一、在阿里云服务器上使用talk程序
Linux系统自带的talk
命令可以让两个登录用户进行实时文字聊天:
- 用户A执行:`talk usernameB
- 用户B会收到通知,并需要执行:
talk usernameA@hostname
- 然后双方就可以开始聊天了,屏幕会分成上下两部分
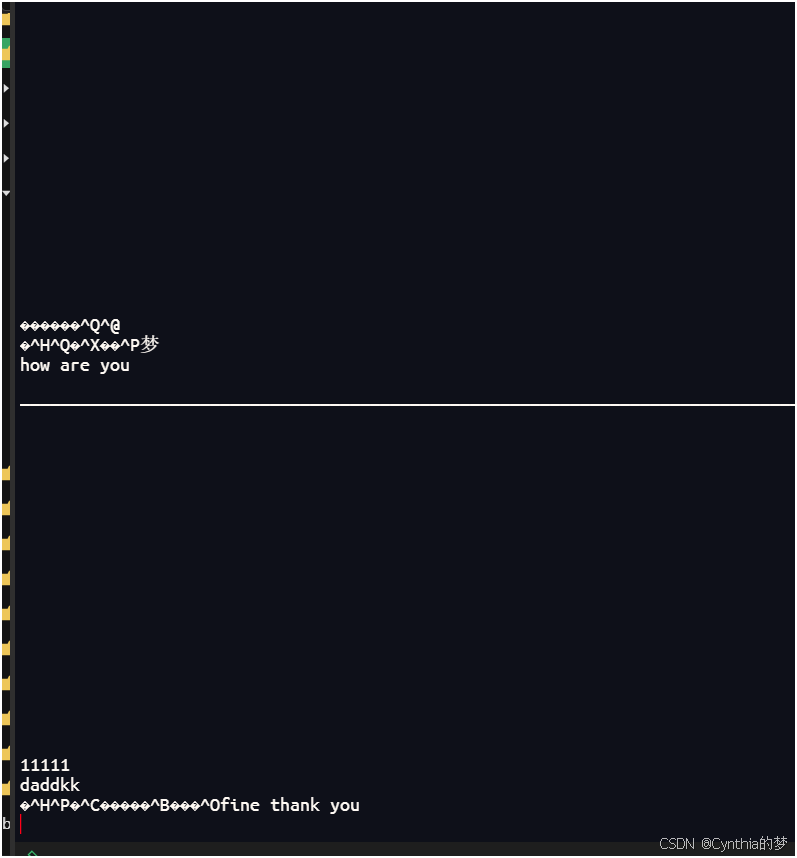
二、用C语言实现简单的进程间通信聊天程序
下面我将展示几种不同的IPC方式实现的简单聊天程序。
1. 使用命名管道(FIFO)
server.c (服务器端)
c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <string.h>
#include <stddef.h> // 添加 size_t 的定义
#define FIFO_FILE "chat_fifo"
int main() {
int fd;
char readbuf[80];
char end[10];
int to_end;
// 创建命名管道
mkfifo(FIFO_FILE, 0666);
while(1) {
fd = open(FIFO_FILE, O_RDONLY);
read(fd, readbuf, sizeof(readbuf));
printf("Client: %s\n", readbuf);
close(fd);
printf("Server: ");
fgets(readbuf, sizeof(readbuf), stdin);
strcpy(end, "quit\n");
to_end = strcmp(readbuf, end);
if (to_end == 0) {
fd = open(FIFO_FILE, O_WRONLY);
write(fd, readbuf, strlen(readbuf));
close(fd);
break;
}
fd = open(FIFO_FILE, O_WRONLY);
write(fd, readbuf, strlen(readbuf));
close(fd);
}
unlink(FIFO_FILE); // 删除FIFO文件
return 0;
}
client.c (客户端)
c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <string.h>
#include <stddef.h> // 添加 size_t 的定义
#define FIFO_FILE "chat_fifo"
int main() {
int fd;
char readbuf[80];
char end[10];
int to_end;
while(1) {
printf("Client: ");
fgets(readbuf, sizeof(readbuf), stdin);
strcpy(end, "quit\n");
to_end = strcmp(readbuf, end);
fd = open(FIFO_FILE, O_WRONLY);
write(fd, readbuf, strlen(readbuf));
close(fd);
if (to_end == 0) {
break;
}
fd = open(FIFO_FILE, O_RDONLY);
read(fd, readbuf, sizeof(readbuf));
printf("Server: %s\n", readbuf);
close(fd);
}
return 0;
}
三、编译和运行
命名管道版本
-
编译:
bashgcc server.c -o server gcc client.c -o client
-
在两个不同的终端中分别运行:
bash./server ./client