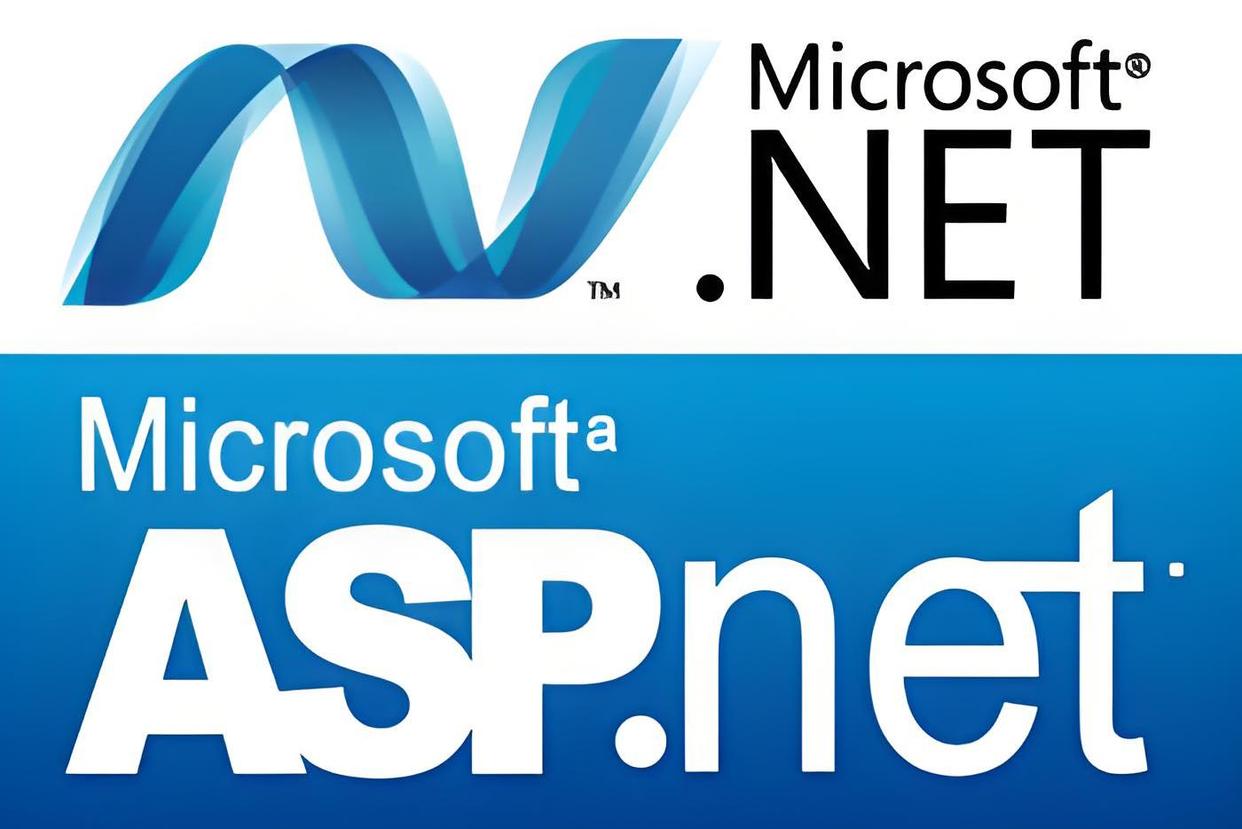
提供一个完整的 ASP.NET MVC 实现增删改查(CRUD)操作的示例。该示例使用 SQL Server 数据库,以一个简单的 Product
实体为例。
步骤 1:创建 ASP.NET MVC 项目
首先,在 Visual Studio 中创建一个新的 ASP.NET MVC 项目。
步骤 2:定义数据模型
在 Models
文件夹下创建 Product.cs
文件:
csharp
using System.ComponentModel.DataAnnotations;
namespace YourNamespace.Models
{
public class Product
{
public int Id { get; set; }
[Required]
public string Name { get; set; }
[Required]
public decimal Price { get; set; }
}
}
步骤 3:创建数据库上下文
在 Models
文件夹下创建 ProductContext.cs
文件:
csharp
using Microsoft.EntityFrameworkCore;
namespace YourNamespace.Models
{
public class ProductContext : DbContext
{
public ProductContext(DbContextOptions<ProductContext> options) : base(options)
{
}
public DbSet<Product> Products { get; set; }
}
}
步骤 4:配置数据库连接
在 appsettings.json
文件中添加数据库连接字符串:
json
{
"ConnectionStrings": {
"DefaultConnection": "Server=(localdb)\\mssqllocaldb;Database=YourDatabaseName;Trusted_Connection=True;MultipleActiveResultSets=true"
},
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft.AspNetCore": "Warning"
}
},
"AllowedHosts": "*"
}
步骤 5:在 Startup.cs
中配置数据库上下文
csharp
using Microsoft.EntityFrameworkCore;
using YourNamespace.Models;
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<ProductContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection")));
services.AddControllersWithViews();
}
步骤 6:创建控制器
在 Controllers
文件夹下创建 ProductController.cs
文件:
csharp
using Microsoft.AspNetCore.Mvc;
using YourNamespace.Models;
using System.Linq;
namespace YourNamespace.Controllers
{
public class ProductController : Controller
{
private readonly ProductContext _context;
public ProductController(ProductContext context)
{
_context = context;
}
// 显示所有产品
public IActionResult Index()
{
var products = _context.Products.ToList();
return View(products);
}
// 创建产品视图
public IActionResult Create()
{
return View();
}
// 处理创建产品的 POST 请求
[HttpPost]
[ValidateAntiForgeryToken]
public IActionResult Create(Product product)
{
if (ModelState.IsValid)
{
_context.Products.Add(product);
_context.SaveChanges();
return RedirectToAction(nameof(Index));
}
return View(product);
}
// 编辑产品视图
public IActionResult Edit(int? id)
{
if (id == null)
{
return NotFound();
}
var product = _context.Products.Find(id);
if (product == null)
{
return NotFound();
}
return View(product);
}
// 处理编辑产品的 POST 请求
[HttpPost]
[ValidateAntiForgeryToken]
public IActionResult Edit(int id, Product product)
{
if (id != product.Id)
{
return NotFound();
}
if (ModelState.IsValid)
{
try
{
_context.Update(product);
_context.SaveChanges();
}
catch
{
if (!ProductExists(product.Id))
{
return NotFound();
}
else
{
throw;
}
}
return RedirectToAction(nameof(Index));
}
return View(product);
}
// 删除产品确认视图
public IActionResult Delete(int? id)
{
if (id == null)
{
return NotFound();
}
var product = _context.Products.Find(id);
if (product == null)
{
return NotFound();
}
return View(product);
}
// 处理删除产品的 POST 请求
[HttpPost, ActionName("Delete")]
[ValidateAntiForgeryToken]
public IActionResult DeleteConfirmed(int id)
{
var product = _context.Products.Find(id);
_context.Products.Remove(product);
_context.SaveChanges();
return RedirectToAction(nameof(Index));
}
private bool ProductExists(int id)
{
return _context.Products.Any(e => e.Id == id);
}
}
}
步骤 7:创建视图
在 Views
文件夹下创建 Product
文件夹,并在其中创建以下视图文件:
Index.cshtml
html
@model IEnumerable<YourNamespace.Models.Product>
@{
ViewData["Title"] = "Product List";
}
<h1>Product List</h1>
<p>
<a asp-action="Create">Create New</a>
</p>
<table class="table">
<thead>
<tr>
<th>
@Html.DisplayNameFor(model => model.Name)
</th>
<th>
@Html.DisplayNameFor(model => model.Price)
</th>
<th></th>
</tr>
</thead>
<tbody>
@foreach (var item in Model)
{
<tr>
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
@Html.DisplayFor(modelItem => item.Price)
</td>
<td>
@Html.ActionLink("Edit", "Edit", new { id = item.Id }) |
@Html.ActionLink("Delete", "Delete", new { id = item.Id })
</td>
</tr>
}
</tbody>
</table>
Create.cshtml
html
@model YourNamespace.Models.Product
@{
ViewData["Title"] = "Create Product";
}
<h1>Create Product</h1>
<form asp-action="Create">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="Name">Name</label>
<input asp-for="Name" class="form-control" />
<span asp-validation-for="Name" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Price">Price</label>
<input asp-for="Price" class="form-control" />
<span asp-validation-for="Price" class="text-danger"></span>
</div>
<button type="submit" class="btn btn-primary">Create</button>
</form>
<div>
<a asp-action="Index">Back to List</a>
</div>
Edit.cshtml
html
@model YourNamespace.Models.Product
@{
ViewData["Title"] = "Edit Product";
}
<h1>Edit Product</h1>
<form asp-action="Edit">
<input type="hidden" asp-for="Id" />
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="Name">Name</label>
<input asp-for="Name" class="form-control" />
<span asp-validation-for="Name" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Price">Price</label>
<input asp-for="Price" class="form-control" />
<span asp-validation-for="Price" class="text-danger"></span>
</div>
<button type="submit" class="btn btn-primary">Save</button>
</form>
<div>
<a asp-action="Index">Back to List</a>
</div>
Delete.cshtml
html
@model YourNamespace.Models.Product
@{
ViewData["Title"] = "Delete Product";
}
<h1>Delete Product</h1>
<h3>Are you sure you want to delete this?</h3>
<div>
<h4>Product</h4>
<hr />
<dl class="row">
<dt class="col-sm-2">
@Html.DisplayNameFor(model => model.Name)
</dt>
<dd class="col-sm-10">
@Html.DisplayFor(model => model.Name)
</dd>
<dt class="col-sm-2">
@Html.DisplayNameFor(model => model.Price)
</dt>
<dd class="col-sm-10">
@Html.DisplayFor(model => model.Price)
</dd>
</dl>
<form asp-action="DeleteConfirmed">
<input type="hidden" asp-for="Id" />
<button type="submit" class="btn btn-danger">Delete</button> |
<a asp-action="Index">Back to List</a>
</form>
</div>
步骤 8:运行项目
运行项目,访问 Product
控制器的 Index
动作,你将看到产品列表页面,并可以进行增删改查操作。