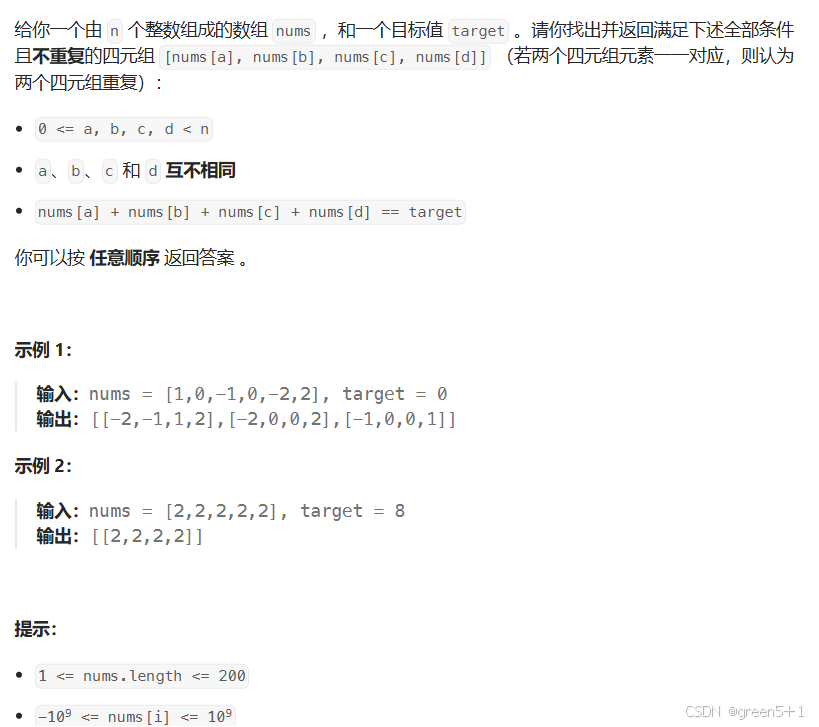
代码来源:代码随想录
c
/**
* Return an array of arrays of size *returnSize.
* The sizes of the arrays are returned as *returnColumnSizes array.
* Note: Both returned array and *columnSizes array must be malloced, assume caller calls free().
*/
int compare(const void *a,const void *b)
{
int a1=*(int *)a;
int b1=*(int *)b;
return (a1>b1)-(a1<b1);
}
int** fourSum(int* nums, int numsSize, int target, int* returnSize, int** returnColumnSizes)
{
//1.对数组进行排序
qsort(nums,numsSize,sizeof(int),compare);
//2.分配空间,解的数量上限是C(200 4),但经验值为40000,避免过度分配内存
int **result=(int **)malloc(sizeof(int *)*40000);
int count=0;//计数器,记录解的数量
for(int i=0;i<numsSize-3;i++)
{
/* 剪枝:如果nums[i]已经大于target且为正数,后面不可能有解 */
if ((nums[i] > target) && (nums[i] >= 0)) {
break;
}
/* 去重:跳过重复的nums[i] */
if ((i > 0) && (nums[i] == nums[i - 1])) {
continue;
}
for(int j=i+1;j<numsSize-2;j++)
{
/* 剪枝:如果前两数之和已经大于target且nums[j]为正数 */
if ((nums[i] + nums[j] > target) && (nums[j] >= 0)) {
break;
}
/* 去重:跳过重复的nums[i] */
if ((j > (i + 1)) && (nums[j] == nums[j - 1])) {
continue;
}
//左指针
int left=j+1;
//右指针
int right=numsSize-1;
while(left<right)
{
long long sum=(long long)nums[i]+nums[j]+nums[left]+nums[right];
if(sum>target)
{
right--;
}
else if(sum<target)
{
left++;
}
else
{
//3.找到符合条件的四元组记录到result中
int *result_tmp=(int *)malloc(sizeof(int)*4);
result_tmp[0]=nums[i];
result_tmp[1]=nums[j];
result_tmp[2]=nums[left];
result_tmp[3]=nums[right];
result[count++]=result_tmp;
//去重,去掉重复的left和right
while ((right > left) && (nums[right] == nums[right - 1])) {
right--;
}
while ((left < right) && (nums[left] == nums[left + 1])) {
left++;
}
right--;
left++;
}
}
}
}
*returnSize=count;
int *column=(int *)malloc(sizeof(int)*count);
for(int i=0;i<count;i++)
{
column[i]=4;
}
*returnColumnSizes=column;
return result;
}