一,Spring介绍
什么是Spring
-
轻量级:Spring 是非侵入性的 - 基于 Spring 开发的应用中的对象可以不依赖于 Spring 的 API
-
依赖注入(DI --- dependency injection、IOC)
-
面向切面编程(AOP --- aspect oriented programming)
-
容器: Spring 是一个容器, 因为它包含并且管理应用对象的生命周期
-
框架: Spring 实现了使用简单的组件配置组合成一个复杂的应用.在 Spring 中可以使用 XML 和Java 注解组合这些对象
-
一站式:在 IOC 和 AOP 的基础上可以整合各种企业应用的开源框架和优秀的第三方类库 (实际上 Spring 自身也提供了展现层的SpringMVC 和 持久层的 Spring JDBC)
二,搭建Spring项目
1.首先使用spring框架要创建一个Maven项目.
在pom文件下导入所需依赖,在resource文件中编写配置文件
2.导入spring需要的包
java
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>4.3.17.RELEASE</version>
</dependency>
</dependencies>
这里导入的是spirngmvc的包,因为maven可以自动加载对应的依赖,所以会自动的把spring所需要的包都导入成功,比较方便,也可以分别去导入spring的每个包,那样会麻烦一些
3.编写配置文件
在resource文件夹中创建applicationContext.xml文件
java
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd">
</beans>
4.让我们测试一下
创建类
java
package com.haina.spring;
public class HelloService {
public void sayHello(){
System.out.println("hello spring");
}
}
编写配置文件
java
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd">
<bean id="hello" class="com.haina.spring.HelloService"></bean>
</beans>
创建测试类
java
package com.haina.spring;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class SpringTest {
public static void main(String[] args) {
String conf="applicationContext.xml";
ApplicationContext context=new ClassPathXmlApplicationContext(conf);
HelloService h1=(HelloService)context.getBean("hello");
h1.sayHello();
}
}
之后的运行结果:
java
D:\Java\jdk21\bin\java.exe "-javaagent:D:\Java\idea2024\IntelliJ IDEA 2024.1\lib\idea_rt.jar=51154:D:\Java\idea2024\IntelliJ IDEA 2024.1\bin" -Dfile.encoding=UTF-8 -Dsun.stdout.encoding=UTF-8 -Dsun.stderr.encoding=UTF-8 -classpath D:\Java\SpringTest\target\classes;C:\Users\CZY\.m2\repository\org\springframework\spring-webmvc\4.3.17.RELEASE\spring-webmvc-4.3.17.RELEASE.jar;C:\Users\CZY\.m2\repository\org\springframework\spring-aop\4.3.17.RELEASE\spring-aop-4.3.17.RELEASE.jar;C:\Users\CZY\.m2\repository\org\springframework\spring-beans\4.3.17.RELEASE\spring-beans-4.3.17.RELEASE.jar;C:\Users\CZY\.m2\repository\org\springframework\spring-context\4.3.17.RELEASE\spring-context-4.3.17.RELEASE.jar;C:\Users\CZY\.m2\repository\org\springframework\spring-core\4.3.17.RELEASE\spring-core-4.3.17.RELEASE.jar;C:\Users\CZY\.m2\repository\commons-logging\commons-logging\1.2\commons-logging-1.2.jar;C:\Users\CZY\.m2\repository\org\springframework\spring-expression\4.3.17.RELEASE\spring-expression-4.3.17.RELEASE.jar;C:\Users\CZY\.m2\repository\org\springframework\spring-web\4.3.17.RELEASE\spring-web-4.3.17.RELEASE.jar com.haina.spring.SpringTest
4月 21, 2025 7:18:59 下午 org.springframework.context.support.ClassPathXmlApplicationContext prepareRefresh
信息: Refreshing org.springframework.context.support.ClassPathXmlApplicationContext@3d012ddd: startup date [Mon Apr 21 19:18:59 CST 2025]; root of context hierarchy
4月 21, 2025 7:18:59 下午 org.springframework.beans.factory.xml.XmlBeanDefinitionReader loadBeanDefinitions
信息: Loading XML bean definitions from class path resource [applicationContext.xml]
hello spring
Process finished with exit code 0
三,IOC编程思想
1.什么是Ioc
Ioc---Inversion of Control,即"控制反转",不是什么技术,而是一种设计思想。在Java开发中,Ioc意味着将你设计好的对象交给容器控制,而不是传统的在你的对象内部直接控制。如何理解好Ioc呢?理解好Ioc的关键是要明确"谁控制谁,控制什么,为何是反转(有反转就应该有正转了),哪些方面反转了",那我们来深入分析一下:
2.谁控制谁,控制什么
传统Java SE程序设计,我们直接在对象内部通过new进行创建对象,是程序主动去创建依赖对象;而IoC是有专门一个容器来创建这些对象,即由Ioc容器来控制对象的创建;谁控制谁?当然是IoC 容器控制了对象;控制什么?那就是主要控制了外部资源获取(不只是对象包括比如文件等)。
3.为何是反转,哪些方面反转了
有反转就有正转,传统应用程序是由我们自己在对象中主动控制去直接获取依赖对象,也就是正转;而反转则是由容器来帮忙创建及注入依赖对象;为何是反转?因为由容器帮我们查找及注入依赖对象,对象只是被动的接受依赖对象,所以是反转;哪些方面反转了?依赖对象的获取被反转了。
四,依赖注入
1.什么是依赖注入
DI(Dependency Injection) --- IOC 的另一种表述方式:即组件以一些预先定义好的方式(例如: setter 方法)接受来自如容器的资源注入. 相对于 IOC 而言,这种表述更直接
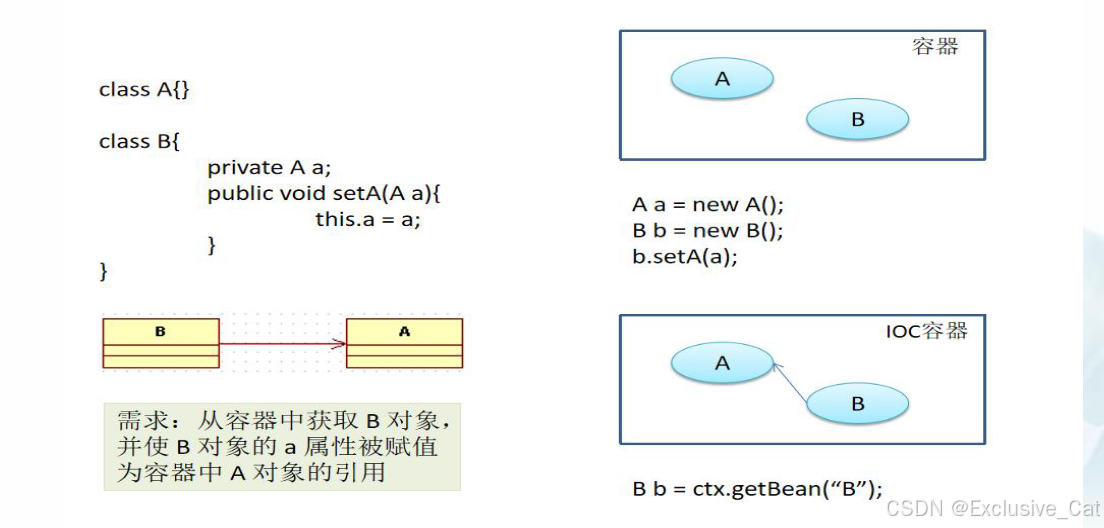
2.创建bean
在spring容器中配置bean
<bean id="hello" class="com.haina.spring.HelloService"/>
id:Bean 的名称。
• 在 IOC 容器中必须是唯一的
• 若 id 没有指定,Spring 自动将全限定性类名作为 Bean 的名字
• id 可以指定多个名字,名字之间可用逗号、分号、或空格分隔
获取bean的方式: 使用ApplicationContext类中提供的getBean方法可以通过id获取也可以通过类型获取
3.spring容器启动过程
- 加载配置文件
- spring会读取配置文件中的bean标签
- 通过bean标签的class属性的值,找到该bean对应的类
- 使用反射技术创建出该类的对象,存储到spring容器中
- spring容器我们可以理解为是一个HashMap
- 对象存储时,key是该bean的id,value是对象
4.依赖注入的方式
首先在HelloService类中加入name,age属性,再生成有参无参的构造方法和成员变量的get,set方法
java
package com.haina.spring;
public class HelloService {
private String name;
private String age;
public HelloService(){
}
public HelloService(String age, String name) {
this.age = age;
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAge() {
return age;
}
public void setAge(String age) {
this.age = age;
}
public void sayHello(){
System.out.println("hello spring"+name+age);
}
}
接下来在配置文件中加入两种注入:
1.settter注入
java
<bean id="hello" class="com.haina.spring.HelloService">
<property name="name" value="aaa"/>
<property name="age" value="16"/>
</bean>
2.构造方法注入
java
<bean id="hello2" class="com.haina.spring.HelloService">
<constructor-arg name="name" value="aaa"/>
<constructor-arg name="age" value="16"/>
</bean>
构造方法可以使用参数名、下标、类型三种形式来注入
最后在sayHello方法中修改一下输出的内容,将两个成员变量也输出,结果为:
java
D:\Java\jdk21\bin\java.exe "-javaagent:D:\Java\idea2024\IntelliJ IDEA 2024.1\lib\idea_rt.jar=51821:D:\Java\idea2024\IntelliJ IDEA 2024.1\bin" -Dfile.encoding=UTF-8 -Dsun.stdout.encoding=UTF-8 -Dsun.stderr.encoding=UTF-8 -classpath D:\Java\SpringTest\target\classes;C:\Users\CZY\.m2\repository\org\springframework\spring-webmvc\4.3.17.RELEASE\spring-webmvc-4.3.17.RELEASE.jar;C:\Users\CZY\.m2\repository\org\springframework\spring-aop\4.3.17.RELEASE\spring-aop-4.3.17.RELEASE.jar;C:\Users\CZY\.m2\repository\org\springframework\spring-beans\4.3.17.RELEASE\spring-beans-4.3.17.RELEASE.jar;C:\Users\CZY\.m2\repository\org\springframework\spring-context\4.3.17.RELEASE\spring-context-4.3.17.RELEASE.jar;C:\Users\CZY\.m2\repository\org\springframework\spring-core\4.3.17.RELEASE\spring-core-4.3.17.RELEASE.jar;C:\Users\CZY\.m2\repository\commons-logging\commons-logging\1.2\commons-logging-1.2.jar;C:\Users\CZY\.m2\repository\org\springframework\spring-expression\4.3.17.RELEASE\spring-expression-4.3.17.RELEASE.jar;C:\Users\CZY\.m2\repository\org\springframework\spring-web\4.3.17.RELEASE\spring-web-4.3.17.RELEASE.jar com.haina.spring.SpringTest
4月 21, 2025 8:47:21 下午 org.springframework.context.support.ClassPathXmlApplicationContext prepareRefresh
信息: Refreshing org.springframework.context.support.ClassPathXmlApplicationContext@3d012ddd: startup date [Mon Apr 21 20:47:21 CST 2025]; root of context hierarchy
4月 21, 2025 8:47:22 下午 org.springframework.beans.factory.xml.XmlBeanDefinitionReader loadBeanDefinitions
信息: Loading XML bean definitions from class path resource [applicationContext.xml]
hello springaaa16
Process finished with exit code 0
5.引用其他的bean
• 组成应用程序的 Bean 经常需要相互协作以完成应用程序的功能. 要使 Bean 能够相互访问, 就必须在 Bean 配置文件中指定对 Bean 的引用
• 在 Bean 的配置文件中, 可以通过 <ref> 元素或 ref属性为 Bean 的属性或构造器参数指定对 Bean 的引用.
• 也可以在属性或构造器里包含 Bean 的声明, 这样的Bean 称为内部 Bean