else if (a == 10) //判断a是否等于10
{
std::cout << "a等于10" << std::endl; //输出a等于10
}
else //如果a不大于10也不等于10
{
std::cout << "a小于10" << std::endl; //输出a小于10
}
}

switch语句
cpp
#include <iostream>
int main()
{
int a = 5; //整形变量
switch (a)
{
case 1:
std::cout << "a=1" << std::endl;
break;
case 2:
std::cout << "a=2" << std::endl;
break;
case 3:
std::cout << "a=3" << std::endl;
break;
case 4:
std::cout << "a=4" << std::endl;
break;
case 5:
std::cout << "a=5" << std::endl;
break;
default:
std::cout << "a>5" << std::endl;
break;
}
}

循环语句
while循环
cpp
#include <iostream>
int main()
{
int a = 5; //整形变量
while (a < 10) {
std::cout << "a is " << a << std::endl;
a++;
}
}
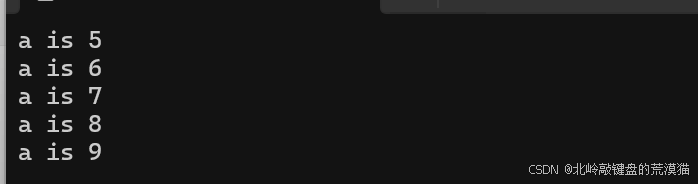
do-while循环
cpp
#include <iostream>
int main()
{
int a = 5; //整形变量
do
{
a += 10;
} while (a < 10);{
std::cout << "a is " << a << std::endl;
a++;
}
}

for循环
cpp
#include <iostream>
int main()
{
int a = 5; //整形变量
for (size_t i = 0; i < a; i++)
{
std::cout << "程序执行了 " << i + 1 << "次!" << std::endl;
}
}
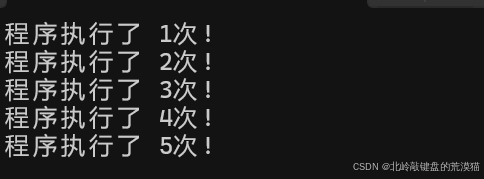
循环控制关键字
break;
continue
字符串类型变量
看一眼,然后遇到使用的情况直接查。
需要导入库
cpp
#include <string>
1. 创建与赋值
cpp
std::string s1; // 空字符串
std::string s2 = "Hello"; // 直接初始化
std::string s3(5, 'a'); // "aaaaa"(重复字符)
s1 = "World"; // 赋值
2. 拼接与追加
cpp
s2 += " World"; // 追加 → "Hello World"
s2.append("!"); // 追加 → "Hello World!"
s2.push_back('!'); // 追加单个字符
std::string s4 = s2 + "123"; // 新建拼接字符串
3. 访问元素
cpp
char c1 = s2[0]; // 下标访问(不检查越界)
char c2 = s2.at(1); // 安全访问(越界抛异常)
s2.front() = 'h'; // 首字符(C++11)
s2.back() = 'D'; // 末字符(C++11)
4. 长度与判空
cpp
int len = s2.size(); // 字符数量(等价length())
if (s2.empty()) { ... } // 是否为空
5. 查找与截取
cpp
size_t pos = s2.find("World"); // 首次出现位置(未找到返回npos)
std::string sub = s2.substr(6, 5); // 从位置6截取5字符 → "World"
6. 修改内容
cpp
s2.insert(5, " C++"); // 在位置5插入 → "Hello C++ World!!"
s2.replace(6, 3, "Hi"); // 替换位置6开始的3字符 → "Hello Hi World!!"
s2.erase(5, 4); // 删除位置5开始的4字符 → "Hello World!!"
s2.clear(); // 清空字符串
7. 输入输出
cpp
std::cout << s2; // 输出
std::getline(std::cin, s2); // 读取整行(包括空格)
8. 遍历字符
cpp
for (char ch : s2) { // 范围for循环(C++11)
std::cout << ch;
}
9. 比较与判断
cpp
if (s2 == "Hello") { ... } // 直接比较字符串
if (s2.starts_with("He")) { ... } // 是否以某前缀开头(C++20)
10. 大小写转换
cpp
#include <algorithm>
// 转大写
std::transform(s2.begin(), s2.end(), s2.begin(), ::toupper);
// 转小写
std::transform(s2.begin(), s2.end(), s2.begin(), ::tolower);
数组使用
1. 固定大小数组 → std::array
cpp
#include <array>
std::array<int, 3> arr = {1, 2, 3}; // 类型安全、可拷贝、自带大小
// 访问元素
arr[0] = 10; // 下标访问(无边界检查)
int val = arr.at(1); // 安全访问(越界抛异常)
// 遍历
for (int num : arr) { // 范围for循环(推荐)
std::cout << num;
}
// 常用操作
bool empty = arr.empty(); // 是否为空(固定数组总为false)
int size = arr.size(); // 元素数量(3)
2. 动态大小数组 → std::vector
cpp
#include <vector>
std::vector<int> vec = {1, 2, 3}; // 动态扩容、内存自动管理
// 添加元素
vec.push_back(4); // 尾部追加(拷贝)
vec.emplace_back(5); // 尾部追加(直接构造,更高效)
// 删除元素
vec.pop_back(); // 删除尾部元素
vec.erase(vec.begin()); // 删除指定位置元素
// 预分配内存(减少扩容开销)
vec.reserve(100); // 预留容量(不改变size)
// 遍历(同std::array)
for (int num : vec) {
std::cout << num;
}
3. 传统 C 风格数组(不推荐,仅了解)
cpp
int arr[3] = {1, 2, 3}; // 栈上分配,大小固定
int* arr_heap = new int[3]{4, 5, 6}; // 堆上分配(需手动delete[])
// 访问元素
arr[0] = 10; // 无越界检查(易崩溃)
// 遍历
for (int i = 0; i < 3; ++i) {
std::cout << arr[i];
}
// 缺点:无法直接获取大小、易内存泄漏、不支持拷贝等
4. 关键对比与推荐
特性 | std::array |
std::vector |
C 风格数组 |
---|---|---|---|
大小固定 | 是 | 否(动态扩容) | 是 |
内存管理 | 自动 | 自动 | 手动(堆分配) |
越界检查 | .at() 方法支持 |
.at() 方法支持 |
无 |
传递参数 | 可拷贝、引用传递 | 可拷贝、引用传递 | 退化为指针 |
适用场景 | 固定数量元素 | 动态增删元素 | 特殊需求 |
多用vector,固定数组大小的需求用array。
指针语法
考虑到指针是C类语言特点,可能别的语言没接触过看这个文章:
一、基础声明与初始化
1.指针声明
推荐写法:数据类型* 指针名
(*
紧贴类型,增强可读性)
cpp
int* pInt; // 推荐:强调int*是一个类型
double* pDouble; // 明确指向double的指针
2.初始化规范
cpp
int* p = new int(10); // 动态初始化(需配对delete)
- 必须初始化:
int val = 5; int* p = &val;
- 未指向对象时置空:
int* p = nullptr
二、核心操作
1.取地址(&)与解引用(*)
cpp
int num = 42;
int* p = # // &取地址
cout << *p; // *解引用 → 输出42
*p = 100; // 通过指针修改原值
2.动态内存管理(优先用智能指针)
-
手动管理(需严格配对)
cppint* arr = new int[5]{1,2,3}; // 动态数组 delete[] arr; // []必须与new[]配对
-
智能指针(推荐)
cpp#include <memory> std::unique_ptr<int> uPtr = std::make_unique<int>(10); // 独占所有权 std::shared_ptr<int> sPtr = std::make_shared<int>(20); // 共享所有权
三、常用场景规范
数组操作
-
数组名即首地址:
int arr[3] = {1,2,3}; int* p = arr;
-
传递数组到函数:
cppvoid printArray(int* arr, int size) { // 必须传大小 for(int i=0; i<size; ++i) cout << arr[i]; // 等价*(arr+i) }
函数参数传递
-
修改原对象时使用指针参数:
cppvoid swap(int* a, int* b) { int tmp = *a; *a = *b; *b = tmp; }
四、安全实践
const修饰
cpp
const int* p1; // 指向常量(值不可改)
int* const p2; // 指针常量(地址不可改)
const int* const p3; // 双常量
避免常见错误
cpp
int* p = new int(5);
delete p; // 正确释放
p = nullptr; // 避免野指针
- 禁止解引用空指针
- 数组越界访问
- 悬垂指针(释放后仍访问)
函数
函数定义:
写到主函数上面,或者声明函数。
要规定返回值。
常规写法
cpp
#include <iostream>
#include <string>
//写到上边
void student(){
std::string name = "张三";
int age = 18;
std::cout << "姓名:" << name << std::endl;
}
int main()
{
student();
}
cpp
#include <iostream>
#include <string>
//写到下面,但是声明
void student();
int main()
{
student();
}
void student() {
std::string name = "张三";
int age = 18;
std::cout << "姓名:" << name << std::endl;
}
cpp
#include <iostream>
#include <string>
//默认=李四及设置默认值
void student(std::string username="李四");
int main()
{
student("张三");
student();
}
void student(std::string username) {
std::string name = username;
int age = 18;
std::cout << "姓名:" << name << std::endl;
}
传址传参
函数内对变量的操作会直接影响到函数外的相应变量。
cpp
#include <iostream>
#include <string>
//默认=李四及设置默认值
void student(std::string &username);
int main()
{
std::string name = "张三";
student(name);
std::cout << name << std::endl;
}
void student(std::string &username) {
username = "李四";
}
函数重载
相同函数名,不同返回值,不同参数类型,根据传参执行对应函数。
cpp
#include <iostream>
#include <string>
//默认=李四及设置默认值
void student(std::string& username);
void student(int& name);
int main()
{
std::string name = "张三";
student(name);
std::cout << name << std::endl;
int age = 1;
student(age);
std::cout << age << std::endl;
}
void student(std::string &username) {
username = "李四";
}
void student(int &name) {
name = 789;
}
数学计算
大体看一遍!都带好了目录,要用的时候直接点到对应位置查阅!!
使用需要导入cmath库
cpp
#include <cmath>
1. 基础计算
cpp
double a = std::abs(-3.5); // 绝对值 → 3.5(兼容整型/浮点)
double b = std::fmod(7.5, 3); // 浮点取余 → 1.5(处理循环进度)
2. 幂与根
cpp
double p = std::pow(2, 3); // 2的3次方 → 8(如计算缩放比例)
double s = std::sqrt(16); // 平方根 → 4(如物理引擎计算距离)
double c = std::cbrt(27); // 立方根 → 3(3D空间计算)
3. 取整处理
cpp
double up = std::ceil(3.2); // 向上取整 → 4(如分页计算)
double down = std::floor(3.8); // 向下取整 → 3(网格对齐)
double rd = std::round(3.5); // 四舍五入 → 4(UI显示)
double cut = std::trunc(3.8); // 截断小数 → 3(取整数部分)
4. 浮点数检查
cpp
bool nan = std::isnan(0.0/0.0); // 是否为非数字(NaN检查)
bool inf = std::isinf(1e300); // 是否为无穷大(溢出处理)
bool valid = std::isfinite(3.14); // 是否为有效数(安全校验)
5. 极值与运算
cpp
double max_v = std::fmax(3, 5); // 最大值 → 5(限制范围)
double min_v = std::fmin(3, 5); // 最小值 → 3(约束边界)
double dist = std::hypot(3, 4); // 直角斜边 → 5(2D/3D距离计算)
6. 特殊值生成
cpp
double nan_val = NAN; // 表示非数字(需C++11)
double inf_val = INFINITY; // 正无穷大(如超阈值标记)
面向对象
基础创建与公有私有
创建类分为公共和私有,公共能在类外使用,私有仅能在类内使用。
cpp
#include <iostream>
#include <string>
class MyClass
{
public:
std::string name="张三";
int age = 18;
private:
std::string address = "北京";
int phone = 123456789;
};
int main()
{
MyClass i;
std::cout << i.name << std::endl;
std::cout << i.age << std::endl;
//std::cout << i.address << std::endl;
}
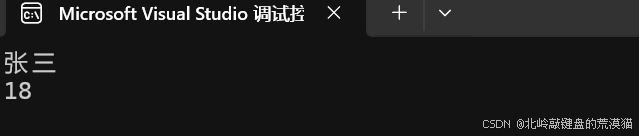
std::cout << i.address << std::endl;
使用了私有的属性,无法调用。
方法定义
cpp
#include <iostream>
#include <string>
class MyClass
{
public:
std::string name="张三";
int age = 18;
void print()
{
std::cout << "姓名:" << name << std::endl;
std::cout << "年龄:" << age << std::endl;
}
private:
std::string address = "北京";
int phone = 123456789;
};
int main()
{
MyClass i;
i.print();
}
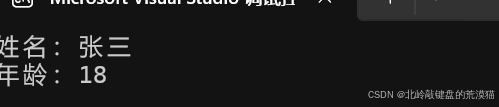
外部实现
内部定义函数,外部实现函数。
cpp
#include <iostream>
#include <string>
class MyClass
{
public:
std::string name="张三";
int age = 18;
void print();
private:
std::string address = "北京";
int phone = 123456789;
};
void MyClass::print()
{
std::cout << "姓名:" << name << std::endl;
std::cout << "年龄:" << age << std::endl;
}
int main()
{
MyClass i;
i.print();
}
构造函数
与类名相同的方法,当我们创建对象的时候就会执行方法。
实现的时候不要写返回值类型。
cpp
#include <iostream>
#include <string>
class MyClass
{
public:
std::string name;
int age;
MyClass(std::string name,int age);
void printInfos();
private:
std::string address = "北京";
int phone = 123456789;
};
MyClass::MyClass(std::string name, int age)
{
this->name = name;
this->age = age;
}
void MyClass::printInfos()
{
std::cout << "姓名:" << name << std::endl;
std::cout << "年龄:" << age << std::endl;
}
int main()
{
MyClass i("张三",18);
i.printInfos();
}
类继承
可以多继承
cpp
#include <iostream>
#include <string>
class Animal
{
public:
int age = 10;
private:
};
class Animal2
{
public:
int age2 = 11;
private:
};
class Dog : public Animal, public Animal2
{
public:
std::string name = "dog";
};
void main()
{
Dog dog;
std::cout << dog.age << std::endl;
std::cout << dog.name << std::endl;
std::cout << dog.age2 << std::endl;
}
类多态
子类可以修改父类方法
cpp
#include <iostream>
#include <string>
class Animal
{
public:
void print()
{
std::cout << "i am Animal" << std::endl;
}
private:
};
class Cat : public Animal
{
public:
void print()
{
std::cout << "i am Cat" << std::endl;
}
private:
};
class Dog : public Animal
{
public:
void print()
{
std::cout << "i am Dog" << std::endl;
}
};
void main()
{
Dog dog;
Cat cat;
dog.print();
cat.print();
}
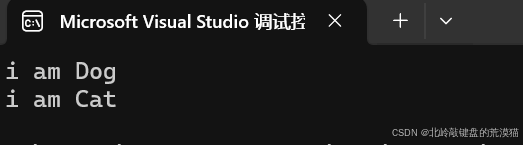
文件操作
文件的创建与写入。
1. 核心文件流类
#include <fstream>
ofstream
: 写入文件(输出文件流)ifstream
: 读取文件(输入文件流)fstream
: 读写文件(需指定模式)
2. 基本文件操作
打开/关闭文件
// 写入文件
ofstream outFile("data.txt"); // 默认覆盖模式
outFile.close();
// 读取文件
ifstream inFile("data.txt");
inFile.close();
// 指定打开模式(如追加)
ofstream logFile("log.txt", ios::app);
常用打开模式
模式标志 | 说明 |
---|---|
ios::in |
读模式(默认ifstream) |
ios::out |
写模式(默认ofstream) |
ios::app |
追加写入(不覆盖原内容) |
ios::binary |
二进制模式 |
3. 文本文件操作
写入文本
cpp
ofstream out("data.txt");
if (out.is_open()) {
out << "Line 1" << endl; // 写入并换行
out << "Value: " << 42 << "\n";// 混合数据类型
}
读取文本
cpp
ifstream in("data.txt");
string line;
int value;
if (in) {
while (getline(in, line)) { // 逐行读取
cout << line << endl;
}
in.seekg(0); // 重置文件指针到开头
in >> value; // 读取单个值(空格分隔)
}
4. 二进制文件操作
写入二进制数据
cpp
struct Person {
char name[20];
int age;
};
Person p = {"Alice", 30};
ofstream out("data.bin", ios::binary);
if (out) {
out.write((char*)&p, sizeof(Person)); // 直接写入内存数据
}
读取二进制数据
cpp
ifstream in("data.bin", ios::binary);
Person p;
if (in) {
in.read((char*)&p, sizeof(Person)); // 读取到内存
cout << p.name << " " << p.age << endl;
}
5. 文件指针控制
cpp
fstream file("data.txt");
// 获取当前读指针位置
streampos pos = file.tellg();
// 移动读指针到第10字节
file.seekg(10, ios::beg);
// 移动写指针到文件末尾
file.seekp(0, ios::end);