引言----感谢大佬的讲解
原型链示意图
原型链问题中需要记住一句话:一切变量和函数都可以并且只能通过__proto__去找它所在原型链上的属性与方法
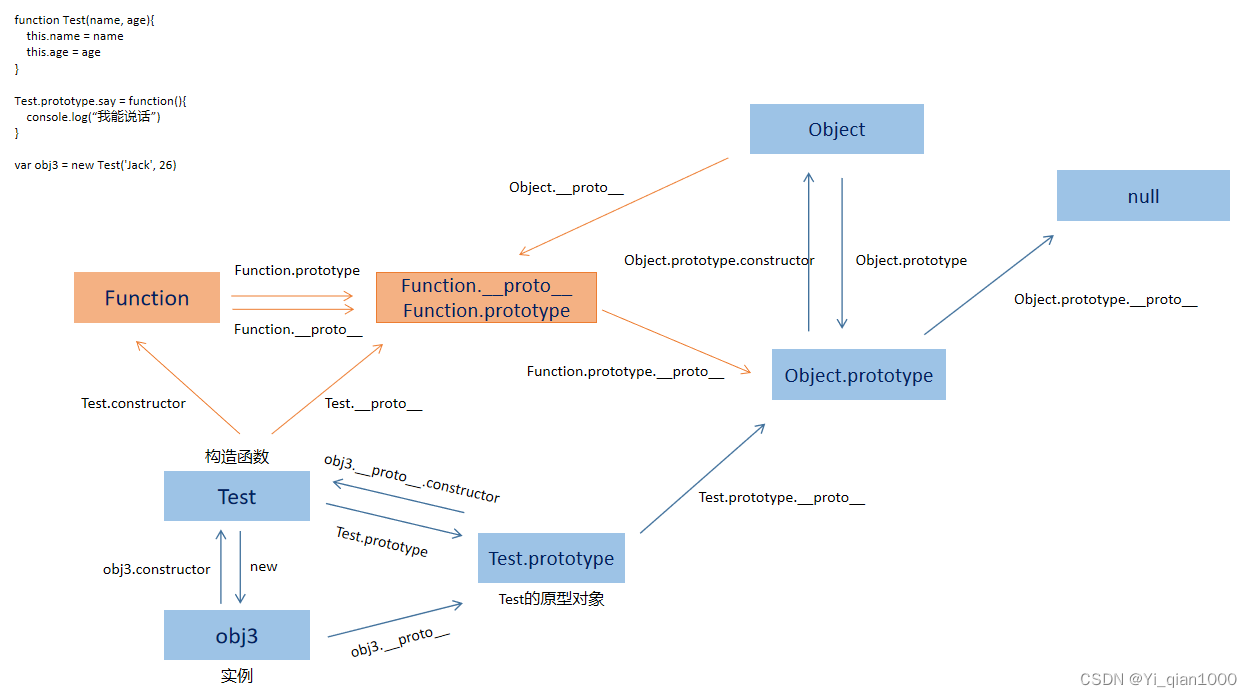
原型链需要注意的点
看上图可以发现
- 函数(构造函数)也可以通过__proto__去找到原型对象也就是Function.prototype
Function.__proto__ === Function.prototype
练习题
typescript
function Test(name, age){
this.name = name
this.age = age
}
var obj = new Test('Jack', 26)
Object.prototype.price = 2000
Function.prototype.price = 300
console.log(Test.price) // 300
console.log(obj.price) // 2000
原型链继承的方式
typescript
function Person (name, age) {
this.name = name
this.age = age
}
// person的子类
function Student (name, age, score) {
Person.call(this, name, age)
this.score = score
}
// 1. 根据原型创建一个空对象
Student.prototype = Object.create(Person.prototype) // Object.create(Person.prototype)创建的对象是一个以Person.prototype为原型的对象
// 2. 修改Student的prototype的constructor
Student.prototype.constructor = Student // 继承
// 3.更新tostring方法
Student.prototype[Symbol.toStringTag] = Student.name
let s1 = new Student('wangwu', 18, 100)
console.log('s1.__proto__', s1.__proto__)
console.log('s1.__proto__.__proto__', s1.__proto__.__proto__)
console.log('Person.prototype', Person.prototype)
console.log('Object.prototype.toString.call(s1)', Object.prototype.toString.call(s1));
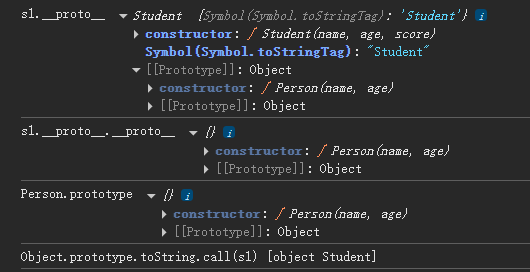