1.定义
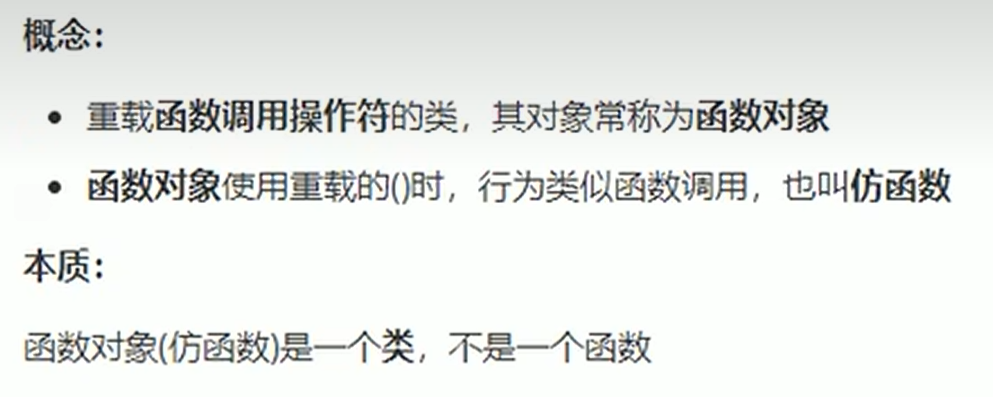
2.特点
、
1.解释第一句
cpp
#include<stdio.h>
using namespace std;
#include<string>
#include<map>
#include <iostream>
class print
{
public:
void operator()(string s)
{
cout << s << endl;
}
};
int main()
{
print print;
print("hello world");
return 0;
}
2.解释第二句
可以有自己的状态:指的是他和普通的函数不同,例如他可以统计自己调用了几次。
也就是说,它可以向函数一样被调用,但是却拥有类的功能,他是类和函数的结合。
cpp
#include<stdio.h>
using namespace std;
#include<string>
#include<map>
#include <iostream>
class print
{
public:
print()
{
this->count = 0;
}
void operator()(string s)
{
cout << s << endl;
this->count++;
}
int count;
};
int main()
{
print print;
print("hello world");
print("hello world");
print("hello world");
print("hello world");
print("hello world");
cout << print.count << endl;
return 0;
}
3.解释第三句
cpp
#include<stdio.h>
using namespace std;
#include<string>
#include<map>
#include <iostream>
class print
{
public:
print()
{
this->count = 0;
}
void operator()(string s)
{
cout << s << endl;
this->count++;
}
int count;
};
void test(print p, string s)
{
p(s);
}
void test01()
{
print print;
test(print, "C++");
}
int main()
{
test01();
return 0;
}
函数对象不仅可以作为函数参数,还可以再调用的函数中去使用自己重载过的小括号
具体见
void test(print p, string s)
{
p(s);
}
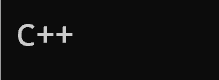
3.内建函数对象
1.定义
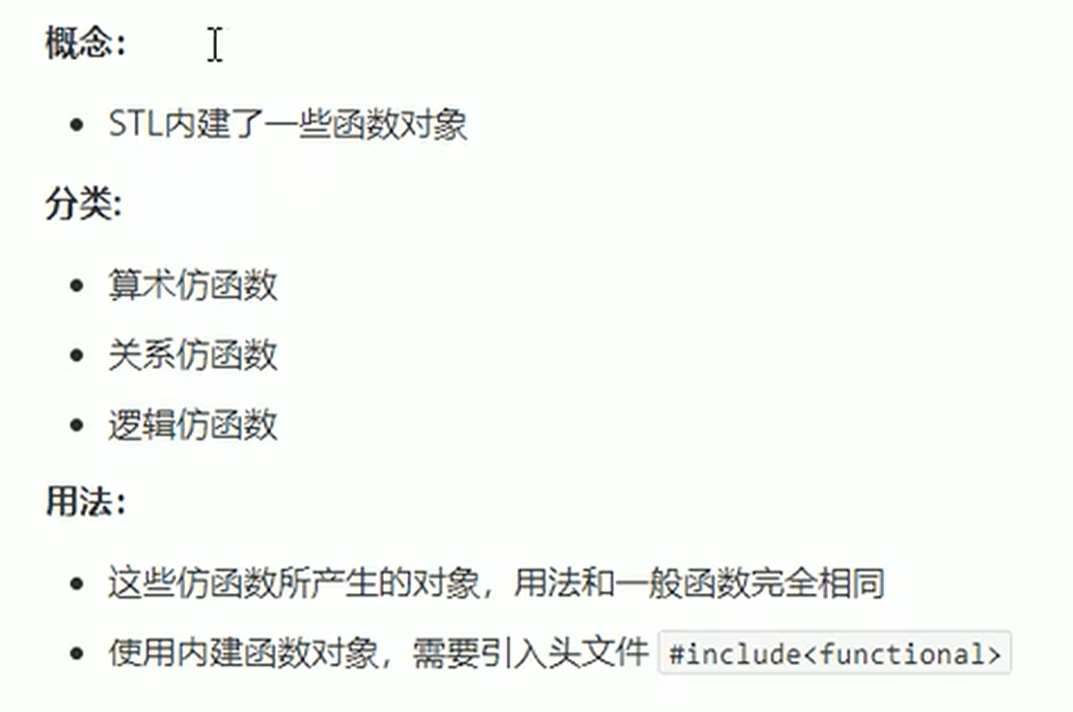
2.内建函数对象-算数仿函数
1.函数原型
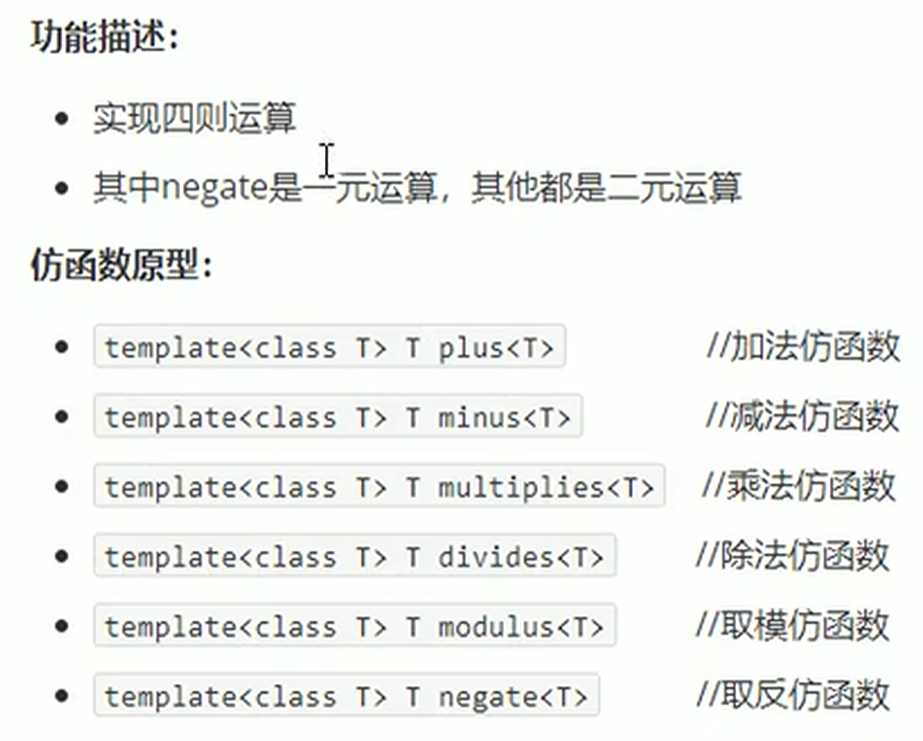
2.取反仿函数(一元仿函数)
cpp
#include<stdio.h>
using namespace std;
#include<string>
#include<vector>
#include<functional>
#include <iostream>
int main()
{
negate<int>n;
cout << n(10) << endl;
return 0;
}
3.加法仿函数(二元仿函数)
cpp
#include<stdio.h>
using namespace std;
#include<string>
#include<vector>
#include<functional>
#include <iostream>
int main()
{
plus<int>n;
cout << n(10,20) << endl;
return 0;
}
注意:plus模板参数列表中只需要有一个int就行,是为了防止出现两个不同类型的数据相加
4.内建函数对象-关系仿函数
1.函数原型
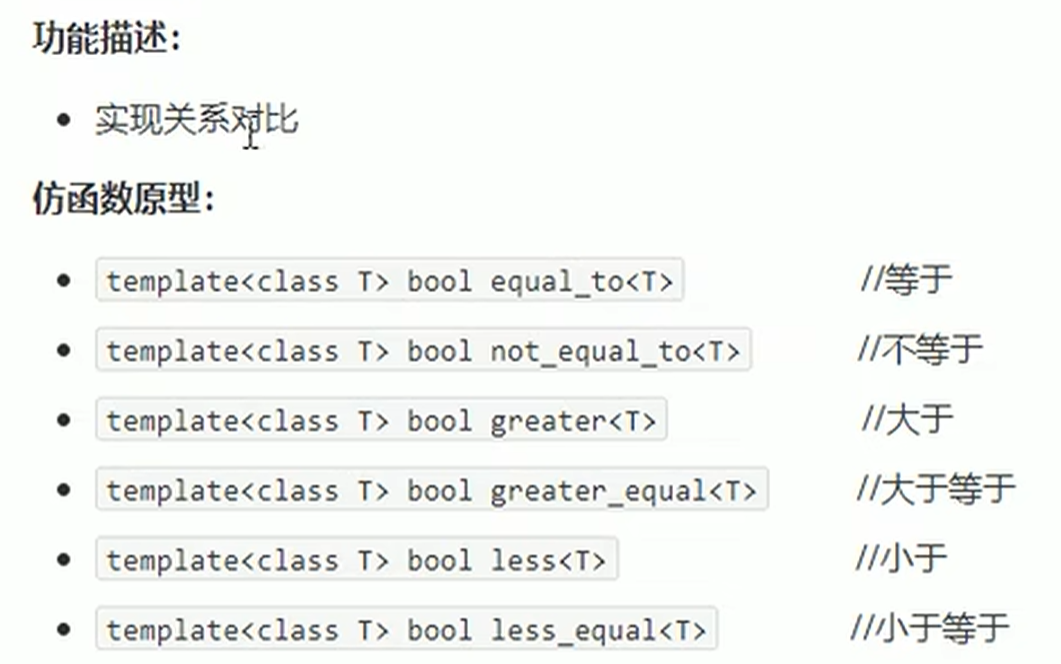
2.代码
cpp
#include<stdio.h>
using namespace std;
#include<string>
#include<vector>
#include<functional>
#include<algorithm>
#include <iostream>
int main()
{
vector<int> v;
v.push_back(1);
v.push_back(5);
v.push_back(3);
v.push_back(2);
sort(v.begin(), v.end(), greater<int>());
for (int i = 0; i < v.size(); i++)
{
cout << v[i] << endl;
}
return 0;
}
5.内建函数对象-逻辑仿函数
1.函数原型
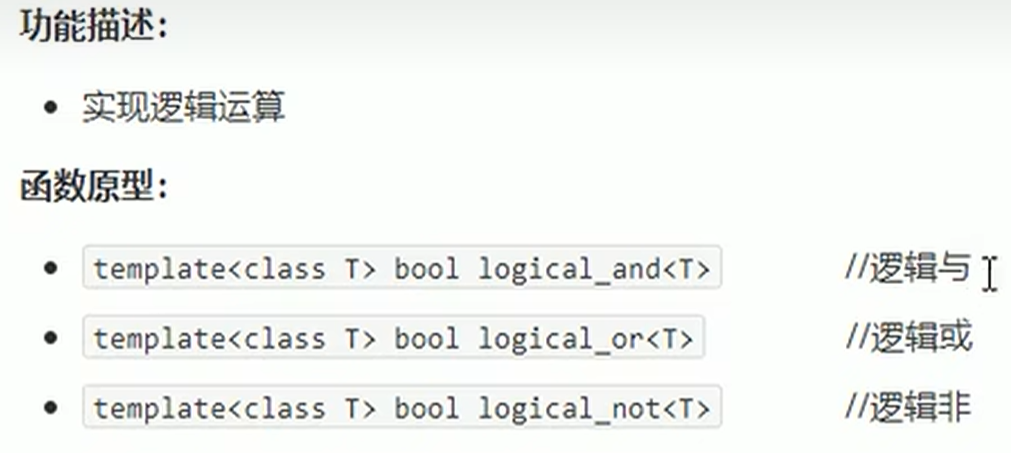
2.代码
cpp
#include<stdio.h>
using namespace std;
#include<string>
#include<vector>
#include<functional>
#include<algorithm>
#include <iostream>
int main()
{
vector<int> v;
v.push_back(1);
v.push_back(5);
v.push_back(3);
v.push_back(2);
vector<int> v1;
v1.resize(v.size());
transform(v.begin(), v.end(),v1.begin(), logical_not<bool>());
for (int i = 0; i < v.size(); i++)
{
cout << v[i] << " ";
}
cout << endl;
for (int i = 0; i < v1.size(); i++)
{
cout << v1[i] << " ";
}
cout << endl;
return 0;
}
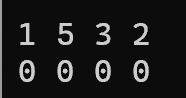
其中用到了搬运函数transform,需要提前给他开辟相应的空间
v1.resize(v.size());
transform(v.begin(), v.end(),v1.begin(), logical_not<bool>());
4.注意
逻辑仿函数实际应用较少,了解即可