文章目录
使用lodashjs库
html
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>页面标题</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/lodash.js/4.17.21/lodash.min.js"></script>
<!-- <link rel="stylesheet" href="styles.css"> -->
<!-- <script src="script.js" defer></script> -->
<style>
.box {
width: 200px;
/* 设置盒子的宽度 */
height: 200px;
/* 设置盒子的高度 */
background-color: lightblue;
/* 设置盒子的背景颜色 */
border: 1px solid #000;
/* 可选: 添加边框 */
display: flex;
/* 使用 flexbox 布局 */
justify-content: center;
/* 水平居中内容 */
align-items: center;
/* 垂直居中内容 */
font-size: 24px;
/* 设置字体大小 */
}
</style>
</head>
<body>
<div class="box"></div> <!-- 创建一个盒子元素 -->
<script>
const box = document.querySelector('.box');
let i = 1;
function mouseMove() {
box.innerHTML = i++; // 显示当前计数器值
console.log(i);
}
// 使用 lodash 的 throttle 函数进行节流
box.addEventListener('mousemove', _.throttle(mouseMove, 3000)); // 每3000毫秒调用一次
</script>
</body>
</html>
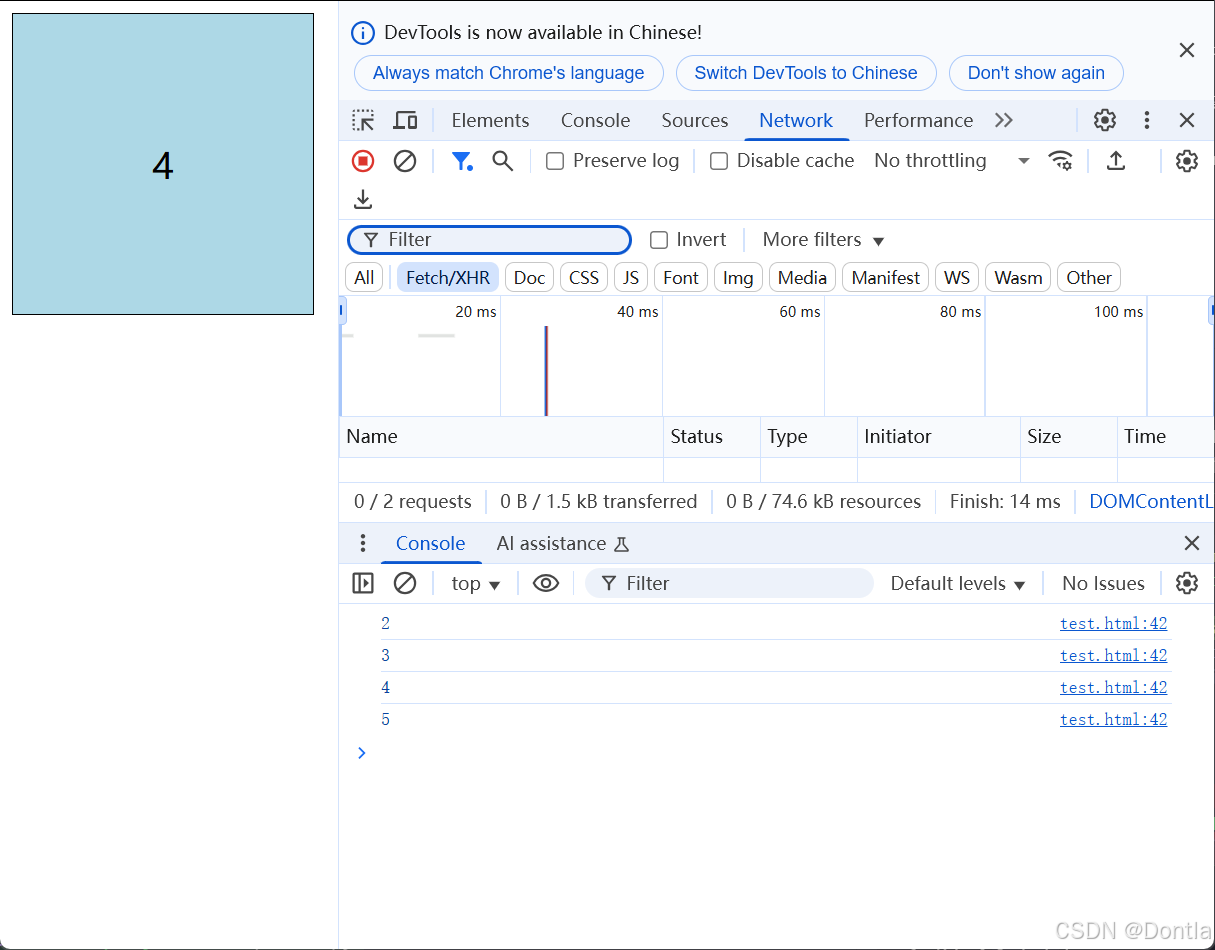
手动实现节流(通过判断之前设定的定时器setTimeout是否存在)
html
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>页面标题</title>
<!-- <link rel="stylesheet" href="styles.css"> -->
<!-- <script src="script.js" defer></script> -->
<style>
.box {
width: 200px;
/* 设置盒子的宽度 */
height: 200px;
/* 设置盒子的高度 */
background-color: lightblue;
/* 设置盒子的背景颜色 */
border: 1px solid #000;
/* 可选: 添加边框 */
display: flex;
/* 使用 flexbox 布局 */
justify-content: center;
/* 水平居中内容 */
align-items: center;
/* 垂直居中内容 */
font-size: 24px;
/* 设置字体大小 */
}
</style>
</head>
<body>
<div class="box"></div> <!-- 创建一个盒子元素 -->
<script>
const box = document.querySelector('.box');
let i = 1;
function mouseMove() {
box.innerHTML = i++; // 显示当前计数器值
console.log(i);
}
function throttle(fn, delay) {
let timer = null;
return function () {
console.log('判断定时器是否为空?', timer);
if (!timer) {
console.log('定时器为空,延迟执行');
timer = setTimeout(() => {
fn(); // 在里面就是延迟执行,在外面就是立即执行
timer = null;
}, delay);
}
console.log('不执行');
}
}
// 使用 lodash 的 throttle 函数进行节流
box.addEventListener('mousemove', throttle(mouseMove, 3000)); // 每3000毫秒调用一次
</script>
</body>
</html>
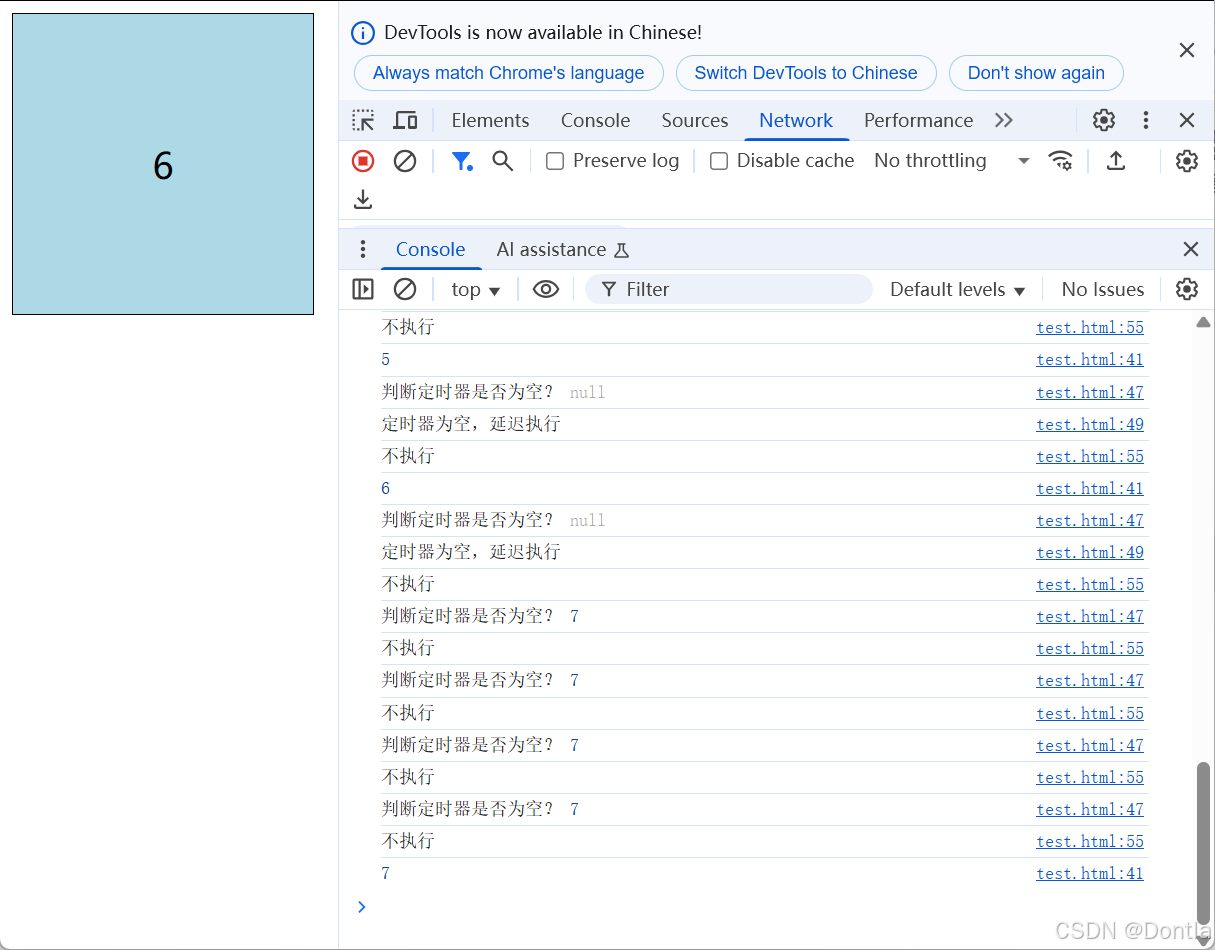