需要提前下好ffmpeg
python
import os
import fnmatch
import subprocess
'''
Bilibili缓存的视频,*280.m4s结尾的是音频文件,*050.m4s结尾的是视频,删除16进制下前9个0,即为正常音/视频
使用os.walk模块,遍历每一个目录,对每个子目录下存在的以上两个文件进行修改
去掉前九个0以后,输出到新的目录output
利用ffmpeg合成新的MP4视频到output目录的outputVideo中,作为最终转换的视频
'''
def conVert(path,outpath):
#需要进行合成的m4s文件列表
mp3lt = []
mp4lt = []
for root,dirs,files in os.walk(path):#对目录进行遍历
#root表示当前的根目录,dirs表示当前目录下所有文件夹,files表示当前目录下所有文件
dirs[:] = [d for d in dirs if d!="output"]#跳过output目录的遍历
if '.a' in files:
#为了提高效率,已经转换过的视频,在视频所处目录下添加一个".a"的空文件,作为标记
#不存在".a"文件的目录,两个m4s后缀的文件分别加入mp3lt和mp4lt中,代表这个视频还没有进行过视频转换,反之亦然
print("目录{}已进行过视频格式转换".format(root))
dirs[:] = []#files是当前目录下的所有文件的列表,若列表中存在".a",则表示不需要进行视频转换,dirs清空,直至遍历下一个目录时重置
continue #跳过
for f in files:#对此次遍历所处的目录下的所有文件进行遍历
if fnmatch.fnmatch(f,'*280.m4s'):#若存在音频文件
mp3lt.append(f)#添加进转换列表中
fn_mp3 = os.path.join(root,f) #去除9个字节后的文件保存路径
with open(fn_mp3,'rb') as fmp3:
fmp3.read(9) #读取前9个字节
remaining_content = fmp3.read() #保留剩余的字节
with open(os.path.join(outpath,f),'wb') as fmp3:
fmp3.write(remaining_content) #将剩余的字节写入新的文件中
if fnmatch.fnmatch(f,'*050.m4s'):#若存在视频文件
mp4lt.append(f)#添加进转换列表中
fn_mp4 = os.path.join(root,f) #去除9个字节后的文件保存路径
with open(fn_mp4,'rb') as fmp4:
fmp4.read(9) #读取前9个字节
remaining_content = fmp4.read() #保留剩余的字节
with open(os.path.join(outpath,f),'wb') as fmp4:
fmp4.write(remaining_content) #将剩余的字节写入新的文件中
with open(os.path.join(root,'.a'),'wb') as a:#创建空文件作为标记
pass
print(f"成功创建标记{os.path.join(root,'.a')}")
return mp3lt,mp4lt#返回列表
def delete_file(path):#删除".a"标记
delete_counted = 0
for root,dirs,files in os.walk(path):
dirs[:] = [d for d in dirs if d!="output"]
if '.a' in files:
os.remove(os.path.join(root,'.a'))
delete_counted += 1
print(f"已删除:{os.path.join(root,'.a')}")
dirs[:] = []
continue
else:
print(f"{os.path.join(root,'.a')}文件不存在")
continue
print(f"已删除数量:{delete_counted}")
def convert_video(mp3lt,mp4lt,outpath):#对m4s文件进行合成
conVert_conuts = len(mp4lt)
output = os.path.join(outpath+'\outputVideo')#最终的mp4视频写入outputVideo文件夹中
if conVert_conuts!=0 :#存在需要进行转换的视频时
for m in range(conVert_conuts):#ffmpeg合成视频的命令
command = [
"ffmpeg",
"-i",os.path.join(outpath,mp4lt[m]),
"-i",os.path.join(outpath,mp3lt[m]),
"-codec",
"copy",
os.path.join(output,mp4lt[m].split(".")[0]+'.mp4')
]
#print(command)
subprocess.run(command)
else:
print("当前没有需要转换的视频")
def convert(path,outpath):
mp3lt,mp4lt = conVert(path,outpath)
print(mp3lt,mp4lt)
convert_video(mp3lt,mp4lt,outpath)
#需要转换时执行convert方法,需要删除".a"标记重新转换视频时,先执行delete_file方法再执行convert方法
def main():
path="D:\BiliDownload\VIdeosBiliBili" #bilibili缓存路径
outpath = "D:\BiliDownload\VIdeosBiliBili\output" #转换的视频保存路径
convert(path,outpath)
#delete_file(path)
main()
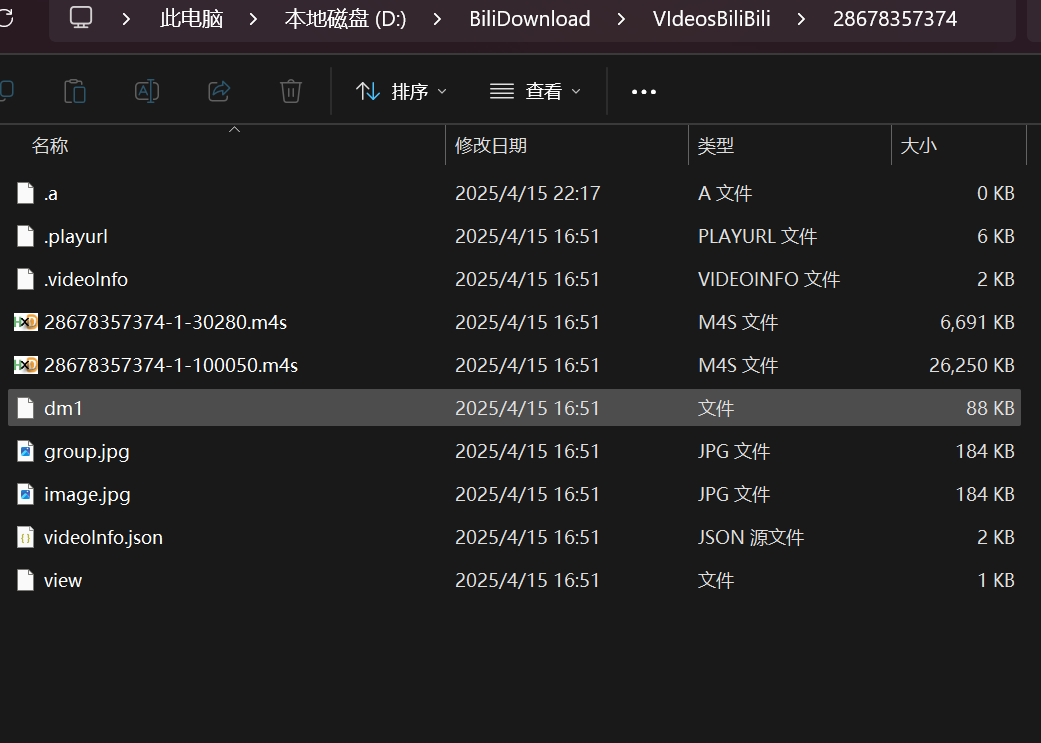
转换结束后可以把output目录下的m4s全部删除掉,或者在python代码中实现