本文原创发布在华为开发者社区。
介绍
本示例展示了如何使用PDFKit提供的能力进行PDF文件内容查看与编辑等相关操作,相关能力通过PDFKit服务接口@kit.PDFKit引入。
效果预览
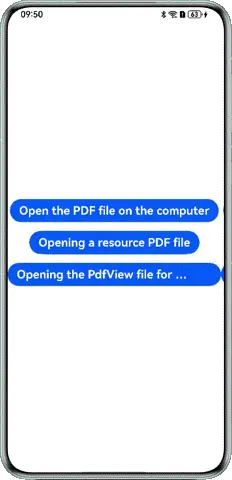
使用说明
进入页面后,
- 点击'Open the PDF file on the computer',通过picker打开本地pdf文件。
- 点击'Opening a resource PDF file',打开rawfile中的pdf文件。
- 点击'Opening the PdfView file for preview',打开pdf预览功能。
实现思路
通过pdfService实现对PDF文档的操作
- 打开PDF文档
typescript
let filePath = '/data/storage/el2/base/haps/View/files/input.pdf';
let document: pdfService.PdfDocument = new pdfService.PdfDocument();
document.loadDocument(filePath);
- 将PDF转换为图片
typescript
let path: string = getContext().filesDir + "/outputImg/";
fs.mkdir(path);
const result: boolean = this.document.convertToImage(path, 0);
- 添加页眉页脚
typescript
let hfInfo: pdfService.HeaderFooterInfo = new pdfService.HeaderFooterInfo();
hfInfo.fontInfo = new pdfService.FontInfo();
hfInfo.fontInfo.fontPath = font.getFontByName("HarmonyOS Sans")?.path; // 确保字体路径存在
hfInfo.fontInfo.fontName = '';
hfInfo.textSize = 10;
hfInfo.charset = pdfService.CharsetType.PDF_FONT_DEFAULT_CHARSET;
hfInfo.underline = false;
hfInfo.textColor = 0x00000000;
hfInfo.leftMargin = 1.0;
hfInfo.topMargin = 40.0;
hfInfo.rightMargin = 1.0;
hfInfo.bottomMargin = 40.0;
hfInfo.headerLeftText = "left H <<dd.mm.yyyy>> <<1/n>>";
hfInfo.headerCenterText = "center H <<m/d/yyyy>> <<1/n>>";
hfInfo.headerRightText = "right H <<m/d>><<1>>";
hfInfo.footerLeftText = "left F <<m/d>><<1>>";
hfInfo.footerCenterText = "center F <<m/d>><<1>>";
hfInfo.footerRightText = "right F <<dd.mm.yyyy>><<1>>";
this.document.addHeaderFooter(hfInfo, 1, 5, true, true);
- 添加水印
typescript
let wminfo: pdfService.TextWatermarkInfo = new pdfService.TextWatermarkInfo();
wminfo.watermarkType = pdfService.WatermarkType.WATERMARK_TEXT;
wminfo.content = "This is Watermark";
wminfo.textSize = 30;
wminfo.textColor = 200;
wminfo.fontInfo = new pdfService.FontInfo();
// 确保字体路径存在
wminfo.fontInfo.fontPath = font.getFontByName("HarmonyOS Sans").path;
wminfo.opacity = 0.5;
wminfo.isOnTop = true;
wminfo.rotation = 45;
wminfo.scale = 1.5;
wminfo.opacity = 0.5;
wminfo.verticalAlignment = pdfService.WatermarkAlignment.WATERMARK_ALIGNMENT_TOP;
wminfo.horizontalAlignment = pdfService.WatermarkAlignment.WATERMARK_ALIGNMENT_LEFT;
wminfo.horizontalSpace = 1.0;
wminfo.verticalSpace = 1.0;
this.document.addWatermark(wminfo, 0, 18, true, true);
- 添加页面背景图片
typescript
let bginfo: pdfService.BackgroundInfo = new pdfService.BackgroundInfo();
bginfo.imagePath = this.backGroundImgPath = getContext().filesDir + "/background.png";
bginfo.backgroundColor = 50;
bginfo.isOnTop = true;
bginfo.rotation = 45;
bginfo.scale = 0.5;
bginfo.opacity = 0.3;
bginfo.verticalAlignment = pdfService.BackgroundAlignment.BACKGROUND_ALIGNMENT_TOP;
bginfo.horizontalAlignment = pdfService.BackgroundAlignment.BACKGROUND_ALIGNMENT_LEFT;
bginfo.horizontalSpace = 1.0;
bginfo.verticalSpace = 1.0;
this.document.addBackground(bginfo, 2, 18, true, true);
- 添加书签
typescript
let mark1: pdfService.Bookmark = this.document.createBookmark();
let mark2: pdfService.Bookmark = this.document.createBookmark();
this.document.insertBookmark(mark1, null, 1);
this.document.insertBookmark(mark2, mark1, 1);
let destInfo: pdfService.DestInfo = mark1.getDestInfo();
destInfo.fitMode = pdfService.FitMode.FIT_MODE_XYZ;
destInfo.pageIndex = 1;
destInfo.left = 20;
destInfo.top = 30;
destInfo.zoom = 1.5;
mark1.setDestInfo(destInfo);
let bookInfo: pdfService.BookmarkInfo = mark1.getBookmarkInfo();
bookInfo.title = "这里是跳到第一页的书签";
bookInfo.titleColor = 12;
bookInfo.isBold = true;
bookInfo.isItalic = true;
mark1.setBookmarkInfo(bookInfo);
通过PdfView组件实现对PDF文档丰富的预览能力
- 打开pdf文件
typescript
let context = getContext() as common.UIAbilityContext;
let dir = context.filesDir;
let filePath = dir + `/input.pdf`;
this.controller = new pdfViewManager.PdfController();
let loadResult: pdfService.ParseResult = await this.controller.loadDocument(filePath);
PdfView({
controller: this.controller,
pageFit: pdfService.PageFit.FIT_WIDTH,
showScroll: true
})
.id('pdfview_app_view')
.layoutWeight(1)
- 设置预览方式
typescript
let context = getContext() as common.UIAbilityContext;
let dir = context.filesDir;
let filePath = dir + `/input.pdf`;
this.controller = new pdfViewManager.PdfController();
let loadResult: pdfService.ParseResult = await this.controller.loadDocument(filePath);
PdfView({
controller: this.controller,
pageFit: pdfService.PageFit.FIT_WIDTH,
showScroll: true
})
.id('pdfview_app_view')
.layoutWeight(1)
- 设置监听
typescript
// 文本选中监听回调
this.controller.registerTextSelectedListener((textSelect: pdfViewManager.TextSelectedParam) => {
hilog.info(0x0000, '', 'registerTextSelectedListener'+textSelect.text);
});
// 图片选中监听回调
this.controller.registerImageSelectedListener((imageSelect: pdfViewManager.ImageSelectedParam) => {
hilog.info(0x0000, '', 'registerImageSelectedListener'+imageSelect.toString());
});
// 页码变化监听回调
this.controller.registerPageChangedListener((pageIndex: number) => {
hilog.info(0x0000, '', 'registerPageChangedListener'+pageIndex.toString());
});
- 搜索关键字
typescript
this.controller.searchKey(this.inputStr, (index: number) => {
hilog.info(0x0000, '', 'searchKey result index:'+index);
});