2999. 统计强大整数的数目
问题
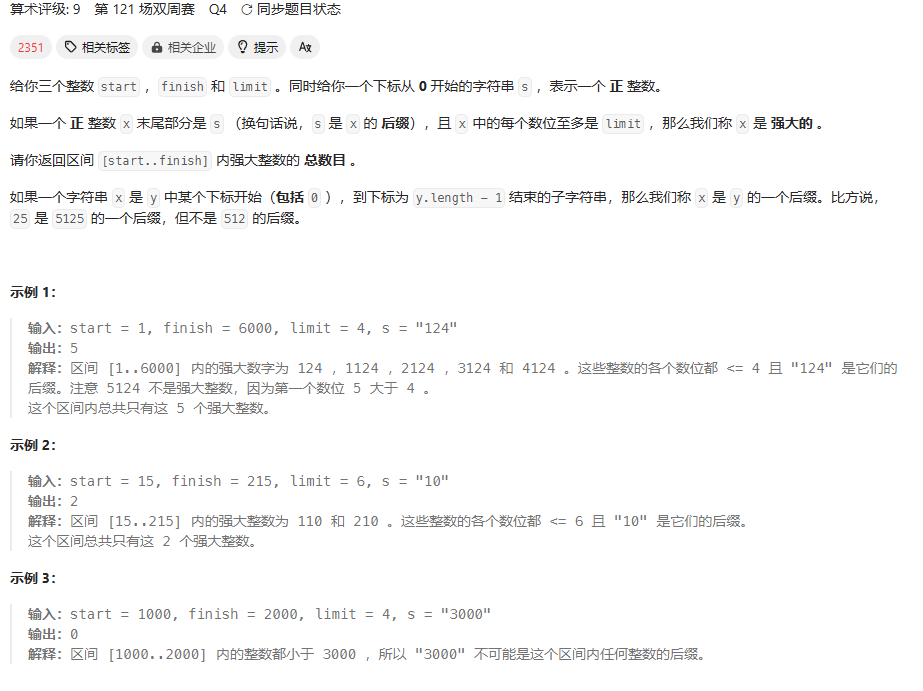
问题分析
题目要求统计区间 [start, finish] 内满足以下条件的整数数目:
整数的每个数位都小于等于 limit。
整数的末尾部分是字符串 s(即 s 是该整数的后缀)。
关键点:
后缀匹配:需要判断一个整数是否以字符串 s 结尾。
数位限制:每个数位必须小于等于 limit。
区间范围:统计的整数必须在 [start, finish] 范围内。
思路
遍历区间:从 start 到 finish,逐个检查每个整数是否满足条件。
后缀匹配:将整数转换为字符串,检查其是否以 s 结尾。
数位限制:检查整数的每个数位是否小于等于 limit。
计数:统计满足条件的整数数量。
代码
python
class Solution:
def numberOfPowerfulInt(self, start: int, finish: int, limit: int, s: str) -> int:
count = 0
# 遍历区间 [start, finish]
for num in range(start, finish + 1):
num_str = str(num)
# 检查是否以 s 结尾
if not num_str.endswith(s):
continue
# 检查每个数位是否 <= limit
if all(int(digit) <= limit for digit in num_str):
count += 1
return count
复杂度分析
时间复杂度:
遍历区间 [start, finish],假设区间长度为 n,每次检查整数的后缀和数位需要 O(k),其中 k 是整数的位数。总时间复杂度为 O(n * k)。(超时)
空间复杂度:
仅使用常量级别的额外空间,空间复杂度为 O(1)。
学习
困难题还是不会做,参考学习
优化时间复杂度:
python
class Solution:
def numberOfPowerfulInt(self, start: int, finish: int, limit: int, s: str) -> int:
high = list(map(int, str(finish))) # 避免在 dfs 中频繁调用 int()
n = len(high)
low = list(map(int, str(start).zfill(n))) # 补前导零,和 high 对齐
diff = n - len(s)
@cache
def dfs(i: int, limit_low: bool, limit_high: bool) -> int:
if i == n:
return 1
# 第 i 个数位可以从 lo 枚举到 hi
# 如果对数位还有其它约束,应当只在下面的 for 循环做限制,不应修改 lo 或 hi
lo = low[i] if limit_low else 0
hi = high[i] if limit_high else 9
res = 0
if i < diff: # 枚举这个数位填什么
for d in range(lo, min(hi, limit) + 1):
res += dfs(i + 1, limit_low and d == lo, limit_high and d == hi)
else: # 这个数位只能填 s[i-diff]
x = int(s[i - diff])
if lo <= x <= hi: # 题目保证 x <= limit,无需判断
res = dfs(i + 1, limit_low and x == lo, limit_high and x == hi)
return res
return dfs(0, True, True)

学习来源:
作者:灵茶山艾府
来源:力扣(LeetCode)