大家好,我是 V 哥。 在鸿蒙 NEXT 开发中,ArkUI 提供了丰富的 3D 变换和手势事件功能,可用于创建生动且交互性强的用户界面。下面详细介绍 ArkUI 的 3D 变换和手势事件,并给出相应的 ArkTS 案例代码。
1. ArkUI 3D 变换
ArkUI 支持多种 3D 变换效果,如旋转、缩放、平移等。通过设置组件的 transform
属性,能实现不同的 3D 变换效果。
常见的 3D 变换属性
rotateX(angle)
:绕 X 轴旋转指定角度。rotateY(angle)
:绕 Y 轴旋转指定角度。rotateZ(angle)
:绕 Z 轴旋转指定角度。scale3d(x, y, z)
:在 X、Y、Z 三个方向上进行缩放。translate3d(x, y, z)
:在 X、Y、Z 三个方向上进行平移。
2. 手势事件
ArkUI 支持多种手势事件,如点击、长按、滑动等。通过监听这些手势事件,能为用户交互提供反馈。
常见的手势事件
onClick()
:点击事件。onLongPress()
:长按事件。onSwipe()
:滑动事件。
3. 案例代码
下面是一个结合 3D 变换和手势事件的完整 ArkTS 案例代码:
typescript
@Entry
@Component
struct Gesture3DExample {
private rotationX: number = 0
private rotationY: number = 0
private scale: number = 1
build() {
Stack({ alignContent: Alignment.Center }) {
Image($r('app.media.example_image'))
.width(200)
.height(200)
.transform({
rotateX: this.rotationX,
rotateY: this.rotationY,
scale3d: [this.scale, this.scale, this.scale]
})
.onClick(() => {
this.rotationX += 10
this.rotationY += 10
})
.onLongPress(() => {
this.scale = this.scale === 1 ? 1.5 : 1
})
.onSwipe((event: SwipeEvent) => {
if (event.direction === SwipeDirection.Left) {
this.rotationY -= 10
} else if (event.direction === SwipeDirection.Right) {
this.rotationY += 10
} else if (event.direction === SwipeDirection.Up) {
this.rotationX -= 10
} else if (event.direction === SwipeDirection.Down) {
this.rotationX += 10
}
})
}
.width('100%')
.height('100%')
}
}
4. 代码解释
- 3D 变换 :借助
transform
属性对Image
组件进行 3D 变换,rotateX
和rotateY
实现绕 X 轴和 Y 轴的旋转,scale3d
实现缩放效果。 - 手势事件 :
onClick()
:每次点击图片时,图片绕 X 轴和 Y 轴各旋转 10 度。onLongPress()
:长按图片时,图片在原始大小和 1.5 倍大小之间切换。onSwipe()
:根据滑动方向,对图片进行不同的旋转操作。
通过上述代码,你可以实现一个具备 3D 变换和手势交互功能的图片组件。
如何在实际项目中应用3D变换和手势事件来提升用户体验?
在实际项目中应用 3D 变换和手势事件可以显著提升用户体验,让应用更加生动、交互性更强。以下是在不同类型项目中应用 3D 变换和手势事件的具体方法:
电商类应用
商品展示
- 3D 旋转展示:商品详情页里,使用 3D 旋转让用户全方位查看商品。例如,用户可通过左右滑动屏幕使商品绕 Y 轴旋转,上下滑动则让商品绕 X 轴旋转。这样用户能从不同角度观察商品细节,就像在实体店中亲手转动商品一样。
- 缩放查看细节:用户双指缩放屏幕时,商品模型可进行 3D 缩放。放大时能看清商品纹理、材质等细节,缩小时可整体把握商品外观。
- 案例代码示例:
typescript
@Entry
@Component
struct ProductDetail {
private rotationX: number = 0
private rotationY: number = 0
private scale: number = 1
build() {
Stack({ alignContent: Alignment.Center }) {
Image($r('app.media.product_image'))
.width(300)
.height(300)
.transform({
rotateX: this.rotationX,
rotateY: this.rotationY,
scale3d: [this.scale, this.scale, this.scale]
})
.onSwipe((event: SwipeEvent) => {
if (event.direction === SwipeDirection.Left) {
this.rotationY -= 10
} else if (event.direction === SwipeDirection.Right) {
this.rotationY += 10
} else if (event.direction === SwipeDirection.Up) {
this.rotationX -= 10
} else if (event.direction === SwipeDirection.Down) {
this.rotationX += 10
}
})
.onPinch((event: PinchEvent) => {
this.scale = event.scale
})
}
.width('100%')
.height('100%')
}
}
商品列表交互
- 卡片翻转效果:商品列表的卡片式布局中,用户点击卡片时,卡片可进行 3D 翻转展示商品更多信息,如价格、评价等。这种交互方式能在有限空间内展示更多内容,增加页面的信息密度。
游戏类应用
角色控制
- 手势控制移动:在角色扮演游戏或动作游戏中,玩家可通过滑动屏幕控制角色在 3D 场景中移动。比如,向左滑动角色向左移动,向上滑动角色向前奔跑等。
- 旋转视角:玩家双指旋转屏幕可改变游戏视角,从不同角度观察游戏场景和角色。这能增强游戏的沉浸感,让玩家更好地掌握游戏局势。
- 案例代码示例:
typescript
@Entry
@Component
struct GameScene {
private playerX: number = 0
private playerY: number = 0
private cameraRotation: number = 0
build() {
Canvas({
onDraw: (canvas: CanvasContext) => {
// 绘制游戏场景和角色
// ...
}
})
.width('100%')
.height('100%')
.onSwipe((event: SwipeEvent) => {
if (event.direction === SwipeDirection.Left) {
this.playerX -= 10
} else if (event.direction === SwipeDirection.Right) {
this.playerX += 10
} else if (event.direction === SwipeDirection.Up) {
this.playerY -= 10
} else if (event.direction === SwipeDirection.Down) {
this.playerY += 10
}
})
.onRotate((event: RotateEvent) => {
this.cameraRotation += event.angle
})
}
}
道具交互
- 3D 拾取效果:玩家点击场景中的道具时,道具以 3D 动画效果被拾取到背包中,如道具旋转、放大后消失。这种视觉反馈能让玩家更直观地感受到道具的获取过程。
设计注意事项
- 适度使用:虽然 3D 变换和手势事件能提升用户体验,但过度使用可能导致界面过于复杂,影响性能和用户操作。应根据项目需求和用户群体合理选择应用场景。
- 反馈清晰:在用户进行手势操作时,要及时给出清晰的视觉反馈,让用户明确操作的结果。例如,在 3D 旋转时,添加旋转动画效果;在缩放时,显示缩放比例变化。
- 兼容性:确保在不同设备和屏幕尺寸上都能正常显示和交互,进行充分的测试和优化。
欢迎关注威哥爱编程,鸿蒙开发就你行。V 哥的第一本鸿蒙 NEXT教材已经出版了《鸿蒙 HarmonyOS NEXT 开发之路 卷1 ArkTS篇》,如果你是小白,这本书可以快速帮助你入门 ArkTS。另外两本也正在加紧印刷中。
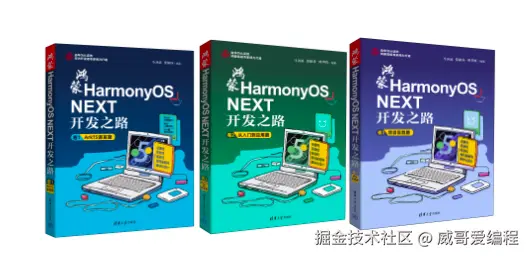