目的:注册自定义的Servlet、Filter、Listener组件到springboot内嵌的Servlet容器,让它们发挥自己的作用
使用Spring Bean 注册Java Web三大组件
路径扫描整合javaweb三大组件
1.三大组件上添加对应注解
在对应组件上分别使用@WebServlet("/annotationServlet")注解来映射"/annotationServlet"请求的Servlet类,
使用@WebFilter(value = {"/antionLogin","/antionMyFilter"})注解来映射"/antionLogin"和"/antionMyFilter"请求的Filter类,
使用@WebListener注解来标注Listener类。
java
@WebServlet("/annotationServlet")
public class MyServlet extends HttpServlet {
java
@WebFilter(value = {"/antionLogin","/antionMyFilter"})
public class MyFilter implements Filter {
java
@WebListener
public class MyListener implements ServletContextListener {
2.主程序启动类上添加@ServletComponentScan注解,开启基于注解方式的Servlet组件扫描支持
java
@ServletComponentScan
@SpringBootApplication
public class MyChapter05Application
3.测试
http://localhost:8080/annotationServlet
http://localhost:8080/antionLogin
http://localhost:8080/antionMyFilter
使用RegistrationBean注册Java Web三大件
使用组件注册方式整合Servlet
1.创建component子包及一个自定义Servlet类MyServlet,使用@Component注解将MyServlet类作为组件注入Spring容器。MyServlet类继承自HttpServlet,通过HttpServletResponse对象向页面输出"hello MyServlet"。
java
@Component
public class MyServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
this.doPost(req, resp);
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
resp.getWriter().write("hello MyServlet");
}
}
2.在config子包下创建Servlet组件配置类ServletConfig,来注册Servlet组件
java
@Configuration
public class ServletConfig {
// 注册servlet组件
@Bean
public ServletRegistrationBean getServlet(MyServlet myServlet) {
ServletRegistrationBean registrationBean =
new ServletRegistrationBean(myServlet, "/myServlet");
return registrationBean;
}
}
3.重启项目,启动成功后,在浏览器上访问http://localhost:8080/myServlet
使用组件注册方式整合Filter
1.在component包下创建一个自定义Filter类MyFilter,使用@Component注解将当前MyFilter类作为组件注入到Spring容器中。MyFilter类实现Filter接口,并重写了init()、doFilter()和destroy()方法,在doFilter()方法中向控制台打印了"hello MyFilter"字符串。
java
@Component
public class MyFilter implements Filter {
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
System.out.println("hello my filter!");
chain.doFilter(request, response);
}
}
2.向Servlet组件配置类注册自定义Filter类
java
@Bean
public FilterRegistrationBean getFilter(MyFilter filter){
FilterRegistrationBean registrationBean = new FilterRegistrationBean(filter);
//过滤出请求路径"/toLoginPage","/myFilter",对它们特殊处理,也就是执行Filter中的方法。
registrationBean.setUrlPatterns(Arrays.asList("/toLoginPage","/myFilter"));
return registrationBean;
}
3、项目启动成功后,在浏览器上访问http://localhost:8080/myFilter,查看控制台打印效果

使用组件注册方式整合Listener
1.创建一个类MyListener
java
@Component
public class MyListener implements ServletContextListener {
@Override
public void contextInitialized(ServletContextEvent servletContextEvent) {
System.out.println("contextInitialized ...");
}
@Override
public void contextDestroyed(ServletContextEvent servletContextEvent) {
System.out.println("contextDestroyed ...");
}
}
2.向Servlet组件配置类注册自定义Listener类
java
@Bean
public ServletListenerRegistrationBean getServletListener(MyListener myListener){
ServletListenerRegistrationBean registrationBean =
new ServletListenerRegistrationBean(myListener);
return registrationBean;
}
3.项目启动成功后查看控制台打印效果
contextInitialized ...
4、正常关闭(保证是正常启动的)
步骤:
①`pom.xml`添加依赖:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
②配置文件`application.properties`:
#开启所有的端点
management.endpoints.web.exposure.include=*
#启用shutdown
management.endpoint.shutdown.enabled=true
③执行关闭请求(POST):
在开发者工具的console输入如下代码,然后按回车:
fetch(new Request('http://localhost:8081/actuator/shutdown',{method:'POST'})).then((resp)=>{console.log(resp)})
结果:
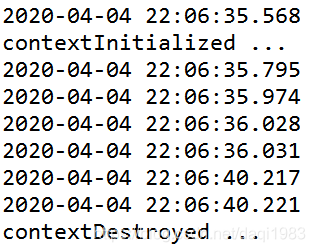