EF框架
组件下载
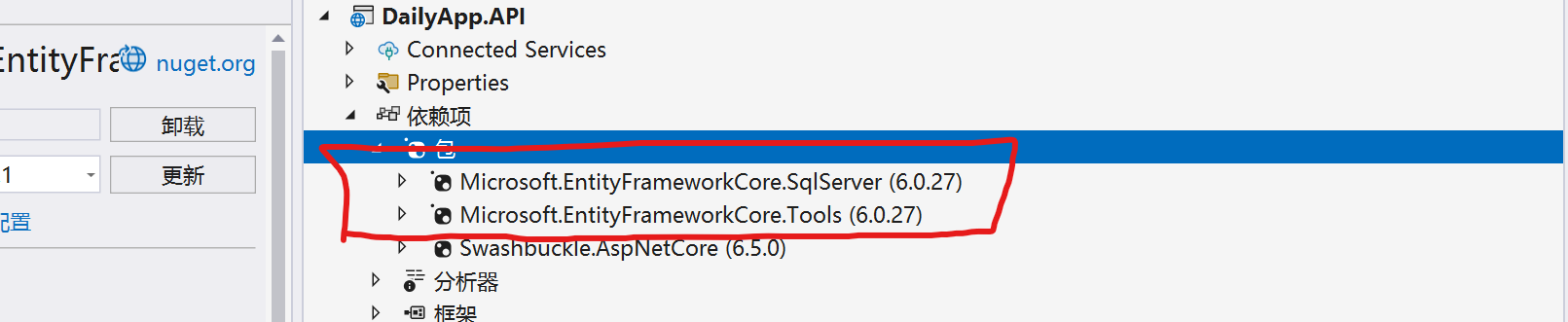
特点
1.DBFirst:数据库优先,从数据库生成Class
2.CodeFirst:代码优先,由实体类生成数据库表结构
3.ModelFirst:模型优先,通过数据库可视化设计器设计数据库,同时生成实体类
CodeFirst
- 建立数据模型
[Table("AccountInfo")]//定义表名
public class AccountInfo
{
[Key]//主键自增
public int AccountId { get; set; }
public int Name { get; set; }
public int Account { get; set; }
public int Password { get; set; }
}
架构缺失
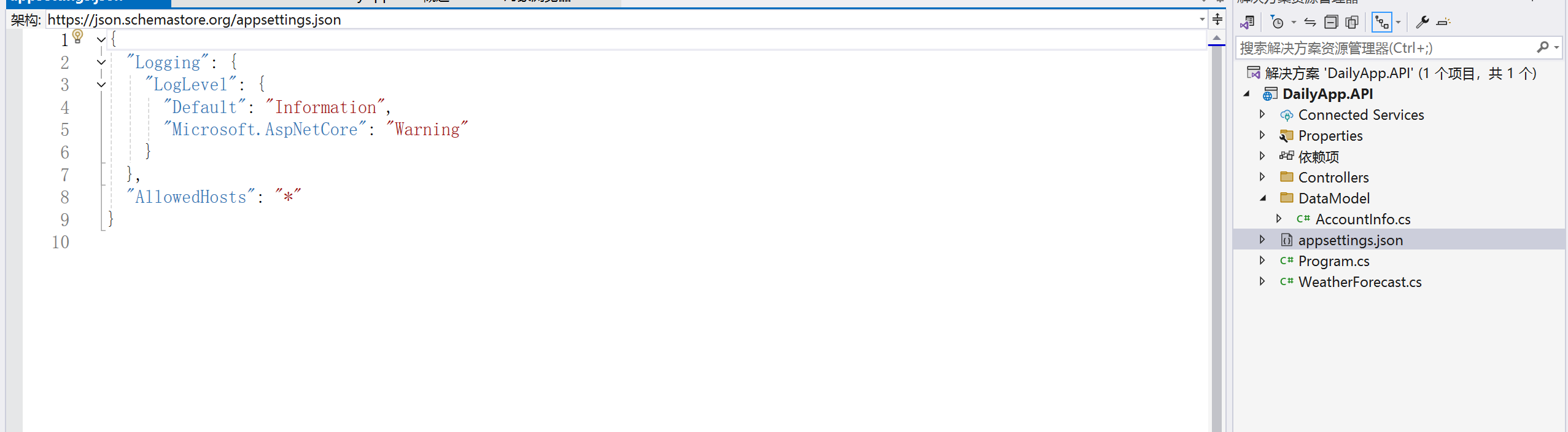
https://json.schemastore.org/appsettings.json
2.appsettings.json中配置连接字符串
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft.AspNetCore": "Warning"
}
},
"AllowedHosts": "*",
"ConnectionStrings": { "ConnStr": "server=LAPTOP-F352TNCB\\SQLEXPRESS;uid=sa;pwd=123456;database=DailyApp" }
}
3.编写数据库上下文类
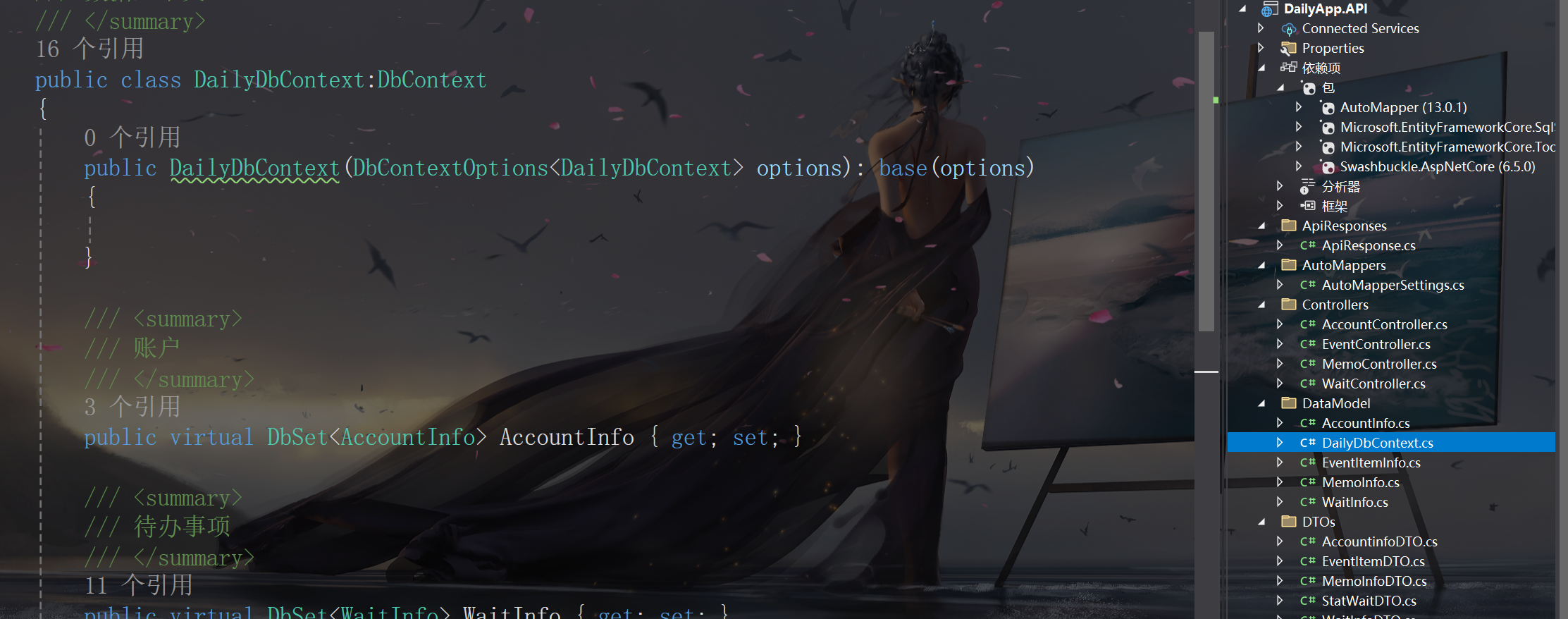
/// <summary>
/// 数据上下文
/// </summary>
public class DailyDbContext:DbContext
{
public DailyDbContext(DbContextOptions<DailyDbContext> options): base(options)
{
}
/// <summary>
/// 账户
/// </summary>
public virtual DbSet<AccountInfo> AccountInfo { get; set; }
}
4.Program中配置注册数据库上下文
builder.Services.AddDbContext<DailyDbContext>(m => m.UseSqlServer(builder.Configuration.GetConnectionString("ConnStr")));
迁移命令
1.点击程序包管理控制台
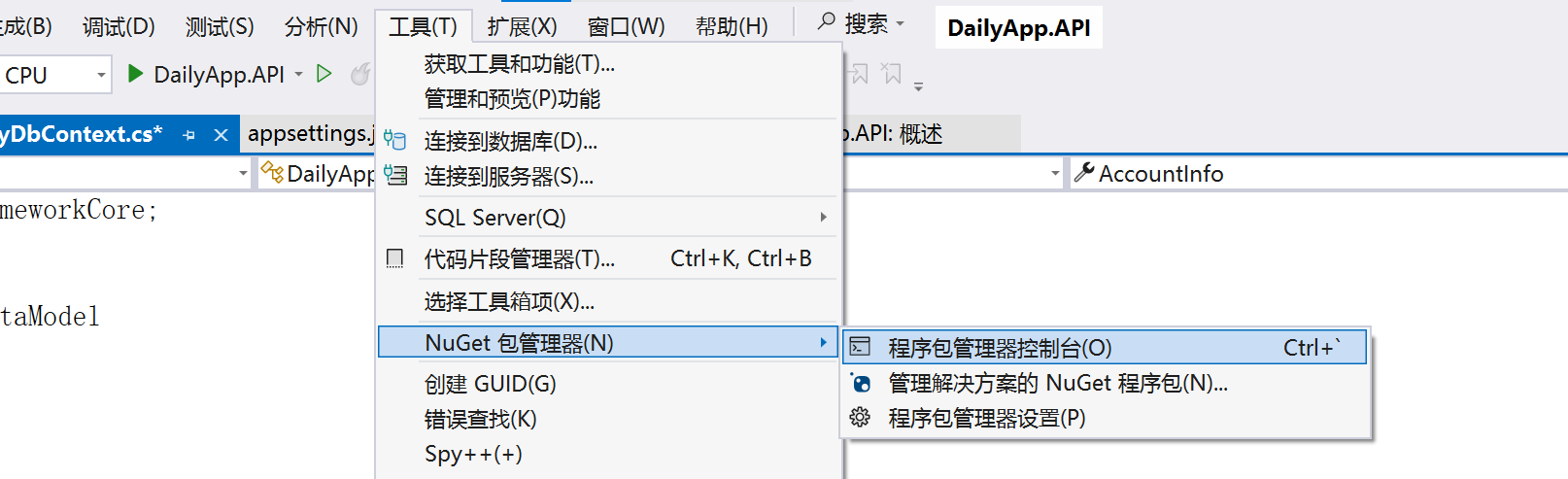
2.输入两行命令
add-migration liubei
//报错请检查连接字符串是否正确
update-database
实际运用dbcontext
[Route("api/[controller]/[action]")]
[ApiController]
public class AccountController : ControllerBase
{
/// <summary>
/// 数据库上下文
/// </summary>
private readonly DailyDbContext db;
private readonly IMapper mapper;
/// <summary>
/// 构造函数
/// </summary>
/// <param name="db"></param>
public AccountController(DailyDbContext _db,IMapper _mapper)
{
db = _db;
mapper= _mapper;
}
[HttpPost]
public IActionResult Reg(AccountinfoDTO accountinfoDTO)
{
ApiResponse res=new ApiResponse();
//业务
try
{
//账号是否存在(没考虑高并发)
var dbAccount=db.AccountInfo.Where(t => t.Account == accountinfoDTO.Account).FirstOrDefault();
if (dbAccount != null)
{
res.ResultCode = -1;
res.Msg = "对不起,账号被注册";
return Ok(res);
}
//如果不存在添加账号
//AccountInfo accountInfo=new AccountInfo() { Name= accountinfoDTO.Name,Account= accountinfoDTO.Account,Password=accountinfoDTO.Password };
AccountInfo accountInfo =mapper.Map<AccountInfo>(accountinfoDTO);
db.AccountInfo.Add(accountInfo);
//保存受影响行数
int result = db.SaveChanges();
if (result == 1)
{
res.ResultCode = 1;
res.Msg = "注册成功";
}
else
{
res.ResultCode = -99;
res.Msg = "注册失败";
}
}
catch (Exception)
{
res.ResultCode = -99;
res.Msg = "异常";
}
return Ok(res);
}
[HttpGet]
public IActionResult Login(string account,string password)
{
ApiResponse apiResponse = new ApiResponse();
try
{
var dbAccount = db.AccountInfo.Where(t => t.Account == account && t.Password == password).FirstOrDefault();
if (dbAccount == null)
{
apiResponse.ResultCode = -1;
apiResponse.Msg = "登陆失败,账号或密码错误";
return Ok(apiResponse);
}
apiResponse.ResultCode = 1;
apiResponse.Msg = "登陆成功";
apiResponse.ResultData = dbAccount;
return Ok(apiResponse);
}
catch (Exception)
{
apiResponse.ResultCode = -99;
apiResponse.Msg = "登陆失败,异常";
return Ok(apiResponse);
}
}
}